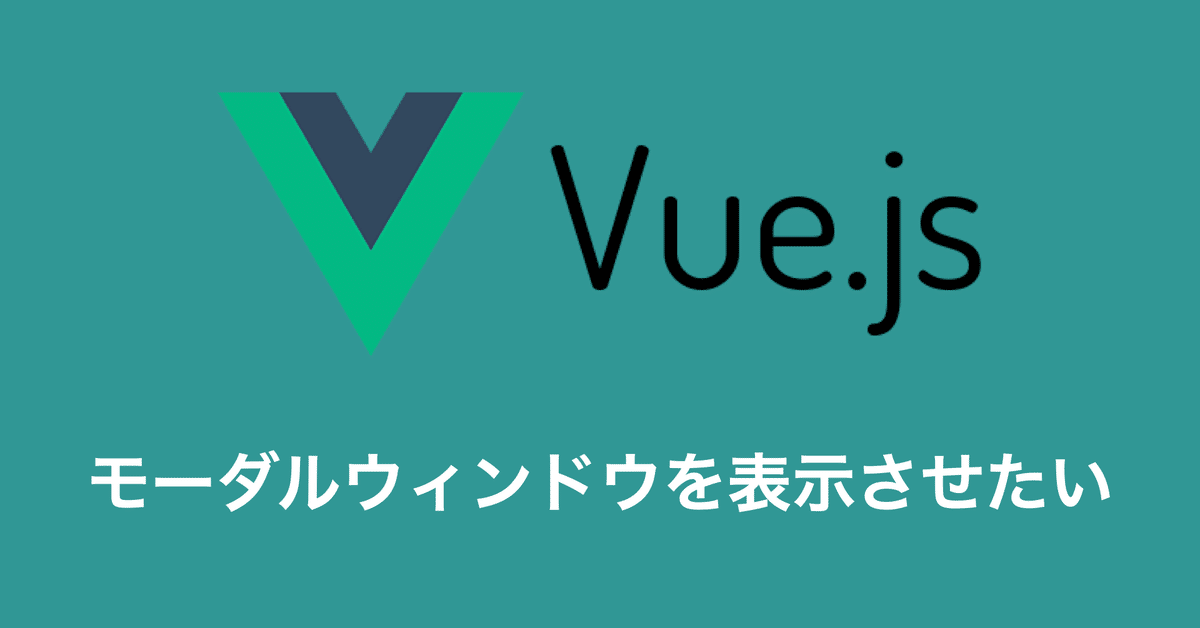
【Vue.js】モーダルウィンドウを表示する
はじめに
コピペでそのまま使えるソースコードを一番下に書いたので、必要な人はそのままお使いください。
モーダルウィンドウって何?
この意味わからずにこの記事にたどり着くのはあまりないと思いますが、モーダルウィンドウとは背景が暗くなって、出てくるこれです。
本編
まずは簡単にボタンとメソッドを作成します。
<div id="example" class="p-4">
// @clickのopenModalモーダルウィンドウを表示する
<button type="button" @click="openModal" class="btn btn-primary">モーダルウィンドウを開く</button>
</div>
<script>
var example = new Vue({
el: '#example',
data() {
return {
showContent: false,
}
},
methods: {
openModal: function() {
this.showContent = true;
}
}
})
</script>
次にモーダルウィンドウの画面を作成
<div id="example" class="p-4">
<button type="button" @click="openModal" class="btn btn-primary">モーダルウィンドウを開く</button>
// この下を追加
<open-modal v-show="showContent" @close="showContent = false"></open-modal>
</div>
// openModal押したことでshowContent = trueになった時に表示されるウィンドウ
Vue.component('open-modal', {
template: `
<div id="overlay">
<div id="content">
<div class="text-right">
<button type="button" v-on:click="$emit('close')" class="btn btn-success">閉じる <i class="fas fa-times"></i></button>
</div>
<div>
<p>開きました!!</p>
</div>
</div>
</div>
`,
});
<style>
#content {
z-index: 2;
width: 80%;
padding: 1em;
background: #fff;
}
#overlay {
z-index: 1;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
display: flex;
align-items: center;
justify-content: center;
}
</style>
// 最後に閉じるボタンのメソッドを追加します
closeModal: function() {
this.showContent = false;
}
こんな感じです。
$emit というのがありますが、これで子コンポーネントから親コンポーネントのメソッドを発火させることが出来るもので、自由に名前を付けられます。以下簡易解説です
// v-on:click="$emit('close')" で親コンポーネントの
<button type="button" v-on:click="$emit('close')" class="btn btn-success">閉じる <i class="fas fa-times"></i></button>
// 子コンポーネントで作られた 'close' というイベントを親で発火させる
<open-modal v-show="showContent" @close="showContent = false"></open-modal>
本来なら Vue.componentでscript内に書くのではなく、componentsディレクトリの中に書けば良いのですが、そのままコピペで使えるようにこのような形で書きました。
ソースコード(コピペでそのまま使えます)
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- script -->
<script src="{{ mix('js/app.js') }}"></script>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<!-- css -->
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<link href="{{ asset('css/order.css') }}" rel="stylesheet">
<link href="{{ mix('/css/app.css') }}" rel="stylesheet">
<title>Vue</title>
<style>
#content {
z-index: 2;
width: 80%;
padding: 1em;
background: #fff;
}
#overlay {
/* 要素を重ねた時の順番 */
z-index: 1;
/* 画面全体を覆う設定 */
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
/* 画面の中央に要素を表示させる設定 */
display: flex;
align-items: center;
justify-content: center;
}
</style>
</head>
<body>
<div id="example" class="p-4">
<button type="button" @click="openModal" class="btn btn-primary">モーダルウィンドウを開く</button>
<open-modal v-show="showContent" @close="showContent = false"></open-modal>
</div>
<script>
Vue.component('open-modal', {
template: `
<div id="overlay">
<div id="content">
<div class="text-right">
<button type="button" v-on:click="$emit('close')" class="btn btn-success">閉じる <i class="fas fa-times"></i></button>
</div>
<div>
<p>開きました!!</p>
</div>
</div>
</div>
`,
});
var example = new Vue({
el: '#example',
data() {
return {
showContent: false,
}
},
methods: {
openModal: function() {
this.showContent = true;
},
closeModal: function() {
this.showContent = false;
}
}
})
</script>
</body>
</html>
参考記事
この記事が気に入ったらサポートをしてみませんか?