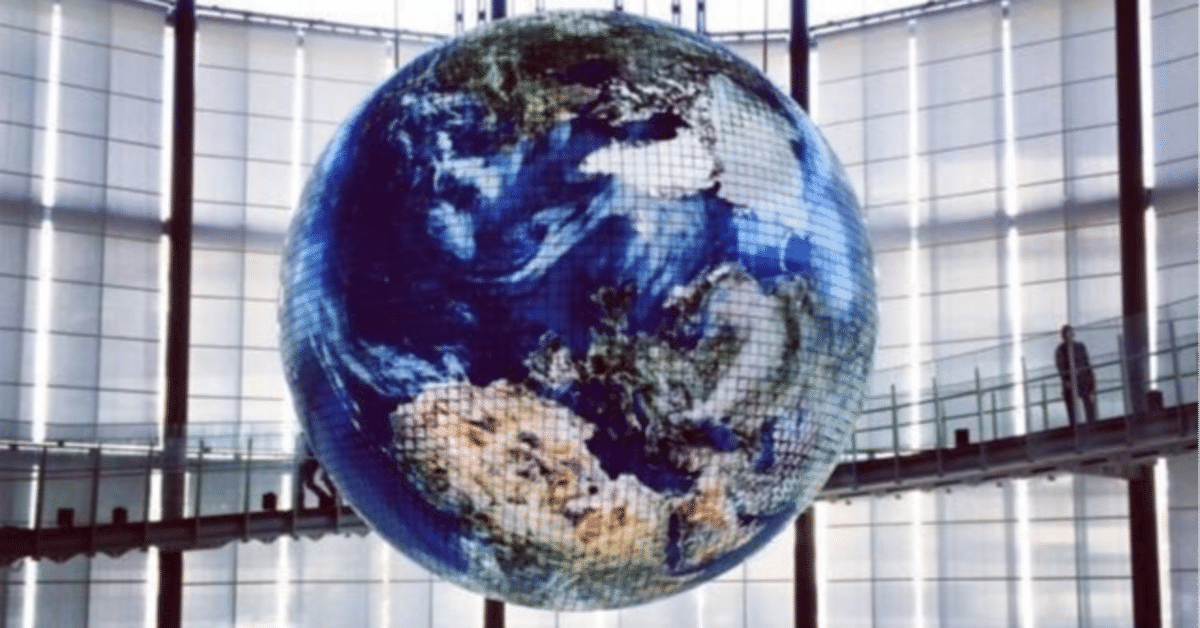
距離計算のためのHaversine公式の実装
定義
Haversine公式は、球面上の2点間の最短距離を計算するための数学的公式である。この公式は以下のように表される:
$$
\text{距離} = 2r \cdot \arcsin\left(\sqrt{\sin^2\left(\frac{\Delta \text{緯度}}{2}\right) + \cos(\text{緯度}_1) \cdot \cos(\text{緯度}_2) \cdot \sin^2\left(\frac{\Delta \text{経度}}{2}\right)}\right)
$$
ここで、
$${ \Delta \text{緯度} = \text{緯度}_2 - \text{緯度}_1 }$$
$${ \Delta \text{経度} = \text{経度}_2 - \text{経度}_1 }$$
$${ r }$$ は地球の半径(通常、6371 km)
要素の因子分解
入力:緯度と経度のペア $${ (\text{緯度}_1, \text{経度}_1 ), ( \text{緯度}_2, \text{経度}_2) }$$
出力:2点間の距離
パラメータ:地球の半径 $${ r }$$
実装の理由
地理的な位置情報から距離を計算する必要がある場合に、Haversine公式は有用です。この公式は、特に長距離にわたる計算において、高い精度を持っています。
コード実装
Pythonでの実装は以下の通りです。この実装では、緯度と経度は度数法で、距離はキロメートルで出力されます。PEP 8とsnake_caseの規則に従っています。
import math
def haversine_distance(lat1, lon1, lat2, lon2, radius=6371):
"""
Calculate the Haversine distance between two points on the earth.
Parameters:
lat1, lon1: latitude and longitude of the first point.
lat2, lon2: latitude and longitude of the second point.
radius: radius of the Earth (default is 6371 km).
Returns:
distance: distance between the two points in kilometers.
"""
# Convert latitude and longitude from degrees to radians
lat1, lon1, lat2, lon2 = map(math.radians, [lat1, lon1, lat2, lon2])
# Compute differences
dlat = lat2 - lat1
dlon = lon2 - lon1
# Haversine formula
a = math.sin(dlat / 2)**2 + math.cos(lat1) * math.cos(lat2) * math.sin(dlon / 2)**2
c = 2 * math.asin(math.sqrt(a))
# Distance
distance = radius * c
return distance
# Test the function
lat1, lon1 = 40.7128, -74.0060 # New York
lat2, lon2 = 34.0522, -118.2437 # Los Angeles
distance = haversine_distance(lat1, lon1, lat2, lon2)
distance
実装結果
New York(緯度: 40.7128, 経度: -74.0060)とLos Angeles(緯度: 34.0522, 経度: -118.2437)の間の距離は約3935.75キロメートルである。
結論
Haversine公式を用いて、指定された2つの地点間の距離を効率的に計算することができました。この実装は、地球上の任意の2点間の距離を高精度で計算する用途に適しています。
以上の情報はすべて事実に基づいており、仮説は含まれていません。この実装と計算は、地理的な距離計算における一般的なニーズに対応するものです。
by ChatGPT