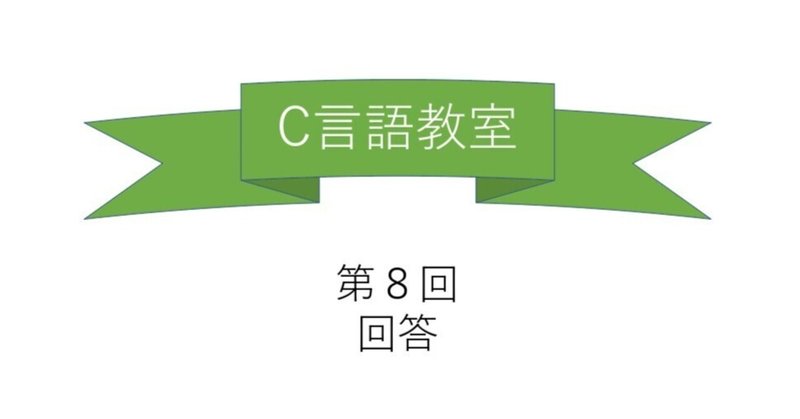
C言語教室 第8回 回答
できたできた(笑)
課題
回答
#include <string.h>
#include <stdlib.h>
void string_string(
char*const ss,
const char*const s1,
const char*const s2
)
{
if (ss == NULL)
{
return;
}
ss[0] = '\0';
if (s1 != NULL)
{
strcat(ss, s1);
}
if (s2 != NULL)
{
strcat(ss, s2);
}
}
char* ssalloc(
const char*const s1,
const char*const s2
)
{
const size_t l1 = (s1 != NULL) ? strlen(s1) : 0;
const size_t l2 = (s2 != NULL) ? strlen(s2) : 0;
char*const ss = malloc(l1 + l2 + 1);
if (ss == NULL)
{
return ss;
}
ss[0] = '\0';
if (s1 != NULL)
{
strncat(ss, s1, l1 + 1);
}
if (s2 != NULL)
{
strncat(ss, s2, l2 + 1);
}
return ss;
}
int main()
{
char ss[256] = {0};
string_string(NULL, "abc", "def");
printf("%s\n", ss);
string_string(ss, "abc", "def");
printf("%s\n", ss);
string_string(ss, "abc", NULL);
printf("%s\n", ss);
string_string(ss, NULL, "def");
printf("%s\n", ss);
char* ssa;
ssa = ssalloc("ABC", "DEF");
printf("%s\n", ssa);
ssa = ssalloc("ABC", NULL);
printf("%s\n", ssa);
ssa = ssalloc(NULL, "DEF");
printf("%s\n", ssa);
return 0;
}
ちょこっと解説
const
「const」使いまくった。
抜けあるかも?(笑)
でも、「const」って面倒。
配列を空の文字列で初期化
空の文字列にするのに、
「ss[0] = '\0';」
以外の方法ないかな。
しっくりこない。
strncat
「strncat」でなくて「strcat」でもいいような気がする。ただ、使い分けるのも面倒なので、できる限り「n」版を使うことにした。
三項演算子
三項演算子を使った。
使わないと const にできない。
スコープ
ああ、やっぱりC言語のスコープ { } で改行すると間延びするなぁ。
どれも改行なくてもいいし、さらにスコープもいらないんだけど。
引数チェック
引数チェックもばっちり!(多分😅)
この記事が気に入ったらサポートをしてみませんか?