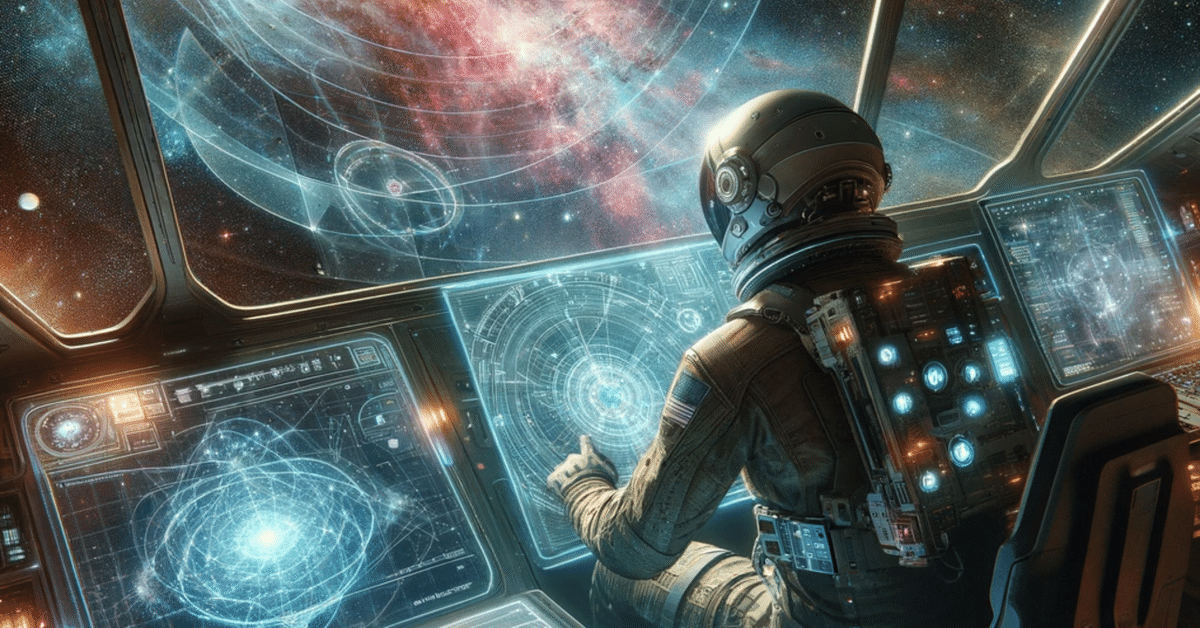
GPT-4-vision : コンテキストを基にしたリアルタイム画像解析を試す
概要
この記事では、PCのカメラを用いてリアルタイムで映像フレームを取得し、それらをBase64形式にエンコードしてOpenAIのGPT-4-visionモデルに送信するプロセスを具体的に紹介しています。この技術的なアプローチは、映像から得られるコンテキスト情報を活用して、現在の状況を分析し、それに基づいて次の瞬間の動きや出来事を予測する新しい方法を提供します。特に、このプロセスは、過去のフレームから得られたデータを使って、映像内の状況の変化をリアルタイムで追跡し、予測することに重点を置いています。
実装
コードの概要
映像フレームのキャプチャ: PCのカメラから映像フレームをリアルタイムでキャプチャします。
フレームのエンコード: キャプチャされたフレームをBase64形式にエンコードします。これにより、画像データをテキスト形式で扱えるようになり、APIを介して簡単に送信できます。
AIモデルへの送信: エンコードされた映像フレームをOpenAIのGPTモデルに送信します。このモデルは、映像の内容を解析し、現在の状況や次の瞬間の予測を行います。
コンテキストの使用: 過去のフレームから得られるコンテキスト情報を利用して、より正確な現在の状況の解析と次の瞬間の予測を行います。
import cv2
import base64
import os
import requests
import time
from openai import OpenAI
from collections import deque
from datetime import datetime
def encode_image_to_base64(frame):
_, buffer = cv2.imencode(".jpg", frame)
return base64.b64encode(buffer).decode('utf-8')
def send_frame_to_gpt(frame, previous_texts, client):
# 前5フレームのテキストとタイムスタンプを結合してコンテキストを作成
context = ' '.join(previous_texts)
# フレームをGPTに送信するためのメッセージペイロードを準備
# コンテキストから前回の予測が現在の状況と一致しているかを評価し、
# 次の予測をするように指示
prompt_message = f"Context: {context}. Assess if the previous prediction matches the current situation. Current: explain the current situation in 10 words or less. Next: Predict the next situation in 10 words or less."
PROMPT_MESSAGES = {
"role": "user",
"content": [
prompt_message,
{"type": "image_url", "image_url": {"url": f"data:image/jpeg;base64,{frame}"}}
],
}
# API呼び出しパラメータ
params = {
"model": "gpt-4-vision-preview",
"messages": [PROMPT_MESSAGES],
"max_tokens": 500,
}
# API呼び出し
result = client.chat.completions.create(**params)
return result.choices[0].message.content
def main():
# OpenAIクライアントの初期化
client = OpenAI(api_key=os.environ['OPENAI_API_KEY'])
# PCのインナーカメラを開く
video = cv2.VideoCapture(0)
# 最近の5フレームのテキストを保持するためのキュー
previous_texts = deque(maxlen=5)
while video.isOpened():
success, frame = video.read()
if not success:
break
# フレームをBase64でエンコード
base64_image = encode_image_to_base64(frame)
# 現在のタイムスタンプを取得
timestamp = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
# GPTにフレームを送信し、生成されたテキストを取得
generated_text = send_frame_to_gpt(base64_image, previous_texts, client)
print(f"Timestamp: {timestamp}, Generated Text: {generated_text}")
# タイムスタンプ付きのテキストをキューに追加
previous_texts.append(f"[{timestamp}] {generated_text}")
# 1秒待機
time.sleep(1)
# ビデオをリリースする
video.release()
if __name__ == "__main__":
main()
実行
仮想環境の作成
# 仮想環境の作成
python -m venv myenv
# 仮想環境の有効化
source myenv/bin/activate
必要なパッケージのインストール
# 必要なパッケージのインストール
pip install opencv-python requests openai
環境変数の設定
export OPENAI_API_KEY="your-api-key"
実行
python your_script.py
Timestamp: 2025-01-01 00:00:00, Generated Text:
Current: Astronaut analyzing a complex star chart on a digital screen.
Timestamp: 2025-01-01 00:00:01, Generated Text:
Current: View of the cockpit filled with flickering control panels and monitors.
Next: Unable to predict without additional context or data.
Timestamp: 2025-01-01 00:00:02, Generated Text:
Current: Astronaut adjusting a glowing holographic navigation system.
Next: Unable to predict future actions or events.
Timestamp: 2025-01-01 00:00:03, Generated Text:
Current: Astronaut communicating through headset, lit by console lights.
Next: Continues communication or turns attention to nearby controls.
Timestamp: 2025-01-01 00:00:04, Generated Text:
Current: Close-up of astronaut's hand switching toggles on a control panel.
Next: May initiate a new course or continue monitoring systems.
Timestamp: 2025-01-01 00:00:05, Generated Text:
Current: Astronaut intently watching a 3D model of a galaxy rotating.
Next: Possible adjustment of the spaceship's trajectory or continued observation.
Timestamp: 2025-01-01 00:00:06, Generated Text:
Current: Reflective helmet visor showing a reflection of distant stars.
Next: Astronaut might turn to engage with a different instrument panel.
Timestamp: 2025-01-01 00:00:07, Generated Text:
Current: View of the cockpit's window revealing a distant nebula.
Next: Man could look up or interact with the cockpit's technology.
Timestamp: 2025-01-01 00:00:08, Generated Text:
Current: Astronaut typing on a virtual keyboard, data screens active.
Next: Continuation of data analysis or interaction with another astronaut.
Timestamp: 2025-01-01 00:00:09, Generated Text:
Current: Glancing outside the cockpit window at a passing meteoroid.
Next: May report the sighting or focus back on internal controls.
この記事が気に入ったらサポートをしてみませんか?