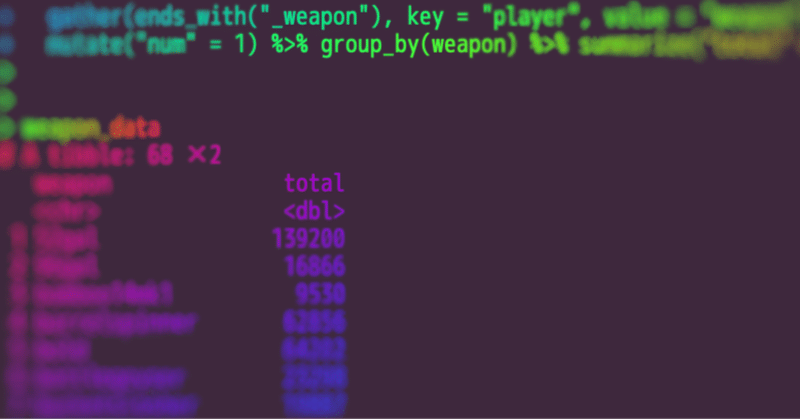
ワードカウント(単語の数を集計する)
tidyverse に用意された関数を使うことで、ワードカウント(登場する単語の種類を集計する)ことも簡単に書けます。下記のような単語の列があったとして、単語ごとに、その個数をカウントするようなケースです。(AAAが3個、BBBが3個、CCCが2個のように。)
グループごとに集計する: group_by(), summarize()
> meta_data %>% select(Id, Symbol)
# A tibble: 9 x 2
Id Symbol
<chr> <chr>
1 id1 AAA
2 id2 AAA
3 id3 AAA
4 id4 BBB
5 id5 BBB
6 id6 BBB
7 id7 CCC
8 id8 CCC
9 id9 DDD
集計するには、まず、各単語の数の列を mutate で追加しておきます。mutate("num" = 1) のように書くと、すべての行が 1 の列ができます。
> meta_data %>% select(Id, Symbol) %>% mutate("num" = 1)
# A tibble: 9 x 3
Id Symbol num
<chr> <chr> <dbl>
1 id1 AAA 1
2 id2 AAA 1
3 id3 AAA 1
4 id4 BBB 1
5 id5 BBB 1
6 id6 BBB 1
7 id7 CCC 1
8 id8 CCC 1
9 id9 DDD 1
次に、group_by() 関数を用いて、Symbol 列の文字でグループ化します。これだけでは何も起こりませんが、続く関数がグループ毎に適用されるようになります。下記のように、summarize(total = sum(num)) として、グループ毎に num の合計 = sum(num) を計算して、total の列に格納します。
result_data <- meta_data %>%
select(Id, Symbol) %>%
mutate("num" = 1) %>%
group_by(Symbol) %>%
summarize("total" = sum(num))
>
>
> result_data
# A tibble: 4 x 2
Symbol total
<chr> <dbl>
1 AAA 3
2 BBB 3
3 CCC 2
4 DDD 1
* group_by(Symbol) %>% summarize("total" = sum(Symbol)) としてもよさそうですが、Symbol は文字列なので、sum() で集計できません。そのため、 num = 1 で、集計用の列を作成したのです。
使用例(ブキの集計)
前回紹介したブキの種類を集計する例にも用いています。
weapon_data <- weapon_no_a1 %>%
gather(ends_with("_weapon"), key = "player", value = "weapon") %>%
mutate("num" = 1) %>% group_by(weapon) %>% summarize("total" = sum(num))
データを縦に並べる: gather()
元のデータは、全プレイヤーのブキが横に並んでいます。(select(-A1_weapon) で A1 を除外してます。)
> weapon_no_a1
# A tibble: 224,636 × 7
A2_weapon A3_weapon A4_weapon B1_we…¹ B2_we…² B3_we…³ B4_we…⁴
<chr> <chr> <chr> <chr> <chr> <chr> <chr>
1 prime_collabo hydra prime_co… momiji sshoot… bucket… clashb…
2 nautilus47 splatroller squiclea… 52gal splatr… jimuwi… prime_…
3 sshooter_collabo bucketslosher_deco maneuver hotbla… rpen_5h widero… momiji
4 maneuver hokusai sshooter… tristr… hissen sshoot… splats…
5 52gal momiji nautilus… furo 52gal furo rpen_5h
6 prime_collabo wideroller pablo_hue spaces… splatr… splats… pablo_…
7 prime_collabo sshooter rpen_5h drivew… prime_… sharp maneuv…
8 jimuwiper rapid nzap85 longbl… bold sshoot… bold
9 sputtery_hue carbon_deco rpen_5h furo sshoot… momiji sputte…
10 dualsweeper rpen_5h sputtery… furo sharp spaces… bold
# … with 224,626 more rows, and abbreviated variable names ¹B1_weapon,
# ²B2_weapon, ³B3_weapon, ⁴B4_weapon
# ℹ Use `print(n = ...)` to see more rows
このままだと、前述のように集計できないので、すべての列 (*_weapon) を1列にまとめます。そのために使用するのが gather() 関数です。
gather(ends_with("_weapon"), key = "player", value = "weapon")
で、weapon の列を縦に連結します。
gather の最初の引数は、ends_with("_weapon") で、「列名が "_weapon" で終わる全ての列」という意味です。
また、gather には key と value の引数が必要です。key は、列名(この場合 A2_weapon, A3_weapon など)の呼び名 (player) です。value は、連結後の値の呼び名(weapon)です。どちらも新たに作成される列の名前になるものなので、任意に指定できます(何でもOK)。
weapon_no_a1 %>%
gather(ends_with("_weapon"), key = "player", value = "weapon")
結果は、下記のようになります。列名だった A2_weapon や A3_weapon などが、player 列となります。また、value に指定した weapon の列ができます。結果は、157万行、2列のデータになります。(Chill season だけの結果に修正)
# A tibble: 1,572,452 × 2
player weapon
<chr> <chr>
1 A2_weapon prime_collabo
2 A2_weapon nautilus47
3 A2_weapon sshooter_collabo
4 A2_weapon maneuver
5 A2_weapon 52gal
6 A2_weapon prime_collabo
7 A2_weapon prime_collabo
8 A2_weapon jimuwiper
9 A2_weapon sputtery_hue
10 A2_weapon dualsweeper
# … with 1,572,442 more rows
# ℹ Use `print(n = ...)` to see more rows
157万行あっても、ここまで来れば、あとは最初の例の通りです。mutate() で集計用の値を設定して、group_by() して、 summarize() で集計するだけです。
weapon_data <- weapon_no_a1 %>%
gather(ends_with("_weapon"), key = "player", value = "weapon") %>%
mutate("num" = 1) %>% group_by(weapon) %>% summarize("total" = sum(num))
68種類の weapon ごとにグループ化して集計するので、結果は、68行のデータになります。
> weapon_data
# A tibble: 68 × 2
weapon total
<chr> <dbl>
1 52gal 55852
2 96gal 6407
3 bamboo14mk1 4052
4 barrelspinner 29348
5 bold 27072
6 bottlegeyser 12348
7 bucketslosher 8211
8 bucketslosher_deco 3838
9 campingshelter 4912
10 carbon 3899
# … with 58 more rows
# ℹ Use `print(n = ...)` to see more rows
この記事が気に入ったらサポートをしてみませんか?