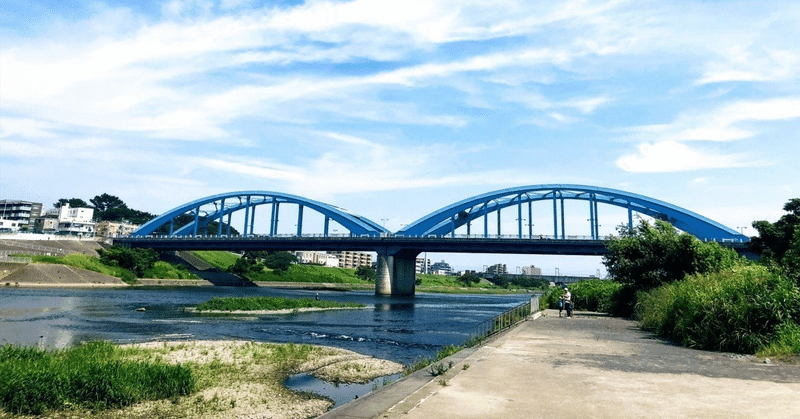
Photo by
masaya_chonan
Coinalyze API②
前回の記事
取得可能なデータの続き
取得可能なデータ
Get open interest history
過去の未決済建玉(Open Interest, OI)のデータ履歴
[
{
"symbol": "BTCUSD_PERP.A", // 取引シンボル
"history": [
{
"t": 1622548800, // データのタイムスタンプ(UNIX時間)
"o": 1000, // 始値
"h": 1100, // 高値
"l": 900, // 安値
"c": 1050 // 終値
}
]
}
]
Get funding rate history
過去の資金調達率(Funding Rate)のデータ履歴
[
{
"symbol": "BTCUSD_PERP.A", // 取引シンボル
"history": [
{
"t": 1622548800, // データのタイムスタンプ(UNIX時間)
"o": 0.0001, // 始値
"h": 0.0002, // 高値
"l": 0.00005, // 安値
"c": 0.00015 // 終値
}
]
}
]
Get predicted funding rate history
過去の予測資金調達率(Predicted Funding Rate)のデータ履歴
[
{
"symbol": "BTCUSD_PERP.A", // 取引シンボル
"history": [
{
"t": 1622548800, // データのタイムスタンプ(UNIX時間)
"o": 0.0001, // 始値
"h": 0.0002, // 高値
"l": 0.00005, // 安値
"c": 0.00015 // 終値
}
]
}
]
Get liquidation history
過去の清算(Liquidation)データ履歴
[
{
"symbol": "BTCUSD_PERP.A", // 取引シンボル
"history": [
{
"t": 1622548800, // データのタイムスタンプ(UNIX時間)
"l": 1000, // ロングポジションの清算量
"s": 800 // ショートポジションの清算量
}
]
}
]
Get long/short ratio history
過去のロング/ショート比率(Long/Short Ratio)のデータ履歴
[
{
"symbol": "BTCUSD_PERP.A", // 取引シンボル
"history": [
{
"t": 1622548800, // データのタイムスタンプ(UNIX時間)
"r": 0.75, // ロング/ショート比率
"l": 1500, // ロングポジションの数量
"s": 500 // ショートポジションの数量
}
]
}
]
Get OHLCV history
過去のOHLCV(Open, High, Low, Close, Volume)データ履歴
[
{
"symbol": "BTCUSD_PERP.A", // 取引シンボル
"history": [
{
"t": 1622548800, // データのタイムスタンプ(UNIX時間)
"o": 35000, // 始値
"h": 35500, // 高値
"l": 34000, // 安値
"c": 34500, // 終値
"v": 1200, // 取引量
"bv": 600, // ベースアセットの取引量
"tx": 100, // 取引数
"btx": 50 // ベースアセットの取引数
}
]
}
]
このデータを使った使用例
BinanceのBTCUSDTのperp契約(PERP.A)のOI履歴データを取得し、そのデータを用いてローソク足チャートを描画する
import requests
import time
import matplotlib.pyplot as plt
import pandas as pd
import mplfinance as mpf
from datetime import datetime
# Coinalyze APIエンドポイント
url = "https://api.coinalyze.net/v1/open-interest-history"
# リクエストパラメータ
params = {
"symbols": "BTCUSDT_PERP.A,ETHUSDT_PERP.A", # 取得するシンボル(最大20個)
"interval": "1hour", # 時間間隔(例: 1min, 5min, 15min, 30min, 1hour, 2hour, 4hour, 6hour, 12hour, daily)
"from": int(time.time()) - 864000, # 開始日時(UNIX時間、秒単位)
"to": int(time.time()), # 終了日時(UNIX時間、秒単位)
"convert_to_usd": "true" # USDに変換するかどうか(true または false)
}
# APIキー
api_key = "APIキーを入力"
# APIリクエストヘッダー
headers = {
"Accept": "application/json",
"api-key": api_key
}
# APIリクエストを送信
try:
response = requests.get(url, headers=headers, params=params)
response.raise_for_status()
data = response.json()
except requests.exceptions.RequestException as e:
print(f"APIリクエストに失敗しました: {e}")
data = []
except ValueError as e:
print(f"JSONの解析に失敗しました: {e}")
data = []
# データのバリデーション
if not isinstance(data, list) or not data:
print("データが正しく取得できませんでした")
else:
# 取得したデータをローソク足チャートで表示
for item in data:
symbol = item.get("symbol")
history = item.get("history")
if not symbol or not isinstance(history, list):
print(f"データが正しく取得できませんでした: {item}")
continue
# データをリストに変換
try:
timestamps = [datetime.fromtimestamp(entry["t"]) for entry in history]
opens = [entry["o"] for entry in history]
highs = [entry["h"] for entry in history]
lows = [entry["l"] for entry in history]
closes = [entry["c"] for entry in history]
except (KeyError, TypeError) as e:
print(f"データの処理中にエラーが発生しました: {e}")
continue
# ローソク足のデータを DataFrame に変換
ohlc_data = pd.DataFrame({"Open": opens, "High": highs, "Low": lows, "Close": closes}, index=pd.to_datetime(timestamps))
# ローソク足チャートを作成
fig, ax = mpf.plot(ohlc_data, type='candle', style='charles', volume=False, xrotation=45, datetime_format='%Y-%m-%d %H:%M', returnfig=True, figsize=(12, 6))
ax[0].set_title(f"Open Interest History for {symbol}")
ax[0].set_xlabel("Date")
ax[0].set_ylabel("Open Interest")
plt.show()
api_keyにapiキーの入力が必要
https://coinalyze.net/account/api-key/
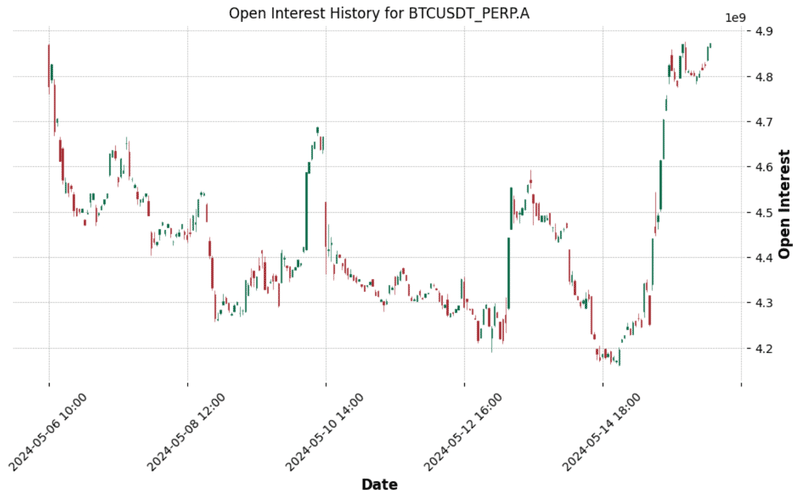
以上になります。
この記事が気に入ったらサポートをしてみませんか?