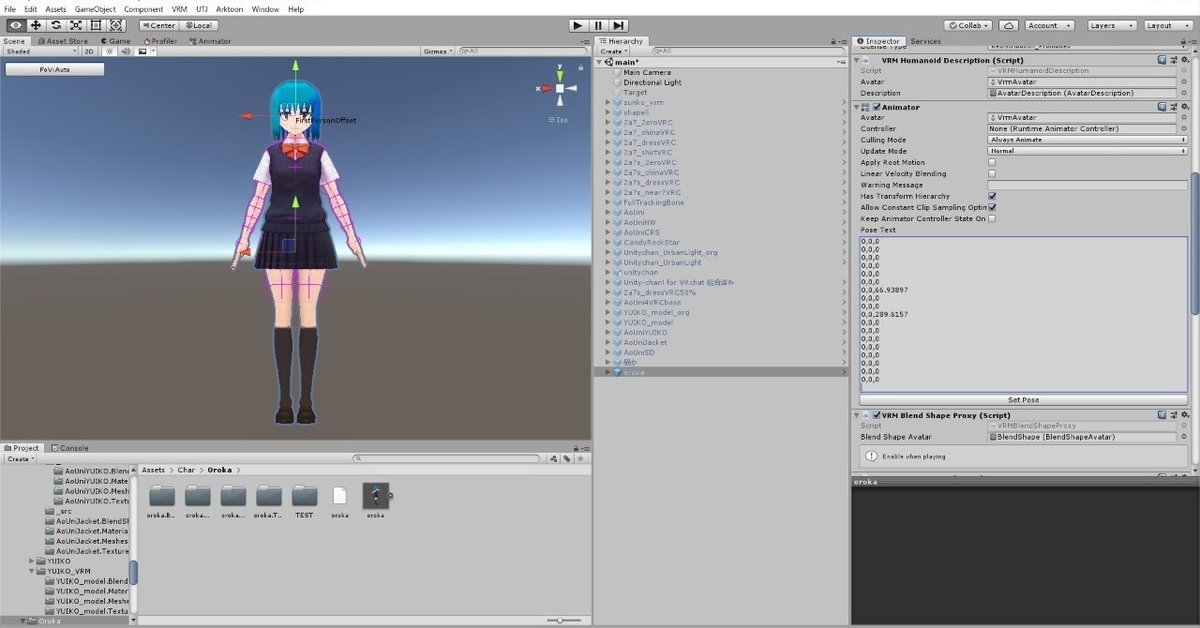
VRMお人形遊びのポーズテキストファイルをUnityで使う方法
概要
コレ
https://120byte.booth.pm/items/1654585
はVRMでポーズを付けられるんですが、付けたポーズをテキストファイルで保存できるので、そのポーズをUnityのインスペクタ拡張を使ってモデルに適用します。
インスペクタ拡張
using System.Collections.Generic;
using UnityEngine;
using UnityEditor;
[CustomEditor(typeof(Animator), true)]
public class SetPose : Editor
{
Animator anim;
List<HumanBodyBones> BoneList;
public string text;
void OnEnable()
{
anim = target as Animator;
BoneList = new List<HumanBodyBones>();
BoneList.Add(HumanBodyBones.Head);
BoneList.Add(HumanBodyBones.Neck);
BoneList.Add(HumanBodyBones.Hips);
BoneList.Add(HumanBodyBones.Spine);
BoneList.Add(HumanBodyBones.Chest);
BoneList.Add(HumanBodyBones.UpperChest);
BoneList.Add(HumanBodyBones.LeftUpperArm);
BoneList.Add(HumanBodyBones.LeftLowerArm);
BoneList.Add(HumanBodyBones.LeftHand);
BoneList.Add(HumanBodyBones.RightUpperArm);
BoneList.Add(HumanBodyBones.RightLowerArm);
BoneList.Add(HumanBodyBones.RightHand);
BoneList.Add(HumanBodyBones.LeftUpperLeg);
BoneList.Add(HumanBodyBones.LeftLowerLeg);
BoneList.Add(HumanBodyBones.LeftFoot);
BoneList.Add(HumanBodyBones.RightUpperLeg);
BoneList.Add(HumanBodyBones.RightLowerLeg);
BoneList.Add(HumanBodyBones.RightFoot);
}
public override void OnInspectorGUI()
{
base.OnInspectorGUI();
EditorGUILayout.LabelField("Pose Text");
text = EditorGUILayout.TextArea(text, GUILayout.Height(250));
if (GUILayout.Button("Set Pose"))
{
Debug.Log(text);
string[] pose = text.Replace("\n", ",").Split(',');
int i = 0;
foreach (HumanBodyBones Bone in BoneList)
{
anim.GetBoneTransform(Bone).localEulerAngles = GetVector3(pose, i);
i++;
}
text = "";
Debug.Log("Complete");
}
}
Vector3 GetVector3(string[] pose, int index)
{
index *= 3;
float x = float.Parse(pose[index + 0]);
float y = float.Parse(pose[index + 1]);
float z = float.Parse(pose[index + 2]);
var vec3 = new Vector3(x, y, z);
return vec3;
}
}
言い訳させてもらうと、拡張系初めて書いたので、色々オカシイ気がしますが、一応動いたので気になる所は直してお使い頂ければと思います。
使い方
Unityに先述のスクリプトを取り込んで、Animatorに拡張されたPose TextへVRMお人形遊びでポーズを保存したテキストファイルの内容を貼り付け、Set Poseボタンを押します。
この記事が気に入ったらサポートをしてみませんか?