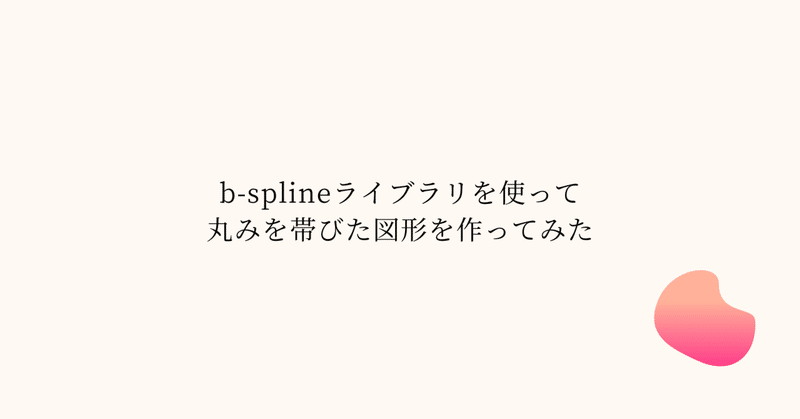
b-splineライブラリを使って丸みを帯びた図形を作ってみた
こんにちわ。nap5です。
b-splineライブラリを使って丸みを帯びた図形を作ってみたので紹介したいと思います。
b-splineを使った何かアウトプットをしてみたかったので、トライしてみました。
よく調べると、pythonのscipyライブラリ補間系ユーティリティにsplrepメソッドがあり、これを使うと実現したい丸みを帯びた図形を作りやすいことが分かりました。
https://stackoverflow.com/a/34807513/15972569
使用したライブラリは以下になります。
https://github.com/thibauts/b-spline
また、numpyライクに操作できるライブラリとしてnumjsを使用しました。
今回のプログラムです。
次数に3,4,5,10を指定してオリジナルなsvgコマンドパスとsvg-path-editorを用いて比較してみました。
import bspline from "b-spline";
import { samples } from "culori";
import { interpolate } from "popmotion";
import nj from "numjs";
const divmod = (n, m) => {
const quotient = Math.floor(n / m);
const remainder = n % m;
return { factor: quotient, fraction: remainder };
};
const niceBspline = ({ points = [], n = 100, degree = 3, periodic = true }) => {
let knots = [];
let count = points.length;
let weights = points.map((p) => {
return 1;
});
// If periodic, extend the point array by count+degree+1
if (periodic) {
const { factor, fraction } = divmod(count + degree + 1, count);
points = [
...[...Array(factor).keys()]
.map((_, index) => {
return points;
})
.flat(),
...points.slice(0, fraction),
];
count = points.length;
weights = points.map((p) => {
return 1;
});
// https://numpy.org/doc/stable/reference/generated/numpy.clip.html
// https://github.com/nicolaspanel/numjs
degree = nj.clip(degree, 1, degree).tolist()[0];
} else {
// If opened, prevent degree from exceeding count-1
degree = nj.clip(degree, 1, count - 1).tolist()[0];
}
// Calculate knot vector
if (periodic) {
knots.push(...nj.arange(0 - degree, count + degree + degree - 1).tolist());
knots = knots.slice(0, count + degree + 1);
} else {
knots.push(
...[...Array(degree).keys()].map((_, index) => {
return 0;
})
);
knots.push(...nj.arange(count - degree + 1).tolist());
knots.push(
...[...Array(degree).keys()].map((_, index) => {
return count - degree;
})
);
}
// do spline
const splinePoints = samples(100).map((t) => {
return bspline(t, degree, points, knots, weights);
});
return { count, degree, points, knots, splinePoints };
};
const makePath = ({ points, noZ }) => {
let path = `M ${points[0][0]},${points[0][1]}`;
for (let i = 1; i < points.length; i++) {
path = path + ` L ${points[i][0]},${points[i][1]}`;
}
path = path + (noZ ? `` : ` Z`);
return path;
};
const formatPoint = ([x, y]) => {
return { x, y };
};
const unFormatPoint = ({ x, y }) => {
return [x, y];
};
const buildPoints = ({ edgeCount }) => {
const stepSize = (Math.PI * 2) / edgeCount;
return [...Array(edgeCount)].map((_, edgeIndex) => {
return [Math.cos(edgeIndex * stepSize), Math.sin(edgeIndex * stepSize)];
});
};
const points = [
[50, 25],
[59, 12],
[50, 10],
[57, 2],
[40, 4],
[41, 12],
];
const { splinePoints: a } = niceBspline({
points,
periodic: true,
degree: 3,
});
const { splinePoints: b } = niceBspline({
points,
periodic: true,
degree: 4,
});
const { splinePoints: c } = niceBspline({
points,
periodic: true,
degree: 5,
});
const { splinePoints: d } = niceBspline({
points,
periodic: true,
degree: 10,
});
const originalPath = makePath({ points, noZ: false });
const splinePathA = makePath({ points: a, noZ: true });
const splinePathB = makePath({ points: b, noZ: true });
const splinePathC = makePath({ points: c, noZ: true });
const splinePathD = makePath({ points: d, noZ: true });
console.log(originalPath);
console.log(splinePathA);
console.log(splinePathB);
console.log(splinePathC);
console.log(splinePathD);
こちらが実行結果になります。
$ time node index.js
M 50,25 L 59,12 L 50,10 L 57,2 L 40,4 L 41,12 Z
M 56,13.833333333333334 L 55.95700775396726,13.329525915894656 L 55.836043666265084,12.874703398986636 L 55.64912671248759,12.462856294812218 L 55.408275868228934,12.087975115574341 L 55.125510109083216,11.744050373475943 L 54.81284841064455,11.425072580719963 L 54.48230974850708,11.125032249509344 L 54.1459130982649,10.837919892047022 L 53.81567743551215,10.557726020535938 L 53.50362173584294,10.27844114717903 L 53.221764974851396,9.994055784179242 L 52.98212612813163,9.698560443739506 L 52.79672417127779,9.385945638062768 L 52.677578079883965,9.050201879351967 L 52.63396128409902,8.686544044670764 L 52.65820363963412,8.297742929413166 L 52.736177886060545,7.889448853738213 L 52.85374405209116,7.467317806160781 L 52.99676216643873,7.037005775195754 L 53.15109225781606,6.604168749358015 L 53.30259435493595,6.1744627171624415 L 53.4371284865112,5.753543667123914 L 53.540554681254605,5.347067587757313 L 53.598732967878966,4.960690467577519 L 53.5975233750971,4.600068295099415 L 53.52278593162177,4.270857058837875 L 53.36038066616579,3.978712747307789 L 53.09616760744196,3.7292913490240296 L 52.717767409839645,3.52781943160476 L 52.23009711439464,3.3753049317787607 L 51.647891010915195,3.270361335354704 L 50.98599606925288,3.211574645203866 L 50.25925925925926,3.197530864197531 L 49.48252755078589,3.2268159952069757 L 48.67064791368434,3.298016041103482 L 47.83846731780616,3.4097170047583267 L 47.00083273300291,3.560504889042794 L 46.172591129126175,3.7489656968281597 L 45.368589476027495,3.973685430985706 L 44.60367474355843,4.2332500943867135 L 43.892693901570546,4.526245689902458 L 43.25049391991541,4.851258220404226 L 42.69135012334686,5.206906668288161 L 42.21993598536809,5.5923639689071765 L 41.83295767593289,6.007262709742047 L 41.52688020221945,6.45124939151059 L 41.298168571405995,6.9239705149306205 L 41.143287790670705,7.425072580719964 L 41.0587028671918,7.954202089596439 L 41.040878808147454,8.511005542277864 L 41.08628062071588,9.095129439482054 L 41.191373312075285,9.706220281926846 L 41.35262188940385,10.343924570330039 L 41.5664913598798,11.007888805409463 L 41.82944673068131,11.69775948788295 L 42.13795300898658,12.41318311846829 L 42.48848447746519,13.153667065512794 L 42.87782185353863,13.914122176085243 L 43.30311515659949,14.683919767686726 L 43.76153639239038,15.452101362569682 L 44.25025756665385,16.207708482986515 L 44.766450685132455,16.939782651189653 L 45.307287753568744,17.6373653894315 L 45.869940777705295,18.289498219964504 L 46.45158176328465,18.885222665041052 L 47.04938271604938,19.413580246913575 L 47.660515641742045,19.86361248783451 L 48.282152546105195,20.224360910056248 L 48.91146543488142,20.484867035831225 L 49.54562631381324,20.634172387411855 L 50.18179344717453,20.661411929037683 L 50.81592787377912,20.563861758076634 L 51.4418813169961,20.353141316920524 L 52.05329078974625,20.042330079010007 L 52.64379330495032,19.644507517785758 L 53.2070258755291,19.172753106688422 L 53.73662551440329,18.640146319158664 L 54.2262292344937,18.059766628637156 L 54.66947404872107,17.444693508564537 L 55.059996970006154,16.808006432381497 L 55.39143501126973,16.162784873528672 L 55.65742518543253,15.522108305446745 L 55.85160450541534,14.899056201576354 L 55.96760998413892,14.306708035358174 L 55.99908756647865,13.758134004741494 L 55.94422063027307,13.261692358060072 L 55.813098848911515,12.813377113652596 L 55.61774119798812,12.407178783721996 L 55.370166653096966,12.037087880471207 L 55.08239418983221,11.697094916103183 L 54.76644278378795,11.381190402820849 L 54.43433141055833,11.083364852827152 L 54.09807904573744,10.797608778325031 L 53.769704664919445,10.517912691517427 L 53.46122724369842,10.238267104607273 L 53.184665757668505,9.952662529797514 L 52.95203918242384,9.655089479291094 L 52.775366493558515,9.339538465290937 L 52.66666666666667,9
M 54.41666666666667,11.208333333333334 L 54.180402781047015,10.871864640578716 L 53.95007943471124,10.542366336447722 L 53.7349411694933,10.21506618063092 L 53.54238290472044,9.886016764477295 L 53.3779499372131,9.552095510994262 L 53.245337941285015,9.21100467484765 L 53.1463929687431,8.861271342361707 L 53.081111448887526,8.502247431519102 L 53.047640188511714,8.134109691960933 L 53.04227637190234,7.757859704986698 L 53.05946756083931,7.375323883554336 L 53.09181169459573,6.989153472280194 L 53.130057089938006,6.602824547439042 L 53.163102441125766,6.220638016964073 L 53.17808451546661,5.847689826444313 L 53.161872293396954,5.489363334610005 L 53.10310744645573,5.150636074021031 L 52.99248116021218,4.8359857022516675 L 52.822734201932256,4.549389978901397 L 52.58865692057857,4.2943267655949 L 52.287089246810424,4.073774025982056 L 51.916920692983794,3.890209825737947 L 51.479090353151335,3.7456123325628505 L 50.9765869030624,3.6414598161822473 L 50.414448600163006,3.578730648346818 L 49.799763283595844,3.557903302832443 L 49.1416683742003,3.578956355440202 L 48.45135087451245,3.641368483996375 L 47.74201104149218,3.7441247036306136 L 47.02783877116042,3.8858920619501633 L 46.32210185407009,4.065347774294837 L 45.636769276017844,4.281243881077542 L 44.98251028806584,4.5324074074074066 L 44.36869440654176,4.817740363089786 L 43.803391413038796,5.136219742626247 L 43.29337135441568,5.48689752521458 L 42.84410454279664,5.8689006747487955 L 42.45976155557146,6.281431139819121 L 42.14321323539539,6.723765853712007 L 41.896030690189235,7.195256734410129 L 41.718485293139345,7.69533068459237 L 41.60954868269753,8.223489591633845 L 41.5668987588584,8.779306663214228 L 41.58723263730123,9.362235177564393 L 41.66707078296109,9.971117068506247 L 41.80298175331097,10.604045582335909 L 41.991584095621356,11.258364118387291 L 42.22954634696015,11.93066622903212 L 42.5135870341928,12.616795619679912 L 42.840474673982186,13.311846148777986 L 43.20702777278869,14.010161827811464 L 43.61011482687018,14.705336821303272 L 44.046654322281995,15.390215446814107 L 44.51361473487694,16.05689217494251 L 45.00801453030534,16.696711629324803 L 45.526922164014955,17.30026858663508 L 46.067455659637815,17.857411419760112 L 46.626732666038606,18.357649965237037 L 47.201691760965495,18.791614876771487 L 47.78902290568539,19.15162557905066 L 48.38516611247775,19.43170114987604 L 48.98631144463461,19.627560320163454 L 49.58839901646063,19.73662147394304 L 50.18711899327309,19.758002648359252 L 50.77791159140182,19.692521533670885 L 51.355967078189295,19.542695473251033 L 51.91622577199059,19.312741463587106 L 52.45337804217334,19.008576154280856 L 52.961864309117836,18.63781584804834 L 53.43587504421691,18.209776500719933 L 53.86935120016443,17.7354728745438 L 54.25615850465588,17.227275573162125 L 54.59097199890167,16.697170498084827 L 54.869749650705565,16.15582690037407 L 55.089749162605834,15.612564306560051 L 55.24952797187515,15.075352518641008 L 55.34894325052075,14.55081161408322 L 55.38915190528431,14.044211945821015 L 55.372610577641964,13.559474142256738 L 55.30307564380438,13.099169107260817 L 55.18560321471666,12.664518020171663 L 55.02654913605843,12.255392335795776 L 54.83356898824373,11.870313784407664 L 54.61561808642117,11.50645437174989 L 54.382951425820174,11.15963641677004 L 54.14690009400171,10.824486933638209 L 53.918223192494835,10.495575590616914 L 53.70590103134684,10.168247980490783 L 53.51706429809829,9.838674527702857 L 53.35699405778294,9.503850488354622 L 53.22912175292781,9.161595950205957 L 53.135029203553174,8.810555832675174 L 53.07444860717254,8.450199886839004 L 53.04526253879262,8.080822695432602 L 53.04350395091342,7.703543672849525 L 53.06335617352814,7.320307065141775 L 53.09715291412326,6.933881950019762 L 53.13537825767844,6.5478622368523025 L 53.166666666666664,6.166666666666666
M 53.38333333333334,8.775000000000002 L 53.30386370660281,8.417272775204179 L 53.240655023666186,8.057326790706064 L 53.19021697955097,7.696105459662391 L 53.14776104939245,7.335189691384629 L 53.10747618974115,6.976704223587133 L 53.062804539870086,6.6232239546353 L 53.00671712308206,6.27768027579374 L 52.93198954801704,5.943267403474403 L 52.83147770995937,5.623348711484768 L 52.69839349214519,5.321363063275971 L 52.526580467069714,5.040731144190982 L 52.31078959779451,4.7847617937127485 L 52.04695493925483,4.556558337712353 L 51.732469339566954,4.358924920697183 L 51.36645816528958,4.194273355919368 L 50.949970529563714,4.064551595419554 L 50.48589381232774,3.9712447166302716 L 49.97872516573425,3.9154133887195157 L 49.43433469405044,3.8977345207938194 L 48.85972863330445,3.9185419101679217 L 48.26281253093161,3.977866890634436 L 47.652154425420704,4.075478980733514 L 47.03674802596025,4.2109265320225235 L 46.42577589208486,4.383577377345702 L 45.82837261332142,4.592659479103838 L 45.253387988835435,4.837301577523934 L 44.70915020707726,5.116573838928869 L 44.20322902542846,5.429528504007081 L 43.74219946466235,5.775240382733262 L 43.33144340725031,6.152836058781879 L 42.975120875515486,6.561472551007394 L 42.67625576529178,7.00028188179522 L 42.436831275720166,7.468312757201645 L 42.25788534431648,7.964472245523437 L 42.13960608203941,8.487467455867439 L 42.081427208358434,9.035747216720168 L 42.08212348632166,9.607443754517458 L 42.139906157623834,10.200314372214024 L 42.252518377674136,10.81168312785309 L 42.417330650664255,11.438382513136009 L 42.63143626463614,12.07669513199183 L 42.89174672655005,12.722295379146951 L 43.1950871381299,13.370191236242366 L 43.53828261274534,14.014683850135757 L 43.91819040759346,14.649434858109128 L 44.33168442908333,15.267628245052016 L 44.77563403909701,15.862143512542058 L 45.24688284719665,16.425728875820074 L 45.742227502831625,16.951174460765166 L 46.258396487545625,17.431485500869837 L 46.79202890718384,17.860055534215117 L 47.33965328410006,18.230839600445613 L 47.89766634936377,18.538527437744676 L 48.462311834967394,18.778716679809502 L 49.0296592660333,18.94808605282618 L 49.595582753020985,19.04456857244488 L 50.15573979969147,19.067524693908997 L 50.70555650099832,19.017896465105103 L 51.24026614072077,18.898202923623113 L 51.75500865376088,18.712354544814886 L 52.2449401333391,18.465437826641693 L 52.705342404590745,18.16349967711336 L 53.13173259816249,17.813331801727404 L 53.519972723808905,17.422255090908212 L 53.8663792439889,16.997904007446195 L 54.16783264746227,16.548010973936904 L 54.42188702288615,16.080190760220205 L 54.62687963241153,15.60172487081946 L 54.78204048527974,15.11934593238061 L 54.88760191141897,14.639022081111403 L 54.94490812976906,14.16574135608104 L 54.95651512046909,13.703306871736284 L 54.92617604153968,13.254259230846237 L 54.85865696571785,12.819987570894662 L 54.75951471344622,12.40088274825808 L 54.63487417104783,11.996491094704043 L 54.491205608901076,11.605668173889477 L 54.33510199961452,11.22673253785902 L 54.173056336201725,10.85761948354324 L 54.01123895025624,10.49603480925705 L 53.85527483012628,10.139608571197943 L 53.710020939089745,9.786048839944335 L 53.57934353352902,9.433295456953868 L 53.46589548110581,9.079673791061714 L 53.37089357918318,8.724048494856214 L 53.293903969094515,8.365973241839662 L 53.23276661997491,8.005771421962383 L 53.18378888719539,7.644483254020676 L 53.142019292135814,7.283773071858157 L 53.10152322349236,6.925835656613952 L 53.05565863858466,6.573302568970793 L 52.99735176466329,6.229148481403229 L 52.919372800216934,5.896597510425748 L 52.81461161627975,5.579029548840955 L 52.676353457738735,5.279886597987703 L 52.49855464464097,5.002579099989277 L 52.27611827350097,4.750392270001536 L 52.005169918608004,4.526392428461058 L 51.68333333333334,4.333333333333334
M 44.13836585097002,9.460604332010583 L 44.09389757691055,9.907810314052309 L 44.08738562805502,10.365200168680108 L 44.11892243393233,10.829858567623496 L 44.188229163496594,11.298712970769795 L 44.29466799819139,11.768555179214435 L 44.43725824193314,12.236067111901836 L 44.61469558251418,12.697850769229344 L 44.8253738622774,13.150462183761046 L 45.06740878199042,13.590448987685313 L 45.3386630446454,14.014391055644532 L 45.636772539428875,14.41894351685919 L 45.959173264338446,14.800881278856778 L 46.303128782870246,15.157144073390368 L 46.66575809985602,15.484880930087007 L 47.04406391789131,15.781492911794851 L 47.43496129286241,16.044672914291986 L 47.835306738952696,16.272441348635724 L 48.24192783547073,16.463176592074568 L 48.65165335917062,16.615639212986956 L 49.06134391033226,16.728989140553693 L 49.46792292452108,16.802795152152253 L 49.86840787067206,16.837036281805958 L 50.25994133597278,16.832095002448497 L 50.639821594981356,16.78874229428863 L 51.00553216053435,16.708114972201127 L 51.35476972330906,16.59168589784263 L 51.685469811427446,16.441227938114576 L 51.995829447257805,16.258772741684417 L 52.28432605160978,16.046565580550183 L 52.54973185185872,15.807017635108805 L 52.79112309612118,15.542657179980052 L 53.00788346524589,15.256081146417841 L 53.19970120838609,14.949908493361518 L 53.366559700518266,14.626736718138138 L 53.50872132283594,14.289102688044576 L 53.626704790410514,13.939448784557417 L 53.72125628678825,13.58009513180144 L 53.79331500320319,13.213218439212916 L 53.843973911746296,12.840837734125074 L 53.87443681805715,12.464807002339327 L 53.88597293081601,12.086814502689228 L 53.87987034342575,11.70838828421476 L 53.857389938703214,11.330907220904717 L 53.81972129106551,10.95561669809341 L 53.7679421435844,10.583647945525664 L 53.70298297156283,10.216039922934613 L 53.625598007687884,9.85376262858983 L 53.53634390272865,9.497740716945318 L 53.435566941349904,9.148876371888027 L 53.32339943693846,8.808070480141453 L 53.199765604463536,8.47624127806936 L 53.06439686838997,8.15433979740561 L 52.91685621560959,7.84336160426044 L 52.75657086332618,7.544354504075398 L 52.582872190898016,7.258422065972302 L 52.39504159488437,6.9867229961187975 L 52.192360680034476,6.730466554268804 L 51.97416400777176,6.490904353483826 L 51.739892499895845,6.269319003176263 L 51.48914554979138,6.067010143503668 L 51.22172993198542,5.88527847095189 L 50.93770371910924,5.725408371755863 L 50.63741360253275,5.588649765843439 L 50.32152425712695,5.476199724146127 L 49.991038679672265,5.389184361335879 L 49.64730875226992,5.328641429253798 L 49.2920356246271,5.295503948430394 L 48.92725986017688,5.29058512109182 L 48.555341638558744,5.314564673838452 L 48.178931638926365,5.367976686705384 L 47.80093353276521,5.451198882504215 L 47.42445927929352,5.564443281136984 L 47.05277862900899,5.707748072890705 L 46.68926439006098,5.88097053721099 L 46.33733509043049,6.083780831919318 L 46.0003966774447,6.315656501194258 L 45.681784842313405,6.575877594903823 L 45.38470945012156,6.863522352346211 L 45.11220240412547,7.177463475369981 L 44.8670700863651,7.516365094442017 L 44.651851303601,7.878680611752905 L 44.468781437497654,8.26265168313344 L 44.319763259886585,8.666308670644328 L 44.20634463693591,9.087472955432094 L 44.129703119209935,9.523761541059011 L 44.0906372070083,9.972594396252523 L 44.08956390110019,10.43120497812706 L 44.12652200679413,10.896654337882847 L 44.20118056062676,11.365849138061552 L 44.31285169443031,11.835563805361854 L 44.46050723676012,12.302466911346567 L 44.6427983707575,12.763151721799147 L 44.85807771448742,13.21417069080531 L 45.10442325862379,13.652073504635972 L 45.37966368105209,14.073448109986316 L 45.68140465251757,14.4749639978742 L 46.007055845865146,14.853416865314493 L 46.353858457688396,15.205773648555855 L 46.718913139329814,15.529216820987656
real 0m0.703s
user 0m0.682s
sys 0m0.153s
svg-path-editorに張り付けて眺めてみます。
M 50,25 L 59,12 L 50,10 L 57,2 L 40,4 L 41,12 Z
M 56,13.833333333333334 L 55.95700775396726,13.329525915894656 L 55.836043666265084,12.874703398986636 L 55.64912671248759,12.462856294812218 L 55.408275868228934,12.087975115574341 L 55.125510109083216,11.744050373475943 L 54.81284841064455,11.425072580719963 L 54.48230974850708,11.125032249509344 L 54.1459130982649,10.837919892047022 L 53.81567743551215,10.557726020535938 L 53.50362173584294,10.27844114717903 L 53.221764974851396,9.994055784179242 L 52.98212612813163,9.698560443739506 L 52.79672417127779,9.385945638062768 L 52.677578079883965,9.050201879351967 L 52.63396128409902,8.686544044670764 L 52.65820363963412,8.297742929413166 L 52.736177886060545,7.889448853738213 L 52.85374405209116,7.467317806160781 L 52.99676216643873,7.037005775195754 L 53.15109225781606,6.604168749358015 L 53.30259435493595,6.1744627171624415 L 53.4371284865112,5.753543667123914 L 53.540554681254605,5.347067587757313 L 53.598732967878966,4.960690467577519 L 53.5975233750971,4.600068295099415 L 53.52278593162177,4.270857058837875 L 53.36038066616579,3.978712747307789 L 53.09616760744196,3.7292913490240296 L 52.717767409839645,3.52781943160476 L 52.23009711439464,3.3753049317787607 L 51.647891010915195,3.270361335354704 L 50.98599606925288,3.211574645203866 L 50.25925925925926,3.197530864197531 L 49.48252755078589,3.2268159952069757 L 48.67064791368434,3.298016041103482 L 47.83846731780616,3.4097170047583267 L 47.00083273300291,3.560504889042794 L 46.172591129126175,3.7489656968281597 L 45.368589476027495,3.973685430985706 L 44.60367474355843,4.2332500943867135 L 43.892693901570546,4.526245689902458 L 43.25049391991541,4.851258220404226 L 42.69135012334686,5.206906668288161 L 42.21993598536809,5.5923639689071765 L 41.83295767593289,6.007262709742047 L 41.52688020221945,6.45124939151059 L 41.298168571405995,6.9239705149306205 L 41.143287790670705,7.425072580719964 L 41.0587028671918,7.954202089596439 L 41.040878808147454,8.511005542277864 L 41.08628062071588,9.095129439482054 L 41.191373312075285,9.706220281926846 L 41.35262188940385,10.343924570330039 L 41.5664913598798,11.007888805409463 L 41.82944673068131,11.69775948788295 L 42.13795300898658,12.41318311846829 L 42.48848447746519,13.153667065512794 L 42.87782185353863,13.914122176085243 L 43.30311515659949,14.683919767686726 L 43.76153639239038,15.452101362569682 L 44.25025756665385,16.207708482986515 L 44.766450685132455,16.939782651189653 L 45.307287753568744,17.6373653894315 L 45.869940777705295,18.289498219964504 L 46.45158176328465,18.885222665041052 L 47.04938271604938,19.413580246913575 L 47.660515641742045,19.86361248783451 L 48.282152546105195,20.224360910056248 L 48.91146543488142,20.484867035831225 L 49.54562631381324,20.634172387411855 L 50.18179344717453,20.661411929037683 L 50.81592787377912,20.563861758076634 L 51.4418813169961,20.353141316920524 L 52.05329078974625,20.042330079010007 L 52.64379330495032,19.644507517785758 L 53.2070258755291,19.172753106688422 L 53.73662551440329,18.640146319158664 L 54.2262292344937,18.059766628637156 L 54.66947404872107,17.444693508564537 L 55.059996970006154,16.808006432381497 L 55.39143501126973,16.162784873528672 L 55.65742518543253,15.522108305446745 L 55.85160450541534,14.899056201576354 L 55.96760998413892,14.306708035358174 L 55.99908756647865,13.758134004741494 L 55.94422063027307,13.261692358060072 L 55.813098848911515,12.813377113652596 L 55.61774119798812,12.407178783721996 L 55.370166653096966,12.037087880471207 L 55.08239418983221,11.697094916103183 L 54.76644278378795,11.381190402820849 L 54.43433141055833,11.083364852827152 L 54.09807904573744,10.797608778325031 L 53.769704664919445,10.517912691517427 L 53.46122724369842,10.238267104607273 L 53.184665757668505,9.952662529797514 L 52.95203918242384,9.655089479291094 L 52.775366493558515,9.339538465290937 L 52.66666666666667,9
M 54.41666666666667,11.208333333333334 L 54.180402781047015,10.871864640578716 L 53.95007943471124,10.542366336447722 L 53.7349411694933,10.21506618063092 L 53.54238290472044,9.886016764477295 L 53.3779499372131,9.552095510994262 L 53.245337941285015,9.21100467484765 L 53.1463929687431,8.861271342361707 L 53.081111448887526,8.502247431519102 L 53.047640188511714,8.134109691960933 L 53.04227637190234,7.757859704986698 L 53.05946756083931,7.375323883554336 L 53.09181169459573,6.989153472280194 L 53.130057089938006,6.602824547439042 L 53.163102441125766,6.220638016964073 L 53.17808451546661,5.847689826444313 L 53.161872293396954,5.489363334610005 L 53.10310744645573,5.150636074021031 L 52.99248116021218,4.8359857022516675 L 52.822734201932256,4.549389978901397 L 52.58865692057857,4.2943267655949 L 52.287089246810424,4.073774025982056 L 51.916920692983794,3.890209825737947 L 51.479090353151335,3.7456123325628505 L 50.9765869030624,3.6414598161822473 L 50.414448600163006,3.578730648346818 L 49.799763283595844,3.557903302832443 L 49.1416683742003,3.578956355440202 L 48.45135087451245,3.641368483996375 L 47.74201104149218,3.7441247036306136 L 47.02783877116042,3.8858920619501633 L 46.32210185407009,4.065347774294837 L 45.636769276017844,4.281243881077542 L 44.98251028806584,4.5324074074074066 L 44.36869440654176,4.817740363089786 L 43.803391413038796,5.136219742626247 L 43.29337135441568,5.48689752521458 L 42.84410454279664,5.8689006747487955 L 42.45976155557146,6.281431139819121 L 42.14321323539539,6.723765853712007 L 41.896030690189235,7.195256734410129 L 41.718485293139345,7.69533068459237 L 41.60954868269753,8.223489591633845 L 41.5668987588584,8.779306663214228 L 41.58723263730123,9.362235177564393 L 41.66707078296109,9.971117068506247 L 41.80298175331097,10.604045582335909 L 41.991584095621356,11.258364118387291 L 42.22954634696015,11.93066622903212 L 42.5135870341928,12.616795619679912 L 42.840474673982186,13.311846148777986 L 43.20702777278869,14.010161827811464 L 43.61011482687018,14.705336821303272 L 44.046654322281995,15.390215446814107 L 44.51361473487694,16.05689217494251 L 45.00801453030534,16.696711629324803 L 45.526922164014955,17.30026858663508 L 46.067455659637815,17.857411419760112 L 46.626732666038606,18.357649965237037 L 47.201691760965495,18.791614876771487 L 47.78902290568539,19.15162557905066 L 48.38516611247775,19.43170114987604 L 48.98631144463461,19.627560320163454 L 49.58839901646063,19.73662147394304 L 50.18711899327309,19.758002648359252 L 50.77791159140182,19.692521533670885 L 51.355967078189295,19.542695473251033 L 51.91622577199059,19.312741463587106 L 52.45337804217334,19.008576154280856 L 52.961864309117836,18.63781584804834 L 53.43587504421691,18.209776500719933 L 53.86935120016443,17.7354728745438 L 54.25615850465588,17.227275573162125 L 54.59097199890167,16.697170498084827 L 54.869749650705565,16.15582690037407 L 55.089749162605834,15.612564306560051 L 55.24952797187515,15.075352518641008 L 55.34894325052075,14.55081161408322 L 55.38915190528431,14.044211945821015 L 55.372610577641964,13.559474142256738 L 55.30307564380438,13.099169107260817 L 55.18560321471666,12.664518020171663 L 55.02654913605843,12.255392335795776 L 54.83356898824373,11.870313784407664 L 54.61561808642117,11.50645437174989 L 54.382951425820174,11.15963641677004 L 54.14690009400171,10.824486933638209 L 53.918223192494835,10.495575590616914 L 53.70590103134684,10.168247980490783 L 53.51706429809829,9.838674527702857 L 53.35699405778294,9.503850488354622 L 53.22912175292781,9.161595950205957 L 53.135029203553174,8.810555832675174 L 53.07444860717254,8.450199886839004 L 53.04526253879262,8.080822695432602 L 53.04350395091342,7.703543672849525 L 53.06335617352814,7.320307065141775 L 53.09715291412326,6.933881950019762 L 53.13537825767844,6.5478622368523025 L 53.166666666666664,6.166666666666666
M 53.38333333333334,8.775000000000002 L 53.30386370660281,8.417272775204179 L 53.240655023666186,8.057326790706064 L 53.19021697955097,7.696105459662391 L 53.14776104939245,7.335189691384629 L 53.10747618974115,6.976704223587133 L 53.062804539870086,6.6232239546353 L 53.00671712308206,6.27768027579374 L 52.93198954801704,5.943267403474403 L 52.83147770995937,5.623348711484768 L 52.69839349214519,5.321363063275971 L 52.526580467069714,5.040731144190982 L 52.31078959779451,4.7847617937127485 L 52.04695493925483,4.556558337712353 L 51.732469339566954,4.358924920697183 L 51.36645816528958,4.194273355919368 L 50.949970529563714,4.064551595419554 L 50.48589381232774,3.9712447166302716 L 49.97872516573425,3.9154133887195157 L 49.43433469405044,3.8977345207938194 L 48.85972863330445,3.9185419101679217 L 48.26281253093161,3.977866890634436 L 47.652154425420704,4.075478980733514 L 47.03674802596025,4.2109265320225235 L 46.42577589208486,4.383577377345702 L 45.82837261332142,4.592659479103838 L 45.253387988835435,4.837301577523934 L 44.70915020707726,5.116573838928869 L 44.20322902542846,5.429528504007081 L 43.74219946466235,5.775240382733262 L 43.33144340725031,6.152836058781879 L 42.975120875515486,6.561472551007394 L 42.67625576529178,7.00028188179522 L 42.436831275720166,7.468312757201645 L 42.25788534431648,7.964472245523437 L 42.13960608203941,8.487467455867439 L 42.081427208358434,9.035747216720168 L 42.08212348632166,9.607443754517458 L 42.139906157623834,10.200314372214024 L 42.252518377674136,10.81168312785309 L 42.417330650664255,11.438382513136009 L 42.63143626463614,12.07669513199183 L 42.89174672655005,12.722295379146951 L 43.1950871381299,13.370191236242366 L 43.53828261274534,14.014683850135757 L 43.91819040759346,14.649434858109128 L 44.33168442908333,15.267628245052016 L 44.77563403909701,15.862143512542058 L 45.24688284719665,16.425728875820074 L 45.742227502831625,16.951174460765166 L 46.258396487545625,17.431485500869837 L 46.79202890718384,17.860055534215117 L 47.33965328410006,18.230839600445613 L 47.89766634936377,18.538527437744676 L 48.462311834967394,18.778716679809502 L 49.0296592660333,18.94808605282618 L 49.595582753020985,19.04456857244488 L 50.15573979969147,19.067524693908997 L 50.70555650099832,19.017896465105103 L 51.24026614072077,18.898202923623113 L 51.75500865376088,18.712354544814886 L 52.2449401333391,18.465437826641693 L 52.705342404590745,18.16349967711336 L 53.13173259816249,17.813331801727404 L 53.519972723808905,17.422255090908212 L 53.8663792439889,16.997904007446195 L 54.16783264746227,16.548010973936904 L 54.42188702288615,16.080190760220205 L 54.62687963241153,15.60172487081946 L 54.78204048527974,15.11934593238061 L 54.88760191141897,14.639022081111403 L 54.94490812976906,14.16574135608104 L 54.95651512046909,13.703306871736284 L 54.92617604153968,13.254259230846237 L 54.85865696571785,12.819987570894662 L 54.75951471344622,12.40088274825808 L 54.63487417104783,11.996491094704043 L 54.491205608901076,11.605668173889477 L 54.33510199961452,11.22673253785902 L 54.173056336201725,10.85761948354324 L 54.01123895025624,10.49603480925705 L 53.85527483012628,10.139608571197943 L 53.710020939089745,9.786048839944335 L 53.57934353352902,9.433295456953868 L 53.46589548110581,9.079673791061714 L 53.37089357918318,8.724048494856214 L 53.293903969094515,8.365973241839662 L 53.23276661997491,8.005771421962383 L 53.18378888719539,7.644483254020676 L 53.142019292135814,7.283773071858157 L 53.10152322349236,6.925835656613952 L 53.05565863858466,6.573302568970793 L 52.99735176466329,6.229148481403229 L 52.919372800216934,5.896597510425748 L 52.81461161627975,5.579029548840955 L 52.676353457738735,5.279886597987703 L 52.49855464464097,5.002579099989277 L 52.27611827350097,4.750392270001536 L 52.005169918608004,4.526392428461058 L 51.68333333333334,4.333333333333334
M 44.13836585097002,9.460604332010583 L 44.09389757691055,9.907810314052309 L 44.08738562805502,10.365200168680108 L 44.11892243393233,10.829858567623496 L 44.188229163496594,11.298712970769795 L 44.29466799819139,11.768555179214435 L 44.43725824193314,12.236067111901836 L 44.61469558251418,12.697850769229344 L 44.8253738622774,13.150462183761046 L 45.06740878199042,13.590448987685313 L 45.3386630446454,14.014391055644532 L 45.636772539428875,14.41894351685919 L 45.959173264338446,14.800881278856778 L 46.303128782870246,15.157144073390368 L 46.66575809985602,15.484880930087007 L 47.04406391789131,15.781492911794851 L 47.43496129286241,16.044672914291986 L 47.835306738952696,16.272441348635724 L 48.24192783547073,16.463176592074568 L 48.65165335917062,16.615639212986956 L 49.06134391033226,16.728989140553693 L 49.46792292452108,16.802795152152253 L 49.86840787067206,16.837036281805958 L 50.25994133597278,16.832095002448497 L 50.639821594981356,16.78874229428863 L 51.00553216053435,16.708114972201127 L 51.35476972330906,16.59168589784263 L 51.685469811427446,16.441227938114576 L 51.995829447257805,16.258772741684417 L 52.28432605160978,16.046565580550183 L 52.54973185185872,15.807017635108805 L 52.79112309612118,15.542657179980052 L 53.00788346524589,15.256081146417841 L 53.19970120838609,14.949908493361518 L 53.366559700518266,14.626736718138138 L 53.50872132283594,14.289102688044576 L 53.626704790410514,13.939448784557417 L 53.72125628678825,13.58009513180144 L 53.79331500320319,13.213218439212916 L 53.843973911746296,12.840837734125074 L 53.87443681805715,12.464807002339327 L 53.88597293081601,12.086814502689228 L 53.87987034342575,11.70838828421476 L 53.857389938703214,11.330907220904717 L 53.81972129106551,10.95561669809341 L 53.7679421435844,10.583647945525664 L 53.70298297156283,10.216039922934613 L 53.625598007687884,9.85376262858983 L 53.53634390272865,9.497740716945318 L 53.435566941349904,9.148876371888027 L 53.32339943693846,8.808070480141453 L 53.199765604463536,8.47624127806936 L 53.06439686838997,8.15433979740561 L 52.91685621560959,7.84336160426044 L 52.75657086332618,7.544354504075398 L 52.582872190898016,7.258422065972302 L 52.39504159488437,6.9867229961187975 L 52.192360680034476,6.730466554268804 L 51.97416400777176,6.490904353483826 L 51.739892499895845,6.269319003176263 L 51.48914554979138,6.067010143503668 L 51.22172993198542,5.88527847095189 L 50.93770371910924,5.725408371755863 L 50.63741360253275,5.588649765843439 L 50.32152425712695,5.476199724146127 L 49.991038679672265,5.389184361335879 L 49.64730875226992,5.328641429253798 L 49.2920356246271,5.295503948430394 L 48.92725986017688,5.29058512109182 L 48.555341638558744,5.314564673838452 L 48.178931638926365,5.367976686705384 L 47.80093353276521,5.451198882504215 L 47.42445927929352,5.564443281136984 L 47.05277862900899,5.707748072890705 L 46.68926439006098,5.88097053721099 L 46.33733509043049,6.083780831919318 L 46.0003966774447,6.315656501194258 L 45.681784842313405,6.575877594903823 L 45.38470945012156,6.863522352346211 L 45.11220240412547,7.177463475369981 L 44.8670700863651,7.516365094442017 L 44.651851303601,7.878680611752905 L 44.468781437497654,8.26265168313344 L 44.319763259886585,8.666308670644328 L 44.20634463693591,9.087472955432094 L 44.129703119209935,9.523761541059011 L 44.0906372070083,9.972594396252523 L 44.08956390110019,10.43120497812706 L 44.12652200679413,10.896654337882847 L 44.20118056062676,11.365849138061552 L 44.31285169443031,11.835563805361854 L 44.46050723676012,12.302466911346567 L 44.6427983707575,12.763151721799147 L 44.85807771448742,13.21417069080531 L 45.10442325862379,13.652073504635972 L 45.37966368105209,14.073448109986316 L 45.68140465251757,14.4749639978742 L 46.007055845865146,14.853416865314493 L 46.353858457688396,15.205773648555855 L 46.718913139329814,15.529216820987656
どうやら、次数を上げていくと、ポリゴンのセンタリングへ近づいて収縮していくようなイメージですかね。また丸みをよく帯びていくようです。面白いです。
実はよーく見ると点がダブっているのですが、これはファクターで点を増幅させているからです。simplify-jsライブラリを使ってpolygonの簡素化で調節してみたんですが、形状に違和感が出るくらいなら、まぁダブってもいいかなということで、そのままにしています。
splineしているパスに関してはZコマンドは指定せずにしています。
これはきれいな見た目を維持するためです。
fillプロパティも指定でき、カラーリングもできるので、指定しなくても問題ないかなという感じです。
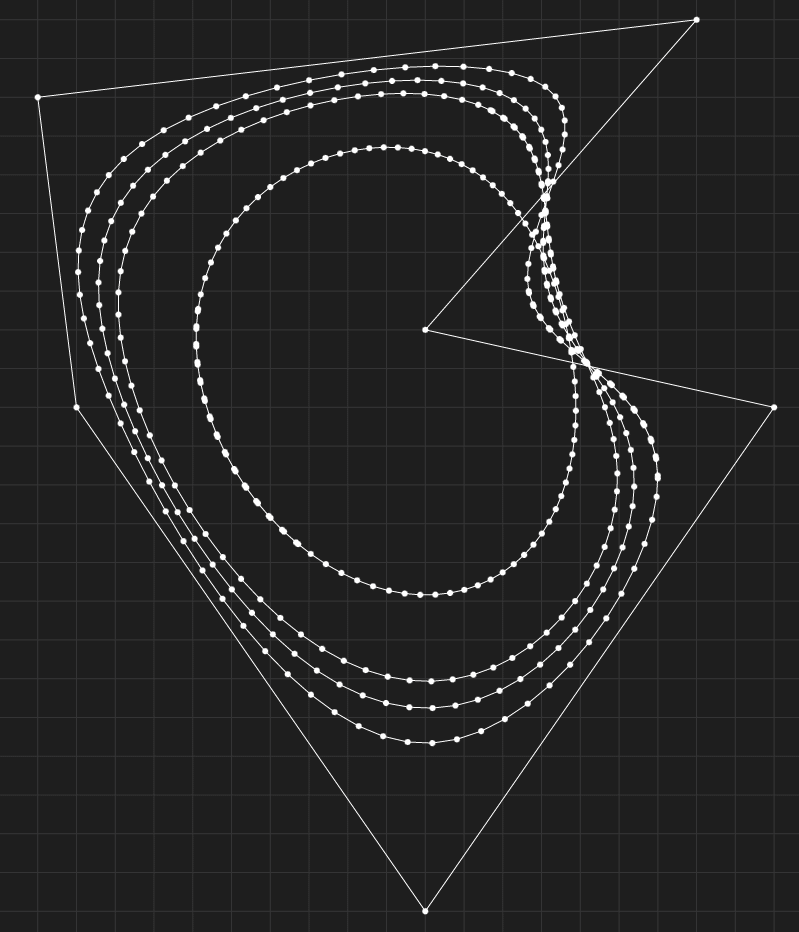
なかなか難しかったんですが、なんとかワークアラウンドを見つけられることができてよかったです。
最後におまけで、三角形でもやってみました。
import bspline from "b-spline";
import { samples } from "culori";
import { interpolate } from "popmotion";
import nj from "numjs";
const divmod = (n, m) => {
const quotient = Math.floor(n / m);
const remainder = n % m;
return { factor: quotient, fraction: remainder };
};
const niceBspline = ({ points = [], n = 100, degree = 3, periodic = true }) => {
let knots = [];
let count = points.length;
let weights = points.map((p) => {
return 1;
});
// If periodic, extend the point array by count+degree+1
if (periodic) {
const { factor, fraction } = divmod(count + degree + 1, count);
points = [
...[...Array(factor).keys()]
.map((_, index) => {
return points;
})
.flat(),
...points.slice(0, fraction),
];
count = points.length;
weights = points.map((p) => {
return 1;
});
// https://numpy.org/doc/stable/reference/generated/numpy.clip.html
// https://github.com/nicolaspanel/numjs
degree = nj.clip(degree, 1, degree).tolist()[0];
} else {
// If opened, prevent degree from exceeding count-1
degree = nj.clip(degree, 1, count - 1).tolist()[0];
}
// Calculate knot vector
if (periodic) {
knots.push(...nj.arange(0 - degree, count + degree + degree - 1).tolist());
knots = knots.slice(0, count + degree + 1);
} else {
knots.push(
...[...Array(degree).keys()].map((_, index) => {
return 0;
})
);
knots.push(...nj.arange(count - degree + 1).tolist());
knots.push(
...[...Array(degree).keys()].map((_, index) => {
return count - degree;
})
);
}
// do spline
const splinePoints = samples(100).map((t) => {
return bspline(t, degree, points, knots, weights);
});
return { count, degree, points, knots, splinePoints };
};
const makePath = ({ points, noZ }) => {
let path = `M ${points[0][0]},${points[0][1]}`;
for (let i = 1; i < points.length; i++) {
path = path + ` L ${points[i][0]},${points[i][1]}`;
}
path = path + (noZ ? `` : ` Z`);
return path;
};
const formatPoint = ([x, y]) => {
return { x, y };
};
const unFormatPoint = ({ x, y }) => {
return [x, y];
};
const buildPoints = ({ edgeCount }) => {
const stepSize = (Math.PI * 2) / edgeCount;
return [...Array(edgeCount)].map((_, edgeIndex) => {
return [Math.cos(edgeIndex * stepSize), Math.sin(edgeIndex * stepSize)];
});
};
const points = buildPoints({ edgeCount: 3 });
const { splinePoints: a } = niceBspline({
points,
periodic: true,
degree: 3,
});
const { splinePoints: b } = niceBspline({
points,
periodic: true,
degree: 4,
});
const { splinePoints: c } = niceBspline({
points,
periodic: true,
degree: 5,
});
const { splinePoints: d } = niceBspline({
points,
periodic: true,
degree: 10,
});
const originalPath = makePath({ points, noZ: false });
const splinePathA = makePath({ points: a, noZ: true });
const splinePathB = makePath({ points: b, noZ: true });
const splinePathC = makePath({ points: c, noZ: true });
const splinePathD = makePath({ points: d, noZ: true });
console.log(originalPath);
console.log(splinePathA);
console.log(splinePathB);
console.log(splinePathC);
console.log(splinePathD);
以下が実行結果になります。
$ time node index.js
M 1,0 L -0.4999999999999998,0.8660254037844387 L -0.5000000000000004,-0.8660254037844385 Z
M -0.2499999999999999,0.4330127018922194 L -0.27907866544230164,0.41345369925640063 L -0.3057086011631467,0.3899961056051008 L -0.32988980716253435,0.36298265421440606 L -0.35162228344046526,0.33275607836040255 L -0.37090602999693906,0.29965911131917655 L -0.3877410468319559,0.2640344863668143 L -0.4021273339455158,0.2262249367794022 L -0.41406489133761853,0.1865731958330262 L -0.42355371900826455,0.14542199680377313 L -0.43059381695745347,0.10311407296772895 L -0.43518518518518534,0.059992157600979805 L -0.43732782369146017,0.016398983979611897 L -0.43702173247627807,-0.027322714620288235 L -0.4342669115396389,-0.07083020492263448 L -0.42906336088154284,-0.11378075365134047 L -0.42141108050198967,-0.1558316275303202 L -0.41131007040097967,-0.196640093283487 L -0.3987603305785126,-0.23586341763475505 L -0.38376186103458854,-0.2731588673080377 L -0.36631466176920746,-0.30818370902724884 L -0.34641873278236934,-0.34059520951630246 L -0.32407407407407435,-0.3700506354991119 L -0.2992806856443223,-0.39620725369959103 L -0.27203856749311317,-0.41872233084165383 L -0.24234849257806118,-0.43725447245107335 L -0.2103047617280861,-0.45162427907864844 L -0.17618332081142035,-0.46196696971220985 L -0.14028098555187654,-0.4684539109898009 L -0.10289457167326796,-0.47125646954946465 L -0.06432089489940754,-0.4705460120292442 L -0.024856770954108105,-0.4664939050671825 L 0.015200984438817185,-0.45927151530132315 L 0.05555555555555528,-0.44905020936970885 L 0.09591012667229348,-0.43600135391038297 L 0.13596788206521887,-0.4202963155613884 L 0.17543200601051825,-0.4021064609607685 L 0.21400568278437865,-0.3816031567465664 L 0.2513920966629871,-0.35895776955682523 L 0.28729443192253085,-0.3343416660295879 L 0.3214158728391968,-0.30792621280289767 L 0.353459603689172,-0.27988277651479776 L 0.3831288087486433,-0.25038272380333126 L 0.410126672293798,-0.21959742130654117 L 0.4341563786008229,-0.1876982356624709 L 0.45492111194590523,-0.15485653350916329 L 0.4721240566052319,-0.12124368148466164 L 0.48546839685499,-0.08703104622700897 L 0.4946573169713666,-0.05238999437424843 L 0.49939400123054845,-0.01749189256442328 L 0.49939400123054845,0.017491892564423513 L 0.49465731697136656,0.052389994374248516 L 0.48546839685499,0.08703104622700919 L 0.472124056605232,0.12124368148466166 L 0.45492111194590545,0.1548565335091632 L 0.43415637860082307,0.18769823566247107 L 0.4101266722937983,0.21959742130654128 L 0.38312880874864336,0.2503827238033315 L 0.3534596036891721,0.27988277651479787 L 0.32141587283919715,0.30792621280289767 L 0.2872944319225312,0.33434166602958804 L 0.2513920966629877,0.3589577695568252 L 0.21400568278437881,0.38160315674656664 L 0.17543200601051853,0.4021064609607687 L 0.135967882065219,0.4202963155613886 L 0.0959101266722939,0.43600135391038297 L 0.055555555555555844,0.44905020936970885 L 0.015200984438817254,0.45927151530132326 L -0.024856770954107835,0.46649390506718275 L -0.06432089489940734,0.47054601202924423 L -0.1028945716732676,0.47125646954946476 L -0.14028098555187601,0.46845391098980105 L -0.17618332081141994,0.4619669697122101 L -0.21030476172808565,0.4516242790786486 L -0.24234849257806107,0.4372544724510734 L -0.2720385674931129,0.41872233084165394 L -0.2992806856443218,0.3962072536995914 L -0.32407407407407407,0.370050635499112 L -0.346418732782369,0.3405952095163026 L -0.3663146617692073,0.3081837090272491 L -0.3837618610345883,0.273158867308038 L -0.3987603305785125,0.23586341763475496 L -0.41131007040097955,0.19664009328348725 L -0.4214110805019896,0.1558316275303205 L -0.42906336088154273,0.11378075365134054 L -0.4342669115396389,0.07083020492263467 L -0.43702173247627807,0.027322714620288277 L -0.43732782369146017,-0.01639898397961173 L -0.43518518518518534,-0.05999215760097932 L -0.43059381695745347,-0.10311407296772875 L -0.4235537190082646,-0.14542199680377288 L -0.4140648913376187,-0.18657319583302628 L -0.40212733394551603,-0.2262249367794019 L -0.38774104683195604,-0.2640344863668143 L -0.3709060299969393,-0.29965911131917633 L -0.3516222834404656,-0.33275607836040216 L -0.32988980716253463,-0.3629826542144059 L -0.3057086011631469,-0.38999610560510056 L -0.2790786654423019,-0.4134536992564007 L -0.2500000000000003,-0.4330127018922192
M -0.3750000000000001,1.249000902703301e-16 L -0.37377613482904226,-0.03493466868082155 L -0.3701105355934177,-0.06953872091855015 L -0.3640211911248727,-0.10350058100765216 L -0.3555380828096508,-0.13652944495629737 L -0.34470318458849325,-0.16835528048635678 L -0.3315704629566389,-0.19872882703340425 L -0.3162058769638239,-0.2274215957467157 L -0.29868737821428193,-0.25422586948926873 L -0.27910491086674427,-0.27895470283774415 L -0.2575604116344396,-0.30144192208252385 L -0.234167809785094,-0.3215421252276927 L -0.2090530271409312,-0.33913068199103746 L -0.1823539780786724,-0.35410373380404714 L -0.15422056952953636,-0.36637819381191306 L -0.12481470097923916,-0.37589174687352844 L -0.09431026446799434,-0.3826028495614891 L -0.0628931445905134,-0.3864907301620927 L -0.030761218496004567,-0.3875553886753392 L 0.0018756441118257458,-0.3858175968149308 L 0.03479558097477392,-0.38131889800827207 L 0.06776473728013914,-0.3741216073964693 L 0.10053726566072224,-0.36430881183433167 L 0.13285532619482754,-0.3519843698903699 L 0.1644490864062613,-0.33727291184679714 L 0.19503672712007197,-0.32031983631871624 L 0.2243280730210402,-0.3012892141501972 L 0.252044771531999,-0.2803521381334485 L 0.2779319808698767,-0.25767997490651295 L 0.3017588443605964,-0.23344409110743383 L 0.3233184904390763,-0.20781585337425434 L 0.34242803264922983,-0.1809666283450174 L 0.358928569643965,-0.1530677826577664 L 0.37268518518518506,-0.12429068295054445 L 0.38358694814378824,-0.09480669586139447 L 0.3915469124996678,-0.06478718802835959 L 0.3965021173417116,-0.034403526089483175 L 0.3984135868678029,-0.003827076682808253 L 0.3972663303848202,0.026770793553622066 L 0.393069342308636,0.05721871798176463 L 0.3858556021641189,0.0873453299635764 L 0.3756820745851318,0.11697926286101419 L 0.36262970931453276,0.14594915003603479 L 0.34680344120417483,0.17408362485059514 L 0.32833219021490634,0.20121132066665195 L 0.30736886141656994,0.2271608708461624 L 0.2840903449880039,0.2517609087510832 L 0.2586975162170412,0.27484006774337116 L 0.23141523550050974,0.2962269811849832 L 0.20249234834423266,0.31575028243787623 L 0.17220159167119584,0.3332386589570115 L 0.14083247324231554,0.34852496335869154 L 0.10866972253507477,0.36145865481158 L 0.07598617016429213,0.3719099101050381 L 0.043042654190287764,0.37976967774212933 L 0.010088020118884294,0.3849496779396194 L -0.022640879098592,0.387382402627976 L -0.054919183065313876,0.3870211154513692 L -0.08653402393895011,0.3838398517676715 L -0.11728452643166801,0.3778334186484571 L -0.14698180781013237,0.36901739487900265 L -0.17544897789550454,0.35742813095828724 L -0.20252113906344463,0.34312274909899143 L -0.22804538624410892,0.3261791432274992 L -0.25188080692215253,0.30669597898389517 L -0.27389848113672677,0.2847926937219677 L -0.2939814814814814,0.2606094965092062 L -0.3120248731045636,0.23430736812680264 L -0.3279357137086173,0.20606806106965164 L -0.34163305355078466,0.1760940995463491 L -0.353047935442705,0.1446087794791943 L -0.36212339475051536,0.11185616850418767 L -0.36881445939485014,0.07810110597103194 L -0.37308814985084104,0.04362920294313302 L -0.37492347914811774,0.008746842197597537 L -0.37431145287080686,-0.0262193694664339 L -0.3712550691575328,-0.06093609743239575 L -0.36576931870141755,-0.09508657982795526 L -0.35788118475008046,-0.12837481973285553 L -0.34762964310563826,-0.16052559194054308 L -0.33506566212470545,-0.19128444295816527 L -0.3202522027183937,-0.2204176910065732 L -0.30326421835231254,-0.24771242602031848 L -0.2841886550465687,-0.27297650964765635 L -0.26312445137576634,-0.29603857525054356 L -0.2401825384690076,-0.3167480279046391 L -0.21548584000989152,-0.33497504439930437 L -0.18916927223651514,-0.35061057323760264 L -0.1613797439414727,-0.3635663346362997 L -0.13227615647185562,-0.3737748205258634 L -0.10202940372925381,-0.3811892945504638 L -0.07082237216975339,-0.3857837920679731 L -0.038849940803939276,-0.38755312014996574 L -0.006318981196892545,-0.3865128575817185 L 0.026551642531806745,-0.3826993548622101 L 0.05953107370808225,-0.3761697342041216 L 0.09237646310336006,-0.3670018895338361 L 0.12483296893456777,-0.3552944864914394 L 0.15663375686413686,-0.3411669624307188 L 0.18749999999999983,-0.3247595264191644
M -0.16250000000000023,-0.28145825622994236 L -0.13918576706209368,-0.2935061001251451 L -0.11480220937141287,-0.3033595055732457 L -0.08951390656789908,-0.31096289774932595 L -0.0634945235600516,-0.31627979501985104 L -0.03692535688195975,-0.319291969681509 L -0.009993881050332349,-0.31999860870005137 L 0.01710770507847123,-0.3184154744491336 L 0.04418393195141025,-0.3145740654491549 L 0.07103751296173252,-0.3085207771060993 L 0.0974707980919431,-0.30031606245037534 L 0.12328722755677474,-0.2900335928756564 L 0.14829278544615596,-0.2777594188777216 L 0.17229745336818145,-0.2635911307932954 L 0.19511666409208123,-0.24763701953888836 L 0.2165727551911896,-0.23001523734963747 L 0.2364964226859152,-0.21085295851814614 L 0.25472817468670966,-0.19028554013332485 L 0.271119785037038,-0.16845568281923112 L 0.2855357469563467,-0.14551259147391038 L 0.29785472668303453,-0.12161113600823553 L 0.307971017117421,-0.09691101208474764 L 0.31579599146471565,-0.07157590185649697 L 0.32125955687798824,-0.04577263470588169 L 0.32431160810113746,-0.01967034798348967 L 0.324923481088201,0.006560352253061997 L 0.323089332828518,0.03274823050028617 L 0.3188265673606497,0.058722261069985876 L 0.3121747510291694,0.08431246735041409 L 0.3031941645909644,0.10935076106743227 L 0.2919643495722658,0.1336717815456714 L 0.27858265462568005,0.1571137349696912 L 0.2631627818872198,0.17951923364513933 L 0.24583333333333346,0.20073613525991152 L 0.22673635713793613,0.22061838214531185 L 0.2060258940294408,0.23902684053721102 L 0.18386652364778855,0.25583013983720715 L 0.16043191090147918,0.27090551187378514 L 0.1359033523246015,0.2841396301634763 L 0.11046832243386451,0.29542944917201797 L 0.08431902008562761,0.30468304357551373 L 0.057650914832931374,0.31182044752159216 L 0.030661293282528188,0.3167744938905672 L 0.0035478054519127687,0.31949165355659787 L -0.023492988873647248,0.3199328746488474 L -0.05026707378408083,0.31807442181264356 L -0.07658443088748236,0.31390871547063803 L -0.10226049295308189,0.30744517108396574 L -0.12711759755421392,0.29871103841340546 L -0.15098644071128683,0.2877522407805387 L -0.1737075301561996,0.2746342136732366 L -0.19513254887973142,0.2594425879566986 L -0.21512508195302632,0.24228277024287137 L -0.23356070662317244,0.22327842041825244 L -0.25032699269175596,0.2025697737772427 L -0.2653235025148606,0.18031196249982745 L -0.2784617910030669,0.15667333712925763 L -0.28966540562145376,0.13183378804972895 L -0.2988698863895969,0.10598306696406448 L -0.3060227658815697,0.0793191083713933 L -0.3110835692259435,0.05204635104483203 L -0.3140238141057864,0.024374059509166546 L -0.31482701075866437,-0.003485354481469999 L -0.31348866197664105,-0.03131801046591344 L -0.31001626310627717,-0.05891023779829091 L -0.3044293020486314,-0.08605025417033775 L -0.2967592592592594,-0.11252984413371855 L -0.28704960774821464,-0.13814603762234612 L -0.27535581308004825,-0.1627027884746997 L -0.2617453333738083,-0.18601265295614666 L -0.246297619303041,-0.20789846828125919 L -0.22910411409578962,-0.22819503113613593 L -0.2102682535345948,-0.24675077620072014 L -0.18990546595649538,-0.2634294546711191 L -0.16814317225302675,-0.2781118127819238 L -0.1451207829955869,-0.29069726534951296 L -0.12098951398555567,-0.3011052548178103 L -0.09591144642584987,-0.309275301949986 L -0.07005811018685852,-0.3151662304953926 L -0.043609030175300996,-0.3187553279488964 L -0.0167502726912603,-0.3200375062897166 L 0.01032700821478906,-0.31902446272026647 L 0.037428024384947696,-0.3157438404049932 L 0.06435580719686321,-0.3102383892092181 L 0.0909126612066984,-0.3025651264379771 L 0.1169016177920999,-0.2927944975748616 L 0.14212788879516958,-0.2810095370208576 L 0.1664003201654317,-0.26730502883318724 L 0.18953284560280417,-0.2517866674641482 L 0.21134594020056724,-0.23457021849995435 L 0.23166807408833207,-0.2157806793995765 L 0.25033716607501166,-0.19555144023358179 L 0.2672020372917887,-0.17402344442297513 L 0.28212386483508667,-0.15134434947803804 L 0.29497763540953736,-0.12766768773717094 L 0.3056535989709517,-0.10315202710573149 L 0.3140587223692885,-0.07796013179487582 L 0.32011814299162406,-0.05225812306039954 L 0.32377662240512145,-0.026214639941576252 L 0.32499999999999996,2.7755575615628914e-17
M -0.12368303571428581,1.1102230246251565e-16 L -0.12324110550259527,-0.010469114731970734 L -0.12191845924406107,-0.020863086825182082 L -0.1197245080793367,-0.031107317792466255 L -0.11667486408990224,-0.041128293355888794 L -0.1127912310704995,-0.050854115354787725 L -0.10810125261170303,-0.060215021523381 L -0.10263831848787121,-0.06914388926610189 L -0.09644133062162741,-0.07757671969952334 L -0.0895544301655816,-0.08545309839972252 L -0.08202668750382382,-0.09271662949075438 L -0.0739117572283752,-0.09931533993113323 L -0.06526750038788147,-0.10520205109840142 L -0.056155576535949464,-0.1103347150345701 L -0.0466410083232678,-0.11467671299499765 L -0.03679172157959698,-0.11819711423769967 L -0.02667806401745872,-0.12087089329670131 L -0.016372305857493597,-0.12267910429943198 L -0.005948125824571254,-0.12360901121185941 L 0.004519913907566481,-0.12365417322364872 L 0.014956900138496928,-0.12281448481665069 L 0.025288104661889667,-0.12109617039104872 L 0.03543952005765541,-0.11851173365207542 L 0.045338391395359265,-0.11507986228391438 L 0.05491373998336149,-0.1108252887537839 L 0.0640968753215946,-0.10577860839582438 L 0.07282189146962646,-0.0999760562188325 L 0.08102614412708528,-0.09345924416167273 L 0.0886507048419375,-0.08627486078297096 L 0.09564078891404132,-0.0784743356155242 L 0.10194615374568716,-0.07011347063979276 L 0.10752146460503334,-0.06125204153465137 L 0.11232662500966514,-0.051953371547263094 L 0.11632706920311936,-0.042283880987491716 L 0.11949401448431832,-0.032312615495692996 L 0.12180467145562025,-0.022110756356023838 L 0.12324241057680957,-0.011751116230567009 L 0.12379688374699092,-0.0013076237726103845 L 0.12346409998121188,0.009145199359678582 L 0.12224645460088815,0.01953276552516408 L 0.12015271171394087,0.029780948570576553 L 0.11719794011914383,0.03981660816109014 L 0.1134034031267169,0.04956810739907554 L 0.10879640314086263,0.058965820197206774 L 0.10341008219691283,0.06794262492997137 L 0.0972831799832147,0.07643438096563476 L 0.0904597512030203,0.08438038477884824 L 0.08298884444163415,0.09172380246234463 L 0.07492414499610671,0.09841207559456383 L 0.06632358439600847,0.10439729757856911 L 0.057248919591480145,0.1096365577462691 L 0.04776528500599331,0.1140922507207391 L 0.03794072084327888,0.11773234874834257 L 0.02784568119807806,0.1205306349512597 L 0.01755252564717005,0.12246689570921512 L 0.007134998090696565,0.12352707065494911 L -0.00333230332466476,0.12370335905896368 L -0.013774461338243789,0.12299428168288228 L -0.024116775899537174,0.12140469749493109 L -0.03428529784605336,0.1189457749631493 L -0.04420735614948723,0.11563491796953235 L -0.05381207502245122,0.11149564671895074 L -0.0630308772791687,0.10655743434793791 L -0.07179797046689958,0.10085550026786566 L -0.08005081242901531,0.09443056160217247 L -0.08773055312414814,0.08732854439575893 L -0.09478244970732541,0.07960025658394612 L -0.10115625207701424,0.071301025006107 L -0.10680655630518449,0.06249029903274267 L -0.1116931235943987,0.05323122364198289 L -0.11578116264517081,0.043590185029782895 L -0.11904157356698757,0.03363633206502866 L -0.12145115172604394,0.023441077103918996 L -0.12299275019050364,0.013077579854913786 L -0.12365539970854729,0.002620218133792647 L -0.12343438543420367,-0.007855950534487536 L -0.12233127989956441,-0.01827573242685409 L -0.12035393201806034,-0.028564342158179788 L -0.11751641219060222,-0.038647944144768014 L -0.11383891387316644,-0.04845418692651988 L -0.1093476122494248,-0.05791272634495294 L -0.10407448093386029,-0.06695573367491468 L -0.09805706790808232,-0.07551838494083402 L -0.09133823216433254,-0.08353932781125722 L -0.08396584279406089,-0.0909611226557448 L -0.07599244251453137,-0.09773065456357005 L -0.06747487787128001,-0.1037995133615785 L -0.058473898587503736,-0.10912433892661666 L -0.049053728751660995,-0.1136671293636875 L -0.03928161274035101,-0.11739550991197605 L -0.02922733896346052,-0.1202829607446943 L -0.01896274469123854,-0.12230900214286494 L -0.008561205376967062,-0.12345933584526533 L 0.0019028879771765496,-0.1237259417043441 L 0.012354659751019051,-0.123107129108567 L 0.022719285184452417,-0.12160754296289633 L 0.03292252658779669,-0.11923812434853386 L 0.042891266430062046,-0.11601602630820554 L 0.052554033438276956,-0.1119644855217109 L 0.06184151785714272,-0.10711265094574933
real 0m0.697s
user 0m0.720s
sys 0m0.108s
図形的には梅干しありのおにぎり🍙スケルトンですね。
M 1,0 L -0.4999999999999998,0.8660254037844387 L -0.5000000000000004,-0.8660254037844385 Z
M -0.2499999999999999,0.4330127018922194 L -0.27907866544230164,0.41345369925640063 L -0.3057086011631467,0.3899961056051008 L -0.32988980716253435,0.36298265421440606 L -0.35162228344046526,0.33275607836040255 L -0.37090602999693906,0.29965911131917655 L -0.3877410468319559,0.2640344863668143 L -0.4021273339455158,0.2262249367794022 L -0.41406489133761853,0.1865731958330262 L -0.42355371900826455,0.14542199680377313 L -0.43059381695745347,0.10311407296772895 L -0.43518518518518534,0.059992157600979805 L -0.43732782369146017,0.016398983979611897 L -0.43702173247627807,-0.027322714620288235 L -0.4342669115396389,-0.07083020492263448 L -0.42906336088154284,-0.11378075365134047 L -0.42141108050198967,-0.1558316275303202 L -0.41131007040097967,-0.196640093283487 L -0.3987603305785126,-0.23586341763475505 L -0.38376186103458854,-0.2731588673080377 L -0.36631466176920746,-0.30818370902724884 L -0.34641873278236934,-0.34059520951630246 L -0.32407407407407435,-0.3700506354991119 L -0.2992806856443223,-0.39620725369959103 L -0.27203856749311317,-0.41872233084165383 L -0.24234849257806118,-0.43725447245107335 L -0.2103047617280861,-0.45162427907864844 L -0.17618332081142035,-0.46196696971220985 L -0.14028098555187654,-0.4684539109898009 L -0.10289457167326796,-0.47125646954946465 L -0.06432089489940754,-0.4705460120292442 L -0.024856770954108105,-0.4664939050671825 L 0.015200984438817185,-0.45927151530132315 L 0.05555555555555528,-0.44905020936970885 L 0.09591012667229348,-0.43600135391038297 L 0.13596788206521887,-0.4202963155613884 L 0.17543200601051825,-0.4021064609607685 L 0.21400568278437865,-0.3816031567465664 L 0.2513920966629871,-0.35895776955682523 L 0.28729443192253085,-0.3343416660295879 L 0.3214158728391968,-0.30792621280289767 L 0.353459603689172,-0.27988277651479776 L 0.3831288087486433,-0.25038272380333126 L 0.410126672293798,-0.21959742130654117 L 0.4341563786008229,-0.1876982356624709 L 0.45492111194590523,-0.15485653350916329 L 0.4721240566052319,-0.12124368148466164 L 0.48546839685499,-0.08703104622700897 L 0.4946573169713666,-0.05238999437424843 L 0.49939400123054845,-0.01749189256442328 L 0.49939400123054845,0.017491892564423513 L 0.49465731697136656,0.052389994374248516 L 0.48546839685499,0.08703104622700919 L 0.472124056605232,0.12124368148466166 L 0.45492111194590545,0.1548565335091632 L 0.43415637860082307,0.18769823566247107 L 0.4101266722937983,0.21959742130654128 L 0.38312880874864336,0.2503827238033315 L 0.3534596036891721,0.27988277651479787 L 0.32141587283919715,0.30792621280289767 L 0.2872944319225312,0.33434166602958804 L 0.2513920966629877,0.3589577695568252 L 0.21400568278437881,0.38160315674656664 L 0.17543200601051853,0.4021064609607687 L 0.135967882065219,0.4202963155613886 L 0.0959101266722939,0.43600135391038297 L 0.055555555555555844,0.44905020936970885 L 0.015200984438817254,0.45927151530132326 L -0.024856770954107835,0.46649390506718275 L -0.06432089489940734,0.47054601202924423 L -0.1028945716732676,0.47125646954946476 L -0.14028098555187601,0.46845391098980105 L -0.17618332081141994,0.4619669697122101 L -0.21030476172808565,0.4516242790786486 L -0.24234849257806107,0.4372544724510734 L -0.2720385674931129,0.41872233084165394 L -0.2992806856443218,0.3962072536995914 L -0.32407407407407407,0.370050635499112 L -0.346418732782369,0.3405952095163026 L -0.3663146617692073,0.3081837090272491 L -0.3837618610345883,0.273158867308038 L -0.3987603305785125,0.23586341763475496 L -0.41131007040097955,0.19664009328348725 L -0.4214110805019896,0.1558316275303205 L -0.42906336088154273,0.11378075365134054 L -0.4342669115396389,0.07083020492263467 L -0.43702173247627807,0.027322714620288277 L -0.43732782369146017,-0.01639898397961173 L -0.43518518518518534,-0.05999215760097932 L -0.43059381695745347,-0.10311407296772875 L -0.4235537190082646,-0.14542199680377288 L -0.4140648913376187,-0.18657319583302628 L -0.40212733394551603,-0.2262249367794019 L -0.38774104683195604,-0.2640344863668143 L -0.3709060299969393,-0.29965911131917633 L -0.3516222834404656,-0.33275607836040216 L -0.32988980716253463,-0.3629826542144059 L -0.3057086011631469,-0.38999610560510056 L -0.2790786654423019,-0.4134536992564007 L -0.2500000000000003,-0.4330127018922192
M -0.3750000000000001,1.249000902703301e-16 L -0.37377613482904226,-0.03493466868082155 L -0.3701105355934177,-0.06953872091855015 L -0.3640211911248727,-0.10350058100765216 L -0.3555380828096508,-0.13652944495629737 L -0.34470318458849325,-0.16835528048635678 L -0.3315704629566389,-0.19872882703340425 L -0.3162058769638239,-0.2274215957467157 L -0.29868737821428193,-0.25422586948926873 L -0.27910491086674427,-0.27895470283774415 L -0.2575604116344396,-0.30144192208252385 L -0.234167809785094,-0.3215421252276927 L -0.2090530271409312,-0.33913068199103746 L -0.1823539780786724,-0.35410373380404714 L -0.15422056952953636,-0.36637819381191306 L -0.12481470097923916,-0.37589174687352844 L -0.09431026446799434,-0.3826028495614891 L -0.0628931445905134,-0.3864907301620927 L -0.030761218496004567,-0.3875553886753392 L 0.0018756441118257458,-0.3858175968149308 L 0.03479558097477392,-0.38131889800827207 L 0.06776473728013914,-0.3741216073964693 L 0.10053726566072224,-0.36430881183433167 L 0.13285532619482754,-0.3519843698903699 L 0.1644490864062613,-0.33727291184679714 L 0.19503672712007197,-0.32031983631871624 L 0.2243280730210402,-0.3012892141501972 L 0.252044771531999,-0.2803521381334485 L 0.2779319808698767,-0.25767997490651295 L 0.3017588443605964,-0.23344409110743383 L 0.3233184904390763,-0.20781585337425434 L 0.34242803264922983,-0.1809666283450174 L 0.358928569643965,-0.1530677826577664 L 0.37268518518518506,-0.12429068295054445 L 0.38358694814378824,-0.09480669586139447 L 0.3915469124996678,-0.06478718802835959 L 0.3965021173417116,-0.034403526089483175 L 0.3984135868678029,-0.003827076682808253 L 0.3972663303848202,0.026770793553622066 L 0.393069342308636,0.05721871798176463 L 0.3858556021641189,0.0873453299635764 L 0.3756820745851318,0.11697926286101419 L 0.36262970931453276,0.14594915003603479 L 0.34680344120417483,0.17408362485059514 L 0.32833219021490634,0.20121132066665195 L 0.30736886141656994,0.2271608708461624 L 0.2840903449880039,0.2517609087510832 L 0.2586975162170412,0.27484006774337116 L 0.23141523550050974,0.2962269811849832 L 0.20249234834423266,0.31575028243787623 L 0.17220159167119584,0.3332386589570115 L 0.14083247324231554,0.34852496335869154 L 0.10866972253507477,0.36145865481158 L 0.07598617016429213,0.3719099101050381 L 0.043042654190287764,0.37976967774212933 L 0.010088020118884294,0.3849496779396194 L -0.022640879098592,0.387382402627976 L -0.054919183065313876,0.3870211154513692 L -0.08653402393895011,0.3838398517676715 L -0.11728452643166801,0.3778334186484571 L -0.14698180781013237,0.36901739487900265 L -0.17544897789550454,0.35742813095828724 L -0.20252113906344463,0.34312274909899143 L -0.22804538624410892,0.3261791432274992 L -0.25188080692215253,0.30669597898389517 L -0.27389848113672677,0.2847926937219677 L -0.2939814814814814,0.2606094965092062 L -0.3120248731045636,0.23430736812680264 L -0.3279357137086173,0.20606806106965164 L -0.34163305355078466,0.1760940995463491 L -0.353047935442705,0.1446087794791943 L -0.36212339475051536,0.11185616850418767 L -0.36881445939485014,0.07810110597103194 L -0.37308814985084104,0.04362920294313302 L -0.37492347914811774,0.008746842197597537 L -0.37431145287080686,-0.0262193694664339 L -0.3712550691575328,-0.06093609743239575 L -0.36576931870141755,-0.09508657982795526 L -0.35788118475008046,-0.12837481973285553 L -0.34762964310563826,-0.16052559194054308 L -0.33506566212470545,-0.19128444295816527 L -0.3202522027183937,-0.2204176910065732 L -0.30326421835231254,-0.24771242602031848 L -0.2841886550465687,-0.27297650964765635 L -0.26312445137576634,-0.29603857525054356 L -0.2401825384690076,-0.3167480279046391 L -0.21548584000989152,-0.33497504439930437 L -0.18916927223651514,-0.35061057323760264 L -0.1613797439414727,-0.3635663346362997 L -0.13227615647185562,-0.3737748205258634 L -0.10202940372925381,-0.3811892945504638 L -0.07082237216975339,-0.3857837920679731 L -0.038849940803939276,-0.38755312014996574 L -0.006318981196892545,-0.3865128575817185 L 0.026551642531806745,-0.3826993548622101 L 0.05953107370808225,-0.3761697342041216 L 0.09237646310336006,-0.3670018895338361 L 0.12483296893456777,-0.3552944864914394 L 0.15663375686413686,-0.3411669624307188 L 0.18749999999999983,-0.3247595264191644
M -0.16250000000000023,-0.28145825622994236 L -0.13918576706209368,-0.2935061001251451 L -0.11480220937141287,-0.3033595055732457 L -0.08951390656789908,-0.31096289774932595 L -0.0634945235600516,-0.31627979501985104 L -0.03692535688195975,-0.319291969681509 L -0.009993881050332349,-0.31999860870005137 L 0.01710770507847123,-0.3184154744491336 L 0.04418393195141025,-0.3145740654491549 L 0.07103751296173252,-0.3085207771060993 L 0.0974707980919431,-0.30031606245037534 L 0.12328722755677474,-0.2900335928756564 L 0.14829278544615596,-0.2777594188777216 L 0.17229745336818145,-0.2635911307932954 L 0.19511666409208123,-0.24763701953888836 L 0.2165727551911896,-0.23001523734963747 L 0.2364964226859152,-0.21085295851814614 L 0.25472817468670966,-0.19028554013332485 L 0.271119785037038,-0.16845568281923112 L 0.2855357469563467,-0.14551259147391038 L 0.29785472668303453,-0.12161113600823553 L 0.307971017117421,-0.09691101208474764 L 0.31579599146471565,-0.07157590185649697 L 0.32125955687798824,-0.04577263470588169 L 0.32431160810113746,-0.01967034798348967 L 0.324923481088201,0.006560352253061997 L 0.323089332828518,0.03274823050028617 L 0.3188265673606497,0.058722261069985876 L 0.3121747510291694,0.08431246735041409 L 0.3031941645909644,0.10935076106743227 L 0.2919643495722658,0.1336717815456714 L 0.27858265462568005,0.1571137349696912 L 0.2631627818872198,0.17951923364513933 L 0.24583333333333346,0.20073613525991152 L 0.22673635713793613,0.22061838214531185 L 0.2060258940294408,0.23902684053721102 L 0.18386652364778855,0.25583013983720715 L 0.16043191090147918,0.27090551187378514 L 0.1359033523246015,0.2841396301634763 L 0.11046832243386451,0.29542944917201797 L 0.08431902008562761,0.30468304357551373 L 0.057650914832931374,0.31182044752159216 L 0.030661293282528188,0.3167744938905672 L 0.0035478054519127687,0.31949165355659787 L -0.023492988873647248,0.3199328746488474 L -0.05026707378408083,0.31807442181264356 L -0.07658443088748236,0.31390871547063803 L -0.10226049295308189,0.30744517108396574 L -0.12711759755421392,0.29871103841340546 L -0.15098644071128683,0.2877522407805387 L -0.1737075301561996,0.2746342136732366 L -0.19513254887973142,0.2594425879566986 L -0.21512508195302632,0.24228277024287137 L -0.23356070662317244,0.22327842041825244 L -0.25032699269175596,0.2025697737772427 L -0.2653235025148606,0.18031196249982745 L -0.2784617910030669,0.15667333712925763 L -0.28966540562145376,0.13183378804972895 L -0.2988698863895969,0.10598306696406448 L -0.3060227658815697,0.0793191083713933 L -0.3110835692259435,0.05204635104483203 L -0.3140238141057864,0.024374059509166546 L -0.31482701075866437,-0.003485354481469999 L -0.31348866197664105,-0.03131801046591344 L -0.31001626310627717,-0.05891023779829091 L -0.3044293020486314,-0.08605025417033775 L -0.2967592592592594,-0.11252984413371855 L -0.28704960774821464,-0.13814603762234612 L -0.27535581308004825,-0.1627027884746997 L -0.2617453333738083,-0.18601265295614666 L -0.246297619303041,-0.20789846828125919 L -0.22910411409578962,-0.22819503113613593 L -0.2102682535345948,-0.24675077620072014 L -0.18990546595649538,-0.2634294546711191 L -0.16814317225302675,-0.2781118127819238 L -0.1451207829955869,-0.29069726534951296 L -0.12098951398555567,-0.3011052548178103 L -0.09591144642584987,-0.309275301949986 L -0.07005811018685852,-0.3151662304953926 L -0.043609030175300996,-0.3187553279488964 L -0.0167502726912603,-0.3200375062897166 L 0.01032700821478906,-0.31902446272026647 L 0.037428024384947696,-0.3157438404049932 L 0.06435580719686321,-0.3102383892092181 L 0.0909126612066984,-0.3025651264379771 L 0.1169016177920999,-0.2927944975748616 L 0.14212788879516958,-0.2810095370208576 L 0.1664003201654317,-0.26730502883318724 L 0.18953284560280417,-0.2517866674641482 L 0.21134594020056724,-0.23457021849995435 L 0.23166807408833207,-0.2157806793995765 L 0.25033716607501166,-0.19555144023358179 L 0.2672020372917887,-0.17402344442297513 L 0.28212386483508667,-0.15134434947803804 L 0.29497763540953736,-0.12766768773717094 L 0.3056535989709517,-0.10315202710573149 L 0.3140587223692885,-0.07796013179487582 L 0.32011814299162406,-0.05225812306039954 L 0.32377662240512145,-0.026214639941576252 L 0.32499999999999996,2.7755575615628914e-17
M -0.12368303571428581,1.1102230246251565e-16 L -0.12324110550259527,-0.010469114731970734 L -0.12191845924406107,-0.020863086825182082 L -0.1197245080793367,-0.031107317792466255 L -0.11667486408990224,-0.041128293355888794 L -0.1127912310704995,-0.050854115354787725 L -0.10810125261170303,-0.060215021523381 L -0.10263831848787121,-0.06914388926610189 L -0.09644133062162741,-0.07757671969952334 L -0.0895544301655816,-0.08545309839972252 L -0.08202668750382382,-0.09271662949075438 L -0.0739117572283752,-0.09931533993113323 L -0.06526750038788147,-0.10520205109840142 L -0.056155576535949464,-0.1103347150345701 L -0.0466410083232678,-0.11467671299499765 L -0.03679172157959698,-0.11819711423769967 L -0.02667806401745872,-0.12087089329670131 L -0.016372305857493597,-0.12267910429943198 L -0.005948125824571254,-0.12360901121185941 L 0.004519913907566481,-0.12365417322364872 L 0.014956900138496928,-0.12281448481665069 L 0.025288104661889667,-0.12109617039104872 L 0.03543952005765541,-0.11851173365207542 L 0.045338391395359265,-0.11507986228391438 L 0.05491373998336149,-0.1108252887537839 L 0.0640968753215946,-0.10577860839582438 L 0.07282189146962646,-0.0999760562188325 L 0.08102614412708528,-0.09345924416167273 L 0.0886507048419375,-0.08627486078297096 L 0.09564078891404132,-0.0784743356155242 L 0.10194615374568716,-0.07011347063979276 L 0.10752146460503334,-0.06125204153465137 L 0.11232662500966514,-0.051953371547263094 L 0.11632706920311936,-0.042283880987491716 L 0.11949401448431832,-0.032312615495692996 L 0.12180467145562025,-0.022110756356023838 L 0.12324241057680957,-0.011751116230567009 L 0.12379688374699092,-0.0013076237726103845 L 0.12346409998121188,0.009145199359678582 L 0.12224645460088815,0.01953276552516408 L 0.12015271171394087,0.029780948570576553 L 0.11719794011914383,0.03981660816109014 L 0.1134034031267169,0.04956810739907554 L 0.10879640314086263,0.058965820197206774 L 0.10341008219691283,0.06794262492997137 L 0.0972831799832147,0.07643438096563476 L 0.0904597512030203,0.08438038477884824 L 0.08298884444163415,0.09172380246234463 L 0.07492414499610671,0.09841207559456383 L 0.06632358439600847,0.10439729757856911 L 0.057248919591480145,0.1096365577462691 L 0.04776528500599331,0.1140922507207391 L 0.03794072084327888,0.11773234874834257 L 0.02784568119807806,0.1205306349512597 L 0.01755252564717005,0.12246689570921512 L 0.007134998090696565,0.12352707065494911 L -0.00333230332466476,0.12370335905896368 L -0.013774461338243789,0.12299428168288228 L -0.024116775899537174,0.12140469749493109 L -0.03428529784605336,0.1189457749631493 L -0.04420735614948723,0.11563491796953235 L -0.05381207502245122,0.11149564671895074 L -0.0630308772791687,0.10655743434793791 L -0.07179797046689958,0.10085550026786566 L -0.08005081242901531,0.09443056160217247 L -0.08773055312414814,0.08732854439575893 L -0.09478244970732541,0.07960025658394612 L -0.10115625207701424,0.071301025006107 L -0.10680655630518449,0.06249029903274267 L -0.1116931235943987,0.05323122364198289 L -0.11578116264517081,0.043590185029782895 L -0.11904157356698757,0.03363633206502866 L -0.12145115172604394,0.023441077103918996 L -0.12299275019050364,0.013077579854913786 L -0.12365539970854729,0.002620218133792647 L -0.12343438543420367,-0.007855950534487536 L -0.12233127989956441,-0.01827573242685409 L -0.12035393201806034,-0.028564342158179788 L -0.11751641219060222,-0.038647944144768014 L -0.11383891387316644,-0.04845418692651988 L -0.1093476122494248,-0.05791272634495294 L -0.10407448093386029,-0.06695573367491468 L -0.09805706790808232,-0.07551838494083402 L -0.09133823216433254,-0.08353932781125722 L -0.08396584279406089,-0.0909611226557448 L -0.07599244251453137,-0.09773065456357005 L -0.06747487787128001,-0.1037995133615785 L -0.058473898587503736,-0.10912433892661666 L -0.049053728751660995,-0.1136671293636875 L -0.03928161274035101,-0.11739550991197605 L -0.02922733896346052,-0.1202829607446943 L -0.01896274469123854,-0.12230900214286494 L -0.008561205376967062,-0.12345933584526533 L 0.0019028879771765496,-0.1237259417043441 L 0.012354659751019051,-0.123107129108567 L 0.022719285184452417,-0.12160754296289633 L 0.03292252658779669,-0.11923812434853386 L 0.042891266430062046,-0.11601602630820554 L 0.052554033438276956,-0.1119644855217109 L 0.06184151785714272,-0.10711265094574933
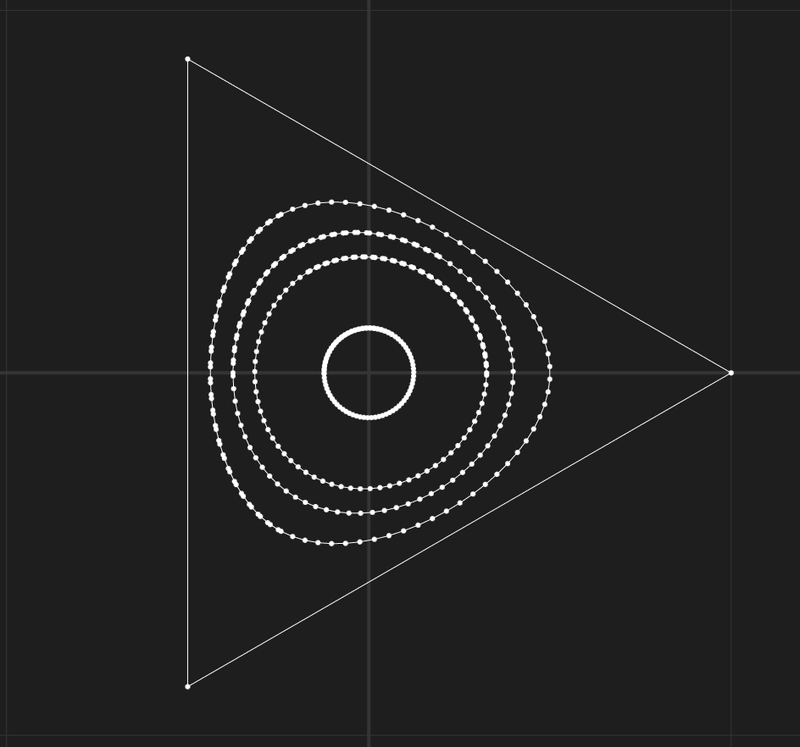
いろいろパラメータ調節してみると面白いかもしれません。
最近では、Twitterでモックアップ動画を公開しているので、こちらもよかったら、覗いてみてください。
最後に、Udemyでコースを公開しました。
良かったら覗いてみてください。
また、コースの内容紹介記事は以下になります。
簡単ですが、以上です。
この記事が気に入ったらサポートをしてみませんか?