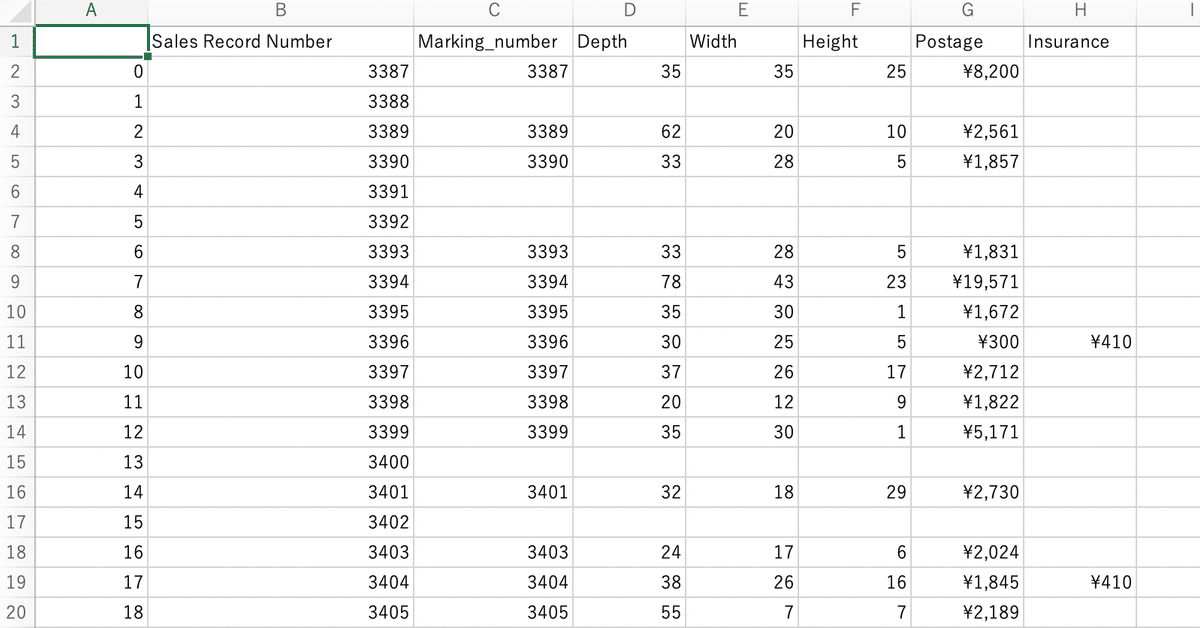
商品の管理番号とラベル作成ツール(Hirogete)の管理番号で一致させて、売上管理表に送料を自動記入させるツールを作った(Python/Selenium)
はじめに
こんにちは。ユウタ@物販×IT(https://twitter.com/ebay_yuta)です。
今回は、商品の管理番号とラベル作成ツール(Hirogete)の管理番号で一致させて、売上管理表に自動記入させるツールを開発しました。
このアイデアは、ランサーズでツール案件を探してる時に、偶然見つけた求人を参考にしたものです。投稿者に感謝だ…。
余談ですが、ランサーズやクラウドワークスで過去の案件を見てみると、かなり有料級の情報(外注のやり方やツールのアイデアなど)がしれっと載ってます。
今回も、Seleniumを使って、自動化していきたいと思います。
処理の流れ
①【ラベル作成ツール(Hirogete)にログイン】
↓
②【発送完了画面を開く】
↓
③【管理番号,(W)幅×(D)奥行×(H)高さ,発送料金,保険金額】をスクレイピングし、情報を抜き取る
↓
④【商品の管理番号がある売上管理表と③で抜き取った管理番号を基準にデータを並び替えて一つのCSVにまとめる】
***動作環境***
Mac OS Mojave
Python 3.7.6
VSCode
ChromeDriver
コードの解説
まずは、下記をインポートします。
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
import time
import json
import requests
from bs4 import BeautifulSoup
import pandas as pd
import csv
次に、addressとpasswordに自分のログイン情報を入力してください。ダウンロードしたChromedriverが存在するパスを指定する。
address= "***"
password="***"
df1 = pd.read_csv('record.csv')
driver = webdriver.Chrome(executable_path='***')
df1の中身は、A列にFileexchangeで抜き出した商品の管理番号のみ貼り付けてある状態です。
次に、hirogete に自動でログインさせ、発送完了画面まで、移動させます。
driver.get('https://main.hirogete.com/#!/login/#page-top')
time.sleep(1)
driver.find_element_by_name("email").send_keys(address)
driver.find_element_by_name("password").send_keys(password)
driver.find_element_by_class_name("btn-primary").click()
time.sleep(5)
#↓のポップアップのxpathは、都度変更してください。
driver.find_element_by_xpath("//*[@id='modal-notice-content-127']/div[1]/div[2]").click()
time.sleep(1)
driver.find_element_by_xpath("//*[@id='modal-notice-content-128']/div[1]/div[2]").click()
BeautifulSoupで必要な情報のみ、抜き取っていきます。多分もっと綺麗なやり方があるけど、いつ間にか複雑になってた。
html = driver.page_source.encode('utf-8')
soup = BeautifulSoup(html, "lxml")
POSTS1 = "tr-odd"
POSTS2 = "tr-even"
posts1 = soup.find_all(class_=POSTS1)
posts2 = soup.find_all(class_=POSTS2)
Marking_number= []
Depth = []
Width = []
Height = []
Postage = []
Insurance = []
Record_number = []
for post in posts1:
try:
marking1 = post.find('input',{'name': 'marking_no'})['value']
Depth1 = post.find('input', {'name':'bundle_depth'})['value']
Width1 = post.find('input', {'name':'bundle_width'})['value']
Height1 = post.find('input', {'name':'bundle_height'})['value']
Postage1 = post.findAll('td')[20].string
Insurance1 = post.findAll('td')[21].string
except Exception as e:
marking1 = ""
Depth1 = ""
Width1 = ""
Height1 = ""
Postage1 = ""
Insurance1 = ""
Marking_number.append(marking1)
Depth.append(Depth1)
Width.append(Width1)
Height.append(Height1)
Postage.append(Postage1)
Insurance.append(Insurance1)
for post2 in posts2:
try:
marking2 = post2.find('input',{'name': 'marking_no'})['value']
Depth2 = post2.find('input', {'name':'bundle_depth'})['value']
Width2 = post2.find('input', {'name':'bundle_width'})['value']
Height2 = post2.find('input', {'name':'bundle_height'})['value']
Postage2 = post2.findAll('td')[20].string
Insurance2 = post2.findAll('td')[21].string
except Exception as e:
marking2 = ""
Depth2 = ""
Width2 = ""
Height2 = ""
Postage2 = ""
Insurance2 = ""
Marking_number.append(marking2)
Depth.append(Depth2)
Width.append(Width2)
Height.append(Height2)
Postage.append(Postage2)
Insurance.append(Insurance2)
商品の管理番号がある売上管理表と抜き取った管理番号を基準にデータを並び替えて一つのCSVにまとめる
for i, rows in df1.iterrows():
record = rows["Sales Record Number"]
Record_number.append(record)
rows = zip(Marking_number,Depth,Width,Height,Postage,Insurance)
rows2 = Record_number
df1 = pd.DataFrame(rows2, columns=["Sales Record Number"]).astype(str)
df2 = pd.DataFrame(rows, columns=["Marking_number","Depth","Width","Height","Postage","Insurance"]).astype(str)
ret = df1.merge(df2, left_on='Sales Record Number', right_on='Marking_number', how='left')
ret.to_csv("postage.csv",encoding='shift_jis')
print("DONE")
処理結果
良い感じに、並び替えがされてますね🤗
ソースコード
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
import time
import json
import sys
import requests
from bs4 import BeautifulSoup
import pandas as pd
import csv
address= "***"
password="***"
df1 = pd.read_csv('record.csv')
driver = webdriver.Chrome(executable_path=’***’)
driver.get('https://main.hirogete.com/#!/login/#page-top')
time.sleep(1)
driver.find_element_by_name("email").send_keys(address)
driver.find_element_by_name("password").send_keys(password)
driver.find_element_by_class_name("btn-primary").click()
time.sleep(5)
driver.find_element_by_xpath("//*[@id='modal-notice-content-127']/div[1]/div[2]").click()
time.sleep(1)
driver.find_element_by_xpath("//*[@id='modal-notice-content-128']/div[1]/div[2]").click()
html = driver.page_source.encode('utf-8')
soup = BeautifulSoup(html, "lxml")
POSTS1 = "tr-odd"
POSTS2 = "tr-even"
posts1 = soup.find_all(class_=POSTS1)
posts2 = soup.find_all(class_=POSTS2)
Marking_number= []
Depth = []
Width = []
Height = []
Postage = []
Insurance = []
Record_number = []
for post in posts1:
try:
marking1 = post.find('input',{'name': 'marking_no'})['value']
Depth1 = post.find('input', {'name':'bundle_depth'})['value']
Width1 = post.find('input', {'name':'bundle_width'})['value']
Height1 = post.find('input', {'name':'bundle_height'})['value']
Postage1 = post.findAll('td')[20].string
Insurance1 = post.findAll('td')[21].string
except Exception as e:
marking1 = ""
Depth1 = ""
Width1 = ""
Height1 = ""
Postage1 = ""
Insurance1 = ""
Marking_number.append(marking1)
Depth.append(Depth1)
Width.append(Width1)
Height.append(Height1)
Postage.append(Postage1)
Insurance.append(Insurance1)
for post2 in posts2:
try:
marking2 = post2.find('input',{'name': 'marking_no'})['value']
Depth2 = post2.find('input', {'name':'bundle_depth'})['value']
Width2 = post2.find('input', {'name':'bundle_width'})['value']
Height2 = post2.find('input', {'name':'bundle_height'})['value']
Postage2 = post2.findAll('td')[20].string
Insurance2 = post2.findAll('td')[21].string
except Exception as e:
marking2 = ""
Depth2 = ""
Width2 = ""
Height2 = ""
Postage2 = ""
Insurance2 = ""
Marking_number.append(marking2)
Depth.append(Depth2)
Width.append(Width2)
Height.append(Height2)
Postage.append(Postage2)
Insurance.append(Insurance2)
for i, rows in df1.iterrows():
record = rows["Sales Record Number"]
Record_number.append(record)
rows = zip(Marking_number,Depth,Width,Height,Postage,Insurance)
rows2 = Record_number
df1 = pd.DataFrame(rows2, columns=["Sales Record Number"]).astype(str)
df2 = pd.DataFrame(rows, columns=["Marking_number","Depth","Width","Height","Postage","Insurance"]).astype(str)
ret = df1.merge(df2, left_on='Sales Record Number', right_on='Marking_number', how='left')
ret.to_csv("postage.csv",encoding='shift_jis')
print("DONE")
もし、コードの指摘や質問がありましたら、Twitterにてご連絡ください。
この記事が気に入ったらサポートをしてみませんか?