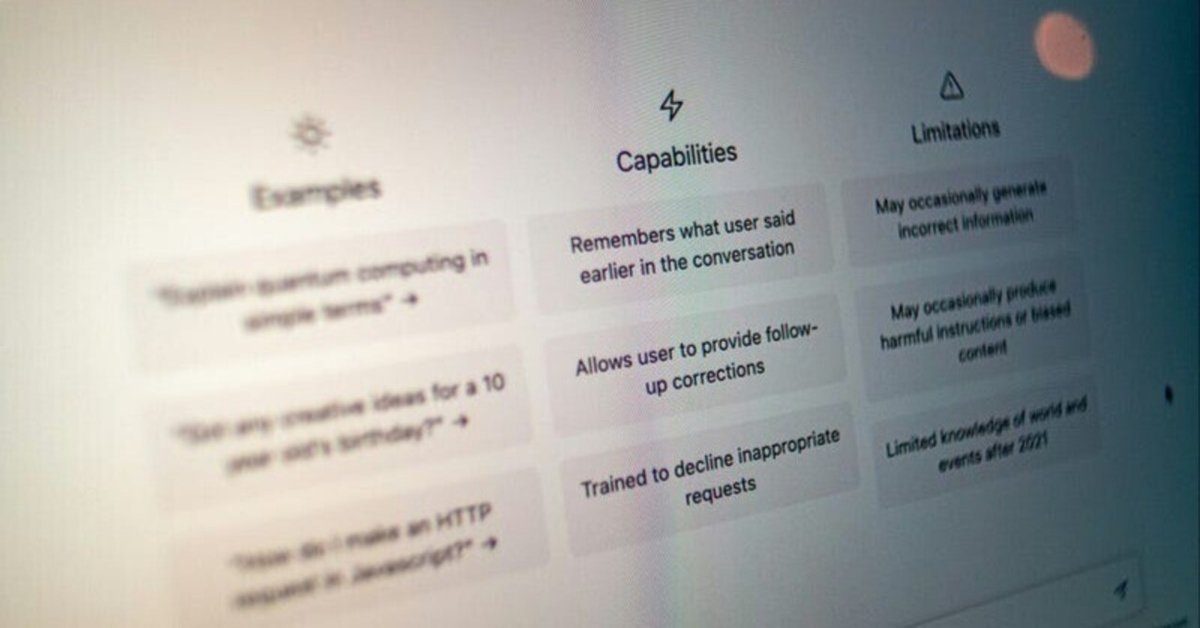
UnityとChatGPT統合デモ:OpenAI APIの使用方法
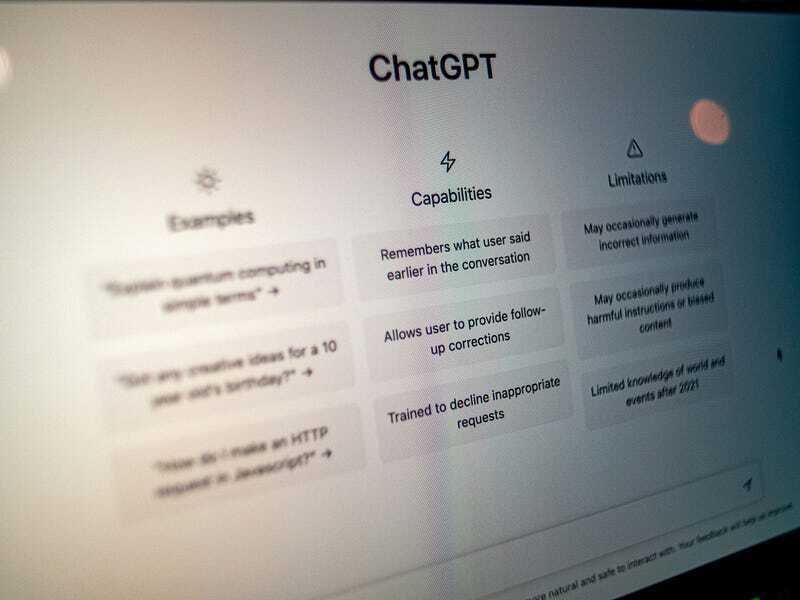
この記事では、OpenAI APIを使用してUnity環境内でChatGPTモデルを活用する方法を説明します。
プロジェクトレポジトリはこちらへ
他の言語のチュートリアル
🔐 OpenAI APIキーの取得
OpenAIアカウントを作成し、APIキーを取得します。OpenAI APIドキュメントからキーを発行できます。
1.OpenAIにログイン
2.カードの登録が必須であるため、Billingタブに移動してカードを登録します。
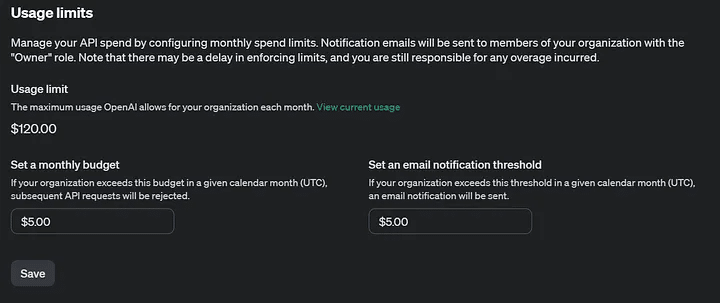
3.View API Keysに移動し、新しいシークレットキーを作成します。
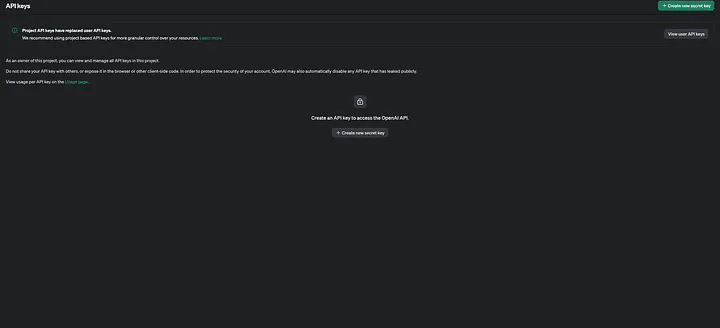
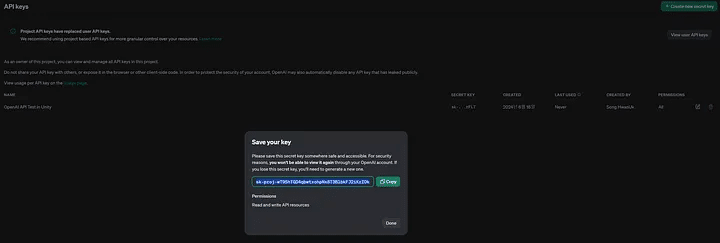
4.キーが正しく生成されたことを確認します。
保存されたキーを確認します。
サポートされているモデルを確認します(このドキュメントでは「gpt-3.5-turbo」モデルを使用しています)。
Windows
cURLのインストール確認
curl --version

cURLコマンドの作成
curl https://api.openai.com/v1/chat/completions ^
-H "Content-Type: application/json" ^
-H "Authorization: Bearer YOUR_API_KEY" ^
-d "{\"model\": \"gpt-3.5-turbo\", \"messages\": [{\"role\": \"user\", \"content\": \"Say this is a test\"}], \"max_tokens\": 5}"
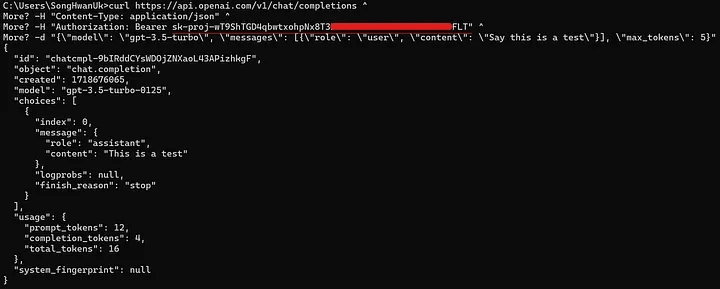
MacOS
curl https://api.openai.com/v1/chat/completions \
-H "Content-Type: application/json" \
-H "Authorization: Bearer YOUR_API_KEY" \
-d '{
"model": "gpt-3.5-turbo",
"messages": [
{
"role": "user",
"content": "Say this is a test"
}
],
"max_tokens": 5
}'
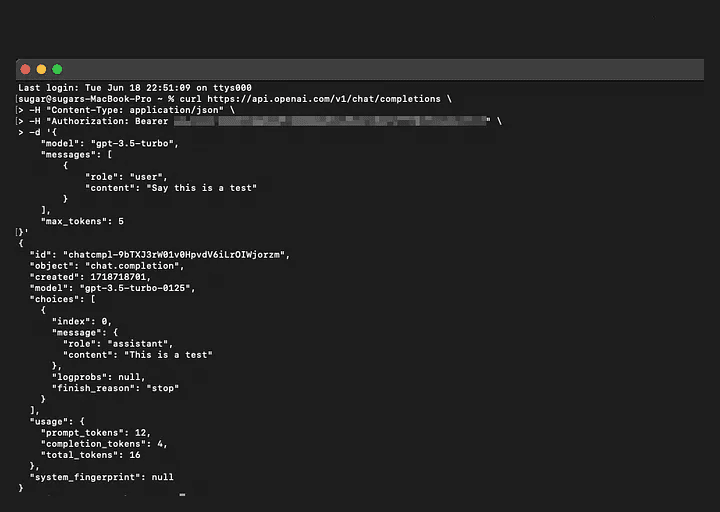
⚙️ パッケージのインストールと通信の準備
パッケージマネージャーを使用して、URLからNuGetForUnityをインストールします。次に、NuGet PackagesタブでNewtonsoft.Jsonを検索してインストールします。
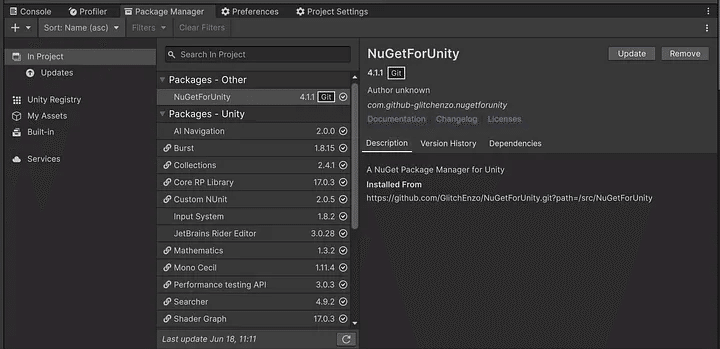
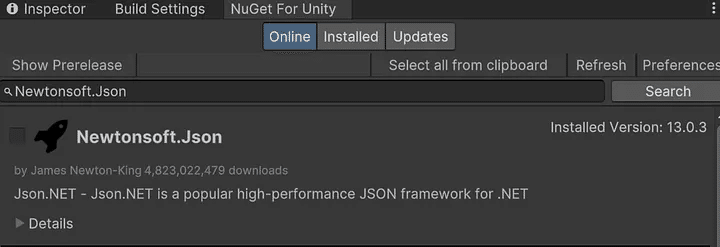
📜 スクリプト作成
using System.Collections;
using UnityEngine;
using UnityEngine.Networking;
using Newtonsoft.Json; // Use Newtonsoft.Json
public class OpenAIChatGPT : MonoBehaviour
{
private string apiKey = "YOUR_API_KEY"; // Replace with an actual API key
private string apiUrl = "https://api.openai.com/v1/chat/completions";
public IEnumerator GetChatGPTResponse(string prompt, System.Action<string> callback)
{
// Setting OpenAI API Request Data
var jsonData = new
{
model = "gpt-3.5-turbo",
messages = new[]
{
new { role = "user", content = prompt }
},
max_tokens = 20
};
string jsonString = JsonConvert.SerializeObject(jsonData);
// HTTP request settings
UnityWebRequest request = new UnityWebRequest(apiUrl, "POST");
byte[] bodyRaw = System.Text.Encoding.UTF8.GetBytes(jsonString);
request.uploadHandler = new UploadHandlerRaw(bodyRaw);
request.downloadHandler = new DownloadHandlerBuffer();
request.SetRequestHeader("Content-Type", "application/json");
request.SetRequestHeader("Authorization", "Bearer " + apiKey);
yield return request.SendWebRequest();
if (request.result == UnityWebRequest.Result.ConnectionError || request.result == UnityWebRequest.Result.ProtocolError)
{
Debug.LogError("Error: " + request.error);
}
else
{
var responseText = request.downloadHandler.text;
Debug.Log("Response: " + responseText);
// Parse the JSON response to extract the required parts
var response = JsonConvert.DeserializeObject<OpenAIResponse>(responseText);
callback(response.choices[0].message.content.Trim());
}
}
public class OpenAIResponse
{
public Choice[] choices { get; set; }
}
public class Choice
{
public Message message { get; set; }
}
public class Message
{
public string role { get; set; }
public string content { get; set; }
}
}
using UnityEngine;
public class ChatGPTExample : MonoBehaviour
{
[SerializeField, TextArea(3, 5)] private string prompt;
void Start()
{
OpenAIChatGPT chatGPT = gameObject.AddComponent<OpenAIChatGPT>();
StartCoroutine(chatGPT.GetChatGPTResponse(prompt, OnResponseReceived));
}
void OnResponseReceived(string response)
{
Debug.Log("ChatGPT Response: " + response);
}
}
📗 使用例
プロンプト質問 : In the extended virtual world, what is the newly coined word that combines “meta,” which means virtual and transcendent, and “universe,” which means world and universe?(仮想と超越を意味する「meta」と、世界と宇宙を意味する「universe」を組み合わせた新しい造語は何ですか?)
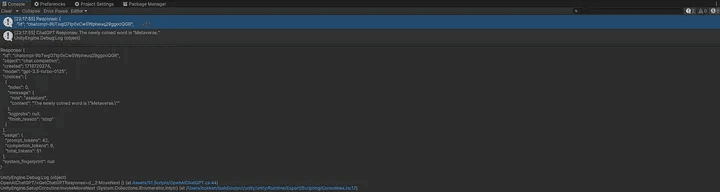

🤔 期待される機能
教育用クイズゲーム
ユーザーがカスタマイズ可能なストーリー生成器
ARベースの教育プラットフォーム
学習テーマを視覚化するためにAR環境で3Dモデルを表示
ChatGPTを使用してリアルタイムのQ&Aや説明を提供
ARベースのツアーガイド
観光スポットの情報をARで視覚的に提供
ChatGPTを使用して観光スポットに関する追加情報を提供し、質問に答える
ARベースのショッピングヘルパー
ARで製品情報を視覚的に提供
ChatGPTを使用して製品説明を提供し、質問に答える