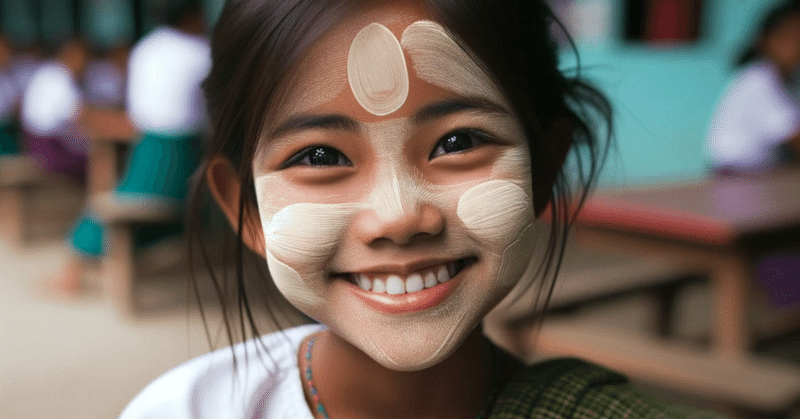
Photo by
tetsuya99
(備忘録) GPT-4-Turbo with JSON Mode
import openai
import json
# openai.api_key = YOURAPIKEY
# プロンプトとして使用するメッセージ
prompt1 = "Please generate a JSON object with the following properties: name, major, school, grades, and club."
# 学生の詳細情報
student_1_description = "David Nguyen is a sophomore majoring in computer science at Stanford University. He is Asian American and has a 3.8 GPA. David is known for his programming skills and is an active member of the university's Robotics Club. He hopes to pursue a career in artificial intelligence after graduating."
# GPT-4のプレビューモデル(GPT-4-Turbo)を使用してレスポンスを生成
openai_response = openai.chat.completions.create(
model='gpt-4-1106-preview',
response_format={ "type": "json_object" },
messages=[
{'role': 'user', 'content': prompt1},
{'role': 'assistant', 'content': student_1_description}
]
)
# JSON文字列を取得
json_string = openai_response.choices[0].message.content
# JSON文字列をPythonオブジェクトに変換
json_data = json.loads(json_string)
# Pythonオブジェクトとして取得できる
print(json_data)
以下の方法でも可能:
import openai
import json
# openai.api_key = YOURAPIKEY
# 学生の詳細情報
student_1_description = "David Nguyen is a sophomore majoring in computer science at Stanford University. He is Asian American and has a 3.8 GPA. David is known for his programming skills and is an active member of the university's Robotics Club. He hopes to pursue a career in artificial intelligence after graduating."
# プロンプトとして使用するメッセージ
prompt1 = f'''
Please extract the following information from the given text and return it as a JSON object:
name
major
school
grades
club
This is the body of text to extract the information from:
{student_1_description}
'''
# GPT-4のプレビューモデルを使用してレスポンスを生成
openai_response = openai.chat.completions.create(
model='gpt-4-1106-preview',
response_format={ "type": "json_object" },
messages=[
{'role': 'user', 'content': prompt1}
]
)
# JSON文字列を取得
json_string = openai_response.choices[0].message.content
# JSON文字列をPythonオブジェクトに変換
json_data = json.loads(json_string)
# Pythonオブジェクトとして取得できる
print(json_data)
おまけ:
Parallel Function Calling の例:
import openai
import json
# openai.api_key = YOURAPIKEY
funcs = [
{
"type": "function",
"function": {
"name": "buy_item",
"description": "Buy an item",
"parameters": {
"type": "object",
"properties": {
"item": {
"type": "string",
"description": "Name of the item",
},
"quantity": {
"type": "number",
"description": "Quantity of the item to purchase",
}
},
"required": ["item", "quantity"],
},
}
}
]
message = "Please buy 1 carton of milk and 6 eggs."
client = openai.OpenAI()
response = client.chat.completions.create(
messages=[
{
"role": "user",
"content": message
}
],
model="gpt-4-1106-preview",
tools=funcs
)
tool_calls = response.choices[0].message.tool_calls
for call in tool_calls:
args = json.loads(call.function.arguments)
print(args["item"], args["quantity"])
よろしければサポートお願いします! いただいたサポートはクリエイターとしての活動費に使わせていただきます!