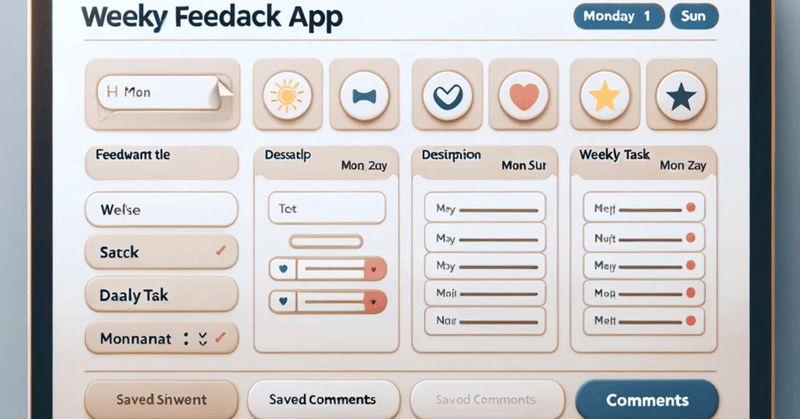
Flaskで週間フィードバックアプリを作成してみた
すごく簡単なアプリを作ってみた。(本当にごく簡単なw)
Flaskで週間フィードバックアプリを作成しよう!
このガイドでは、Flaskを使って週間フィードバックアプリを作成する方法をステップバイステップで説明します。このアプリは、毎日ランダムなフィードバックを表示し、コメントを入力して保存する機能を持っています。
必要なツール
Python(バージョン3.6以上)
Flask
HTML、CSS、JavaScriptの基本的な知識
1. プロジェクトのセットアップ
ディレクトリ構造
まず、プロジェクトのディレクトリ構造を以下のように設定します。
your_project_directory/
├── app.py
├── templates/
│ └── index.html
└── static/
├── script.js
└── styles.css
必要なパッケージをインストール
Flaskをインストールします。
pip install Flask
2. Flaskアプリケーションの作成
`app.py`の作成
以下の内容で`app.py`を作成します。
from flask import Flask, render_template, request, jsonify, redirect
from datetime import datetime
app = Flask(__name__)
feedback_data = [
{
'title': '1. 自分の強みや興味を見極める',
'description': '自分の強みや興味を見極め、それを活かす方法を考える。',
'feedback': '自己分析を行い、自分の強みや興味をリストアップする。',
'daily': '自己分析の時間を取り、自分の強みや興味をリストアップする。',
'weekly': '自己分析の結果を元に、強みや興味を活かすための具体的な行動計画を立てる。'
},
# ... 他のフィードバックデータ ...
]
comments = []
@app.route('/')
def home():
return render_template('index.html')
@app.route('/feedback')
def get_feedback():
return jsonify(feedback_data)
@app.route('/submit', methods=['POST'])
def submit_comment():
comment = request.form.get('user-comment')
title = request.form.get('feedback-title')
if comment and title:
current_date = datetime.now().strftime('%Y-%m-%d')
comments.append({'date': current_date, 'comment': comment, 'title': title})
return redirect('/')
@app.route('/comments')
def get_comments():
return jsonify(comments)
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0', port=5001)
3. テンプレートの作成
`templates/index.html`の作成
以下の内容で`index.html`を作成します。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>週間フィードバックアプリ</title>
<link rel="stylesheet" type="text/css" href="{{ url_for('static', filename='styles.css') }}">
</head>
<body>
<h1>週間フィードバックアプリ</h1>
<div id="choices">
<button onclick="showRandomFeedback('月曜日')">月曜日</button>
<button onclick="showRandomFeedback('火曜日')">火曜日</button>
<button onclick="showRandomFeedback('水曜日')">水曜日</button>
<button onclick="showRandomFeedback('木曜日')">木曜日</button>
<button onclick="showRandomFeedback('金曜日')">金曜日</button>
<button onclick="showRandomFeedback('土曜日')">土曜日</button>
<button onclick="showRandomFeedback('日曜日')">日曜日</button>
</div>
<div id="result"></div>
<form action="/submit" method="POST" id="feedback-form">
<textarea id="user-comment" name="user-comment" placeholder="コメントを入力"></textarea><br>
<input type="hidden" id="feedback-title" name="feedback-title">
<button type="submit">送信</button>
</form>
<h2>コメント一覧:</h2>
<ul id="feedback-entries">
</ul>
<script src="{{ url_for('static', filename='script.js') }}"></script>
</body>
</html>
4. スタティックファイルの作成
`static/script.js`の作成
以下の内容で`script.js`を作成します。
const feedbackData = [
{
title: '1. 自分の強みや興味を見極める',
description: '自分の強みや興味を見極め、それを活かす方法を考える。',
feedback: '自己分析を行い、自分の強みや興味をリストアップする。',
daily: '自己分析の時間を取り、自分の強みや興味をリストアップする。',
weekly: '自己分析の結果を元に、強みや興味を活かすための具体的な行動計画を立てる。'
},
// ... 他のフィードバックデータ ...
];
function showRandomFeedback(day) {
const randomIndex = Math.floor(Math.random() * feedbackData.length);
const feedback = feedbackData[randomIndex];
const resultDiv = document.getElementById('result');
const currentDate = new Date().toLocaleDateString('ja-JP');
resultDiv.innerHTML = `<h2>${day} (${currentDate})</h2>
<h3 id="feedback-title-display">${feedback.title}</h3>
<p>${feedback.description}</p>
<h3>フィードバック:</h3>
<p>${feedback.feedback}</p>
<h3>今日やること:</h3>
<p>${feedback.daily}</p>
<h3>一週間以内にやること:</h3>
<p>${feedback.weekly}</p>`;
document.getElementById('feedback-title').value = feedback.title;
}
window.onload = function() {
fetch('/comments')
.then(response => response.json())
.then(comments => {
const feedbackEntries = document.getElementById('feedback-entries');
feedbackEntries.innerHTML = '';
comments.forEach(entry => {
const li = document.createElement('li');
li.textContent = `${entry.date} - ${entry.title}: ${entry.comment}`;
feedbackEntries.appendChild(li);
});
});
}
`static/styles.css`の作成
以下の内容で`styles.css`を作成します。
body {
font-family: Arial, sans-serif;
text-align: center;
padding-top: 50px;
}
#choices button {
margin: 10px;
font-size: 20px;
padding: 10px 20px;
}
#result {
margin-top: 20px;
font-size: 24px;
}
textarea {
width: 80%;
height: 100px;
margin-bottom: 10px;
}
5. アプリケーションの起動
プロジェクトディレクトリで以下のコマンドを実行してFlaskサーバーを起動します。
python app.py
ブラウザで`http://127.0.0.1:5001`を開き、アプリケーションが動作していることを確認します。
6. 使い方
各曜日ボタンをクリックすると、その曜日に対応したフィードバックがランダムに表示されます。
フィードバックに対してコメントを入力し、送信ボタンをクリックすると、コメントが保存されます。
保存されたコメントはコメント一覧に日付とともに表示されます。
これで、週間フィードバックアプリの完成です。FlaskとシンプルなHTML、CSS、JavaScriptを組み合わせることで、動的なWebアプリケーションを簡単に構築できます。このアプリケーションをベースに、さらに機能を追加してカスタマイズしてみてください。
データは以下にようなものを使っています。
{
'title': '1. 自分の強みや興味を見極める',
'description': '自分の強みや興味を見極め、それを活かす方法を考える。',
'feedback': '自己分析を行い、自分の強みや興味をリストアップする。',
'daily': '自己分析の時間を取り、自分の強みや興味をリストアップする。',
'weekly': '自己分析の結果を元に、強みや興味を活かすための具体的な行動計画を立てる。'
},
{
'title': '2. 目標設定の明確化',
'description': '具体的かつ達成可能な目標を設定する。',
'feedback': 'SMARTゴールのフレームワークを使って目標を設定する。',
'daily': 'SMARTゴールのフレームワークを使って、短期および長期の目標を設定する。',
'weekly': '設定した目標に向けての進捗を確認し、必要に応じて目標を見直す。'
},
{
'title': '3. 新しいことに挑戦する',
'description': '新しいことに挑戦し、失敗を恐れずに経験を積む。',
'feedback': '新しいタスクやプロジェクトに取り組む際の具体的な計画を立てる。',
'daily': '新しいプロジェクトを一つ選び、それに取り組む計画を立てる。',
'weekly': '挑戦した結果を評価し、次の改善点を見つける。'
},
ただこれを日々の保存として使うにまだまだですね。
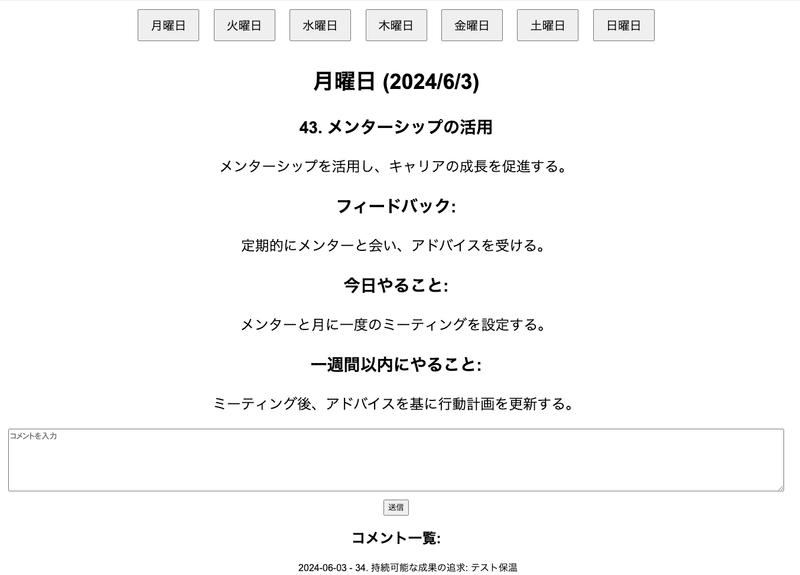
おもしろきこともなき世を面白く 議論メシ4期生http://gironmeshi.net/ メンタリストDaiGo弟子 強みほがらかさと発散思考 外資系企業でインフラエンジニア