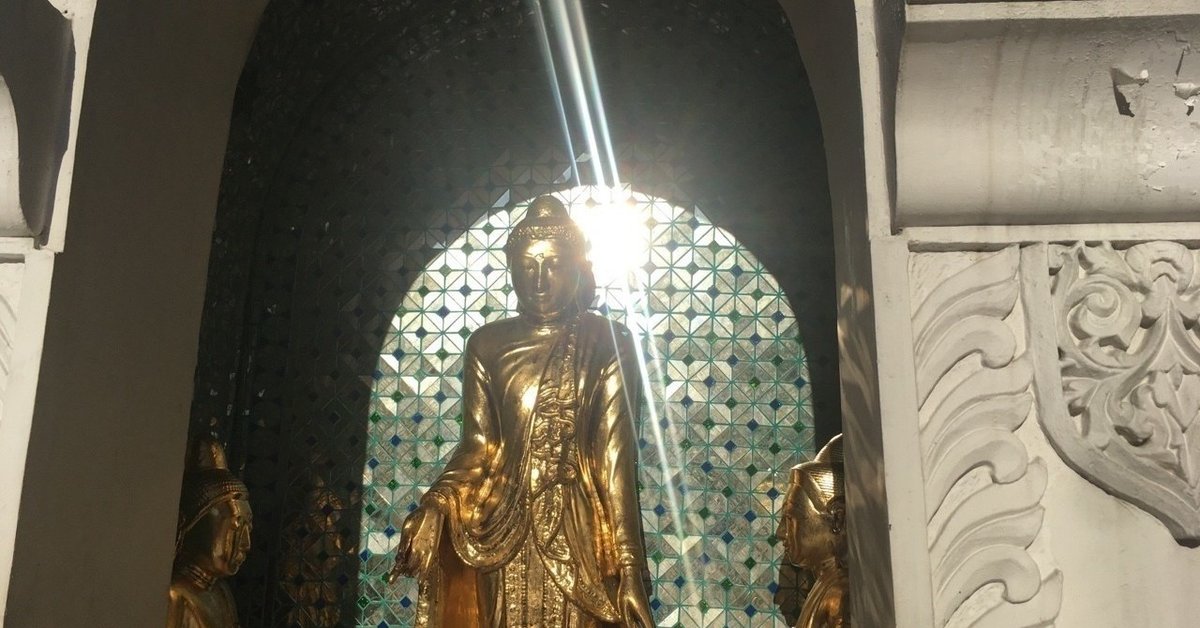
Photo by
5dmsoup
python:flask,Jinja2を使って、HTMLへ関数を組み込む
JInja2を使って、HTMLへ関数を入れ込む
文字入力
message = "Jinja2のテスト"をhtmlへ入れ込む
#ファイル名:app.py
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
message = "Jinja2のテスト"
return render_template('index.html', message=message)
app.run(host="localhost", debug=True)
#ファイル名:index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Jinja2のテスト</title>
</head>
<body>
<h1>Jinja2のテスト</h1>
<h1>{{ message }} </h1>
</body>
</html>
辞書からの入力
message = {"test": "Jinja2のテスト", "test2": "Jinja2のテスト2"}をhtmlへ入れ込む
#ファイル名:app.py
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
message = {"test": "Jinja2のテスト", "test2": "Jinja2のテスト2"}
return render_template('index.html', message=message)
app.run(host="localhost", debug=True)
#ファイル名:index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Jinja2のテスト</title>
</head>
<body>
<h1>Jinja2のテスト</h1>
<h1>{{ message.test }} </h1>
<h1>{{ message.test2 }} </h1>
</body>
</html>
リストの値をランダムに出力
{{ message | random }}
{{ ["Jinja2のテスト", "Jinja2のテスト2", "Jinja2のテスト3"] | random }}
#ファイル名:app.py
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
message = ["Jinja2のテスト", "Jinja2のテスト2", "Jinja2のテスト3"]
return render_template('index.html', message=message)
app.run(host="localhost", debug=True)
#ファイル名:index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Jinja2のテスト</title>
</head>
<body>
<h1>Jinja2のテスト</h1>
<h1>{{ message | random }} </h1>
<h1>{{ ["Jinja2のテスト", "Jinja2のテスト2", "Jinja2のテスト3"] | random }} </h1>
</body>
</html>
リストをインデックスで呼び出す
message = ["Jinja2のテスト", "Jinja2のテスト2", "Jinja2のテスト3"]
#ファイル名:app.py
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
message = ["Jinja2のテスト", "Jinja2のテスト2", "Jinja2のテスト3"]
return render_template('index.html', message=message)
app.run(host="localhost", debug=True)
#ファイル名:index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Jinja2のテスト</title>
</head>
<body>
<h1>Jinja2のテスト</h1>
<h1>{{ message[0] }} </h1>
<h1>{{ message[1] }} </h1>
<h1>{{ message[2] }} </h1>
</body>
</html>
if関数
#ファイル名:app.py
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
message = ["Jinja2のテスト", "Jinja2のテスト2", "Jinja2のテスト3"]
return render_template('index.html', message=message)
app.run(host="localhost", debug=True)
#ファイル名:index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Jinja2のテスト</title>
</head>
<body>
<h1>Jinja2のテスト</h1>
{% if message | random == "Jinja2のテスト" %}
<h2>合格</h2>
{% elif message | random == "Jinja2のテスト2" %}
<h2>不合格</h2>
{% else %}
<h2>論外</h2>
{% endif %}
</body>
</html>
for文
#ファイル名:app.py
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
message = ["Jinja2のテスト", "Jinja2のテスト2", "Jinja2のテスト3"]
return render_template('index.html', message=message)
app.run(host="localhost", debug=True)
#ファイル名:index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Jinja2のテスト</title>
</head>
<body>
<h1>Jinja2のテスト</h1>
{% for v in message %}
■{{ v }}<br>
{% endfor %}
</body>
</html>
フォームで受け取って、受け取った情報を表示させる
#ファイル名:app.py
from flask import Flask, render_template, url_for, request, redirect, session
app = Flask(__name__)
app.secret_key = 'hogehoge'
@app.route("/", methods=['POST','GET'])
def index():
return render_template('index.html')
@app.route("/todo", methods=['GET', 'POST'])
def todo():
if request.method == 'POST':
todo = request.form['todo']
if todo:#todoがない1度目はこれがないとエラーになる
todo_list = []
if 'todo' in session:#todoがない1度目はこれがないとエラーになる
todo_list = session['todo']
todo_list.append(todo)
session['todo'] = todo_list
return render_template('index.html')
#ファイル名:index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Jinja2のテスト</title>
</head>
<body>
<h1>Jinja2のテスト</h1>
<form method="POST" action={{ url_for('todo') }}>
<p>タスク内容:<input type="text" name="todo" size="40"></p>
<button type="submit">送信</button>
</form>
<h3>タスク一覧</h3>
<ul>
{% for v in session['todo'] %}
<li>{{ v }}</li>
{% endfor %}
</ul>
</body>
</html>
受け取った情報を全部消す
#ファイル名:app.py
from flask import Flask, render_template, url_for, request, redirect, session
app = Flask(__name__)
app.secret_key = 'hogehoge'
@app.route("/", methods=['POST','GET'])
def index():
return render_template('index.html')
@app.route("/todo", methods=['GET', 'POST'])
def todo():
if request.method == 'POST':
todo = request.form['todo']
if todo:#todoがない1度目はこれがないとエラーになる
todo_list = []
if 'todo' in session:#todoがない1度目はこれがないとエラーになる
todo_list = session['todo']
todo_list.append(todo)
session['todo'] = todo_list
return render_template('index.html')
@app.route("/session_clear",methods=['GET', 'POST'])
def session_clear():
session.pop('todo')
return redirect(url_for('todo'))
app.run(host="localhost", debug=True)
#ファイル名:index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Jinja2のテスト</title>
</head>
<body>
<h1>Jinja2のテスト</h1>
<form method="POST" action={{ url_for('todo') }}>
<p>タスク内容:<input type="text" name="todo" size="40"></p>
<button type="submit">送信</button>
</form>
<h3>タスク一覧</h3>
<ul>
{% for v in session['todo'] %}
<li>{{ v }}</li>
{% endfor %}
</ul>
<a href="{{ url_for('session_clear')}}">全部消す</a>
</body>
</html>
過去記事
pythonに関することは過去にいくつか書いていますのでよろしければそちらもご参照ください。
この記事が気に入ったらサポートをしてみませんか?