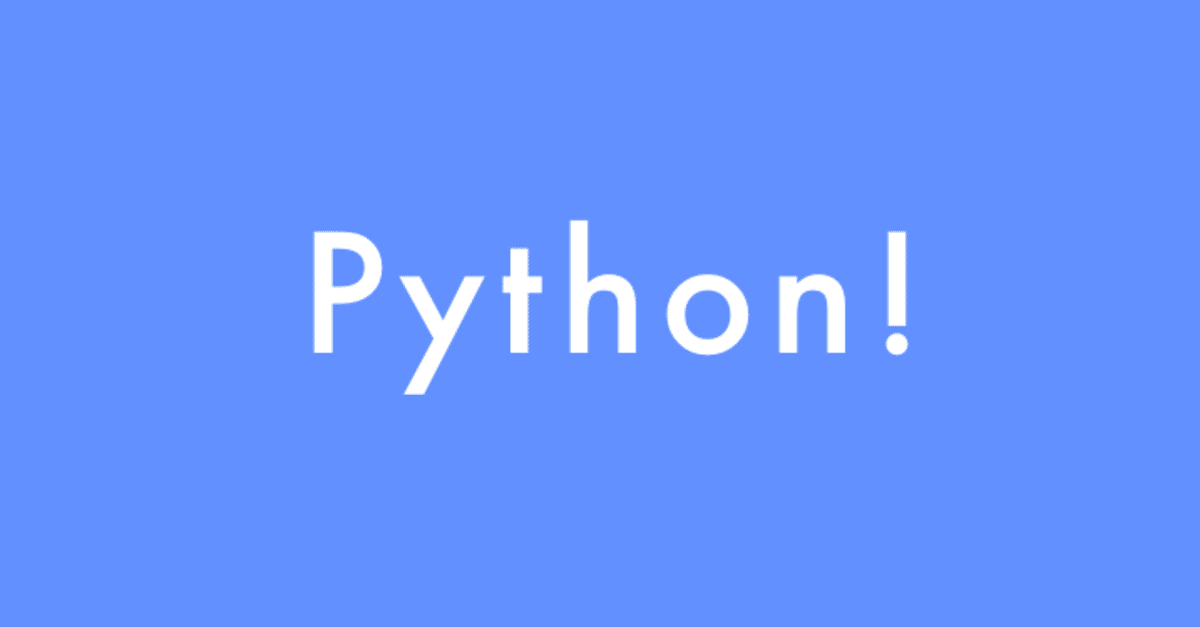
Photo by
dngri
Python 文法勉強誌(タプル)
この記事は、「challenge-every-month全員でアウトプット芸人 Advent Calendar」と「後回し改善ウィーク」の6日目の記事になります。
Pythonの PyQ でやっているが、タプルについて今日学んだ。
タプルとは、リストに似たシーケンス型のオブジェクト。要素の参照はリストと同様にインデックスを指定する。
sample_tuple = (1 , 'A', 'sample')
print(sample_tuple[0]) # 1と表示される
print(sample_tuple[1]) # Aと表示される
print(sample_tuple[2]) # sampleと表示される
注意点としてリストと違い、1度生成されたタプルは要素の変更・追加・削除ができない。リストにあった「append(),insert(),pop()」のような関数はない。このタプルの性質のことを「イミュータブル」という。
sample_tuple = (1 , 'A', 'sample')
sample_tuple[0] = 100
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: 'tuple' object does not support item assignment
要素は変更できないが、変数に入れているタプルの値を再代入したりすることは可能である。
sample_tuple = (1 , 'A', 'sample')
sample_tuple = (100,)
>>> print(sample_tuple[0])
100
>>> print(sample_tuple[1])
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
IndexError: tuple index out of range
また、タプルの要素が1つの場合、要素の最後にはカンマを書かないとタプルと認識されず、値として認識される。
sample_tuple = ('tada',)
print(one_tuple) # ('tada',)と表示される
value = ('tada')
print(value) # tadaと表示される
繰り返しでの使い方
タプルは辞書型のキーとしても使える。
sample_tuple = {(1, 2): 'A'}
print(sample_tuple[(1, 2)])
辞書型のキーとして使えるのであれば、繰り返し文でも使うことができる。
sample_tuple = {(1989,9): 'tada', (1990,10): 'tarou', (1991, 11): 'jirou'}
for birthday, name in sample_tuple.items():
year = str(birthday[0])
month = str(birthday[1])
print(name + "が生まれたのは" +year + "年" + month + "月" + "です。")
# 出力結果
tadaが生まれたのは1989年9月です。
tarouが生まれたのは1990年10月です。
jirouが生まれたのは1991年11月です。
参考記事
タプルとリストの違いについて詳細にまとめてくださっている記事があり勉強させてもらったのでこちらもリンクを貼っておく。
余談
Python の言語思想についてのページをざっとみてみると、どういう考えを持った言語なのかを知れて面白い。Python のインタプリタモードで「import this」と打ってみると以下の 「The Zen of Python」をみることができる。
>>> import this
The Zen of Python, by Tim Peters
Beautiful is better than ugly. # 美しさは醜いものよりも優れている
Explicit is better than implicit. # 明示的は暗黙的より優れている
Simple is better than complex. # シンプルは複雑より優れている
Complex is better than complicated. # 複雑は煩わしいことより優れている
Flat is better than nested. # フラットは入れ子より優れている
Sparse is better than dense. # 疎は蜜よりも優れている
Readability counts. # 読みやすさが重要である
Special cases aren't special enough to break the rules. # 特別な場合は、ルールを破るほど特別なわけではない
Although practicality beats purity. # 実用性は純度を上回るものの
Errors should never pass silently. # エラーは黙って渡されるべきではない
Unless explicitly silenced. # 明示的に沈黙してない限り
In the face of ambiguity, refuse the temptation to guess. # 曖昧さに直面して、推測する誘惑を拒否する
There should be one-- and preferably only one --obvious way to do it. # それをするための一つの、そして好ましくは一つだけの明白な方法があるべき
Although that way may not be obvious at first unless you're Dutch. # お連打人でない限り、そのやり方が最初は明白ではいかもしれない
Now is better than never. # 今ではないよりマシ
Although never is often better than *right* now. # 今は正しいよりも多く場合決していいことではない
If the implementation is hard to explain, it's a bad idea. # 実装を説明するのが難しい場合、それは悪い考えだ
If the implementation is easy to explain, it may be a good idea. # 実装がしやすいのであればいい考えだ
Namespaces are one honking great idea -- let's do more of those! # 名前空間は素晴らしいアイデアの1つ
この記事が気に入ったらサポートをしてみませんか?