みなとみらいでAR映えしてきた話
IwakenLab主催でのARイベントに参加してきました!
今回はunityではなく8th wall でVPSを用いてAR表現してきました!
(VPSとはVisual Positioning Systemです)
今回のテーマがみなとみらいでAR映えした作品を作ろうということで
コンセプト:
横浜×クリスマス×オルゴールで、穏やかなARを楽しもう!
というテーマで作成しました。
では、さっそく作った作品を見ていただきます。
最初は動画です
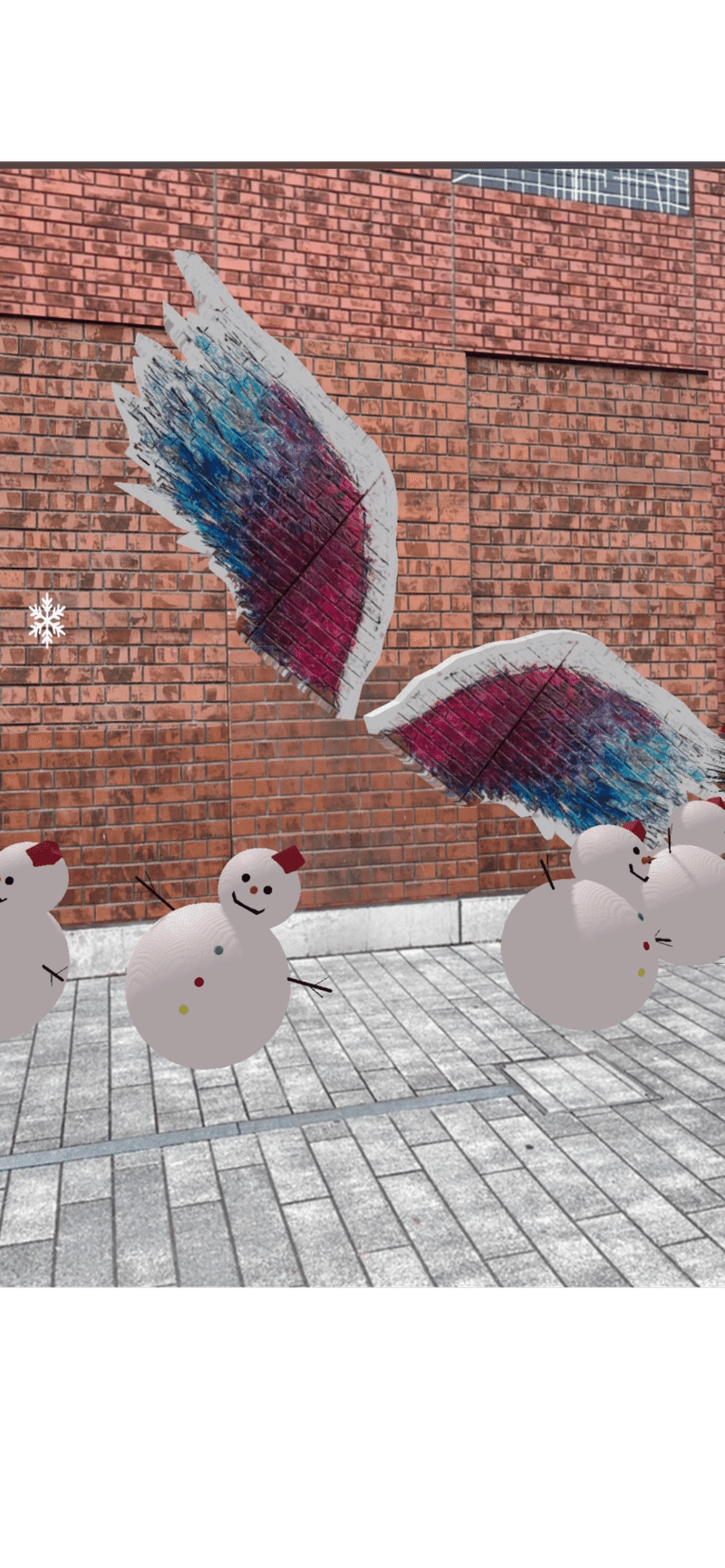
こだわりポイント:
雪だるまがとても可愛い
羽について、平和など作者の強いメッセージがあったので変に加工せずそのまま3Dに持っていき、動かしました!
初めて触ったので、コードの書き方もあまりわからなかったですが以下のように書きました!
//snow.js
const particleTexture = new THREE.TextureLoader().load('./assets/rdesign_14089e-ysunmnc96j.png') // 雪のテクスチャをロード
const particlesnow = {
schema: {
preset: {default: 'snow', oneOf: ['snow']},
particleCount: {type: 'int', default: 100},
size: {type: 'number', default: 0.5},
velocityValue: {type: 'vec3', default: {x: 0, y: 0.5, z: 1}},
velocitySpread: {type: 'vec3', default: {x: 0.5, y: 0.5, z: 0.5}},
},
init() {
// パーティクルシステムの初期化
const particleMaterial = new THREE.PointsMaterial({
size: this.data.size,
map: particleTexture,
transparent: true, // 透明部分を扱うために必要
blending: THREE.AdditiveBlending, // ブレンディングを設定
})
// 三.jsのパーティクルシステムを作成
const particleSystem = new THREE.Points(
new THREE.BufferGeometry(),
particleMaterial
)
// パーティクルの位置設定
const positions = new Float32Array(this.data.particleCount * 3)
for (let i = 0; i < this.data.particleCount; i++) {
positions[i * 3] = Math.random() * 6 - 2 // X座標
positions[i * 3 + 1] = Math.random() * 4 // Y座標(高さ)
positions[i * 3 + 2] = Math.random() * 6 - 2 // Z座標
}
particleSystem.geometry.setAttribute(
'position',
new THREE.Float32BufferAttribute(positions, 3)
)
this.particleSystem = particleSystem
this.el.setObject3D('particle-system', this.particleSystem)
},
tick() {
// パーティクルの動きを更新
const positions = this.particleSystem.geometry.attributes.position.array
const velocity = 1
for (let i = 0; i < positions.length; i += 3) {
positions[i + 1] -= velocity * 0.01 // Y座標を減少させる(下降)
if (positions[i + 1] < 0) {
// 画面下に到達した場合、上部から再スタート
positions[i + 1] = 4
}
}
this.particleSystem.geometry.attributes.position.needsUpdate = true
},
}
export {particlesnow}
//sound.js
const scenesound = {
init() {
const {sceneEl} = this.el
const soundEl = document.querySelector('#mySound')
// ユーザーインタラクションを要求するイベントリスナー
window.addEventListener('click', function playSound() {
soundEl.play().then(() => {
console.log('Sound played successfully')
}).catch((error) => {
console.error('Sound play failed:', error)
})
// イベントリスナーを削除
window.removeEventListener('click', playSound)
})
},
}
export {scenesound}
次はocclusionにも注意して実装していきたいです!
この記事が気に入ったらサポートをしてみませんか?