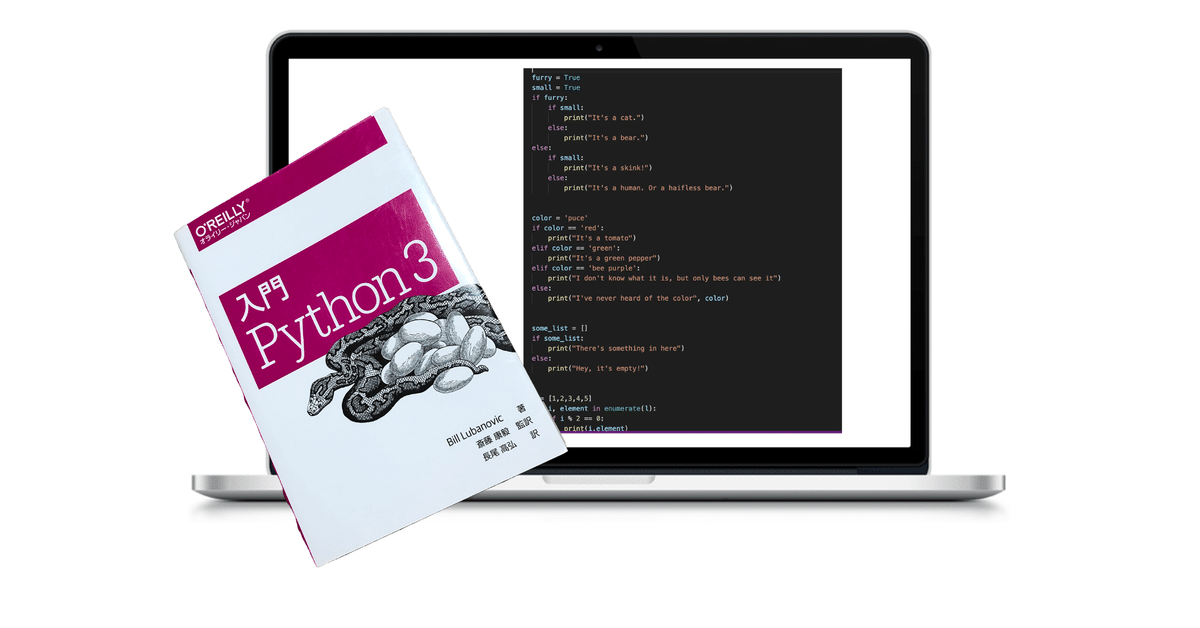
Python3 自習・復習vol.010
for による反復処理
Python は、イテレータ(イテレーションごとにリスト、辞書などから要素をひとつずつ取り出して返すもの)を頻繁に使う。そうすれば、データ構造のサイズやどのように実装されているのかを知らなくても、データ構造の角要素を操作できるから。その場で作ったデータも for で受け付けられるので、コンピュータのメモリに全部収めきれないようなデータストリームも処理可能。
>>> rabbits = ['Flopsy', 'Mopsy', 'Cottontail', 'Peter']
>>> current = 0
>>> while current < len(rabbits):
... print(rabbits[current])
... current += 1
...
Flopsy
Mopsy
Cottontail
Peter
>>>
上記も間違ってはいないが、次のようにした方がPython らしいコードになるそうです。
>>> for rabbit in rabbits:
... print(rabbit)
...
Flopsy
Mopsy
Cottontail
Peter
>>>
rabbits のようなリストは、文字列、タプル、辞書、集合、その他とともに、Python のイテラブル(イテレーターに対応している)オブジェクト。タプルやリストを for で処理すると、一度に一つずつ要素が取り出される。文字列を for で処理すると、次に示すように一度に1文字ずつが生成される。
>>> word = 'cat'
>>> for letter in word:
... print(letter)
...
c
a
t
>>>
辞書(または辞書の keys 関数)を for で処理すると、キーが返される。次の例では、キーはボードゲーム「Clue」(北米以外では「Cluedo」)のカード種類を表す。
>>> accusation = {'room': 'ballroom', 'weapon': 'lead pipe', 'person': 'Col.Mustard'}
>>> for card in accusation: # or for card in accusation.key():
... print(card)
...
room
weapon
person
>>>
キーではなく、値を反復処理したい場合には、辞書の values() 関数を使う。
>>> for value in accusation.values():
... print(value)
...
ballroom
lead pipe
Col.Mustard
>>>
キーと値の両方をタプルで返したい場合は、items() 関数を使う。
>>> for item in accusation.items():
... print(item)
...
('room', 'ballroom')
('weapon', 'lead pipe')
('person', 'Col.Mustard')
>>>
タプルの各要素を個別の変数に代入する作業は、ワンステップで可能なことを覚えておこう! items() が返すここのタプルについて、第一の値(key)を card 、第二の値(value)を contents に代入することができる。
>>> for card, contents in accusation.items():
... print('Cardd', card, 'has the contents', contents)
...
Cardd room has the contents ballroom
Cardd weapon has the contents lead pipe
Cardd person has the contents Col.Mustard
>>>
break による中止
for ループに break 文を入れると、while ループの時と同様に、そこでループを中止する。
continue による次のイテレーションの開始
for ループに continue を入れると、while ループの時と同様に、ループの次のイテレーションにジャンプする。
else による break のチェック
while と同様に、for は正常終了したかどうかをチェックするオプションのelse を持っている。break が呼び出されなければ、else 文が実行される。
この機能は、for ループが break 呼び出しで途中で終了しておらず、最後まで実行されたことを確かめたい時に役立つ。
次の例は、チーズショップにチーズがあれば、そのチーズの名前を表示してbreak する。
>>> cheeses = []
>>> for cheese in cheeses:
... print('This shop has some lovely', cheese)
... brak
... else: # breakしていないということは、チーズがないということ。
... print('This is not much of a cheese shop, is it?')
...
This is not much of a cheese shop, is it?
>>>
while の時と同様に、for ループの else は、何かを探すために for ループを使い、それが見つからなかた時に else が呼び出されると考える。
else を使わずに同様の効果を得るためには、次のようにする。
>>> cheeses = []
>>> found_one = False
>>> for cheese in cheeses:
... forund_one = True
... print('This shop has some lovely', cheese)
... break
...
>>> if not found_one:
... print('This is not much of a cheese shop, is it?')
...
This is not much of a cheese shop, is it?
>>>
最後までお読みいただき、本当にありがとうございます。 明日も継続して学習のアウトプットを続けていきたいと思いますので、また覗きにきていただけると嬉しいです! 一緒に学べる仲間が増えると、もっと喜びます!笑 これからも宜しくお願い致しますm(__)m