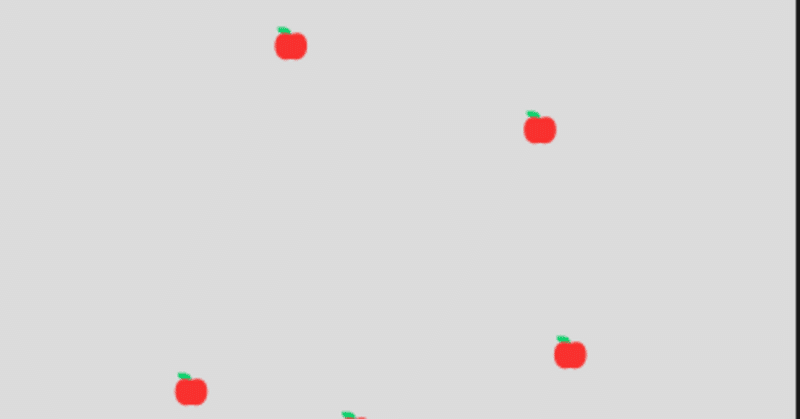
🍎リンゴが上から降ってきてそれを拾うと点数が入る簡単なゲームをp5.jsで作りたいのでベースソースを作ってください
p5.jsを使って、リンゴが降ってくるシンプルなゲームのベースを作成することができます。以下に、基本的なゲームのソースコードを示します。このコードでは、リンゴが上からランダムに降り、画面の下まで到達すると再び上から降り始めます。プレイヤーはマウスを使ってキャッチャーを動かし、リンゴをキャッチすることで点数を獲得します。
let apple;
let catcher;
let score;
function setup() {
createCanvas(400, 400);
apple = new Apple();
catcher = new Catcher();
score = 0;
}
function draw() {
background(220);
apple.display();
apple.update();
catcher.display();
catcher.move(mouseX);
if (apple.isCaught(catcher.x, catcher.width)) {
score += 1;
apple.reset();
}
fill(0);
textSize(16);
text(`Score: ${score}`, 10, 30);
}
class Apple {
constructor() {
this.reset();
}
display() {
fill(255, 0, 0); // red color
ellipse(this.x, this.y, this.size, this.size);
}
update() {
this.y += this.speed;
if (this.y > height) {
this.reset();
}
}
reset() {
this.x = random(width);
this.y = 0;
this.size = 20;
this.speed = random(2, 5);
}
isCaught(catcherX, catcherWidth) {
let distance = dist(this.x, this.y, catcherX + catcherWidth / 2, height - 20);
return distance < this.size / 2 + catcherWidth / 2;
}
}
class Catcher {
constructor() {
this.width = 50;
this.x = width / 2;
}
display() {
fill(0);
rect(this.x, height - 40, this.width, 20);
}
move(positionX) {
this.x = positionX - this.width / 2;
}
}
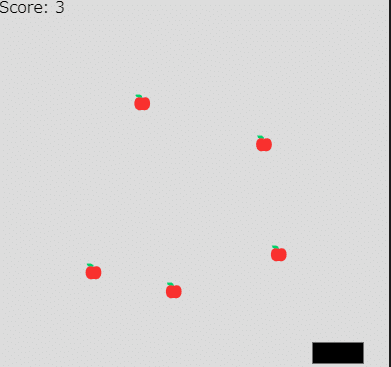
お願い致します