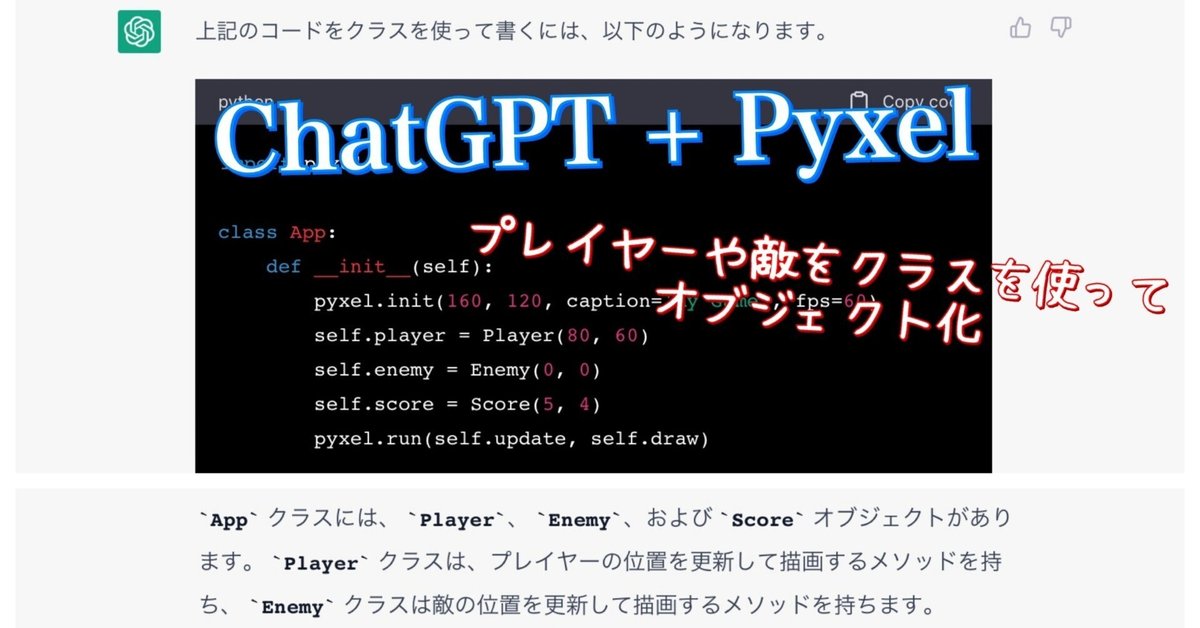
ChatGPTにPyxelのコード書いてもらった その2 クラスを使って
今話題のChat GPT に前回のコードを貼り付けて
「クラスを使って」と入力
もとのコード
import pyxel
class App:
def __init__(self):
pyxel.init(160, 120, caption='My Game', fps=60)
self.player_x = 80
self.player_y = 60
self.enemy_x = 0
self.enemy_y = 0
self.score = 0
pyxel.run(self.update, self.draw)
def update(self):
if pyxel.btn(pyxel.KEY_UP):
self.player_y = max(0, self.player_y - 2)
if pyxel.btn(pyxel.KEY_DOWN):
self.player_y = min(120, self.player_y + 2)
if pyxel.btn(pyxel.KEY_LEFT):
self.player_x = max(0, self.player_x - 2)
if pyxel.btn(pyxel.KEY_RIGHT):
self.player_x = min(160, self.player_x + 2)
self.enemy_x += 1
if self.enemy_x > 160:
self.enemy_x = 0
self.enemy_y = pyxel.random(120)
self.score += 1
if abs(self.enemy_x - self.player_x) < 8 and abs(self.enemy_y - self.player_y) < 8:
pyxel.quit()
def draw(self):
pyxel.cls(0)
pyxel.rect(self.player_x, self.player_y, 8, 8, 9)
pyxel.rect(self.enemy_x, self.enemy_y, 8, 8, 8)
pyxel.text(5, 4, 'SCORE: {}'.format(self.score), 7)
App()
クラスを使ったコード(5, 35, 39, 60行目修正)
import pyxel
class App:
def __init__(self):
pyxel.init(160, 120, title='My Game', fps=60) # caption --> title
self.player = Player(80, 60)
self.enemy = Enemy(0, 0)
self.score = Score(5, 4)
pyxel.run(self.update, self.draw)
def update(self):
self.player.update()
self.enemy.update()
if self.enemy.is_off_screen():
self.enemy.reset()
self.score.increase()
if self.player.collides_with(self.enemy):
pyxel.quit()
def draw(self):
pyxel.cls(0)
self.player.draw()
self.enemy.draw()
self.score.draw()
class Player:
def __init__(self, x, y):
self.x = x
self.y = y
def update(self):
if pyxel.btn(pyxel.KEY_UP):
self.y = max(0, self.y - 2)
if pyxel.btn(pyxel.KEY_DOWN):
self.y = min(120-8, self.y + 2) # 120 --> 120-8
if pyxel.btn(pyxel.KEY_LEFT):
self.x = max(0, self.x - 2)
if pyxel.btn(pyxel.KEY_RIGHT):
self.x = min(160-8, self.x + 2) # 160 --> 160-8
def draw(self):
pyxel.rect(self.x, self.y, 8, 8, 9)
def collides_with(self, other):
return abs(self.x - other.x) < 8 and abs(self.y - other.y) < 8
class Enemy:
def __init__(self, x, y):
self.x = x
self.y = y
def update(self):
self.x += 1
def draw(self):
pyxel.rect(self.x, self.y, 8, 8, 8)
def reset(self):
self.x = 0
self.y = pyxel.rndi(0, 112) # random(120) --> rndi(0, 112)
def is_off_screen(self):
return self.x > 160
class Score:
def __init__(self, x, y):
self.x = x
self.y = y
self.value = 0
def increase(self):
self.value += 1
def draw(self):
pyxel.text(self.x + 40, self.y, 'SCORE: {}'.format(self.value), 7)
App()
以下のような説明がありました。これってゲームのプログラムのお手本みたい。
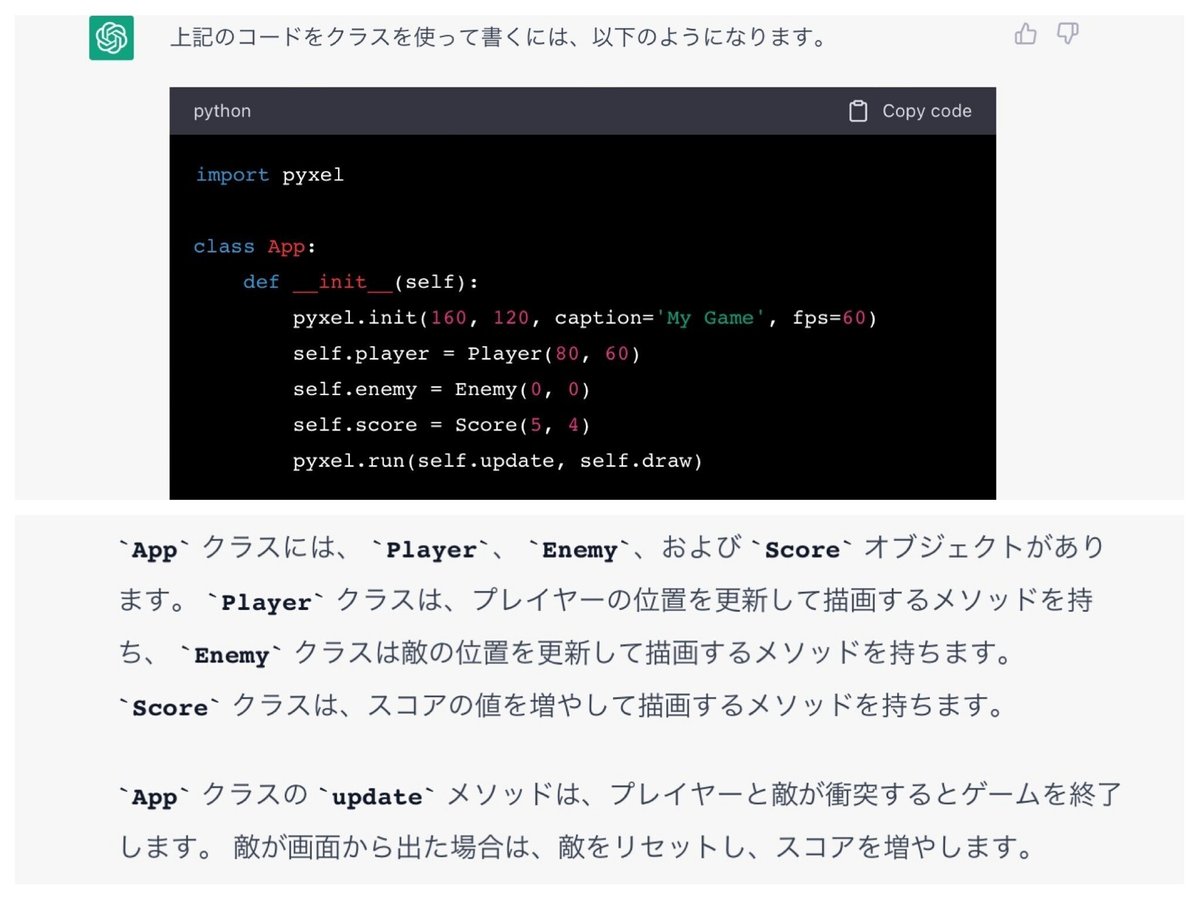
これからChatGPTで何ができるか楽しみ(^-^)
Pyxelのインストール
Windows なら pip install -U pyxel
詳しくは下記参照
この記事が気に入ったらサポートをしてみませんか?