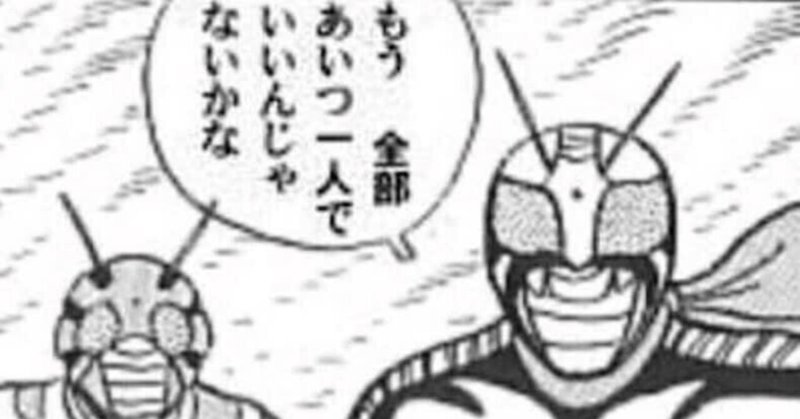
AI編集者がプログラム修正に挑戦!その結果は?
このタイトルをChatGPTが出した時点で大爆笑でした
はじめに - 実験の背景と目的について説明します。
なんとなくChatGPTにプログラミングをさせるとすごいことになるんだろうなと思っていたのですが、具体的にどういう使い方をすれば良いのかがわからなかったので、コーディングと、特にデータ分析者にありがちな汚いコードを直してもらうプロセスをAIにしてもらおうと考えてみました。
プログラム修正のプロセス - ChatGPTがプログラム修正に取り組む様子を詳しく解説します。
最初にデータフレーム内のある条件に沿ってNAを任意の変数で埋めてくれるコードを書いてくれと言ったところ普通に返ってきました
input:Could you make python function that inserted dataframe with variable into na data with certain condition and return dataframe
import pandas as pd
def fill_na(df, var, condition):
df.loc[condition, :] = df.loc[condition, :].fillna(var)
return df
Here, df is the input DataFrame, var is the variable to use to fill in the NaN values, and condition is a boolean condition that is used to determine which rows of the DataFrame to fill in.
For example, suppose you have a DataFrame with some NaN values and you want to fill in the NaN values in the 'Age' column where the 'Sex' column is 'Female':
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, np.nan, 30, np.nan],
'Sex': ['Female', 'Male', 'Female', 'Male']}
df = pd.DataFrame(data)
You can call the fill_na() function with the appropriate arguments to fill in the NaN values where the 'Sex' column is 'Female':
df = fill_na(df, 0, df['Sex'] == 'Female')
実はここ、テストデータがいみがないんですね。
まぁちゃんと自前でテストを直しておきましたが。
で、じゃあこれをきれいにしてくれ、pep8に従って、データクラスを使って、しかも型ヒントも使うんだ。と言ってみると、ちゃんと直してくれます
input: make it approprite with pep8 standard and data class and type hint like annotation
Sure, here's an updated version of the function that conforms to PEP8 standards, uses a data class, and includes type hints for the function arguments and return value:
from typing import Any, Union
import pandas as pd
from dataclasses import dataclass
@dataclass
class FillNaArgs:
df: pd.DataFrame
var: Any
condition: Union[pd.Series, bool]
def fill_na(args: FillNaArgs) -> pd.DataFrame:
args.df.loc[args.condition, :] = args.df.loc[args.condition, :].fillna(args.var)
return args.df
まぁ一部うん?と思うところはあるんですが、
全然いけますね
修正前と修正後の比較 - ChatGPTが修正したプログラムと修正前のプログラムを比較して、どのように変化したかを分析します。
そこで今度は自分で以前書いた汚い関数を入れてみました
could you make a comment and put more appropriate title for below function
def read_pickles(filelist):
number = len(file_list)
loop_one = 10
loop_two = math.ceil(number /10)
price_data_two = pd.DataFrame()
for one in range(loop_one):
price_data_one = pd.DataFrame()
for two in range(loop_two):
print(loop_two * one + two, end=" ")
try:
file = filelist[loop_two * one + two ]
data = pd.read_pickle(file)
price_data_one = pd.concat([price_data_one, data], axis=0)
except EOFError:
print(file)
except IndexError:
break
price_data_one = price_data_one.drop_duplicates()
price_data_two = pd.concat([price_data_two, price_data_one], axis=0)
return price_data_two
Sure! Here's the updated function with a more appropriate title and some comments:
import math
import pandas as pd
def read_pickles_and_concatenate(file_list):
"""
Reads a list of pickle files and concatenates their contents into a single Pandas DataFrame.
Args:
file_list (list): A list of file paths to read.
Returns:
pd.DataFrame: A single Pandas DataFrame containing the contents of all the input files.
"""
number = len(file_list)
loop_one = 10
loop_two = math.ceil(number / 10)
price_data_two = pd.DataFrame()
for one in range(loop_one):
price_data_one = pd.DataFrame()
for two in range(loop_two):
print(loop_two * one + two, end=" ")
try:
file = file_list[loop_two * one + two]
data = pd.read_pickle(file)
price_data_one = pd.concat([price_data_one, data], axis=0)
except EOFError:
print(file)
except IndexError:
break
price_data_one = price_data_one.drop_duplicates()
price_data_two = pd.concat([price_data_two, price_data_one], axis=0)
return price_data_two
この後はちゃんとpep8なんかにも従ってねと言ったら対応してくれました
import math
import pandas as pd
from typing import List
from dataclasses import dataclass
@dataclass
class ReadAndConcatArgs:
file_list: List[str]
def read_pickles_and_concatenate(args: ReadAndConcatArgs) -> pd.DataFrame:
"""
Reads a list of pickle files and concatenates their contents into a single Pandas DataFrame.
Args:
args.file_list (List[str]): A list of file paths to read.
Returns:
pd.DataFrame: A single Pandas DataFrame containing the contents of all the input files.
"""
number = len(args.file_list)
loop_one = 10
loop_two = math.ceil(number / 10)
price_data_two = pd.DataFrame()
for one in range(loop_one):
price_data_one = pd.DataFrame()
for two in range(loop_two):
print(loop_two * one + two, end=" ")
try:
file = args.file_list[loop_two * one + two]
data = pd.read_pickle(file)
price_data_one = pd.concat([price_data_one, data], axis=0)
except EOFError:
print(file)
except IndexError:
break
price_data_one = price_data_one.drop_duplicates()
price_data_two = pd.concat([price_data_two, price_data_one], axis=0)
return price_data_two
すっげぇ
もう本当にこいつだけでいいんじゃないかな?
成果と課題 - ChatGPTのプログラム修正の成果と課題をまとめ、AI編集者の限界について考察します。
限界?
えーっと、一部怪しいところはあったので、そういうのは今後の課題ですね。
アウトプットのコードの頭にCSSとかrとか書いてあって、お茶目だなぁと思いました。
そのうち治ると思います
何を設計すれば良いのかとか、
どういう流れを作るべきなのかは引き続き人間なのかな?
ここも怪しい気持ちもしないでもないですが、
コーディングの半分ぐらいはAIがやっちゃってくれるんじゃないかな?
特にコードをきれいにしてくださいとかって依頼に関してははーいって言って、ChatGPTに流し込んじゃったほうが早いと思います。
まとめ - 実験を通じて得た知見をまとめ、今後のAI技術の進化について展望します。
さて、冒頭の画像に戻ってきますが、
もう全部あいつ一人でいいんじゃないかな?
そんな気持ちにさせてくれなくもない今日このごろです。
明日から仕事のコードをそれっぽく加工して、そう見えないようにして、
AIに読みやすくコメントを追加してもらって、色々としてもらえますね
少し前のグーグルのプログラマーがプログラミングを海外のエンジニア二外注して、自分は遊んで給料をもらっていたなんて話がありましたが、
これは普通にそれができそうなレベルですね
コパイロットできちゃうんじゃないかな?
この記事が気に入ったらサポートをしてみませんか?