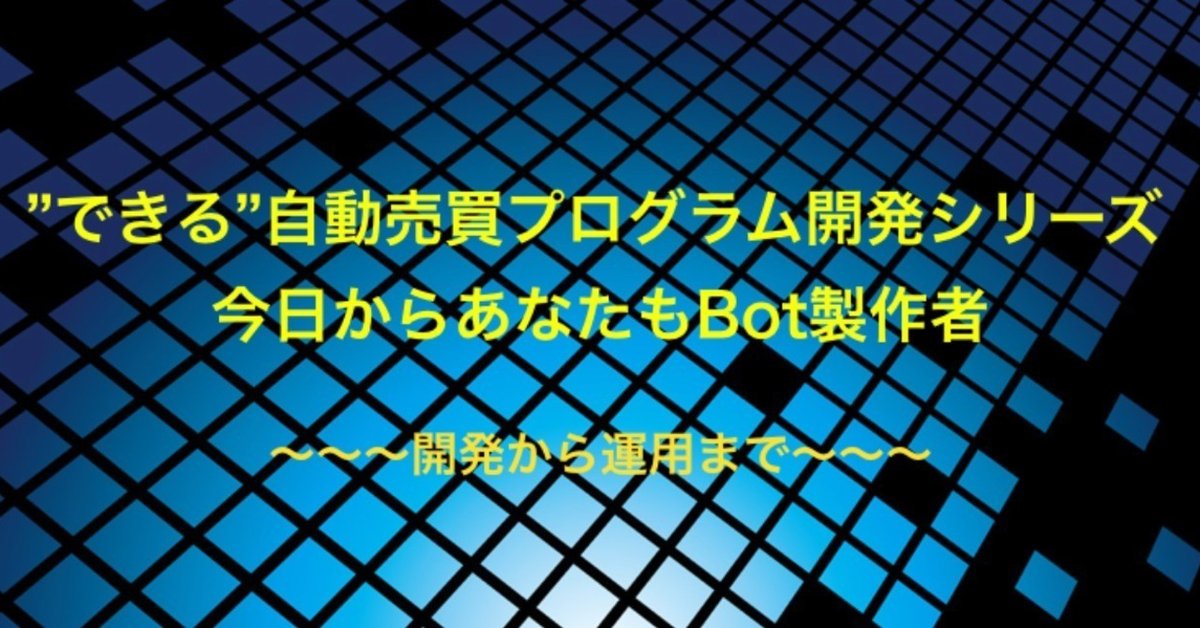
[python] bitflyer ohlcv取得
目次
・cryptowat.chからデータ取得
・pythonで記述
・動作状況
●cryptowat.chからデータ取得
ビットコイン界隈の方々で知らない人はおそらく居ない「cryptowat.ch」からデータを取得します。
大変膨大な取引所のリアルタイムOHLCVデータ等が無料で手に入るとあって、使用している人も結構多いのではないでしょうか。
●pythonで記述
さっそくコードです。
#===============================================
# 外部モジュール
#===============================================
import urllib3
import json
import datetime
import time
# InsecureRequestWarning:のワーニングた毎回出力されるので、cryptowat.chのワーニングは出さないように抑制する。
# 詳細は https://urllib3.readthedocs.io/en/latest/advanced-usage.html#ssl-warnings
urllib3.disable_warnings()
#===============================================
# 常数
#===============================================
# OHLCV足の周期
PERIOD = 60 # 秒
# OHLCV足の取得数
SIZE = 10 # 個
# sleep時間
SLEEP_T = 1
# httpオブジェクト取得
HTTP = urllib3.PoolManager()
# ==================================
# getOHLCV
# OHLCVデータ取得関数
# params:
# http: HTTPオブジェクト
# start: 開始時間(UNIXTIME)
# period: 足の期間(秒)
# return:
# resp: OHLCVデータ
# ==================================
def getOHLCV(http, start, period):
# cryptowat.chのURLを設定
url = 'https://api.cryptowat.ch/markets/bitflyer/btcfxjpy/ohlc?after=' + str(start) + '&periods=' + str(period)
# レスポンス取得
resp = json.loads(http.request('GET', url).data.decode('utf-8'))['result'][str(period)]
# 戻り値
return resp
# おまじない
if __name__ == '__main__':
# 1秒待ってのループ処理
while True:
try:
# start時間(UNIXTIME)を取得
start = int(time.time()) - PERIOD * SIZE
# OHLCV取得
resp = getOHLCV(HTTP, start, PERIOD)
# 表示
print('----------------------------------------------------------------------')
print('date time , open , high , low , close , volume ')
print('----------------------------------------------------------------------')
for r in resp:
print(str(datetime.datetime.fromtimestamp(r[0])) + ", " + str(r[1]) + ", " + str(r[2]) + ", " + str(r[3]) + ", " + str(r[4]) + ", " + str(r[5]) + ", " + str(r[6]))
except Exception as e:
print("exception: ", e.args)
# sleep
time.sleep(SLEEP_T)
このコードで使用している取引所は
・BitFlyer FX
です。
https://api.cryptowat.ch/markets/bitflyer/btcfxjpy
を指定しています。
引数としては、
・after : 取得開始日時(UNIXTIME)
・period : 足間隔(秒)
です。
上記の例では1分足を 現在時刻から10個分の過去足から取得しています。
●動作状況
コードを「get_ohlcv.py」というファイルに格納し、実行は
python3 get_ohlcv.py
で実行開始です。
----------------------------------------------------------------------
date time , open , high , low , close , volume
----------------------------------------------------------------------
2018-07-15 19:52:00, 727031, 727300, 724895, 726302, 704.2473, 511388770
2018-07-15 19:53:00, 726232, 727000, 726011, 726490, 333.0071, 241912800
2018-07-15 19:54:00, 726490, 727000, 726400, 727000, 324.58936, 235892380
2018-07-15 19:55:00, 726950, 727000, 726170, 726334, 286.39075, 208086930
2018-07-15 19:56:00, 726330, 726498, 726000, 726267, 180.42708, 131027770
2018-07-15 19:57:00, 726208, 726589, 726107, 726250, 252.79616, 183608200
2018-07-15 19:58:00, 726193, 726249, 725650, 725910, 267.87173, 194448460
2018-07-15 19:59:00, 725926, 726253, 725766, 725892, 231.88284, 168347820
2018-07-15 20:00:00, 725873, 725962, 725000, 725647, 307.5684, 223134580
2018-07-15 20:01:00, 725614, 725648, 725233, 725444, 188.50922, 136760030
2018-07-15 20:02:00, 725459, 725614, 725365, 725381, 109.28505, 79285700
2018-07-15 20:03:00, 725400, 725483, 725278, 725483, 54.665718, 39652804
----------------------------------------------------------------------
date time , open , high , low , close , volume
----------------------------------------------------------------------
2018-07-15 19:52:00, 727031, 727300, 724895, 726302, 704.2473, 511388770
2018-07-15 19:53:00, 726232, 727000, 726011, 726490, 333.0071, 241912800
2018-07-15 19:54:00, 726490, 727000, 726400, 727000, 324.58936, 235892380
2018-07-15 19:55:00, 726950, 727000, 726170, 726334, 286.39075, 208086930
2018-07-15 19:56:00, 726330, 726498, 726000, 726267, 180.42708, 131027770
2018-07-15 19:57:00, 726208, 726589, 726107, 726250, 252.79616, 183608200
2018-07-15 19:58:00, 726193, 726249, 725650, 725910, 267.87173, 194448460
2018-07-15 19:59:00, 725926, 726253, 725766, 725892, 231.88284, 168347820
2018-07-15 20:00:00, 725873, 725962, 725000, 725647, 307.5684, 223134580
2018-07-15 20:01:00, 725614, 725648, 725233, 725444, 188.50922, 136760030
2018-07-15 20:02:00, 725459, 725614, 725365, 725381, 109.28505, 79285700
2018-07-15 20:03:00, 725400, 725483, 725278, 725483, 54.665718, 39652804
のような表示が出ればOKです。
ソフトウェア・エンジニアを40年以上やってます。 「Botを作りたいけど敷居が高い」と思われている方にも「わかる」「できる」を感じてもらえるように頑張ります。 よろしくお願い致します。