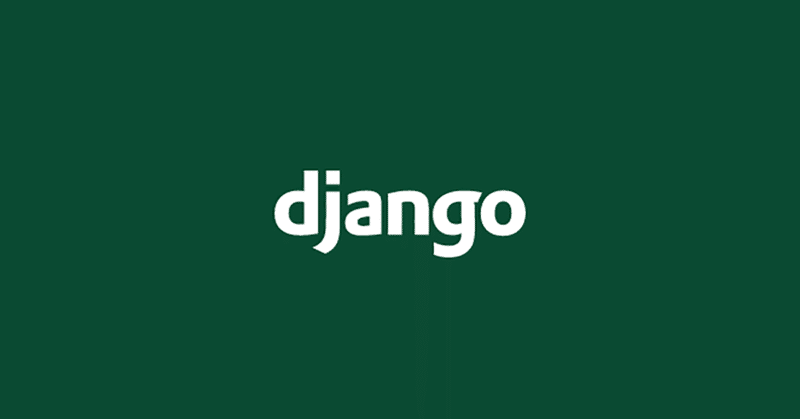
Django 入門 (4) - POSTとGET
「Django」のPOSTとGETの使い方をまとめました。
・Django 3.0.8
前回
1. POSTの利用
(1) 「hello_app/hello/templates/hello/index.html」を以下のように編集。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
</head>
<body>
<h3>{{message}}</h3>
<form action="{% url 'form' %}" method="post">
{% csrf_token %}
<input type="text" id="message" name="message">
<input type="submit" value="送信">
</form>
</body>
</html>
テンプレートタグは、次のとおりです。
{% csrf_token %} : CSRF無効化
(2) 「hello_app/hello/urls.py」を以下のように編集。
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
path('form', views.form, name='form'),
]
(3) 「hello_app/hello/views.py」を以下のように編集。
request.POST['<パラメータ名>'] でPOSTのパラメータを取得しています。
from django.shortcuts import render
def index(request):
params = {'message':'メッセージを入力してください。'}
return render(request, 'hello/index.html', params)
def form(request):
message = request.POST['message']
params = {'message':message+'を入力しました。'}
return render(request, 'hello/index.html', params)
(4) Webアプリを実行し、ブラウザで「http://localhost:8000/hello」を開く。
2. GETの利用
(1) 「hello_app/hello/templates/hello/index.html」を以下のように編集。
formタグの「method="post"」を「method="get"」に変更しています。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
</head>
<body>
<h3>{{message}}</h3>
<form action="{% url 'form' %}" method="get">
{% csrf_token %}
<input type="text" id="message" name="message">
<input type="submit" value="送信">
</form>
</body>
</html>
(2) 「hello_app/hello/views.py」を以下のように編集。
request.GET['<パラメータ名>'] でGETのパラメータを取得しています。
from django.shortcuts import render
def index(request):
params = {'message':'メッセージを入力してください。'}
return render(request, 'hello/index.html', params)
def form(request):
message = request.GET['message']
params = {'message':message+'を入力しました。'}
return render(request, 'hello/index.html', params)
(3) Webアプリを実行し、ブラウザで「http://localhost:8000/hello」を開く。
【おまけ】 HttpRequest と HttpResponse
「HttpRequest」の主なプロパティとメソッドは、次のとおりです。
◎ 主なプロパティ
・GET : GETパラメータの辞書
・POST : POSTパラメータの辞書
・COOKIES : Cookieの辞書
・FILES : ファイルの辞書 (キーはファイル名、値はUploadedFile)
・scheme : スキーム(http, https)
・body : 生のHTTPボディ
・path : パス (/music/bands/the_beatles/)
・path_info : パス情報 (/bands/the_beatles/)
・method : メソッド (GET, POST)
・encoding : エンコード
・content_type : CONTENT_TYPE
・content_params : CONTENT_TYPEヘッダに含まれる辞書
・headers : ヘッダ
・resolver_match : 解決されたURLを表すResolverMatch
◎ 主なメソッド
・get_host() : ホスト。
・get_port() : ポート
・get_full_path() : パス。
・get_full_path_info() : フルパス情報。
・build_absolute_uri(location) : 絶対URI形式のパス。
・get_signed_cookie(key, default, salt, max_age) : Cookie。
・is_secure() : httpsかどうか。
・is_ajax() : XMLHttpRequestによる通信かどうか。
・read(size) : ボディの読み込み。
・readline() : ボディの読み込み。
・readlines() : ボディの読み込み。
「HttpResponse」の主なプロパティとメソッドは、次のとおりです。
◎ コンストラクタ
HttpResponse(content, content_type, status, reason, charset)
◎ 主なプロパティ
・content : コンテンツ。
・charset : 応答がエンコードされる文字セット。
・status_code : HTTP Status Code。
・reason_phrase : HTTP Reason Phrase。
・streaming : 常にfalse。
・closed : レスポンスが閉じられた場合True。
◎ 主なメソッド
・has_header(header) : ヘッダを持つかどうか。
・set_cookie(key, value, max_age, expires, path, domain, secure, httponly, samesite) : Cookieの指定。
・set_signed_cookie(key, value, salt, max_age, expires, path, domain, secure, httponly, samesite) : Cookieの指定。
・delete_cookie(key, path, domain, samesite) : Cookieの削除。
次回
この記事が気に入ったらサポートをしてみませんか?