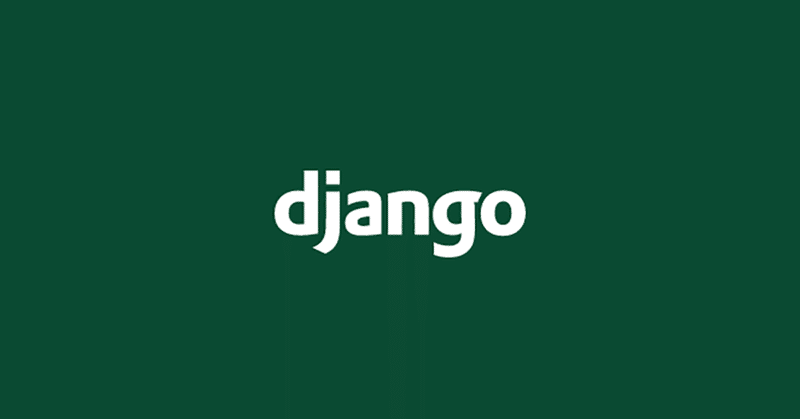
Django 入門 (3) - テンプレートと静的ファイル
「Django」のテンプレートと静的ファイルの使い方をまとめました。
・Django 3.0.8
前回
1. テンプレートと静的ファイルの前準備
「テンプレート」「静的ファイル」を使うには、「hello_app/hello_app/settings.py」の「INSTALLED_APPS」に、テンプレートを利用するアプリを追加します。
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'hello',
]
2. テンプレートの利用
テンプレートの利用の手順は、次のとおりです。
(1) 「hello_app/hello/templates/hello/index.html」を作成し、以下のように編集。
「テンプレート」は、「<プロジェクト名>/<アプリ名>/templates/<アプリ名>/」に配置します。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>{{title}}</title>
</head>
<body>
<h1>{{message}}</h1>
</body>
</html>
テンプレートタグは、次のとおりです。
{{変数名}} : 変数の埋め込み部分
(2) アプリ「hello_app/hello」の「views.py」を以下のように編集。
from django.shortcuts import render
from django.http import HttpResponse
def index(request):
params = {
'title' : 'helloworld',
'message' : 'Hello World!'
}
return render(request, 'hello/index.html', params)
(3) Webアプリを実行し、ブラウザで「http://localhost:8000/hello」を開く。
3. 静的ファイルの利用
静的ファイルの利用の手順は、次のとおりです。
(1) 「hello_app/hello/static/hello/style.css」を作成し、以下のように編集。
h1 {
color:red;
}
(2) 「hello_app/hello/templates/hello/index.html」を以下のように編集。
「静的ファイル」は、「<プロジェクト名>/<アプリ名>/static/<アプリ名>/」に配置します。
{% load static %}
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>{{title}}</title>
<link rel="stylesheet" type="text/css" href="{% static 'hello/style.css' %}" />
</head>
<body>
<h1>{{message}}</h1>
</body>
</html>
テンプレートタグは、次のとおりです。
{% load static %} : 静的ファイルを利用可能にする命令
{% static 'hello/style.css' %} : 静的ファイルのパス
(3) Webアプリを実行し、ブラウザで「http://localhost:8000/hello」を開く。
4. ページの遷移
ページの遷移の手順は、次のとおりです。
(1) 「hello_app/hello/templates/hello/index.html」を作成し、以下のように編集。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
</head>
<body>
トップページです。<br>
<a href="{% url 'next' %}">次へ</a>
</body>
</html>
テンプレートタグは、次のとおりです。
{% url '<名前>' %} : リンクのパス。<名前>はurlpatternsのpathのname。
(2) 「hello_app/hello/templates/hello/next.html」を作成し、以下のように編集。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
</head>
<body>
次のページです。<br>
<a href="{% url 'index' %}">戻る</a>
</body>
</html>
(3) 「hello_app/hello/urls.py」を以下のように編集。
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
path('next', views.next, name='next'),
]
(4) 「hello_app/hello/views.py」を以下のように編集。
from django.shortcuts import render
from django.http import HttpResponse
def index(request):
return render(request, 'hello/index.html')
def next(request):
return render(request, 'hello/next.html')
(5) Webアプリを実行し、ブラウザで「http://localhost:8000/hello」を開く。
【おまけ】 settings.pyの初期設定
「settings.py」の初期設定は、次のとおりです。
import os
# プロジェクトのベースパス (/Users/<username>/work/hello_app/)
BASE_DIR = os.path.dirname(os.path.dirname(os.path.abspath(__file__)))
# 秘密鍵 (本番環境の秘密鍵は秘密にする)
SECRET_KEY = 'XXXXXXXXXXXX'
# デバッグ (本番環境ではFalseにする)
DEBUG = True
# サイトを配信できるホスト名のリスト
ALLOWED_HOSTS = []
# アプリのリスト
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
]
# ミドルウェアのリスト
MIDDLEWARE = [
'django.middleware.security.SecurityMiddleware',
'django.contrib.sessions.middleware.SessionMiddleware',
'django.middleware.common.CommonMiddleware',
'django.middleware.csrf.CsrfViewMiddleware',
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django.contrib.messages.middleware.MessageMiddleware',
'django.middleware.clickjacking.XFrameOptionsMiddleware',
]
# ルートのURL設定のパス
ROOT_URLCONF = 'hello_app.urls'
# テンプレートエンジンの設定のリスト
TEMPLATES = [
{
'BACKEND': 'django.template.backends.django.DjangoTemplates',
'DIRS': [],
'APP_DIRS': True,
'OPTIONS': {
'context_processors': [
'django.template.context_processors.debug',
'django.template.context_processors.request',
'django.contrib.auth.context_processors.auth',
'django.contrib.messages.context_processors.messages',
],
},
},
]
# WSGIアプリオブジェクトのパス
WSGI_APPLICATION = 'hello_app.wsgi.application'
# データベースの設定
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': os.path.join(BASE_DIR, 'db.sqlite3'),
}
}
# パスワード検証のバリデーターのリスト
AUTH_PASSWORD_VALIDATORS = [
{
'NAME': 'django.contrib.auth.password_validation.UserAttributeSimilarityValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.MinimumLengthValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.CommonPasswordValidator',
},
{
'NAME': 'django.contrib.auth.password_validation.NumericPasswordValidator',
},
]
# 国際化の設定
LANGUAGE_CODE = 'en-us'
TIME_ZONE = 'UTC'
USE_I18N = True
USE_L10N = True
USE_TZ = True
# 静的ファイル(CSS、JavaScript、画像)の設定
STATIC_URL = '/static/'
【おまけ】 組み込みタグとフィルタの一覧
組み込みタグの一覧は、次のとおりです。
・autoescape
・block
・comment
・csrf_token
・cycle
・debug
・extends
・filter
・firstof
・for
・for ... empty
・if
・ifequal
・ifnotequal
・ifchanged
・include
・load
・lorem
・now
・regroup
・resetcycle
・spaceless
・templatetag
・url
・verbatim
・widthratio
・with
フィルタの一覧は、次のとおりです。
・add
・addslashes
・capfirst
・center
・cut
・date
・default
・default_if_none
・dictsort
・dictsortreversed
・divisibleby
・escape
・escapejs
・filesizeformat
・first
・floatformat
・force_escape
・get_digit
・iriencode
・join
・json_script
・last
・length
・length_is
・linebreaks
・linebreaksbr
・linenumbers
・ljust
・lower
・make_list
・phone2numeric
・pluralize
・pprint
・random
・rjust
・safe
・safeseq
・slice
・slugify
・stringformat
・striptags
・time
・timesince
・timeuntil
・title
・truncatechars
・truncatechars_html
・truncatewords
・truncatewords_html
・unordered_list
・upper
・urlencode
・urlize
・urlizetrunc
・wordcount
・wordwrap
・yesno
国際化タグとフィルタの一覧は、次のとおりです。
・i18n
・l10n
・tz
その他のタグとフィルタライブラリの一覧は、次のとおりです。
・django.contrib.humanize
・static
・static
・get_static_prefix
・get_media_prefix
次回
この記事が気に入ったらサポートをしてみませんか?