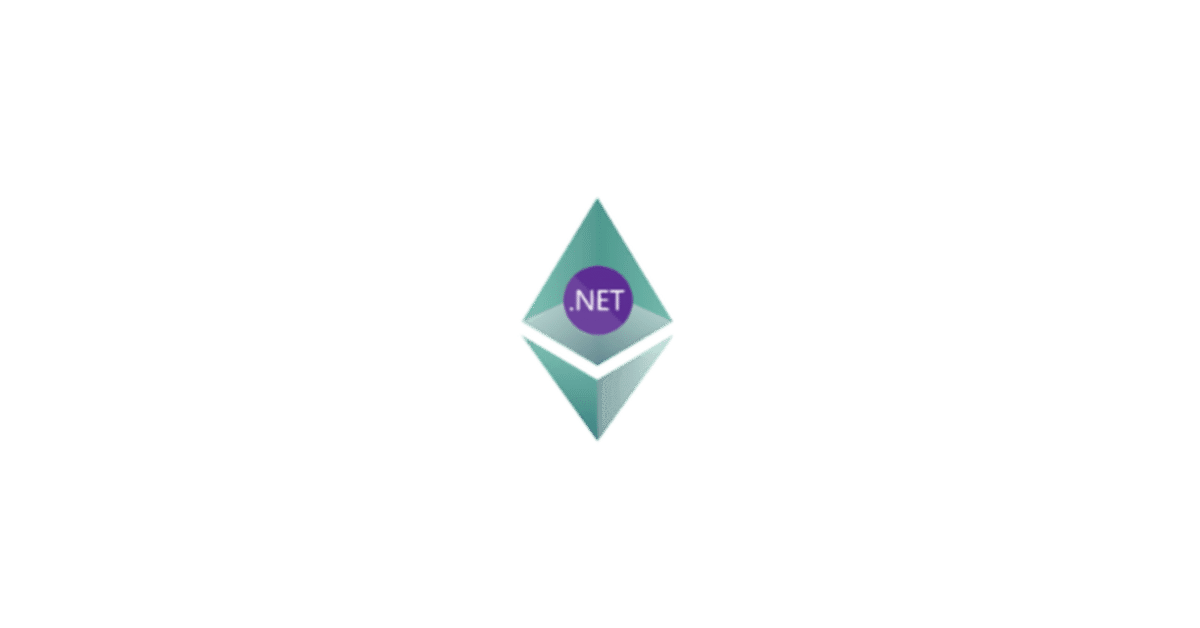
Nethereum 入門 (4) - TestChains
「TestChains」の使い方をまとめました。
前回
1. TestChains
「TestChains」(Devchain) は、ローカルでテスト用のブロックチェーンを起動するために必要なもの一式が含まれているリポジトリです。
このテスト用のブロックチェーンは、作業をプライベートに保ちながら、トランザクションの検証時間を短縮します。各チェーンは、コンセンサスモデルとしてPoA (Proof of Authority)を使用します。
2. TestChainsの起動
「TestChains」を起動する手順は、次のとおりです。
(1) TestChainsのリポジトリをクローン。
(2) startgeth.bat (Windows) またはstartgeth.sh (Mac) を実行。
・geth-clique-windows/startgeth.bat
・geth-clique-mac/startgeth.sh
$ cd TestChains/geth-clique-mac/geth-clique-mac
$ ./startgeth.sh
3. TestChainsの利用
TestChainsを利用したサンプルコードを作成します。
(1) dotnetプロジェクトを作成し、Program.csを以下のように編集。
using System;
using System.Text;
using Nethereum.Hex.HexConvertors.Extensions;
using System.Threading.Tasks;
using Nethereum.Web3;
using Nethereum.Web3.Accounts;
namespace NethereumSample
{
class Program
{
static void Main(string[] args)
{
GetAccountBalance().Wait();
Console.ReadLine();
}
static async Task GetAccountBalance()
{
// 送信元アカウントの準備 (TestChainsで提供されてるテスト用アカウント)
var privateKey = "0xb5b1870957d373ef0eeffecc6e4812c0fd08f554b37b233526acc331bf1544f7";
var chainId = 444444444500;
var account = new Account(privateKey, chainId);
Console.WriteLine("Our account: " + account.Address);
// アカウントを使用してWeb3インスタンスを生成
var web3 = new Web3(account);
// 送信元アカウントの残高の確認
var fromAddress = "0x12890d2cce102216644c59daE5baed380d84830c";
var balance = await web3.Eth.GetBalance.SendRequestAsync(fromAddress);
Console.WriteLine("Sender account balance before sending Ether: " + balance.Value + " Wei");
Console.WriteLine("Sender account balance before sending Ether: " + Web3.Convert.FromWei(balance.Value) + " Ether");
// 送信先アカウントの残高の確認
var toAddress = "0x13f022d72158410433cbd66f5dd8bf6d2d129924";
balance = await web3.Eth.GetBalance.SendRequestAsync(toAddress);
Console.WriteLine("Receiver account balance before sending Ether: " + balance.Value + " Wei");
Console.WriteLine("Receiver account balance before sending Ether: " + Web3.Convert.FromWei(balance.Value) + " Ether");
// etherの転送
var transaction = await web3.Eth.GetEtherTransferService()
.TransferEtherAndWaitForReceiptAsync(toAddress, 1.11m);
// 送信先アカウントの残高の確認
balance = await web3.Eth.GetBalance.SendRequestAsync(toAddress);
Console.WriteLine("Receiver account balance after sending Ether: " + balance.Value);
Console.WriteLine("Receiver account balance after sending Ether: " + Web3.Convert.FromWei(balance.Value) + " Ether");
}
}
}
(2) 「dotnet run」で実行。
$ dotnet run
Our account: 0x12890D2cce102216644c59daE5baed380d84830c
Sender account balance before sending Ether: 10880332376531662572355584 Wei
Sender account balance before sending Ether: 10880332.376531662572355584 Ether
Receiver account balance before sending Ether: 0 Wei
Receiver account balance before sending Ether: 0 Ether
Receiver account balance after sending Ether: 1110000000000000000
Receiver account balance after sending Ether: 1.11 Ether