TypeScriptのはじめかた(Windows)
<前提>
NodeJS、VSCodeがコンピュータにインストールされていること
node -v
1. 任意のディレクトリにプロジェクトフォルダを作成する
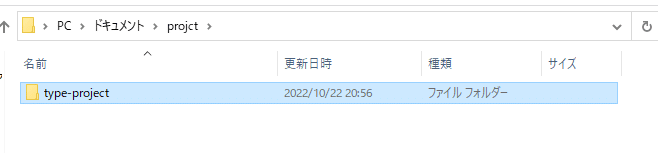
2. プロジェクトフォルダに移動して、VSCodeを開く
cd "C:\Users\user-name\Documents\projct\type-project"
code .
3. package.jsonを作成
npm init -y
4. TypeScript、ts-nodeを開発環境にインストール
npm i -D typescript ts-node
<任意> HTTPクライアントをインストール
npm i axios node-fetch
npm i -D @types/node-fetch
5. tsconfig.jsonを作成
npx tsc --init
6. tsconfig.jsonを修正
{
"compilerOptions": {
"module": "ES2022",
"moduleResolution": "node",
}
}
7. package.jsonを修正
{
"scripts": {
"dev": "node --loader ts-node/esm src/index.ts"
},
"type": "module",
}
8. srcフォルダとその配下にindex.tsを作成して実行
npm run dev
<おまけ> axiosを用いたHTTPリクエスト(サンプル)
import axios, { AxiosRequestConfig, AxiosResponse, AxiosError } from "axios";
// ***** axios *****
const url = "https://jsonplaceholder.typicode.com/users";
const method = "get";
interface OptionType {
url: string;
method: string;
}
interface User {
id: number;
name: string;
username: string;
email: string;
address: {};
phone: string;
website: string;
company: {};
}
const axiosOption: AxiosRequestConfig<OptionType> = { url, method };
axios<User[], AxiosResponse<User[], OptionType>, OptionType>(axiosOption)
.then((response) => {
console.log({
data: response.data,
status: response.status,
});
})
.catch((error: AxiosError<User[], OptionType>) => {
console.log(
{ isAxiosError: error.isAxiosError },
{ message: error.message }
);
});
この記事が気に入ったらサポートをしてみませんか?