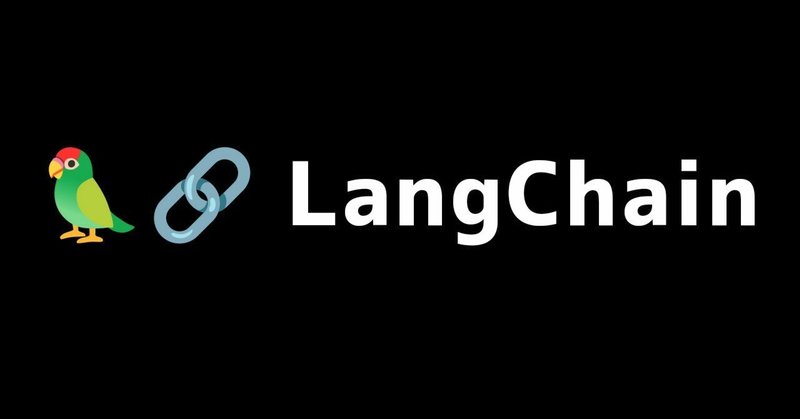
LangChainのメモリについてのメモ
注意:メモなので、不親切な説明+適当な情報が含まれます。
概要-メモリ
デフォルトでは、チェーンとエージェントは状態を持たず、つまり各入力クエリを独立して扱います。一部のアプリケーション(チャットボットはその一例です)では、以前の相互作用を短期的にも長期的にも覚えることが非常に重要です。そのために「メモリ」という概念が存在しています。
Conversational Memory
より簡単な形のメモリの一つは、チャットボットにおいて以前の会話を覚えることです。これを実現する方法には、いくつかの異なる方法があります。
Buffer:これは、過去N回の相互作用をコンテキストとして渡すだけです。Nは固定の数値、相互作用の長さなどに基づいて選択できます。
Summary:これは、以前の会話を要約し、生のダイアログ自体の代わりにその要約を渡すことを意味します。バッファと比較して、情報を圧縮するため、より多くの情報が失われますが、コンテキストの長さ制限に遭遇する可能性が低くなります。
Combination:上記の2つの方法の組み合わせで、サマリーを計算するが、一部の以前の相互作用も直接渡す方法です!
Entity Memory
より複雑な形のメモリは、会話中の特定のエンティティに関する情報を覚えることです。これは、時間の経過とともに情報を覚えるより直接的で組織化された方法です。より構造化された形式にすることの利点は、特定のエンティティについて知られていることを簡単に調べることができることです。このタイプのメモリの使用方法についてのガイドについては、このノートブックを参照してください。
以下メモリーごとにサンプルプログラム+動作メモ。
A.ConversationBufferMemory
ConversationBufferMemory とは?
入出力をすべて記録して、されたコンテキストに追加するシンプルなタイプのメモリ。
ComverstionBufferMemory 引数
human_prefix: str = "Human"
ai_prefix: str = "AI"
buffer: str = ""
output_key: str | None = None
input_key: str | None = None
memory_key: str = "history") -> None
サンプルプログラム
from langchain.chains.conversation.memory import ConversationBufferMemory
from langchain import OpenAI, LLMChain, PromptTemplate
import os
os.environ["OPENAI_API_KEY"] = "..."
template = """You are a chatbot having a conversation with a human.
{chat_history}
Human: {human_input}
Chatbot:"""
prompt = PromptTemplate(
input_variables=["chat_history", "human_input"],
template=template
)
memory = ConversationBufferMemory(memory_key="chat_history")
llm_chain = LLMChain(
llm=OpenAI(),
prompt=prompt,
verbose=True,
memory=memory,
)
print(llm_chain.predict(human_input="Hi there my friend"))
print(llm_chain.predict(human_input="Not to bad - how are you?"))
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
Chatbot:
> Finished chain.
Hi there, how can I help you?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
AI: Hi there, how can I help you?
Human: Not to bad - how are you?
Chatbot:
> Finished chain.
I'm doing well, thanks for asking! How about you?
JP:
Prompt after formatting:あなたは人間と会話をしているチャットボットです。
Human:こんにちは、私の友人
Chatbot:こんにちは、どうされましたか?
Human: 人間:悪くはないですね、お元気ですか?
Chatbot:私は元気です!聞いてくれてありがとう。あなたはどうですか?
参照
B.ConversationSummaryMemory
ConversationSummaryMemory 引数
buffer: str = ""
human_prefix: str = "Human"
ai_prefix: str = "AI"
llm: BaseLLM
prompt: BasePromptTemplate = SUMMARY_PROMPT
memory_key: str = "history"
output_key: str | None = None
input_key: str | None = None) -> None
サンプルプログラム
from langchain.chains.conversation.memory import ConversationBufferMemory
from langchain.chains.conversation.memory import ConversationSummaryMemory
from langchain import OpenAI, LLMChain, PromptTemplate
import os
os.environ["OPENAI_API_KEY"] = "...."
template = """You are a chatbot having a conversation with a human.
{chat_history}
Human: {human_input}
Chatbot:"""
prompt = PromptTemplate(
input_variables=["chat_history", "human_input"],
template=template
)
memory = ConversationSummaryMemory(llm=OpenAI(),memory_key="chat_history")
llm_chain = LLMChain(
llm=OpenAI(),
prompt=prompt,
verbose=True,
memory=memory,
)
print("Result: "+llm_chain.predict(human_input="Hi there my friend"))
print("Result: "+llm_chain.predict(human_input="Not to bad - how are you?"))
print("Result: "+llm_chain.predict(human_input="Not to bad - how are you?"))
print("Result: "+llm_chain.predict(human_input="Not to bad - how are you?"))
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
Chatbot:
> Finished chain.
Hi there! How can I help you?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
AI: Hi there! How can I help you?
Human: Not to bad - how are you?
Chatbot:
> Finished chain.
I'm doing great, thanks for asking!
AI: Hi there! How can I help you?
Human: Not to bad - how are you?
AI: Hi there! How can I help you?
Human: Not to bad - how are you?
AI: I'm doing great, thanks for asking!
Human: Not to bad - how are you?
Chatbot:
Result: Hi there! It's nice to meet you. How are you doing today?
> Entering new LLMChain chain...Prompt after formatting:You are a chatbot having a conversation with a human.
The human greets the AI, to which the AI responds with a friendly greeting and inquires how the human is doing.Human: Not to bad - how are you?Chatbot:
> Finished chain.
新しいLLMChainチェーンに入力しています...
フォーマット後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間:こんにちは、私の友人
Chatbot:
チェーンの終了。
こんにちは!何かお手伝いできますか?
新しいLLMChainチェーンに入力しています...
フォーマット後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間:こんにちは、私の友人
AI:こんにちは!何かお手伝いできますか?
人間:悪くないです - あなたはどうですか?
Chatbot:
チェーンの終了。
私は素晴らしいです、お尋ねいただきありがとうございます!
AI:こんにちは!何かお手伝いできますか?
人間:悪くないです - あなたはどうですか?
AI:こんにちは!何かお手伝いできますか?
人間:悪くないです - あなたはどうですか?
AI:私は素晴らしいです、お尋ねいただきありがとうございます!
人間:悪くないです - あなたはどうですか?
Chatbot:
結果:こんにちは!あなたに会えてうれしいです。今日はお元気ですか?
新しいLLMChainチェーンに入力しています...
フォーマット後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間が挨拶をすると、AIは友好的に応えて、人間の様子を尋ねます。人間:悪くないです - あなたはどうですか?Chatbot:
チェーンの終了。
C.ConversationBufferWindowMemory
ConversationBufferWindowMemoryとは?
ConversationBufferWindowMemoryは、会話のやり取りを時間経過に応じてリストに保持します。
これは、最後のK個のやり取りのみを使用します。
これは、最近のやり取りのスライディングウィンドウを保持するために役立ちます。そうすることで、バッファがあまりにも大きくなるのを防ぐことができます。
ConversationBufferWindowMemory引数
human_prefix: str = "Human"
ai_prefix: str = "AI"
buffer: List[str] = list
memory_key: str = "history"
output_key: str | None = None
input_key: str | None = None
k: int = 5
サンプルプログラム
from langchain.chains.conversation.memory import ConversationBufferMemory,ConversationSummaryMemory,ConversationBufferWindowMemory
from langchain import OpenAI, LLMChain, PromptTemplate
import os
os.environ["OPENAI_API_KEY"] = "..."
template = """You are a chatbot having a conversation with a human.
{chat_history}
Human: {human_input}
Chatbot:"""
prompt = PromptTemplate(
input_variables=["chat_history", "human_input"],
template=template
)
# memory = ConversationSummaryMemory(llm=OpenAI(),memory_key="chat_history")
memory = ConversationBufferWindowMemory(k=2,memory_key="chat_history")
llm_chain = LLMChain(
llm=OpenAI(),
prompt=prompt,
verbose=True,
memory=memory,
)
print("Result: "+llm_chain.predict(human_input="Hi there my friend"))
print("Result: "+llm_chain.predict(human_input="Not to bad - how are you?"))
print("Result: "+llm_chain.predict(human_input="Not to bad - how are you?"))
print("Result: "+llm_chain.predict(human_input="Not to bad - how are you?"))
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
Chatbot:
> Finished chain.
Result: Hi there! How can I help you today?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
AI: Hi there! How can I help you today?
Human: Not to bad - how are you?
Chatbot:
> Finished chain.
Result: I'm doing great, thanks for asking! How about you?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
AI: Hi there! How can I help you today?
Human: Not to bad - how are you?
AI: I'm doing great, thanks for asking! How about you?
Human: Not to bad - how are you?
Chatbot:
> Finished chain.
Result: I'm doing great, thanks for asking! What can I do for you today?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Not to bad - how are you?
AI: I'm doing great, thanks for asking! How about you?
Human: Not to bad - how are you?
AI: I'm doing great, thanks for asking! What can I do for you today?
Human: Not to bad - how are you?
Chatbot:
> Finished chain.
Result: I'm doing great, thanks for asking! Is there anything I can help you with?
新しいLLMChainチェーンに入ります...
書式設定後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間:こんにちは、友達よ
チャットボット:
チェーン終了。
結果:こんにちは!今日は何かお手伝いできますか?
新しいLLMChainチェーンに入ります...
書式設定後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間:こんにちは、友達よ
AI:こんにちは!今日は何かお手伝いできますか?
人間:悪くないですね - あなたはどうですか?
チャットボット:
チェーン終了。
結果:私は元気です。お尋ねいただき、ありがとうございます!あなたはいかがですか?
新しいLLMChainチェーンに入ります...
書式設定後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間:こんにちは、友達よ
AI:こんにちは!今日は何かお手伝いできますか?
人間:悪くないですね - あなたはどうですか?
AI:私は元気です。お尋ねいただき、ありがとうございます!あなたはいかがですか?
人間:悪くないですね - あなたはどうですか?
チャットボット:
チェーン終了。
結果:私は元気です。お尋ねいただき、ありがとうございます!今日は何かお手伝いできますか?
新しいLLMChainチェーンに入ります...
書式設定後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間:悪くないですね - あなたはどうですか?
AI:私は元気です。お尋ねいただき、ありがとうございます!あなたはいかがですか?
人間:悪くないですね - あなたはどうですか?
AI:私は元気です。お尋ねいただき、ありがとうございます!今日は何かお手伝いできますか?
人間:悪くないですね - あなたはどうですか?
チャットボット:
チェーン終了。
結果:私は元気です。お尋ねいただき、ありがとうございます!何かお手伝いできますか?
D.ConversationSummaryBufferMemory
ConversationSummaryBufferMemoryとは?
ConversationSummaryBufferMemoryは、前述の2つのアイデアを組み合わせています。最近の相互作用のバッファをメモリに保持しますが、古い相互作用を完全にフラッシュするのではなく、サマリーにコンパイルして両方を使用します。ただし、以前の実装とは異なり、相互作用の数ではなくトークンの長さを使用して、相互作用をフラッシュするタイミングを決定します。
ConversationSummaryBufferMemory引数
buffer: List[str] = list
max_token_limit: int = 2000
moving_summary_buffer: str = ""
llm: BaseLLM
prompt: BasePromptTemplate = SUMMARY_PROMPT
memory_key: str = "history"
human_prefix: str = "Human"
ai_prefix: str = "AI"
output_key: str | None = None
input_key: str | None = None
サンプルプログラム
from langchain.chains.conversation.memory import ConversationBufferMemory,ConversationSummaryMemory,ConversationBufferWindowMemory,ConversationSummaryBufferMemory
from langchain import OpenAI, LLMChain, PromptTemplate
import os
os.environ["OPENAI_API_KEY"] = "..."
template = """You are a chatbot having a conversation with a human.
{chat_history}
Human: {human_input}
Chatbot:"""
prompt = PromptTemplate(
input_variables=["chat_history", "human_input"],
template=template
)
# memory = ConversationSummaryMemory(llm=OpenAI(),memory_key="chat_history")
# memory = ConversationBufferWindowMemory(k=2,memory_key="chat_history")
memory = ConversationSummaryBufferMemory(llm=OpenAI(),memory_key="chat_history")
llm_chain = LLMChain(
llm=OpenAI(),
prompt=prompt,
verbose=True,
memory=memory,
)
print("Result: "+llm_chain.predict(human_input="Hi there my friend"))
print("Result: "+llm_chain.predict(human_input="Not to bad - how are you?"))
print("Result: "+llm_chain.predict(human_input="Not to bad - how are you?"))
print("Result: "+llm_chain.predict(human_input="Not to bad - how are you?"))
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
Chatbot:
> Finished chain.
Result: Hello there! How can I help you?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
AI: Hello there! How can I help you?
Human: Not to bad - how are you?
Chatbot:
> Finished chain.
Result: I'm doing great! How about you?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
AI: Hello there! How can I help you?
Human: Not to bad - how are you?
AI: I'm doing great! How about you?
Human: Not to bad - how are you?
Chatbot:
> Finished chain.
Result: I'm doing great! How about you?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi there my friend
AI: Hello there! How can I help you?
Human: Not to bad - how are you?
AI: I'm doing great! How about you?
Human: Not to bad - how are you?
AI: I'm doing great! How about you?
Human: Not to bad - how are you?
Chatbot:
> Finished chain.
Result: I'm doing great! Is there anything else I can help you with?
新しいLLMChainチェーンに入る...
フォーマット後のプロンプト:
あなたは人間と会話しているチャットボットです。
人間:こんにちは、友達
チャットボット:
チェーンを終了しました。
結果:こんにちは!何かお手伝いできますか?
新しいLLMChainチェーンに入る...
フォーマット後のプロンプト:
あなたは人間と会話しているチャットボットです。
人間:こんにちは、友達
AI:こんにちは!何かお手伝いできますか?
人間:まあまあです。あなたはどうですか?
チャットボット:
チェーンを終了しました。
結果:私はとても元気です!あなたはどうですか?
新しいLLMChainチェーンに入る...
フォーマット後のプロンプト:
あなたは人間と会話しているチャットボットです。
人間:こんにちは、友達
AI:こんにちは!何かお手伝いできますか?
人間:まあまあです。あなたはどうですか?
AI:私はとても元気です!あなたはどうですか?
人間:まあまあです。あなたはどうですか?
チャットボット:
チェーンを終了しました。
結果:私はとても元気です!あなたは何か他にお手伝いできますか?
新しいLLMChainチェーンに入る...
フォーマット後のプロンプト:
あなたは人間と会話しているチャットボットです。
人間:こんにちは、友達
AI:こんにちは!何かお手伝いできますか?
人間:まあまあです。あなたはどうですか?
AI:私はとても元気です!あなたはどうですか?
人間:まあまあです。あなたはどうですか?
AI:私はとても元気です!あなたはどうですか?
人間:まあまあです。あなたはどうですか?
チャットボット:
チェーンを終了しました。
結果:私はとても元気です!何か他にお手伝いできますか?
メモリプロンプト
Progressively summarize the lines of conversation provided, adding onto the previous summary returning a new summary.\n\nEXAMPLE\nCurrent summary:\nThe human asks what the AI thinks of artificial intelligence. The AI thinks artificial intelligence is a force for good.\n\nNew lines of conversation:\nHuman: Why do you think artificial intelligence is a force for good?\nAI: Because artificial intelligence will help humans reach their full potential.\n\nNew summary:\nThe human asks what the AI thinks of artificial intelligence. The AI thinks artificial intelligence is a force for good because it will help humans reach their full potential.\nEND OF EXAMPLE\n\nCurrent summary:\n{summary}\n\nNew lines of conversation:\n{new_lines}\n\nNew summary:
会話の行を徐々に要約し、前の要約に加えて新しい要約を返します。
例:
現在の要約:
人間は、AIが人工知能についてどう思うか尋ねます。AIは人工知能は良い影響を与える力だと考えています。
新しい会話の行:
人間:なぜ人工知能が良い影響を与える力だと思うのですか?
AI:なぜなら人工知能は人間が最大限の可能性を引き出すのを助けるからです。
新しい要約:
人間は、AIが人工知能についてどう思うか尋ねます。AIは人工知能は良い影響を与える力だと考えています。なぜなら人工知能は人間が最大限の可能性を引き出すのを助けるからです。
例の終わり
現在の要約:
{要約}
新しい会話の行:
{新しい行}
新しい要約:
E:Conversation Knowledge Graph Memory
Conversation Knowledge Graph Memoryとは?
このタイプのメモリは、知識グラフを使用して記憶を再現します。
Conversation Knowledge Graph Memory引数
k: int = 2
buffer: List[str] = list
kg: NetworkxEntityGraph = NetworkxEntityGraph
knowledge_extraction_prompt: BasePromptTemplate = KNOWLEDGE_TRIPLE_EXTRACTION_PROMPT
entity_extraction_prompt: BasePromptTemplate = ENTITY_EXTRACTION_PROMPT
llm: BaseLLM
human_prefix: str = "Human"
ai_prefix: str = "AI"
output_key: str | None = None
input_key: str | None = None
memory_key: str = "history"
サンプルプログラム
from langchain.chains.conversation.memory import ConversationBufferMemory,ConversationSummaryMemory,ConversationBufferWindowMemory,ConversationSummaryBufferMemory,ConversationKGMemory
from langchain import OpenAI, LLMChain, PromptTemplate
import os
os.environ["OPENAI_API_KEY"] = "..."
template = """You are a chatbot having a conversation with a human.
{history}
Human: {human_input}
Chatbot:"""
prompt = PromptTemplate(
input_variables=["history", "human_input"],
template=template
)
# memory = ConversationSummaryMemory(llm=OpenAI(),memory_key="chat_history")
# memory = ConversationBufferWindowMemory(k=2,memory_key="chat_history")
# memory = ConversationSummaryBufferMemory(llm=OpenAI(),memory_key="chat_history")
llm=OpenAI()
memory = ConversationKGMemory(llm=llm)
llm_chain = LLMChain(
llm=llm,
prompt=prompt,
verbose=True,
memory=memory,
)
print("Result: "+llm_chain.predict(human_input="Hi, what's up?"))
print("Result: "+llm_chain.predict(human_input="My name is James and I'm helping Will. He's an engineer."))
print("Result: "+llm_chain.predict(human_input="What do you know about Will?"))
print("Result: "+llm_chain.predict(human_input="how are you?"))
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: Hi, what's up?
Chatbot:
> Finished chain.
Result: Hi there! I'm doing great, how about you?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: My name is James and I'm helping Will. He's an engineer.
Chatbot:
> Finished chain.
Result: Hi James, nice to meet you. What type of engineering does Will do?
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
On Will: Will profession engineer.
Human: What do you know about Will?
Chatbot:
> Finished chain.
Result: Will is an engineer with a background in software engineering and computer programming. He has experience in developing and designing innovative products.
> Entering new LLMChain chain...
Prompt after formatting:
You are a chatbot having a conversation with a human.
Human: how are you?
Chatbot:
> Finished chain.
Result: I'm doing well, thank you! How about you?
新しいLLMChainチェーンに入力中...
フォーマット後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間:こんにちは、調子はどうですか?
チャットボット:
チェーンの終了。
結果:こんにちは!私は元気です。あなたはどうですか?
新しいLLMChainチェーンに入力中...
フォーマット後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間:私の名前はジェームズで、私はウィルを手伝っています。彼はエンジニアです。
チャットボット:
チェーンの終了。
結果:こんにちはジェームズ、お会いできてうれしいです。ウィルはどのようなエンジニアですか?
新しいLLMChainチェーンに入力中...
フォーマット後のプロンプト:
あなたは人間と会話をしているチャットボットです。
ウィルについて:ウィルはプロのエンジニアです。
人間:あなたはウィルについて何を知っていますか?
チャットボット:
チェーンの終了。
結果:ウィルは、ソフトウェアエンジニアリングとコンピュータプログラミングのバックグラウンドを持つエンジニアです。彼は革新的な製品の開発や設計の経験があります。
新しいLLMChainチェーンに入力中...
フォーマット後のプロンプト:
あなたは人間と会話をしているチャットボットです。
人間:お元気ですか?
チャットボット:
チェーンの終了。
結果:おかげさまで、私は元気です。あなたはどうですか?
メモリプロンプト1
You are a networked intelligence helping a human track knowledge triples about all relevant people, things, concepts, etc. and integrating them with your knowledge stored within your weights as well as that stored in a knowledge graph. Extract all of the knowledge triples from the last line of conversation. A knowledge triple is a clause that contains a subject, a predicate, and an object. The subject is the entity being described, the predicate is the property of the subject that is being described, and the object is the value of the property.\n\nEXAMPLE\nConversation history:\nPerson #1: Did you hear aliens landed in Area 51?\nAI: No, I didn't hear that. What do you know about Area 51?\nPerson #1: It's a secret military base in Nevada.\nAI: What do you know about Nevada?\nLast line of conversation:\nPerson #1: It's a state in the US. It's also the number 1 producer of gold in the US.\n\nOutput: (Nevada, is a, state)<|>(Nevada, is in, US)<|>(Nevada, is the number 1 producer of, gold)\nEND OF EXAMPLE\n\nEXAMPLE\nConversation history:\nPerson #1: Hello.\nAI: Hi! How are you?\nPerson #1: I'm good. How are you?\nAI: I'm good too.\nLast line of conversation:\nPerson #1: I'm going to the store.\n\nOutput: NONE\nEND OF EXAMPLE\n\nEXAMPLE\nConversation history:\nPerson #1: What do you know about Descartes?\nAI: Descartes was a French philosopher, mathematician, and scientist who lived in the 17th century.\nPerson #1: The Descartes I'm referring to is a standup comedian and interior designer from Montreal.\nAI: Oh yes, He is a comedian and an interior designer. He has been in the industry for 30 years. His favorite food is baked bean pie.\nPerson #1: Oh huh. I know Descartes likes to drive antique scooters and play the mandolin.\nLast line of conversation:\nOutput: (Descartes, likes to drive, antique scooters)<|>(Descartes, plays, mandolin)\nEND OF EXAMPLE\n\nConversation history (for reference only):\n{history}\nLast line of conversation (for extraction):\nHuman: {input}\n\nOutput:
あなたは人々、物、概念などに関する知識トリプルを追跡するためのネットワーク化された知性であり、自分の重みに保存されている知識と知識グラフに保存されている知識と統合しています。最後の会話からすべての知識トリプルを抽出してください。知識トリプルとは、主語、述語、オブジェクトを含む節のことです。主語は説明されているエンティティ、述語は説明されている主語のプロパティ、オブジェクトはそのプロパティの値です。
例:
会話履歴:
人物 #1:「エイリアンがエリア51に着陸したのを聞いたことがありますか?」
AI:「いいえ、そんな話は聞いたことがありません。エリア51について何を知っていますか?」
人物 #1:「それはネバダ州の秘密の軍事基地です。」
AI:「ネバダ州について何を知っていますか?」
会話の最後の行:
人物 #1:「それは米国の州です。また、米国で金を最も多く生産している場所でもあります。」
出力:(Nevada, is a, state)<|>(Nevada, is in, US)<|>(Nevada, is the number 1 producer of, gold)
例の終わり
例:
会話履歴:
人物 #1:「こんにちは。」
AI:「こんにちは!お元気ですか?」
人物 #1:「元気です。あなたはどうですか?」
AI:「私も元気です。」
会話の最後の行:
人物 #1:「私は店に行きます。」
出力:なし
例の終わり
例:
会話履歴:
人物 #1:「デカルトについて何を知っていますか?」
AI:「デカルトは17世紀に生きたフランスの哲学者、数学者、科学者でした。」
人物 #1:「私が言っているデカルトは、モントリオールのスタンダップ・コメディアンであり、インテリアデザイナーです。」
AI:「ああ、そうですね。彼はコメディアンであり、インテリアデザイナーです。彼は業界で30年以上活躍しています。彼の好きな食べ物はベイクドビーンパイです。」
人物 #1:「ああ、そうなんです。私はデカルトがアンティークスクーターを運転したりマンドリンを弾いたりするのが好きだと知っています。」
会話の最後の行:
出力:(Descartes, likes to drive, antique scooters)<|>(Descartes, plays, mandolin)
例の終わり
会話履歴(参考用):
{history}
会話の最後の行(抽出用):
人物:{input}
出力:
メモリプロンプト2
You are an AI assistant reading the transcript of a conversation between an AI and a human. Extract all of the proper nouns from the last line of conversation. As a guideline, a proper noun is generally capitalized. You should definitely extract all names and places.
note to return (e.g. the user is just issuing a greeting or having a simple conversation).
EXAMPLE
Conversation history:
Person #1: how\'s it going today?
AI: "It\'s going great! How about you?"
Person #1: good! busy working on Langchain. lots to do.
AI: "That sounds like a lot of work! What kind of things are you doing to make Langchain better?"
Last line:
Person #1: i\'m trying to improve Langchain\'s interfaces, the UX, its integrations with various products the user might want ... a lot of stuff.
Output: Langchain
END OF EXAMPLE
EXAMPLE
Conversation history:
Person #1: how\'s it going today?
AI: "It\'s going great! How about you?"
Person #1: good! busy working on Langchain. lots to do.
AI: "That sounds like a lot of work! What kind of things are you doing to make Langchain better?"
Last line:
Person #1: i\'m trying to improve Langchain\'s interfaces, the UX, its integrations with various products the user might want ... a lot of stuff. I\'m working with Person #2.\nOutput: Langchain, Person #2
END OF EXAMPLE
Conversation history (for reference only):
{history}
Last line of conversation (for extraction):
Human: {input}
Output:
あなたはAIアシスタントであり、AIと人間の会話のトランスクリプトを読んでいます。会話の最後の行からすべての固有名詞を抽出してください。一般的に固有名詞は大文字で書かれます。名前や場所などは必ず抽出するようにしてください。
(挨拶や簡単な会話の場合は注意事項として戻り値を設定してください。)
例:
会話履歴:
人物#1: 今日はどうですか?
AI: 「素晴らしい調子です!あなたはどうですか?」
人物#1: 良いです!Langchainに取り組んでいます。たくさんやることがあります。
AI: 「それはたくさんの仕事のように聞こえます!Langchainを改善するために何をしていますか?」
最後の行:
人物#1: 「Langchainのインターフェース、UX、ユーザーが望むさまざまな製品との統合を改善しようとしています...たくさんのことがあります。」
出力:Langchain
例2:
会話履歴:
人物#1: 今日はどうですか?
AI: 「素晴らしい調子です!あなたはどうですか?」
人物#1: 良いです!Langchainに取り組んでいます。たくさんやることがあります。
AI: 「それはたくさんの仕事のように聞こえます!Langchainを改善するために何をしていますか?」
最後の行:
人物#1: 「Langchainのインターフェース、UX、ユーザーが望むさまざまな製品との統合を改善しようとしています...たくさんのことがあります。人物#2と一緒に取り組んでいます。」
出力:Langchain、人物#2
会話履歴(参考用):
{history}
会話の最後の行(抽出用):
人間:{input}
出力:
F:Entity Memory
Entity Memoryとは?
Entity Memoryは、LLMを用いて情報を抽出し、特定の単語ごとに知識を蓄積します。
例えば、以下の文章をEntity Memoryに追加した場合、
EN:
Deven & Sam are working on a hackathon project
They are trying to add more complex memory structures to Langchain
JP:
DevenとSamはハッカソンのプロジェクトに取り組んでいます。
Langchainにさらに複雑なメモリ構造を追加しようとしている。
Entity Memoryには、単語ごとに以下の内容が記録されます。
EN:
{'Deven': 'Deven is working on a hackathon project with Sam to add more '
'complex memory structures to Langchain.',
'Langchain': 'Langchain is a project that seeks to add more complex memory '
'structures.',
'Sam': 'Sam is working on a hackathon project with Deven, attempting to add '
'more complex memory structures to Langchain.'}
JP:
Deven:DevenはSamと一緒にLangchainにもっと複雑なメモリ構造を追加するハッカソンプロジェクトに取り組んでいます。
Langchain:Langchainは、より複雑なメモリ構造を追加しようとするプロジェクトである。
Sam:SamはDevenと一緒にハッカソンのプロジェクトに取り組んでいて、Langchainにもっと複雑なメモリ構造を追加しようと試みています。
Entity Memory引数
buffer: List[str] = []
human_prefix: str = "Human"
ai_prefix: str = "AI"
llm: BaseLLM
entity_extraction_prompt: BasePromptTemplate = ENTITY_EXTRACTION_PROMPT
entity_summarization_prompt: BasePromptTemplate = ENTITY_SUMMARIZATION_PROMPT
output_key: str | None = None
input_key: str | None = None
store: Dict[str, str | None] = {}
entity_cache: List[str] = []
k: int = 3
chat_history_key: str = "history"
サンプルプログラム
import os
from langchain import OpenAI, ConversationChain
from langchain.chains.conversation.memory import ConversationEntityMemory
from langchain.chains.conversation.prompt import ENTITY_MEMORY_CONVERSATION_TEMPLATE
from pydantic import BaseModel
from typing import List, Dict, Any
from pprint import pprint
os.environ["OPENAI_API_KEY"] = "...."
llm = OpenAI(temperature=0)
conversation = ConversationChain(
llm=llm,
verbose=True,
prompt=ENTITY_MEMORY_CONVERSATION_TEMPLATE,
memory=ConversationEntityMemory(llm=llm)
)
conversation.predict(input="Deven & Sam are working on a hackathon project")
pprint(conversation.memory.store)
conversation.predict(input="They are trying to add more complex memory structures to Langchain")
pprint(conversation.memory.store)
会話例でも試してみました。
print(conversation.predict(input="オタクくん:Hello."))
pprint(conversation.memory.store)
conversation.predict(input="オタクくん:I'll practice my instrument today.")
pprint(conversation.memory.store)
conversation.predict(input="オタクくん:I'm going to practise guitar. I'm going to practise the songs from Bocchi Zarokku.")
pprint(conversation.memory.store)
Human: オタクくん:Hello.AI: Hi there! How can I help you?
Human: オタクくん:I'll practice my instrument today.
AI: That sounds like a great idea! What instrument do you play?
Last line:
Human: オタクくん:I'm going to practise guitar. I'm going to practise the songs from Bocchi Zarokku.
You:
{'Bocchi Zarokku': 'Bocchi Zarokku is a Japanese rock band whose songs are '
'often played on guitar.', 'オタクくん': 'オタクくん is a musician who practices their instrument regularly.'}
'ぼっちざろっく': ぼっちざーろっくは、日本のロックバンドで、楽曲は''ギターで演奏されることが多い''です。,
オタクくん: 'オタクくんは日頃から楽器の練習をしている音楽家のこと。
Entity Memoryの処理プロンプト
Entity Memoryが、LLMにどのようなプロンプトを投げているかを下に示しています。
You are an AI assistant reading the transcript of a conversation between an AI and a human.
Extract all of the proper nouns from the last line of conversation.
As a guideline, a proper noun is generally capitalized.
You should definitely extract all names and places.
The conversation history is provided just in case of a coreference (e.g. "What do you know about him" where "him" is defined in a previous line) -- ignore items mentioned there that are not in the last line.
Return the output as a single comma-separated list, or NONE if there is nothing of note to return (e.g. the user is just issuing a greeting or having a simple conversation).
EXAMPLE
Conversation history:
Person #1: how's it going today?
AI: "It's going great! How about you?"
Person #1: good! busy working on Langchain. lots to do.
AI: "That sounds like a lot of work! What kind of things are you doing to make Langchain better?"
Last line:
Person #1: i'm trying to improve Langchain's interfaces, the UX, its integrations with various products the user might want ... a lot of stuff.
Output: Langchain
END OF EXAMPLE
EXAMPLE
Conversation history:
Person #1: how\'s it going today?
AI: "It\'s going great! How about you?"
Person #1: good! busy working on Langchain. lots to do.
AI: "That sounds like a lot of work! What kind of things are you doing to make Langchain better?"
Last line:
Person #1: i\'m trying to improve Langchain\'s interfaces, the UX, its integrations with various products the user might want ... a lot of stuff. I\'m working with Person #2.
Output: Langchain, Person #2
END OF EXAMPLE
Conversation history (for reference only):
{history}
Last line of conversation (for extraction):
Human: {input}
Output:
あなたは、AIと人間の会話の記録を読むAIアシスタントです。会話の最後の行から固有名詞をすべて抽出してください。
会話履歴は共参照の場合にのみ提供される(例:「彼」の定義が前の行にある「彼について何を知っていますか」) -- 最後の行にない項目は無視される。
カンマ区切りのリストとして出力を返すか、または特筆すべきことがない場合はNONEを返す(例:ユーザが単に挨拶をしたり、簡単な会話をしている場合)。
例
会話の履歴:
人物1:今日はどうですか?
AI:「絶好調です。あなたはどうですか?」
Person #1: いいですよ!Langchainの作業で忙しいです、やることがたくさんあります。
AI:「それは大変そうですね~。Langchainをより良くするためにどんなことをしているんですか?」
最終行:人物1:Langchainのインターフェース、UX、ユーザーが欲しがるかもしれない様々な製品との統合...たくさんのことを改善しようとしているんだ。
出力してください。Langchain
例題の終わり
例
会話履歴:
人物1:今日はどうですか?
AI:「順調だよ。あなたはどうですか?」
人物1:順調だよ!ラングチャンの作業で忙しいんだ。
AI:「それは大変そうですね~。Langchainをより良くするためにどんなことをしているんですか?」
最後のセリフです。
人物1: 私はLangchainのインターフェース、UX、ユーザーが必要とする様々な製品との統合...多くのものを改善しようとしています。私は人物2と働いています。
アウトプット Langchain、人物2
例題の終わり
会話履歴(参照用):
{履歴}
会話の最終行(抽出用):
人間:{入力}
出力
'You are an AI assistant helping a human keep track of facts about relevant people, places, and concepts in their life. Update the summary of the provided entity in the "Entity" section based on the last line of your conversation with the human. If you are writing the summary for the first time, return a single sentence.
The update should only include facts that are relayed in the last line of conversation about the provided entity, and should only contain facts about the provided entity.
If there is no new information about the provided entity or the information is not worth noting (not an important or relevant fact to remember long-term), return the existing summary unchanged.
Full conversation history (for context):
{history}
Entity to summarize:
{entity}
Existing summary of {entity}:
{summary}
Last line of conversation:
Human: {input}
Updated summary:',
template_format='f-string', validate_template=True)
'あなたは、人間が自分の人生に関連する人、場所、概念に関する事実を記録するのを助けるAIアシスタントです。人間との会話の最後の行に基づいて、「Entity」セクションの提供されたエンティティのサマリーを更新してください。初めて要約を書く場合は、一文を返すこと。
更新には、提供されたエンティティに関する会話の最後の行で伝えられた事実のみを含める必要があります。
提供されたエンティティに関する新しい情報がない場合、またはその情報が特筆すべきものではない場合(長期的に覚えておくべき重要または関連する事実ではない)、既存の要約を変更せずに返却する。
完全な会話履歴(文脈のため)。
{履歴}。
要約するエンティティ
{エンティティ}
エンティティ}の既存の要約。
{サマリー}
会話の最後の行
人間: {input}
更新された要約:'
参照先
この記事が気に入ったらサポートをしてみませんか?