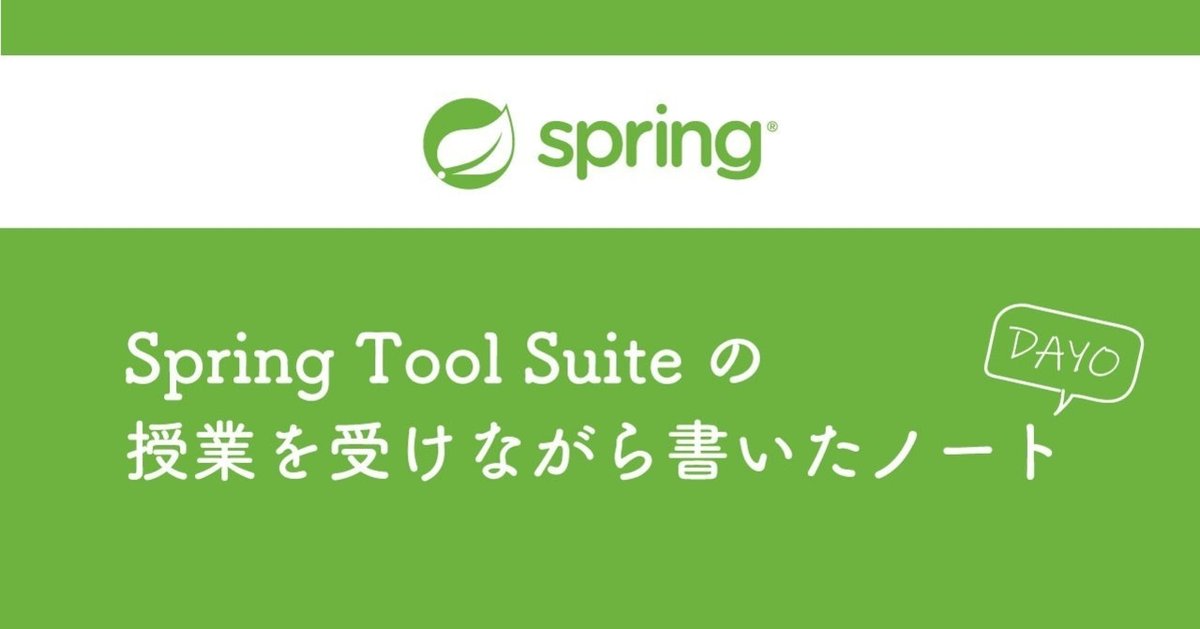
Springフレームワーク05:GETとPOSTとデータベース連携のキソ
ぜんぜんまとまってないので、後から修正しま…
【エントリーポイント】
STSのエントリーポイント(メインメソッドがある)は
SpringSampleApplication.java
※ これは自動で作られる
【HelloController.java】
全体のソースコード
package com.example.demo.trySpring;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
public class HelloController {
@Autowired
private HelloService helloService;
@GetMapping("/hello")
public String getHello() {
// hello.htmlに画面遷移
return "hello";
}
@PostMapping("/hello")
public String postRequest(@RequestParam("text1") String str, Model model) {
// 画面から受け取った文字列をModelに登録
model.addAttribute("sample", str);
// helloResponse.htmlに画面遷移
return "helloResponse";
}
@PostMapping("/hello/db")
public String postDbRequest(@RequestParam("text2") String str, Model model) {
// Stringからint型に変換
int id = Integer.parseInt(str);
// 1件検索
Employee employee = helloService.findOne(id);
// 検索結果をModelに登録
model.addAttribute("id", employee.getEmployeeId());
model.addAttribute("name", employee.getEmployeeName());
model.addAttribute("age", employee.getAge());
// helloResponseDB.htmlに画面遷移
return "helloResponseDB";
}
}
解説↓
【GET】
コントローラ<HelloController.java>にて
最初のGETリクエストを受けるメソッド名
get〇〇()
〇〇はユーザーによる定義でOK
@GetMapping("/hello") ←URLマッピング
http://localhost:8080/URLマッピング
<HelloController.java>
@GetMapping("/hello")
public String getHello() {
// hello.htmlに画面遷移(フォワードみたいな感じ)
return "hello"; // ←hello.htmlを省略して書いている
}
【POST】
hello.htmlはsrc/main/resources/templatesに置く
※ th:はThymleafを使う際のプレフィックス
<hello.html>
全体のソースコード
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"></meta>
<title>Hello World</title>
</head>
<body>
<h1>Hello World</h1>
<form method="post" action="/hello">
<!-- ポイント:th:value -->
好きな文字を入力:<input type="text" name="text1" th:value="${text1_value}" />
<input type="submit" value="クリック" />
</form>
<br />
<form method="post" action="/hello/db">
従業員IDを入力:<input type="text" name="text2" th:value="${text2_value}" />
<input type="submit" value="クリック" />
</form>
<body>
</html>
コントローラ(HelloController.java)にて
最初のPOSTリクエストを受けるメソッド名
postRequest(name属性に入力された文字列,モデル)
<HelloController.java>
@PostMapping("/hello")
public String postRequest(@RequestParam("text1") String str, Model model) {
// 画面から受け取った文字列をModelに登録
model.addAttribute("sample", str);
// helloResponse.htmlに画面遷移
return "helloResponse";
}
【データベースを使用】
hello.htmlで入力される
<hello.html>該当箇所
・
・
<form method="post" action="/hello/db">
従業員IDを入力:<input type="text" name="text2" th:value="${text2_value}" />
<input type="submit" value="クリック" />
</form>
・
・
src/main/resouces/application.properties
という設定ファイルを作る
<application.properties>
spring.datasource.url=jdbc:h2:mem:testdb;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE
spring.datasource.driver-class-name=org.h2.Driver
spring.datasouce.username=sa
spring.datasouce.password=
spring.datasource.sql-script-encoding=UTF-8
spring.h2.console.enabled=true
spring.datasource.initialization-mode=always
spring.datasource.schema=classpath:schema.sql
spring.datasource.data=classpath:data.sql
※ schema.sqlとdata.sqlはSpringBoot起動時に実行されるので、毎度テーブルとレコードが作られる
※ H2Databaseはメモリ内にできるのでSTSを終了するたびにリセットされる
→テストが簡単になる
<HelloRepository.java>
HelloRepository.javaはサーブレットにおけるDAOに該当する
HelloService.javaはサーブレットにおけるLogicモデルのようなもの
@Repository
public class HelloRepository {
@Autowired //インスタンス化のような機能
private JdbcTemplate jdbcTemplate;
public Map<String, Object> findOne(int id) {
// SELECT文
String query = "SELECT "
+ " employee_id,"
+ " employee_name,"
+ " age "
+ "FROM employee "
+ "WHERE employee_id=?";
// 検索実行
Map<String, Object> employee = jdbcTemplate.queryForMap(query, id);
return employee; // Map形式で情報が詰め込まれる
}
}
<HelloService.java>
package com.example.demo.trySpring;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class HelloService {
@Autowired
private HelloRepository helloRepository;
public Employee findOne(int id) {
// 1件検索実行
Map<String, Object> map = helloRepository.findOne(id);
// Mapから値を取得
int employeeId = (Integer) map.get("employee_id");
String employeeName = (String) map.get("employee_name");
int age = (Integer) map.get("age");
// Employeeクラスに値をセット
Employee employee = new Employee();
employee.setEmployeeId(employeeId);
employee.setEmployeeName(employeeName);
employee.setAge(age);
return employee;
}
}
※ employeeはSTS上ではドメインと呼ぶ
リポジトリとサービス間で渡すデータのこと
(モデルクラスとも呼ぶ)
<Employee.java>
package com.example.demo.trySpring;
import lombok.Data;
@Data //GetterとSetterを内部的に自動生成
public class Employee {
private int employeeId; //従業員ID
private String employeeName; //従業員名
private int age; //年齢
}
→コントローラ(HelloController.java)に戻る
<HelloController.java>該当箇所
@PostMapping("/hello/db")
public String postDbRequest(@RequestParam("text2") String str, Model model) {
// Stringからint型に変換
int id = Integer.parseInt(str);
// 1件検索
Employee employee = helloService.findOne(id);
// 検索結果をModelに登録
model.addAttribute("id", employee.getEmployeeId());
model.addAttribute("name", employee.getEmployeeName());
model.addAttribute("age", employee.getAge());
// helloResponseDB.htmlに画面遷移
return "helloResponseDB";
}
→ビュー(HelloResponse.html)に渡す
◆デバッガ使うよ!
・HelloRepository.javaの String query = "SELECT "メソッド内の先頭行にブレイクポイントをはる
・HelloService.javaのint employeeId = (Integer) map.get("employee_id");Mapに入ってきた内容を見たいので、次の行にはる
・プロジェクト名を右クリック > デバッグ > Spring Bootアプリケーションで実行
もしも気に入ったら、サポートお願いします! おやつを買いますので、餌付けができます。