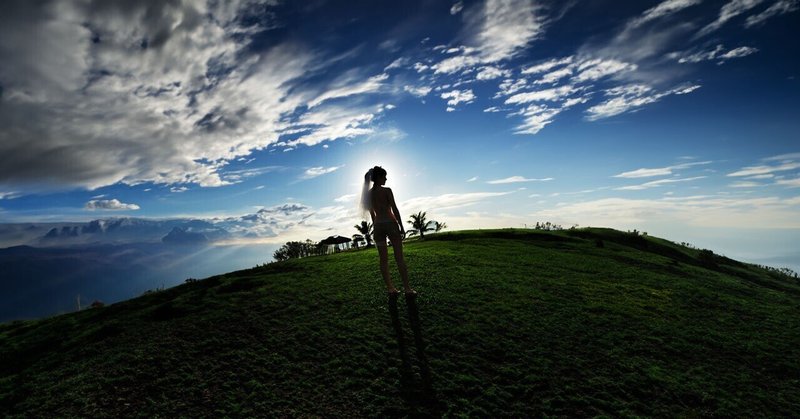
一行で note のマガジン内記事一覧を取得 ~ MATLAB で note 非公式 API を使う
まえがき
note のマガジン、デフォルトで表示されるのは14記事だけで、全記事を表示するには「もっとみる」をクリックする必要があります。
RSS があるのですが、無料ユーザーで25本、Pro 版で50本までしか配信されない仕様になっています。
つまり、マガジン内の全記事情報を外部から簡単に取ることができません。
また、「カード」表示だと書き出し部分が1センテンスしか含まれません。
「リスト」が一番表示文字数が多いのですが、タイトル画像も大きく場所を取り過ぎます。
レイアウトは3種類から選択できますが自由度が低く、それぞれ書き出し部分の制限があります。
もう少し自由にできないかと言うことで、note の API を使って MATLAB で情報を取得し、自分で html にフォーマッティングしてみます。
xml にした方が汎用性があるのかもしれませんが、その辺はよく知らないのでとりあえず、MATLAB で note の API を使って簡単に情報取得する例としてやってみました。
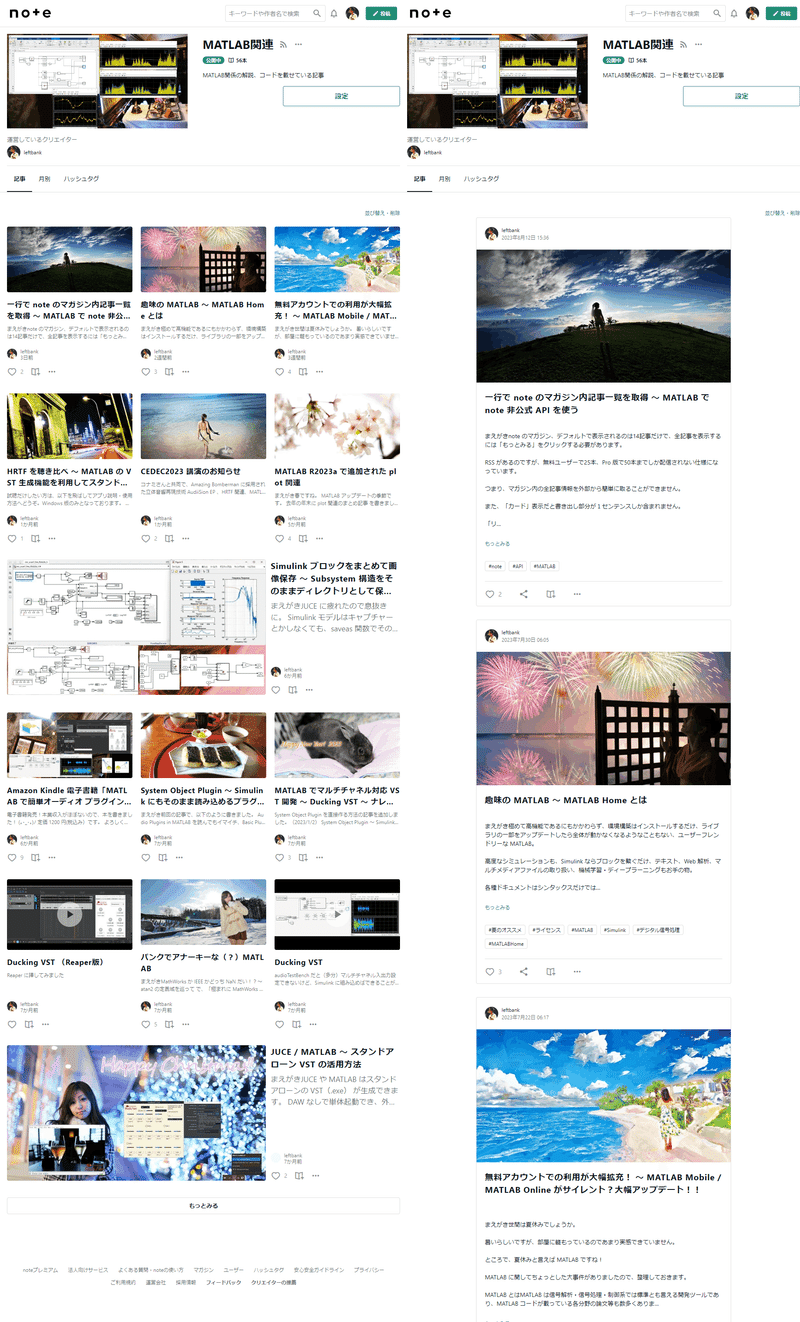
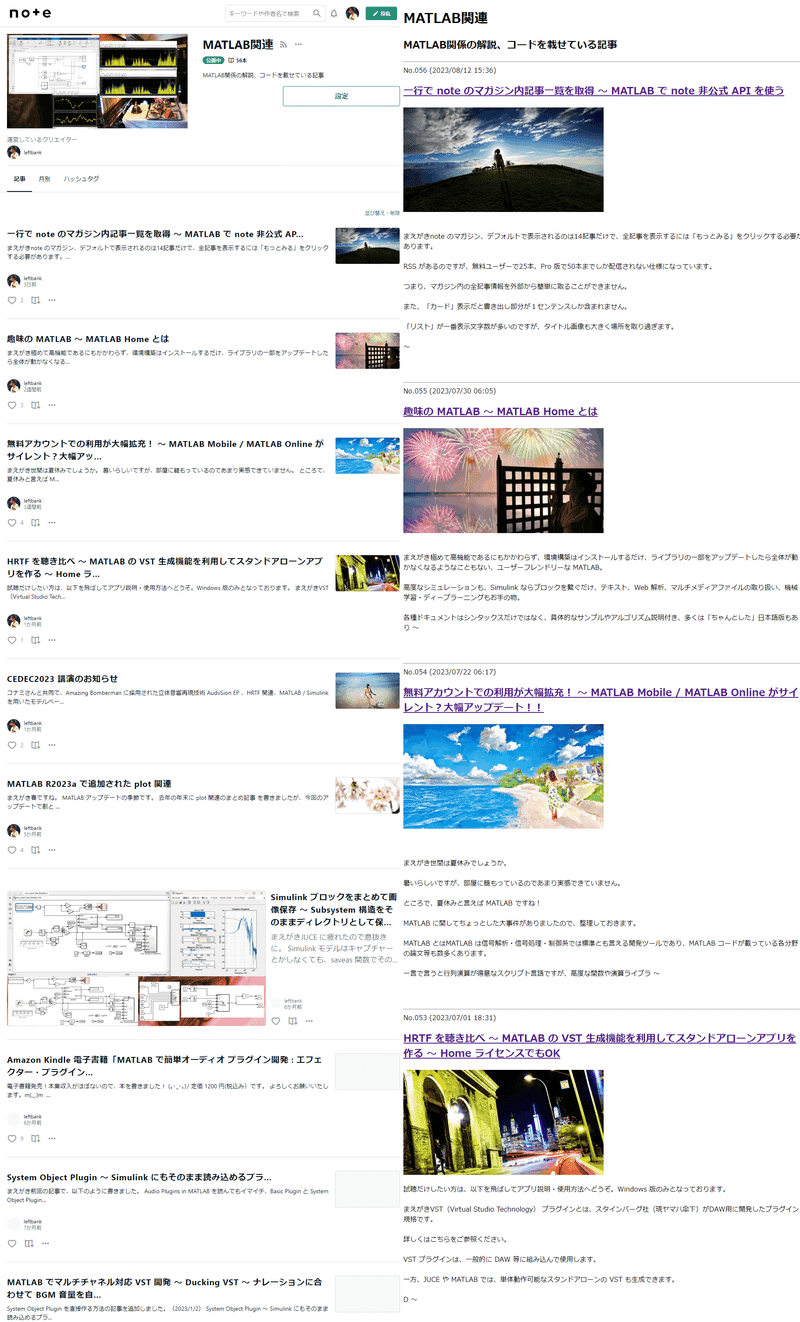
無料アカウントで使える MATLAB Online (basic)、MATLAB Mobile でも動作します。
note API
「非公式」で、v1/v2/v3があるようです。
今回、v1で必要な情報は取れたのでそれを使います。
「非公式」なので、突然なくなるかもしれませんが・・。
v1:https://note.com/api/v1
v2:https://note.com/api/v2
v3:https://note.com/api/v3
https://note.com/api/v1/layout/magazine/[マガジンKey]/
でマガジントップの情報が、
https://note.com/api/v1/layout/magazine/m088894fecb57/section?page=[ページ番号]
で各ページの情報が取れます。
MATLAB webread 関数
MATLAB の標準関数で、Web サービスからコンテンツを読み取ります。
詳細は公式ドキュメントをご覧ください。
様々な Web サービスに対応しています。
内容をパースして構造体にして返してくれます。
note の API であれば、一行で例えば以下のような情報が取れます。
-マガジントップ
code = webread("https://note.mu/api/v1/layout/magazine/m088894fecb57/"); % m088894fecb57 はマガジン Key
code.data.magazine_layout.
name:マガジンタイトル
description:マガジン説明
note_count:総記事数 各ページにも総記事数情報があります
archive_note_counters:年/月毎の記事数
category_note_counters:タグ毎の記事数
-各ページ
code = webread("https://note.com/api/v1/layout/magazine/m088894fecb57/section?page=1");
code.data.section.is_last_page:続きがある場合は0、最後のページは1になります
code.data.section.total_count:記事本数
code.data.section.contents.
key:記事キー https://note.com/[ユーザー名]/n/[記事 Key] が各記事の URL になります
type:Text/Audio/Movie 等の記事種類
name:タイトル
body:Text 記事冒頭 240文字
description:Audio/Movie 記事の内容説明
eyecatch:タイトル画像 URL
publish_at:公開日時
thumbnail_external_url:Movieタイプ記事のサムネイル URL
取れる記事内容は240文字です。
それ以上欲しい場合は、
code = webread('[記事 URL]');
str = extractHTMLText(code);
などとして取り出し、お好きに処理してください。
また、古いページは返ってくるフォーマットが違っていて、もう一段下に各記事毎に分かれています。次に示すスクリプトでは、その場合は構造体を組替える処理を入れてあります。
スクリプト
clear
note_Top = "https://note.com/leftbank/"; % top page of your note
API_URL = "https://note.mu/api/v1/layout/magazine/"; % API URL
mag_MATLAB_KEY = "m088894fecb57/";
mag_URL = API_URL + mag_MATLAB_KEY;
mag_Sec = mag_URL + "section?page=";
codeMag = webread(mag_URL); % read the magazine top
noteN = codeMag.data.magazine_layout.note_count; % total number of articles in the magazine
fid = fopen('noteMag_MATLAB.html','w'); % output html file name
% html header
fprintf(fid,"<!DOCTYPE html>\n");
fprintf(fid,"<html>\n");
fprintf(fid,"<head>\n");
fprintf(fid," <meta charset=""utf-8"">\n");
fprintf(fid," <title>%s</title>\n",codeMag.data.magazine_layout.name);
fprintf(fid,"</head>\n");
fprintf(fid,"<body>\n");
fprintf(fid,"<div><h1>%s</h1></div>\n",codeMag.data.magazine_layout.name); % magazine title
fprintf(fid,"<div><h2>%s</h2></div>\n",codeMag.data.magazine_layout.description); % magazine description
fprintf(fid,"<hr>\n");
pg = 1;
articleCount = 0;
while 1==1
url = mag_Sec + num2str(pg);
code = webread(url); % read each page
articleN = size(code.data.section.contents,1); % article number of the page
oldNewFlag = isfield(code.data.section.contents,'key'); % if haven't fields on top then old format page
if oldNewFlag % new format page
keys = {code.data.section.contents.key};
titles = {code.data.section.contents.name};
descriptions = {code.data.section.contents.description}; % for MovieNote/SounNote
forewords = {code.data.section.contents.body};
titleImages = {code.data.section.contents.eyecatch};
thumbnails = {code.data.section.contents.thumbnail_external_url}; % for MovieNote
pub = {code.data.section.contents.publish_at};
else % old format page
c = code.data.section.contents{1,1};
for k = 2:articleN
c0 = code.data.section.contents{k,1};
if isfield(c0,'audio')
c0 = rmfield(c0,'audio');
end
c = [c, c0];
end
keys = {c.key};
titles = {c.name};
descriptions = {c.description};
forewords = {c.body};
titleImages = {c.eyecatch};
thumbnails = {c.thumbnail_external_url};
pub = {c.publish_at};
end
for k = 1:articleN
% pubDate = split(pub{k});
% pubDate = pubDate{1}; % Date only
pubDate = pub{k}; % Date Time
if isempty(forewords{k}) % MovieNote
forewords{k} = descriptions{k};
titleImages{k} = thumbnails{k};
end
fprintf(fid,"<div>No.%03d (%s)<br></div>", noteN - articleCount, pubDate); % article No. (Date Time)
fprintf(fid,"<div><h2>");
fprintf(fid,"<a href=""%s"">", note_Top + "n/" + keys{k}); % article link
fprintf(fid,"%s</h2>\n", titles{k}); % title
if ~isempty(titleImages{k}) % except for SoundNote
fprintf(fid,"<p><img src=""%s"" width=""50%%""></p></a>\n", titleImages{k}); % title image
end
forW = forewords{k};
forW = strrep(forW,newline,"<br>"); % replace LF to <br>
fprintf(fid,"<p>%s ~<br></p>\n", forW); % foreword
fprintf(fid,"</div><br><br>\n\n");
fprintf(fid,"<hr>\n");
articleCount = articleCount + 1;
end
if (code.data.section.is_last_page == 1)
break
end
pg = pg + 1;
end
fprintf(fid,"</body>\n");
fprintf(fid,"</html>\n");
fclose(fid);
最初の方の URL や Key は適宜入れ替えてください。
デバッグ時は、出力される html を確認しながらの方が便利なので、fprintf を fid なしで画面表示し、
publish('[スクリプトファイル名.m]');
web('[スクリプトファイル名.html]')
とする方法もお勧めです。
それと、MATLAB Online でも MATLAB Mobile でも動作はしますが、MATLAB Online で生成した html ダブルクリックすると、なぜか画像が note から接続拒否されて?表示されないようです。
また、MATLAB Mobile でもそのまま開くことはできないようなので、いずれの場合も DL してからお使いください。
生成例
上記スクリプトで生成した html ファイルをここに置いてあります。
自動更新できるわけでもないのでアレですが、Web 系は疎いので・・。
う~ん、どなたか、もっと面白い使い方をお願いします。(;¬_¬)
The title image was created using Adobe Generative Fill based on this picture.
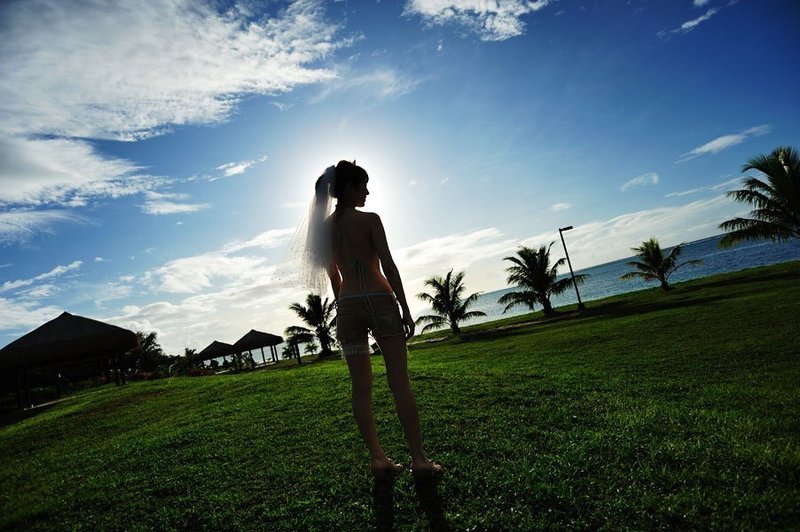
この記事が気に入ったらサポートをしてみませんか?