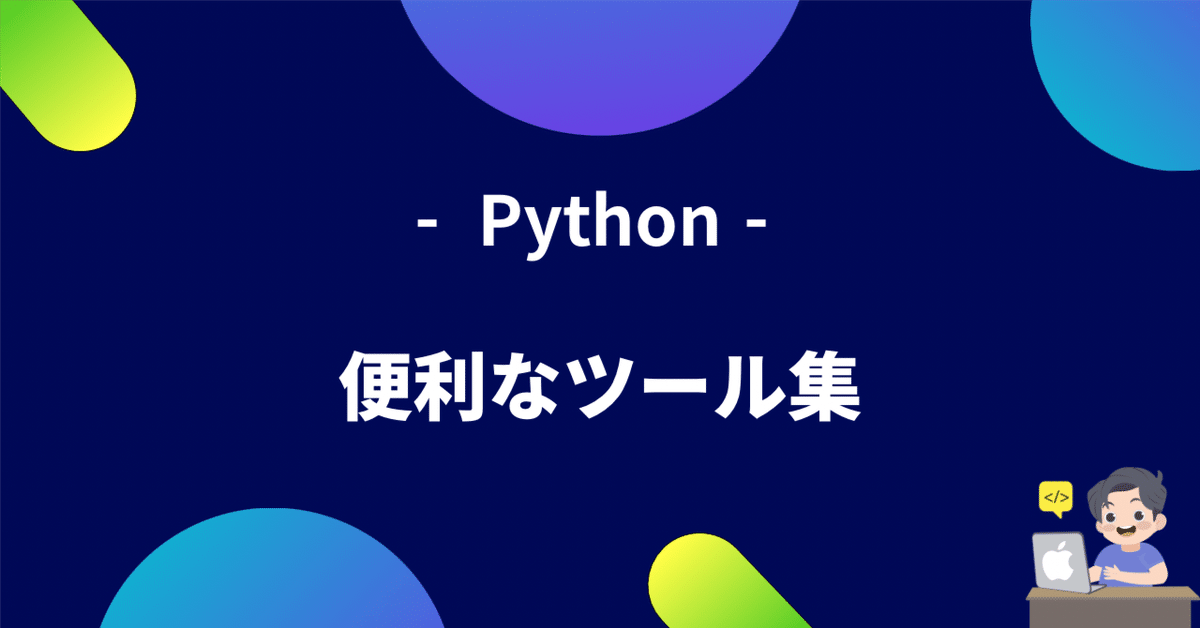
【Python】便利なツール集
Pythonには多くの便利なツールやライブラリがあります。ここでは、様々な用途に役立つツールやライブラリをカテゴリー別に紹介します。
1. データ処理・分析
Pandas: データ解析のための強力なライブラリ。データフレームを使って効率的にデータを操作できます。
import pandas as pd
df = pd.read_csv('data.csv')
df.head()
NumPy: 数値計算のためのライブラリ。多次元配列の操作が簡単に行えます。
import numpy as np
arr = np.array([1, 2, 3, 4])
print(arr)
SciPy: 数値計算、統計、信号処理など、科学技術計算のためのライブラリ。
from scipy import stats
data = [1, 2, 3, 4, 5]
print(stats.mean(data))
2. 機械学習・人工知能
Scikit-learn: 機械学習のためのライブラリ。分類、回帰、クラスタリングなどのアルゴリズムが豊富に揃っています。
from sklearn import datasets, model_selection, svm
digits = datasets.load_digits()
X_train, X_test, y_train, y_test = model_selection.train_test_split(digits.data, digits.target, test_size=0.2)
clf = svm.SVC()
clf.fit(X_train, y_train)
print(clf.score(X_test, y_test))
TensorFlow: Googleが開発したオープンソースの機械学習ライブラリ。ディープラーニングモデルの構築に使用されます。
import tensorflow as tf
model = tf.keras.models.Sequential([tf.keras.layers.Dense(128, activation='relu'), tf.keras.layers.Dense(10, activation='softmax')])
Keras: TensorFlowの高レベルAPI。ディープラーニングモデルを簡単に構築できます。
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([Dense(128, activation='relu'), Dense(10, activation='softmax')])
3. データビジュアライゼーション
Matplotlib: 2Dプロット作成のためのライブラリ。グラフやチャートを描画できます。
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
Seaborn: Matplotlibを基にした統計データ可視化ライブラリ。美しいグラフが簡単に描けます。
import seaborn as sns
sns.set(style="darkgrid")
tips = sns.load_dataset("tips")
sns.scatterplot(x="total_bill", y="tip", data=tips)
Plotly: インタラクティブなグラフを作成できるライブラリ。ウェブベースの可視化が可能です。
import seaborn as sns
sns.set(style="darkgrid")
tips = sns.load_dataset("tips")
sns.scatterplot(x="total_bill", y="tip", data=tips)
4. Web開発
Django: 高機能なWebフレームワーク。迅速な開発とクリーンなデザインを支援します。
django-admin startproject myproject
Flask: 軽量なWebフレームワーク。シンプルなアプリケーションに最適です。
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello():
return "Hello, World!"
if __name__ == '__main__':
app.run()
FastAPI: 高速なAPIを簡単に作成できるモダンなWebフレームワーク。
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def read_root():
return {"Hello": "World"}
5. 自然言語処理 (NLP)
NLTK: 自然言語処理のためのライブラリ。テキスト解析、トークン化、タグ付けなどに使用できます。
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
text = "Hello, how are you?"
print(word_tokenize(text))
spaCy: 高速で効率的な自然言語処理ライブラリ。エンティティ認識や文解析に強い。
import spacy
nlp = spacy.load("en_core_web_sm")
doc = nlp("Apple is looking at buying U.K. startup for $1 billion")
for ent in doc.ents:
print(ent.text, ent.label_)
TextBlob: テキストデータの処理と解析を簡単に行えるライブラリ。感情分析などに使用。
from textblob import TextBlob
text = "I love programming."
blob = TextBlob(text)
print(blob.sentiment)
6. 画像処理
Pillow: Python Imaging Libraryのフォーク。画像の操作や処理が簡単に行えます。
from PIL import Image
img = Image.open("example.jpg")
img = img.rotate(45)
img.save("rotated_example.jpg")
OpenCV: 画像処理とコンピュータビジョンのためのライブラリ。リアルタイム画像処理に強い。
import cv2
img = cv2.imread('example.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
cv2.imshow('Gray Image', gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
7. 自動化
Selenium: ウェブブラウザを操作するためのライブラリ。ウェブサイトの自動テストに使用されます。
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.example.com")
print(driver.title)
driver.quit()
BeautifulSoup: HTMLやXMLの解析ライブラリ。ウェブスクレイピングに使用されます。
from bs4 import BeautifulSoup
import requests
response = requests.get("https://www.example.com")
soup = BeautifulSoup(response.content, "html.parser")
print(soup.title.string)
PyAutoGUI: GUIの自動化ライブラリ。マウスやキーボードの操作を自動化できます。
import pyautogui
pyautogui.moveTo(100, 100, duration=1)
pyautogui.click()
8. ネットワーク・ウェブサービス
Requests: HTTPリクエストを送るためのライブラリ。ウェブAPIとの通信に便利です。
import requests
response = requests.get('https://api.github.com')
print(response.json())
Socket: ネットワーク通信を行うためのライブラリ。ソケットプログラミングに使用されます。
import socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect(('www.example.com', 80))
s.sendall(b'GET / HTTP/1.1\r\nHost: www.example.com\r\n\r\n')
response = s.recv(4096)
print(response.decode('utf-8'))
s.close()
9. データベース
SQLAlchemy: データベースの操作を簡単に行うためのORM(オブジェクトリレーショナルマッピング)ライブラリ。
from sqlalchemy import create_engine
engine = create_engine('sqlite:///example.db')
connection = engine.connect()
result = connection.execute("SELECT 'hello world'")
for row in result:
print(row)
connection.close()
SQLite3: 組み込みデータベースを操作するためのライブラリ。軽量で使いやすい。
import sqlite3
conn = sqlite3.connect('example.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)''')
c.execute("INSERT INTO users (name) VALUES ('Alice')")
conn.commit()
conn.close()
10. その他
Pygame: ゲーム開発用のライブラリ。シンプルな2Dゲームを作成できます。
import pygame
pygame.init()
screen = pygame.display.set_mode((640, 480))
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
pygame.quit()
Click: コマンドラインインターフェース(CLI)を作成するためのライブラリ。
import click
@click.command()
@click.option('--count', default=1, help='Number of greetings.')
@click.option('--name', prompt='Your name', help='The person to greet.')
def hello(count, name):
for _ in range(count):
click.echo(f'Hello, {name}!')
if __name__ == '__main__':
hello()
Rich: カラフルなターミナル出力を作成するためのライブラリ。
from rich.console import Console
console = Console()
console.print("Hello, [bold magenta]World[/bold magenta]!")
まとめ
これらのツールを使いこなすことで、Pythonをさらに効率的に活用できるようになります。各ツールの公式ドキュメントを参照し、さらに深く理解していくことをおすすめします。
この記事が気に入ったらサポートをしてみませんか?