電験三種の電力公式
import numpy as np
# 充電電流の計算
def calculate_charging_current(f, C, V):
return 2 * np.pi * f * C * V / np.sqrt(3)
# 発電機出力の計算
def calculate_generator_output(water_flow, effective_head):
return 9.8 * water_flow * effective_head
# 年間発電電力量の計算
def calculate_annual_energy(generator_output, utilization_rate):
hours_per_year = 365 * 24
return generator_output * hours_per_year * utilization_rate
# 各種パラメータを設定
f = 50 # 周波数(Hz)
C = 1e-6 # 静電容量(F)
V = 220 # 電圧(V)
water_flow = 10 # 発電使用水量(m^3/s)
effective_head = 50 # 有効落差(m)
utilization_rate = 0.5 # 設備利用率
# 計算を実行
charging_current = calculate_charging_current(f, C, V)
generator_output = calculate_generator_output(water_flow, effective_head)
annual_energy = calculate_annual_energy(generator_output, utilization_rate)
# 結果を出力
print("充電電流:", charging_current, "A")
print("発電機出力:", generator_output, "W")
print("年間発電電力量:", annual_energy, "Wh")
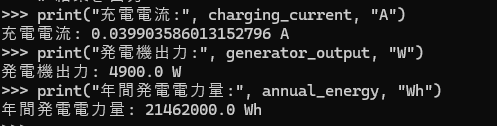
import numpy as np
import matplotlib.pyplot as plt
# 定数の設定
V = 10 # 電圧
v = 1 # 電圧降下
R = 5 # 抵抗
X = 3 # 反応
theta = np.linspace(0, 2*np.pi, 100) # 角度の範囲
# 式の計算
numerator = V * v * np.cos(theta)
denominator = R * np.cos(theta) + X * np.sqrt(1 - np.cos(theta)**2)
voltage_drop = numerator / denominator
# プロット
plt.plot(theta, voltage_drop)
plt.title('Voltage Drop vs Angle')
plt.xlabel('Angle (theta)')
plt.ylabel('Voltage Drop')
plt.grid(True)
plt.show()
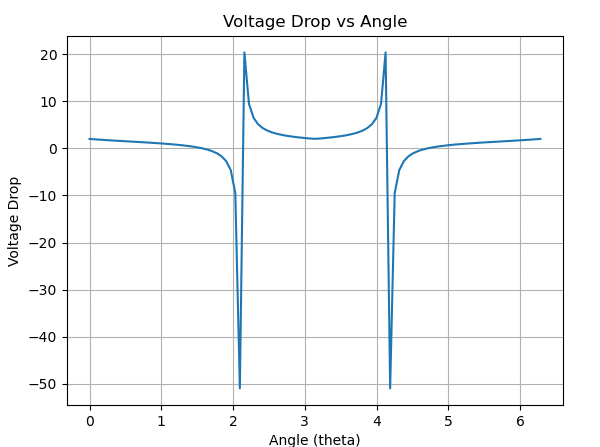
import numpy as np
import matplotlib.pyplot as plt
# 関数定義
def calculate_sag(w, L, T):
return (w * L**2) / (8 * T)
# パラメータ設定
w = 0.1 # 電線の単位長さあたりの重量 (重量/長さ) [例: kg/m]
L = np.linspace(50, 200, 100) # 電線の実長 (span) [例: m]
T = 1000 # 電線の張力 [例: N]
# たるみを計算
sag = calculate_sag(w, L, T)
# プロット
plt.plot(L, sag)
plt.title('Sag vs Span')
plt.xlabel('Span (m)')
plt.ylabel('Sag (m)')
plt.grid(True)
plt.show()
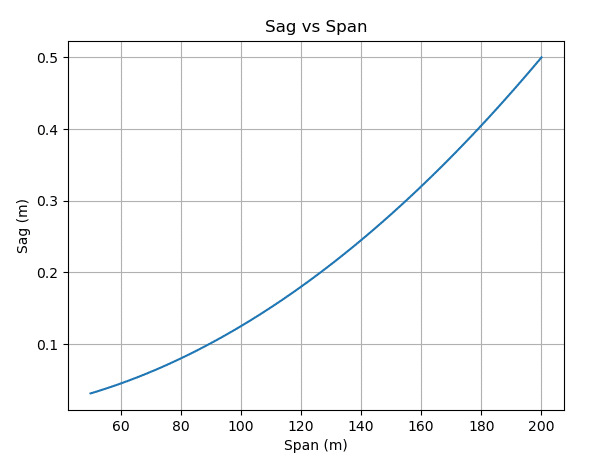
この記事が気に入ったらサポートをしてみませんか?