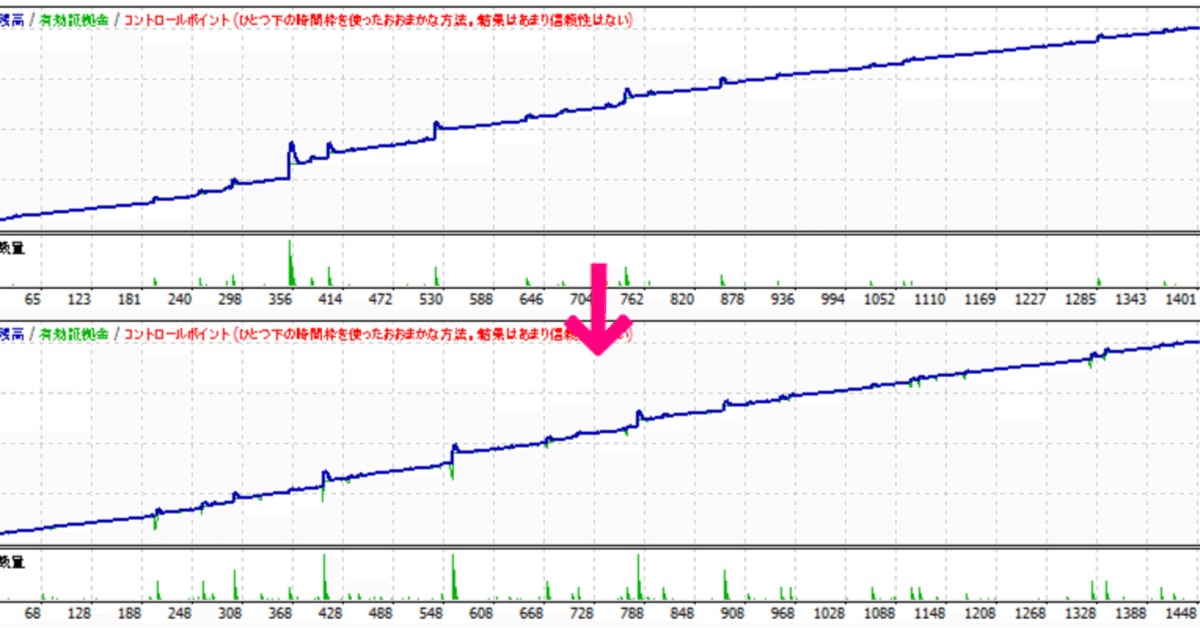
「Grid Free3」をさらにバージョンアップしてみた→「Grid Free4」
変更点①RSIのエントリー条件
Grid Free3ではRSIのエントリー条件が、デフォルト設定では
①1本前のローソク足で30以下、70以上
②現在のローソク足で30より上、70より下に抜けたらエントリー
になっていました。
Grid Free4のデフォルト設定では、
①1本前のローソク足で29より下、71より上
②現在のローソク足で29+1以上、71-1以下になったらエントリー
という条件に変えてみました。
bool is_buy()
{
double rsi = iRSI(NULL,0,rsikikan,0,1);
double rsi2 = iRSI(NULL,0,rsikikan,0,0);
if(rsi < rsilow && rsi2 >= rsilow+agehaba)
{
return true;
}
return false;
}
なので、パラメーターの設定項目は
・RSI下ライン(買い)
・RSI下からの上げ幅(買い)
・RSI上ライン(売り)
・RSI上からの下げ幅(売り)
というようにしてみました。
変更点②両建てエントリー可能
Grid Free3では買いエントリーが入っている時は売りエントリーが入らないようになっていました。
Grid Free4では買いエントリーが入っているときでも売りエントリーが入れられるようにしてみました。
これによって、例えば買いエントリーがなかなか決済できないでいる間に、売りエントリーの条件が揃ったら売りのエントリーもできるようになります。
//エントリー処理
if(is_buy() && is_up_trend() && posi_count(OP_BUY) == 0)
{
position_entry(OP_BUY);
}
if(is_sell() && is_down_trend() && posi_count(OP_SELL) == 0)
{
position_entry(OP_SELL);
}
変更点③ナンピンエントリーにもスプレッドフィルター
Grid Free3ではスプレッドフィルターを、一番上の方のエントリー処理のところに入れていました。
Grid Free4では、ずっと下の方にある
void position_entry(int side)
のところと、
void position_entry_nanpin(int side,double bairitu)
のところに入れてみました。
void position_entry(int side)
{
double qty = lots;
if(side==0 && MarketInfo(Symbol(),MODE_SPREAD) < MaxSp)
{
bool res= OrderSend(NULL,side,qty,Ask,0,0,0,NULL,MagicNumber,0,clrGreen);
}
if(side==1 && MarketInfo(Symbol(),MODE_SPREAD) < MaxSp)
{
bool res= OrderSend(NULL,side,qty,Bid,0,0,0,NULL,MagicNumber,0,clrRed);
}
}
void position_entry_nanpin(int side,double bairitu)
{
double qty = lots;
if(side==0 && MarketInfo(Symbol(),MODE_SPREAD) < MaxSp)
{
bool res= OrderSend(NULL,side,qty * bairitu,Ask,0,0,0,NULL,MagicNumber,0,clrGreen);
}
if(side==1 && MarketInfo(Symbol(),MODE_SPREAD) < MaxSp)
{
bool res= OrderSend(NULL,side,qty * bairitu,Bid,0,0,0,NULL,MagicNumber,0,clrRed);
}
}
変更点④パラメーターを少し書き換え
Grid Free3ではナンピン幅と表記していたところを、グリッド幅に変更し、マジックナンバーのデフォルト設定を777999から77701に変更してみました。
あとはRSIの条件を変えたので、RSIの設定項目も増えました。
input int MagicNumber = 77701;//マジックナンバー
input double lots = 0.1;//ロット数
input int MaxSp = 45;//最大スプレッド
input int rikakuhaba = 150;//利確幅
input int nanpinhaba =300;//グリッド幅
input double lotbai = 1.5;//ロット倍率
input int rsikikan =14;//RSI計算期間
input int rsilow =29;//RSI下ライン(買い)
input int agehaba =1;//RSI下からの上げ幅(買い)
input int rsihigh =71;//RSI上ライン(売り)
input int sagehaba =1;//RSI上からの下げ幅(売り)
input int shift1 =100;//モメンタムフィルター1
input int shift2 =200;//モメンタムフィルター2
バックテストの比較
Grid Free3のNZDCADのバックテスト↓
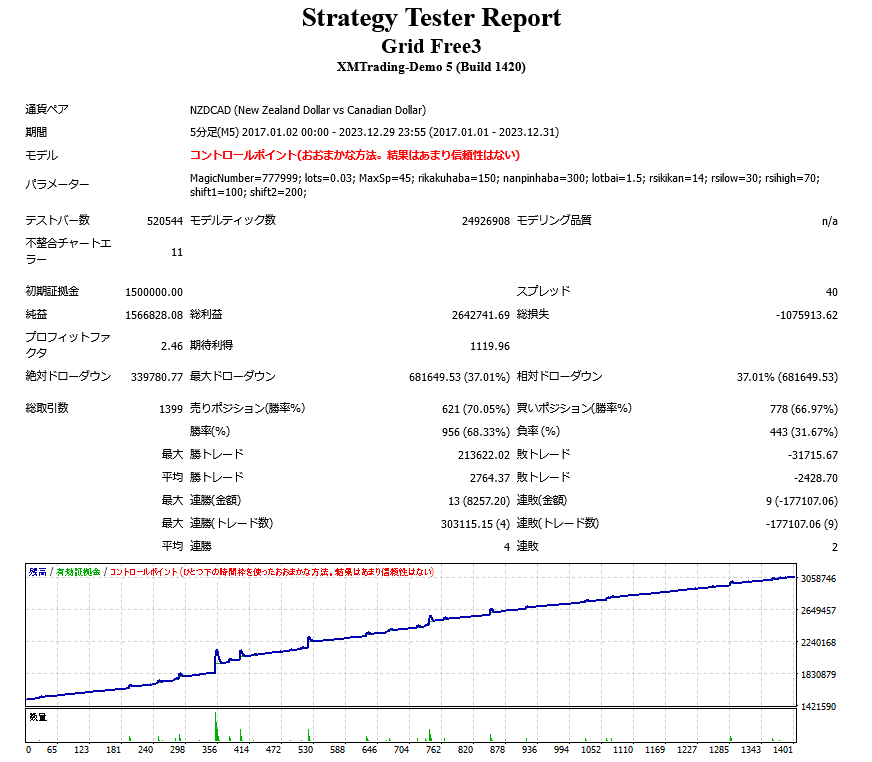
Grid Free4のNZDCADのバックテスト↓
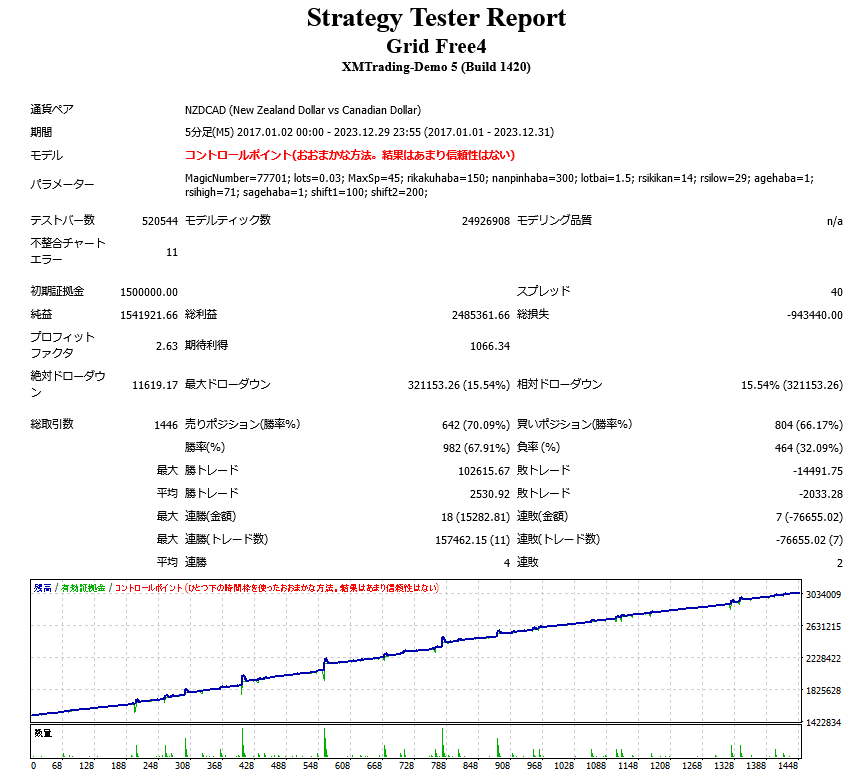
Grid Free4のソースコード
#property copyright "けんじ2405"
#property link "https://note.com/just_pothos4327"
#property version "1.00"
#property strict
input int MagicNumber = 77701;//マジックナンバー
input double lots = 0.1;//ロット数
input int MaxSp = 45;//最大スプレッド
input int rikakuhaba = 150;//利確幅
input int nanpinhaba =300;//グリッド幅
input double lotbai = 1.5;//ロット倍率
input int rsikikan =14;//RSI計算期間
input int rsilow =29;//RSI下ライン(買い)
input int agehaba =1;//RSI下からの上げ幅(買い)
input int rsihigh =71;//RSI上ライン(売り)
input int sagehaba =1;//RSI上からの下げ幅(売り)
input int shift1 =100;//モメンタムフィルター1
input int shift2 =200;//モメンタムフィルター2
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void OnTick()
{
//エントリー処理
if(is_buy() && is_up_trend() && posi_count(OP_BUY) == 0)
{
position_entry(OP_BUY);
}
if(is_sell() && is_down_trend() && posi_count(OP_SELL) == 0)
{
position_entry(OP_SELL);
}
//決済処理
if(posi_count(OP_BUY) > 0)
{
if(position_average_price(0) + rikakuhaba * _Point < Bid)
{
position_close(0);
}
}
if(posi_count(OP_SELL) > 0)
{
if(position_average_price(1) - rikakuhaba * _Point > Ask)
{
position_close(1);
}
}
//ナンピンマーチン
nanpin_martin_judge();
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
bool is_buy()
{
double rsi = iRSI(NULL,0,rsikikan,0,1);
double rsi2 = iRSI(NULL,0,rsikikan,0,0);
if(rsi < rsilow && rsi2 >= rsilow+agehaba)
{
return true;
}
return false;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
bool is_sell()
{
double rsi = iRSI(NULL,0,rsikikan,0,1);
double rsi2 = iRSI(NULL,0,rsikikan,0,0);
if(rsi > rsihigh && rsi2 <= rsihigh-sagehaba)
{
return true;
}
return false;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
bool is_up_trend()
{
double close = iClose(NULL,0,0);
double close100 = iClose(NULL,0,shift1);
double close200 = iClose(NULL,0,shift2);
double mom100 = close - close100;
double mom200 = close - close200;
//「momが0超え」なら上昇トレンド
if(0 < mom100 && 0 < mom200)
{
return true;
}
return false;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
bool is_down_trend()
{
double close = iClose(NULL,0,0);
double close100 = iClose(NULL,0,shift1);
double close200 = iClose(NULL,0,shift2);
double mom100 = close - close100;
double mom200 = close - close200;
//「momが0未満」なら下降トレンド
if(0 > mom100 && 0 > mom200)
{
return true;
}
return false;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
int posi_count(int side)
{
int count = 0;
for(int i = OrdersTotal() - 1; i >= 0; i--)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if(OrderType() == side)
{
if(OrderSymbol()==Symbol())
{
if(OrderMagicNumber()==MagicNumber)
{
count++;
}
}
}
}
}
return count;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void position_entry(int side)
{
double qty = lots;
if(side==0 && MarketInfo(Symbol(),MODE_SPREAD) < MaxSp)
{
bool res= OrderSend(NULL,side,qty,Ask,0,0,0,NULL,MagicNumber,0,clrGreen);
}
if(side==1 && MarketInfo(Symbol(),MODE_SPREAD) < MaxSp)
{
bool res= OrderSend(NULL,side,qty,Bid,0,0,0,NULL,MagicNumber,0,clrRed);
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void position_entry_nanpin(int side,double bairitu)
{
double qty = lots;
if(side==0 && MarketInfo(Symbol(),MODE_SPREAD) < MaxSp)
{
bool res= OrderSend(NULL,side,qty * bairitu,Ask,0,0,0,NULL,MagicNumber,0,clrGreen);
}
if(side==1 && MarketInfo(Symbol(),MODE_SPREAD) < MaxSp)
{
bool res= OrderSend(NULL,side,qty * bairitu,Bid,0,0,0,NULL,MagicNumber,0,clrRed);
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void position_close(int side)
{
for(int i = OrdersTotal() - 1; i >= 0; i--)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if(OrderType() == side)
{
if(OrderSymbol()==Symbol())
{
if(OrderMagicNumber()==MagicNumber)
{
bool res= OrderClose(OrderTicket(), OrderLots(), OrderClosePrice(), 0, clrBlue);
}
}
}
}
}
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
double position_average_price(int side)
{
double lots_sum = 0;
double price_sum = 0;
double average_price = 0;
for(int i = OrdersTotal() - 1; i >= 0; i--)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if(OrderType() == side)
{
if(OrderSymbol()==Symbol())
{
if(OrderMagicNumber()==MagicNumber)
{
lots_sum += OrderLots();
price_sum += OrderOpenPrice() * OrderLots();
}
}
}
}
}
if(lots_sum && price_sum)
{
average_price = price_sum/lots_sum;
}
return average_price;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
double position_entry_price(int side)
{
double entry_prices[50];
double entry_price=0;
ArrayInitialize(entry_prices,0.0);
if(side ==1)
{
ArrayInitialize(entry_prices,9999999.0);
}
for(int i = OrdersTotal() - 1; i >= 0; i--)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if(OrderType() == side)
{
if(OrderSymbol()==Symbol())
{
if(OrderMagicNumber()==MagicNumber)
{
entry_prices[i] = OrderOpenPrice();
}
}
}
}
}
if(side==0)
{
entry_price = entry_prices[ArrayMaximum(entry_prices,WHOLE_ARRAY,0)];
}
if(side==1)
{
entry_price = entry_prices[ArrayMinimum(entry_prices,WHOLE_ARRAY,0)];
}
return entry_price;
}
//+------------------------------------------------------------------+
//| |
//+------------------------------------------------------------------+
void nanpin_martin_judge()
{
if(posi_count(OP_BUY) > 0)
{
int position_num = posi_count(OP_BUY);
double entry_price = position_entry_price(OP_BUY);
double nanpin_price = entry_price - (nanpinhaba * _Point) * position_num;
double lots_bairitu = MathPow(lotbai,position_num);
if(nanpin_price > Ask)
{
position_entry_nanpin(OP_BUY,lots_bairitu);
}
}
if(posi_count(OP_SELL) > 0)
{
int position_num = posi_count(OP_SELL);
double entry_price = position_entry_price(OP_SELL);
double nanpin_price = entry_price + (nanpinhaba * _Point) * position_num;
double lots_bairitu = MathPow(lotbai,position_num);
if(nanpin_price < Bid)
{
position_entry_nanpin(OP_SELL,lots_bairitu);
}
}
}
//+------------------------------------------------------------------+
もし間違いや直した方が良い所があったら教えてください。
もしよかったら、記事の下の「記事をサポート」をお願い致します。お金が稼げなくて困っています。奨学金の返済、生活費、病院代、勉強代などに使いたいと思っております。
この記事が気に入ったらサポートをしてみませんか?