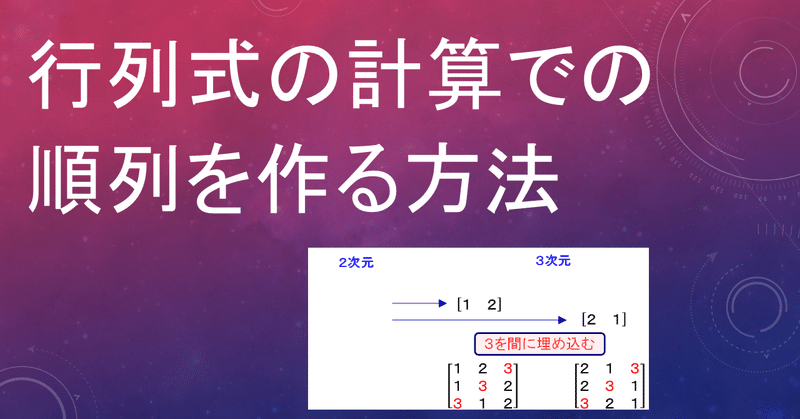
行列式の計算での順列を作る方法
行列式の計算をするときなどに必要な数字の全ての順列を作る方法を考えます。はじめに、2次元の配列[[1,2],[2,1]]を3次元に増やす方法を作成します。
import copy
def d_plus(index):
temp = []
dim=len(index[0])+1
for i in range(len(index)):
for j in reversed(range(dim)):
row = copy.deepcopy(index[i])
row.insert(j, dim)
temp.append(row)
return sorted(temp)
index = [[1, 2], [2, 1]]
d_plus(index)
#[[1, 2, 3], [1, 3, 2], [2, 1, 3], [2, 3, 1], [3, 1, 2], [3, 2, 1]]
これを、さらに次元を増やすため再帰関数にします。4次元の場合は、index_m関数に引数として4を指定するだけです。
def index_m(n,index=[[1]]):
print('in')
if n ==1:
return index
else:
return index_m(n-1,d_plus(index))
index_m(4)
かなりめんどくさいので、itertoolsを使ってみます。
import itertools
l = list(itertools.permutations(range(4)))
これだけでできてしまいます。ここでlistを作っているので、ここから1つ1つ読んで処理すればよいわけですが、1度使った要素は必要なくなるので、次の方法は非常に洗練された方法となります。
i = itertools.permutations(range(4))
print("itertoolsのアウトプット:", i)
print("1回目:", next(i))
print("2回目:", next(i))
print("3回目:", next(i))
#1回目: (0, 1, 2, 3)
#2回目: (0, 1, 3, 2)
#3回目: (0, 2, 1, 3)
このように、nextで次々とデータを呼び出し、計算が終わったらメモリから消えてしまうので、限られたメモリを効率的に使うことができます。
この記事が気に入ったらサポートをしてみませんか?