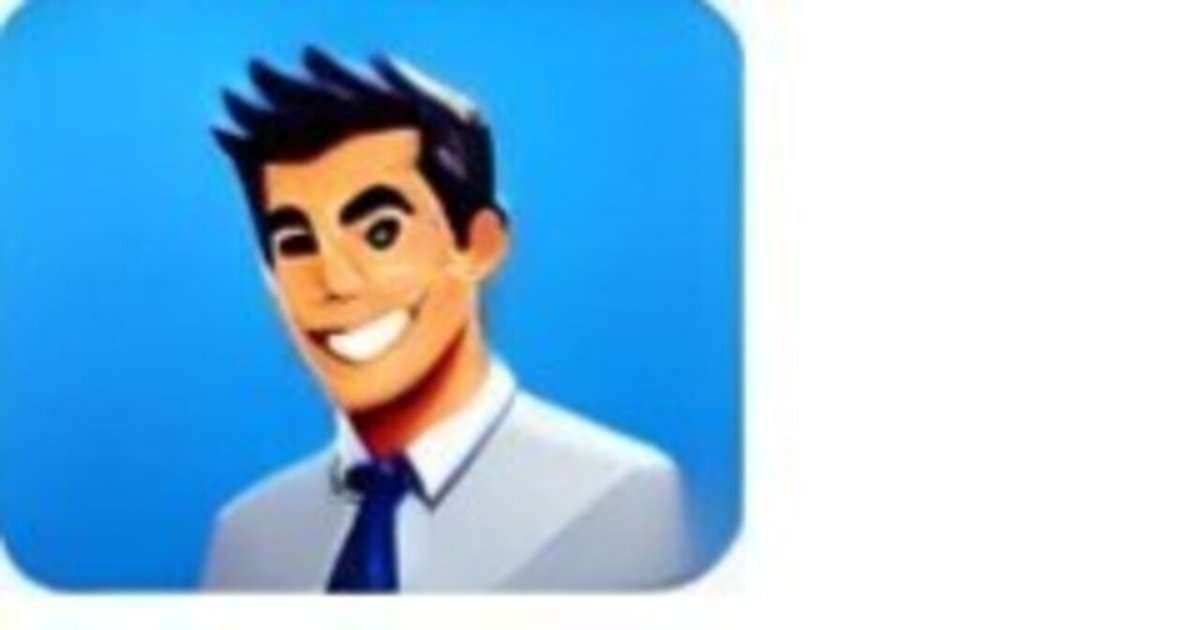
OpenAIを使った音声要約(whisper-1)
事前にOpenAIのAPIキーは取得しておいてください
環境 Ubuntu22.04
whisper 1.1.10
openai 1.35.7
APIキーは事前にExport しておいてください
モデルもご自由に変更してください
コード例
-----------------------------------------------------------------------------
import openai
import streamlit as st
import tempfile
from openai import OpenAI
client = OpenAI()
st.title("音声文字起こしと要約")
サポートされている音声ファイルの形式
SUPPORTED_FORMATS = ["mp3", "flac", "m4a", "mp4", "mpeg", "mpga", "oga", "ogg", "wav", "webm"]
音声ファイルのアップロード
audio_file = st.file_uploader("音声ファイルをアップロードしてください", type=SUPPORTED_FORMATS)
音声ファイルがアップロードされた場合
if audio_file is not None:
file_details = {"FileName": audio_file.name, "FileType": audio_file.type}
st.write(file_details)
st.audio(audio_file, format='audio/mp3')
# Whisperで音声から文字起こし
with st.spinner("文字起こし中..."):
# 一時ファイルに音声ファイルを保存
with tempfile.NamedTemporaryFile(delete=False, suffix=".mp3") as temp_file:
temp_file.write(audio_file.getbuffer())
temp_file_path = temp_file.name
# 一時ファイルを開いて転写
with open(temp_file_path, "rb") as f:
transcript = client.audio.transcriptions.create(
model="whisper-1",
file=f,
response_format="text"
)
st.write("文字起こし結果:")
st.write(transcript)
# 要約の指示を入力
prompt_base = st.text_area("要約の指示を入力してください", "次の文章を200字 程度で要約してください。")
if st.button("要約する"):
prompt = prompt_base + "\n\n" + transcript
with st.spinner("要約中..."):
response = client.chat.completions.create(
model="gpt-4-0613",
messages=[
{
"role": "system",
"content": "あなたは文字起こしの要約を作成するアシスタントです。提供された文字起こしのテキストを元に要約を生成してください。要約はMarkdownで出力してください。"
},
{
"role": "user",
"content": f"音声文字起こしの内容: {transcript}"
}
],
temperature=0,
)
st.write("要約結果:")
st.write(response.choices[0].message.content)
streamlitでアプリを起動してください
通常なら http://localhost:8501 で動けばOKです
この記事が気に入ったらサポートをしてみませんか?