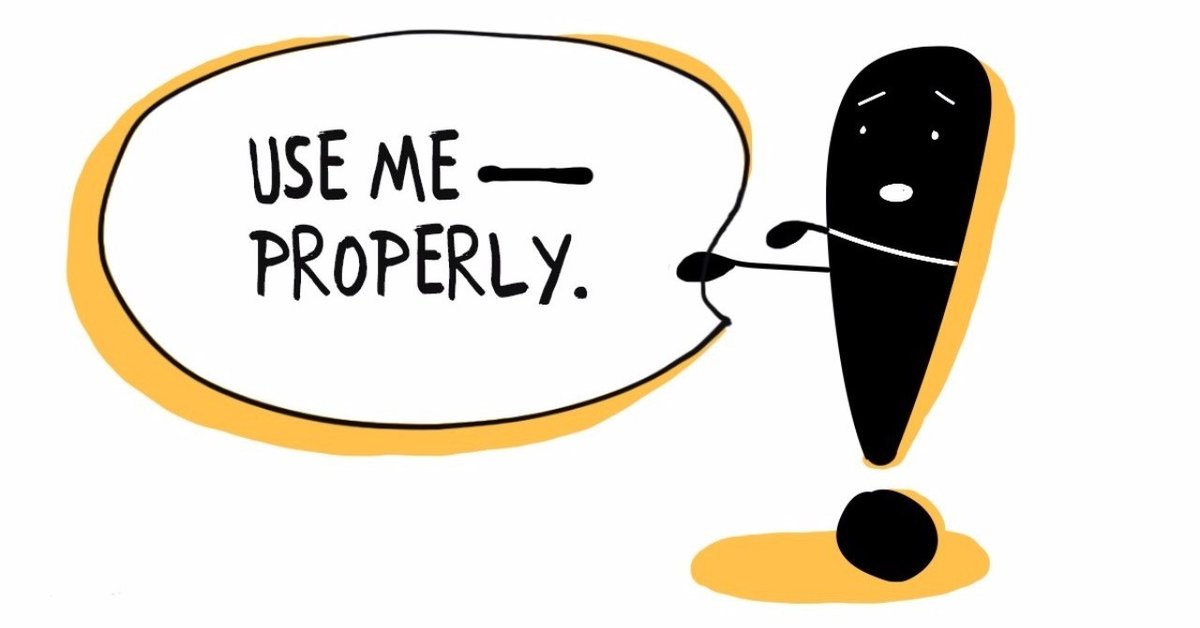
Factorial with recursion
Ever wonder how recursion works in code? Imagine when you are given 6!, you first multiply the next integer factorial in sequence (6! = 6*5!). You repeat the process until 0 is reached. This works the same way in programming. Just think of each multiplication as a small process written as a function. The function gets called until 0 is reached.
#include <iostream>
using namespace std;
int factorial(int i) {
if( i != 0 ) {
return i*factorial(i-1);
} else {
return 1;
}
};
int main()
{
for(int i = 0; i <= 6; i++)
cout << i << "! : " << factorial(i) << endl;
return 0;
}
#Output
0! : 1
1! : 1
2! : 2
3! : 6
4! : 24
5! : 120
6! : 720
...Program finished with exit code 0
この記事が気に入ったらサポートをしてみませんか?