python クラスの継承
class Car(object):
def drive(self):
print('drive')
class MyCar(Car):
pass
MyCarクラスは、Carのクラスを継承している。’pass’は何も持たないクラスとしている。my_carはCarクラスの関数を使える。
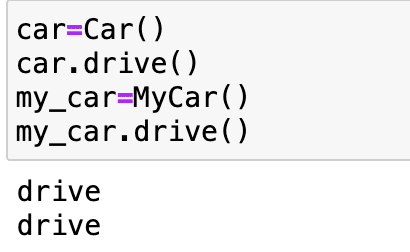
継承したクラスは継承元の関数の上書き実行(オーバーライド)ができる。
class Car(object):
def drive(self):
print('drive')
class MyCar(Car):
def drive(self):
print('drive faster')
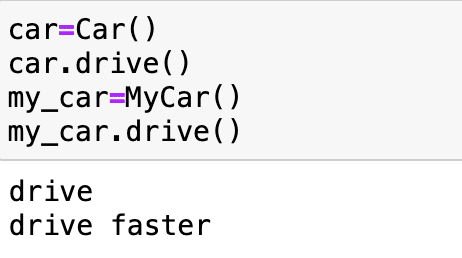
継承元の同名の関数を呼びたいときは、super()をつける。
class Car(object):
def __init__(self,model=None):
self.model=model
def drive(self):
print('drive')
class MyCar(Car):
def __init__(self,model='SUV',enable_autorun=False):
super().__init__(model)
self.enable_autorun=enable_autorun
def drive(self):
print('drive faster')
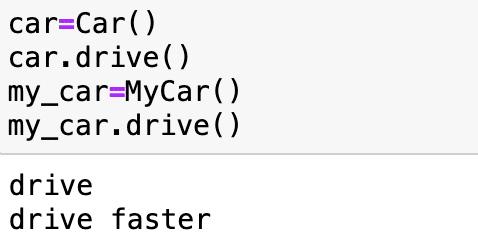
クラス外からオブジェクト変数を書き換えられたくない場合は、変数の前に'_'をつけ、この変数を返すだけの関数を定義し、@property宣言をする。これをプロパティのゲッターを呼ぶ。また、property変数に値を入れたい場合は、プロパティのセッター関数を宣言する。
class Car(object):
def __init__(self,model=None):
self.model=model
def drive(self):
print('drive')
class MyCar(Car):
def __init__(self,model='SUV',enable_autorun=False):
super().__init__(model)
self._enable_autorun=enable_autorun
def drive(self):
print('drive faster')
@property
def enable_auto_run(self):
return self._enable_auto_run
@enable_auto_run.setter
def enable_auto_run(self,is_enable):
self._enable_auto_run=is_enable
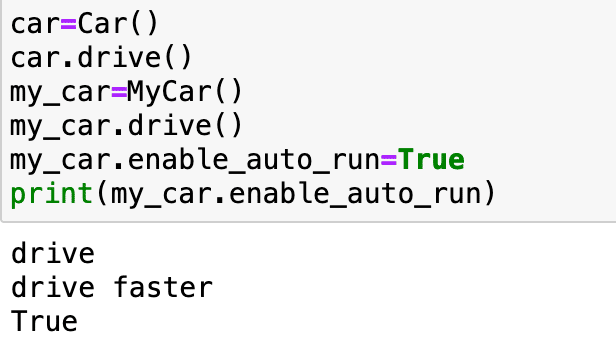
クラス外から参照もされたくない場合は、変数の前に"_"を二つつけると、ゲッターを通じてもエラーが返る。クラス内の関数からは呼び出せている。
class Car(object):
def __init__(self,model=None):
self.model=model
def drive(self):
print('drive')
class MyCar(Car):
def __init__(self,model='SUV',enable_autorun=False):
super().__init__(model)
self.__enable_autorun=enable_autorun
def drive(self):
print(self.__enable_autorun)
print('drive faster')
@property
def enable_auto_run(self):
return self.__enable_auto_run
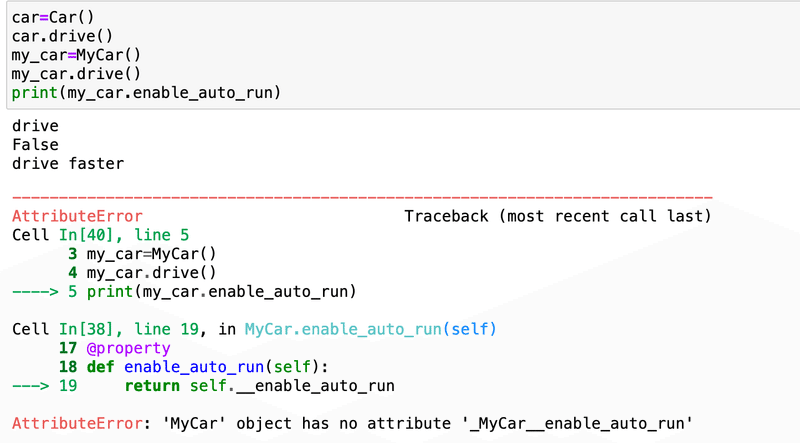
クラス外から"_"付きの変数を宣言するのは、中のクラス変数がわからないので、やめた方が良い。
この記事が気に入ったらサポートをしてみませんか?