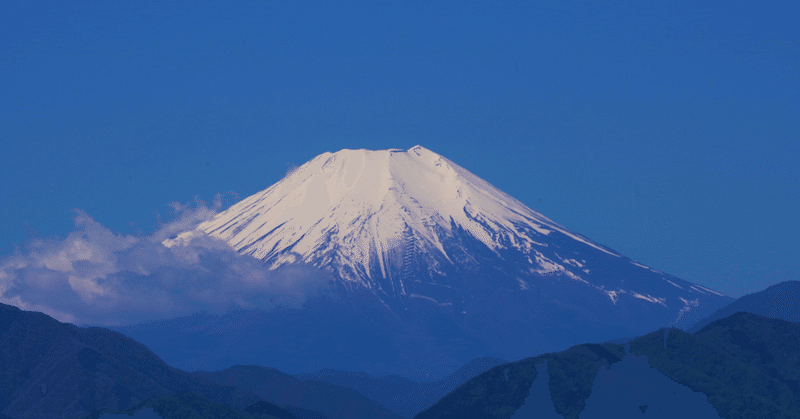
Photo by
chocho3
PythonでAzureのGPT-4oをやってみた
OpenAIのGPT-4oをPythonで実行する情報が少なかったため、自分で試してみた結果をまとめたメモを紹介します。GPT-4oはAzureで提供されていますが、実行方法や利用例についての情報が限られているため、必要なライブラリのインストール方法などを解説します。さらに、実際に実行して得られる結果についてもご紹介します。
1. GPT-4oとは?
GPT-4oはテキストだけでなく、言語や画像、音声、動画のすべてを処理できるマルチモーダルモデルです。そのため画像や動画の内容を理解し、それに基づいた出力ができます。
2. GPT-4oを動作させる環境構築
ライブラリのインストール
pip install openai==0.28
import openai
print(openai.__version__)
3. GPT-4oを用いて画像を解析
import os
import openai
from openai import util
import base64
import json
openai.api_type = "azure"
openai.api_base = "Azureのエンドポイント"
openai.api_version = "2024-05-01-preview"
openai.api_key = "AzureのAPIキー"
img_path = "./test.png" ♯解析したい画像パス
# 画像をBase64文字列としてエンコード
def encode_img(image_path):
with open(image_path, "rb") as image_file:
return base64.b64encode(image_file.read()).decode("utf-8")
base64_image = encode_img(img_path )
try:
response = openai.ChatCompletion.create(
engine="gpt-4o", #ここはAzureでデプロイした名前に変えてください
messages = [{"role":"system","content":""}, {"role":"user","content":[
{"type": "text", "text": "これは何の画像ですか"},
{"type": "image_url", "image_url": {"url": f"data:image/png;base64,{base64_image}"}},
] },],
temperature=0.5,top_p=0.95,frequency_penalty=0,presence_penalty=0,stop=None)
response_txt = util.convert_to_dict(response)
output = response_txt["choices"][0]["message"]["content"]
print(output)
except Exception as e:
print(e)
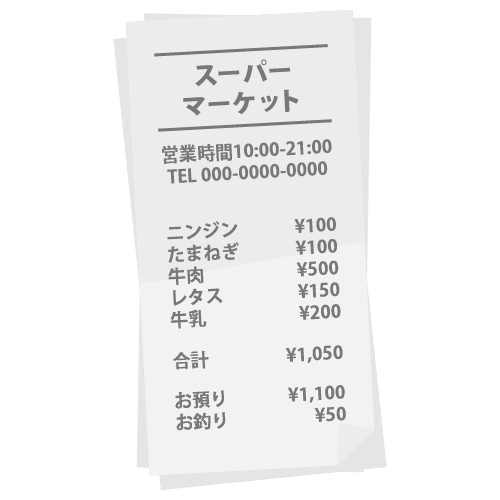
解析結果
これはスーパーマーケットのレシートです。レシートには、購入した商品のリストとその価格、合計金額、お預かり金額、お釣りが記載されています。具体的には、以下のような内容です:
- ニンジン: ¥100
- たまねぎ: ¥100
- 牛肉: ¥500
- レタス: ¥150
- 牛乳: ¥200
合計金額は ¥1,050 で、お預かり金額は ¥1,100、そしてお釣りは ¥50 です。