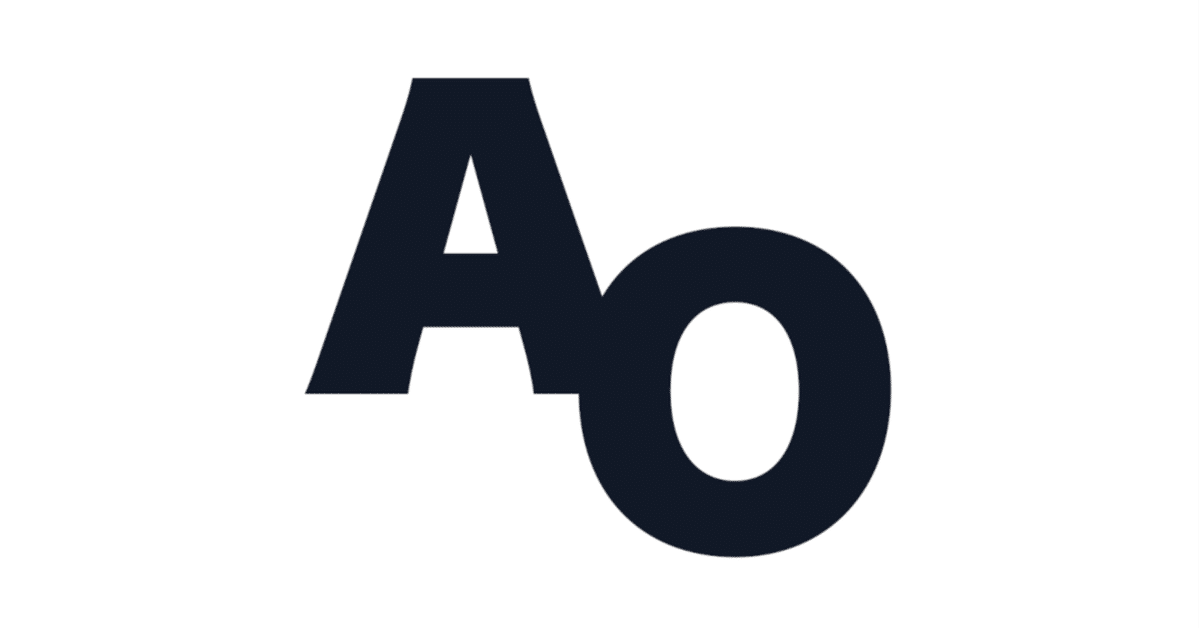
How to animate Tailwind's UI component Flowbite's Navbar
This is a memo on how to implement a simple animation where a menu appears from top to bottom.
Please refer to the Flowbite website for the basic implementation method of navigation menu using Flowbite.
Tailwind CSS Navbar - Flowbite
How to Customize
1.Add "transition" to the div tag class of the menu.
class="....transition-all ease-in-out duration-500"
2.Create your own class by inheriting from Collapse class
class MenuCollapse extends Collapse {
private body = document.querySelector('body');
public collapse() {
//console.log('menu collapse');
// Scroll settings for body
this.body.classList.remove('h-full');
this.body.classList.remove('overflow-hidden');
// Show/hide hamburger icon
this._triggerEl.querySelector('.open-button').classList.remove('hidden');
this._triggerEl.querySelector('.close-button').classList.add('hidden');
// Set height to 0
this._targetEl.style.height = '0px';
if (this._triggerEl) {
this._triggerEl.setAttribute('aria-expanded', 'false');
}
this._visible = false;
this._options.onCollapse(this);
}
public expand() {
//console.log('menu expand');
// Scroll settings for body
this.body.classList.add('h-full');
this.body.classList.add('overflow-hidden');
// Show/hide hamburger icon
this._triggerEl.querySelector('.open-button').classList.add('hidden');
this._triggerEl.querySelector('.close-button').classList.remove('hidden');
// Set height to "window height - header height"
this._targetEl.style.height = (window.innerHeight - 92) + 'px';
if (this._triggerEl) {
this._triggerEl.setAttribute('aria-expanded', 'true');
}
this._visible = true;
this._options.onExpand(this);
}
}
3.Setup Collapse using your own class.
const navbarStickyMenu = document.getElementById('navbar-sticky-menu');
const navbarStickyButton = document.getElementById('navbar-sticky-button');
const navbarStickyCollapse = new MenuCollapse(
navbarStickyMenu,
navbarStickyButton,
{
onCollapse: () => {
},
onExpand: () => {
},
onToggle: () => {
}
}
);
You can create various animations by changing the CSS class added to the menu's div tag and customizing the implementation in the collapse and expand methods of your own class. I'm glad if you can use it as a reference.
A website that implements the above animation
この記事が気に入ったらサポートをしてみませんか?