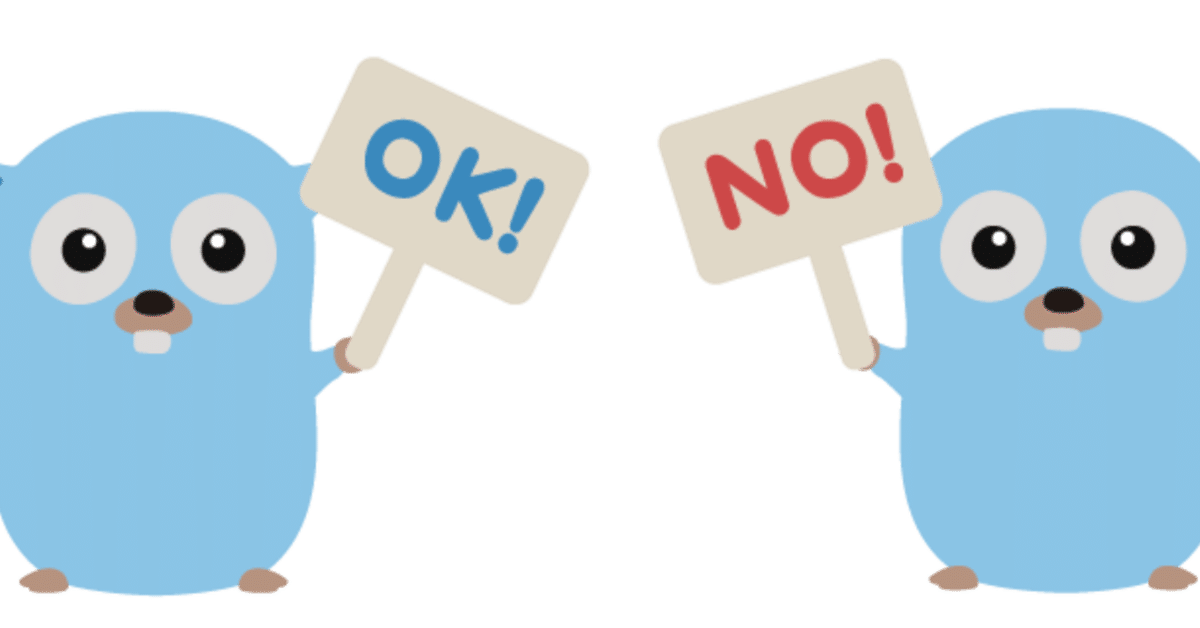
Go -⑨基本-
◼︎Semaphore
◼︎◼︎Acquire
◼︎出力
WAIT...
DONE
WAIT...
DONE
WAIT...
DONE
WAIT...
DONE
◼︎コード
package main
import (
"context"
"fmt"
"golang.org/x/sync/semaphore"
"time"
)
var s *semaphore.Weighted = semaphore.NewWeighted(1)
func longProcess(ctx context.Context) {
// semaphoreを利用して、goroutineが出来る処理を制限する(1つに制限している)
if err := s.Acquire(ctx,1); err != nil{
fmt.Println(err)
return
}
defer s.Release(1)
fmt.Println("WAIT...")
time.Sleep(1 * time.Second)
fmt.Println("DONE")
}
func main() {
ctx := context.TODO()
go longProcess(ctx)
go longProcess(ctx)
go longProcess(ctx)
time.Sleep(2 * time.Second)
go longProcess(ctx)
time.Sleep(4 * time.Second)
}
◼︎◼︎TryAcquire
◼︎出力
Cloud not get lock
WAIT...
Cloud not get lock
DONE
WAIT...
DONE
◼︎コード
package main
import (
"context"
"fmt"
"golang.org/x/sync/semaphore"
"time"
)
var s *semaphore.Weighted = semaphore.NewWeighted(1)
func longProcess(ctx context.Context) {
// goroutineのブロックをする必要ない場合に利用する
isAcquire := s.TryAcquire(1)
if !isAcquire {
fmt.Println("Cloud not get lock")
return
}
defer s.Release(1)
fmt.Println("WAIT...")
time.Sleep(1 * time.Second)
fmt.Println("DONE")
}
func main() {
ctx := context.TODO()
go longProcess(ctx)
go longProcess(ctx)
go longProcess(ctx)
time.Sleep(2 * time.Second)
go longProcess(ctx)
time.Sleep(4 * time.Second)
}
◼︎ini
設定ファイルの読み込みに利用する。
◼︎出力
int 8080
string test.sql
string
◼︎コード
config.ini
[web]
port = 8080
[db]
name = test.sql
package main
import (
"fmt"
"github.com/go-ini/ini"
)
type ConfigStruct struct {
Port int
DBName string
SQLDriver string
}
var Config ConfigStruct
// メソッドで、Keyがない場合のデフォルト値を入れることもできる。
func init(){
cfg, _ := ini.Load("config.ini")
Config = ConfigStruct{
Port: cfg.Section("web").Key("port").MustInt(),
DBName: cfg.Section("db").Key("name").MustString("example.sql"),
SQLDriver: cfg.Section("db").Key("driver").String(),
}
}
func main() {
fmt.Printf("%T %v\n", Config.Port, Config.Port)
fmt.Printf("%T %v\n", Config.DBName, Config.DBName)
fmt.Printf("%T %v\n", Config.SQLDriver, Config.SQLDriver)
}
◼︎talib
◼︎出力
2016-03-31 00:00,205.91,206.41,205.33,193.83,94584100.00
[0 0 11.805025388205461 2.73740299059981 1.6236145007809848 8.28276201332699 56.41093654581726 13.209683294186611 56.23407877811769 24.26308776781728 29.15150126743054 12.988550998986303 41.14944083869526 82.6083640242499 40.111402479191675 68.71927598497307 38.7695052579787 56.656763720177054 88.43641270428762 86.5098320714449 27.27358495574894 49.751253557198574 56.78193860478494 12.891573135287814 6.223209030030611 6.605809189054875 5.844176427837823 1.3065066569506432 71.21992187988707 86.82099120825414 93.62390630531856 74.1225775896882 70.76484327828459 92.3046485919883 40.064063270628615 57.33840301252735 83.15608766482244 67.45581951284822 29.534747362248353 83.77724522793423 87.53193626320235 91.13023192356466 94.01095515483145 94.83636888913551 19.86613045708022 53.06989685980222 58.661457430917515 92.86882716916688 81.92305418766816 63.053206475370615 85.96845864560721 94.07378384127738 96.55410383206664 97.35655749852971 82.74034187947362 17.658891684800132 15.982584430696933 32.95562442470049 90.85196755261097 94.99246655520517 63.206779141782654]
[0 0 0 0 0 0 0 0 0 0 0 0 0 180.4454934285715 179.86045550476197 179.67368623746037 179.25285553913233 179.01074306724803 179.37763545828162 179.68685033051074 179.51822961977598 179.5147064704725 179.54918560774283 179.12243299337712 178.4360727275935 177.84248049724772 177.30801643094802 176.5433187734883 176.35221987035652 176.58067628764232 177.16648931595668 177.57536167382912 177.91846078398524 178.56359574612054 178.81496537997114 179.14290812930832 179.7198599787339 180.16359224823603 180.35675394847124 181.0933806220084 181.84313280574062 182.59049909830853 183.31953361853405 183.9713750027295 184.2623295356989 184.6370934642724 184.98190033570273 185.68356695760903 186.25915642992783 186.71671237260412 187.25963138959023 187.8915482709782 188.53975890151443 189.13800584797917 189.64265600158194 189.910260401371 190.13086621452155 190.337149919252 190.75233046335174 191.22533106823818 191.57239065913976]
◼︎コード
package main
import (
"fmt"
"github.com/markcheno/go-quote"
"github.com/markcheno/go-talib"
)
func main() {
spy, _ := quote.NewQuoteFromYahoo("spy", "2016-01-01", "2016-04-01", quote.Daily, true)
fmt.Print(spy.CSV())
// RSIの結果を表示
rsi2 := talib.Rsi(spy.Close, 2)
fmt.Println(rsi2)
// 14日前からClose時の売買平均を算出
mva := talib.Ema(spy.Close, 14)
fmt.Println(mva)
}
この記事が気に入ったらサポートをしてみませんか?