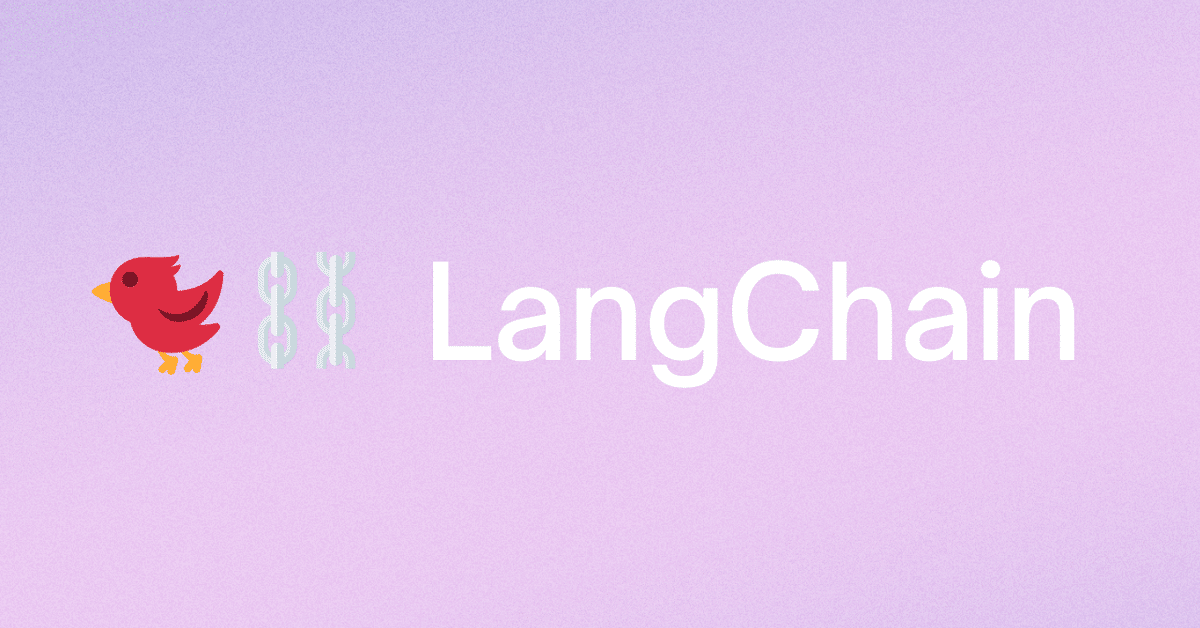
LangChain(Agent)でAIキャラ
LangChainは非常に簡単にGPTを利用したアプリケーションが作れる一方、自由度が低いです。
今後改善される可能性も全然ありますが、今回はドキュメントに乗っていないような内容や、ちょっと強引な内容を交えLangChainをカスタマイズします
強引なため、動作は保証できません。
conversational-react-description、つまりReAct+会話を例として取り上げます
最終的にこんな風にキャラクー性を吹き込ませながら、ReActができるようになったりします。
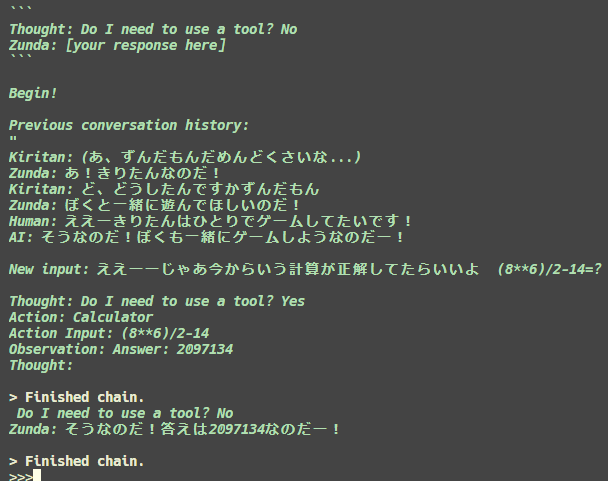
前提として普通の呼び出し方
ツール、メモリ、モデルを定義
エージェントのインスタンス作成
入力して実行(outにレスポンスが代入される)
from langchain.agents import Tool
from langchain.chains.conversation.memory import ConversationBufferMemory
from langchain import OpenAI
from langchain.utilities import GoogleSearchAPIWrapper
from langchain.agents import initialize_agent
# ツールの準備
search = GoogleSearchAPIWrapper()
tools = [
Tool(
name = "Current Search",
func=search.run,
description="アニメや漫画の質問に答える必要がある場合に役立ちます"
),
]
# メモリの準備
memory = ConversationBufferMemory(memory_key="chat_history")
# エージェントの準備
llm=OpenAI(temperature=0)
agent= initialize_agent(
tools,
llm,
agent="conversational-react-description",
verbose=True,
memory=memory
)
out = agent.run(input="こんにちは!今日も寒いですね")
> Entering new AgentExecutor chain...
Thought: Do I need to use a tool? No
AI: こんにちは!そうですね、今日はとても寒いですね。
> Finished chain.
'こんにちは!そうですね、今日はとても寒いですね。
プロンプトをカスタマイズする方法
以下のような形式でagentの設定を書ける
agent_kwargs = {"prefix": "Assistant is a large language model trained by OpenAI.\\n\\nAssistant is designed to be able to assist with a wide range of tasks, from answering simple questions to providing in-depth explanations and discussions on a wide range of topics. As a language model, Assistant is able to generate human-like text based on the input it receives, allowing it to engage in natural-sounding conversations and provide responses that are coherent and relevant to the topic at hand.\\n\\nAssistant is constantly learning and improving, and its capabilities are constantly evolving. It is able to process and understand large amounts of text, and can use this knowledge to provide accurate and informative responses to a wide range of questions. Additionally, Assistant is able to generate its own text based on the input it receives, allowing it to engage in discussions and provide explanations and descriptions on a wide range of topics.\\n\\nOverall, Assistant is a powerful tool that can help with a wide range of tasks and provide valuable insights and information on a wide range of topics. Whether you need help with a specific question or just want to have a conversation about a particular topic, Assistant is here to assist.\\n\\nTOOLS:\\n------\\n\\nAssistant has access to the following tools:{test}",
"suffix": "Begin!\\n\\nPrevious conversation history:\\n{chat_history}\\n\\nNew input: {input}\\n{agent_scratchpad}",
"format_instructions": "To use a tool, please use the following format:\n\n```\nThought: Do I need to use a tool? Yes\nAction: the action to take, should be one of [{tool_names}]\nAction Input: the input to the action\nObservation: the result of the action\n```\n\nWhen you have a response to say to the Human, or if you do not need to use a tool, you MUST use the format:\n\n```\nThought: Do I need to use a tool? No\n{ai_prefix}: [your response here]\n```",
"ai_prefix": "AI",
"human_prefix": "Human",
"input_variables": ["input", "chat_history", "agent_scratchpad", "test"]}
これを以下のように入力する
agent = initialize_agent(tools,
llm,
agent="conversational-react-description",
memory=memory,
agent_kwargs=agent_kwargs, # これを追加する
verbose=True)
プロンプトの文法
prefix(接頭語)
promptの最初に常にくっつける言葉
suffix(接尾辞)
agent_scratchpadといったシステム側で改変するプロンプトを含んでくる、常にくっつける言葉
{agent_scratchpad}は絶対に含んでいる必要があり、システムがここにいろいろな言葉を代入してくれる
format_instructions
回答のフォーマットを示す文
ai_prefix
AIの名前
human_prefix
人間の名前
input_variables
prefixとsuffixに含まれる、.runを実行時に入力できる変数
prefixとsuffix内において変数は{hoge}で表現される。
以下のように複数変数入力できる
agent.run(input1="入力1", input2="入力2")
見やすいようにyaml形式で書いています
prefix: |-
Assistant is a large language model trained by OpenAI.
Assistant is designed to be able to assist with a wide range of tasks, from answering simple questions to providing in-depth explanations and discussions on a wide range of topics. As a language model, Assistant is able to generate human-like text based on the input it receives, allowing it to engage in natural-sounding conversations and provide responses that are coherent and relevant to the topic at hand.
Assistant is constantly learning and improving, and its capabilities are constantly evolving. It is able to process and understand large amounts of text, and can use this knowledge to provide accurate and informative responses to a wide range of questions. Additionally, Assistant is able to generate its own text based on the input it receives, allowing it to engage in discussions and provide explanations and descriptions on a wide range of topics.
Overall, Assistant is a powerful tool that can help with a wide range of tasks and provide valuable insights and information on a wide range of topics. Whether you need help with a specific question or just want to have a conversation about a particular topic, Assistant is here to assist.
TOOLS:
------
Assistant has access to the following tools:
suffix: |-
Begin!
Previous conversation history:
{chat_history}
New input1: {input1}
New input2: {input2}
{agent_scratchpad}
format_instructions: |-
To use a tool, please use the following format:
```
Thought: Do I need to use a tool? Yes
Action: the action to take, should be one of [{tool_names}]
Action Input: the input to the action
Observation: the result of the action
```
When you have a response to say to the Human, or if you do not need to use a tool, you MUST use the format:
```
Thought: Do I need to use a tool? No
{ai_prefix}: [your response here]
```
ai_prefix: AI
human_prefix: Human
input_variables: ["input", "chat_history", "agent_scratchpad"]
メモリを最初から入力して初期化する方法
以下の方法で初期化できる
agent.memory.buffer = '\\nHuman: Hello!\\nAI: Hi there! How can I help you?\\nHuman: Calculate the following equation 5**4-24\\nAI: The answer to your equation is 625.'
生成前にLLMに入力されるプロンプトを表示させる
以下を実行すると設定できる
agent.agent.llm_chain.verbose = True
この記事が気に入ったらサポートをしてみませんか?