Streamlit 複数ページ multipage
Streamlitのバージョン1.10.0以降では、マルチページアプリケーションの作成がサポートされています。
方法はいくつかあります。
一番簡単なのはプロジェクト内にpagesというディレクトリを作成するとその中のスクリプトファイルへの移動できるサイドバーを勝手に作ってくれる。欠点は細かいカスタマイズをするときは不便。
手順
1. ディレクトリ構造の準備:
まず、プロジェクトディレクトリを設定し、その中にpagesディレクトリを作成します。このpagesディレクトリには、各ページのスクリプトファイルを配置します。
myapp
├── main.py
└── pages
├── about.py
└── myprofile.py
2. メインページ(main.py)の作成:
これはアプリケーションのメインエントリーポイントです。このファイルには、アプリケーション全体の設定やナビゲーションメニューが含まれます。
import streamlit as st
st.set_page_config(page_title="Streamlit App", page_icon=":shark:")
st.title("This is a title")
st.write("Hello World!")
st.markdown("""## This is a markdown
### Streamlit is awesome!
マークダウンでかけて便利!
- リスト
> 引用
```python
import streamlit as st
st.title("This is a title")
st.write("Hello World!")
```
""")
main.pyを作成した段階では以下のような画面となる
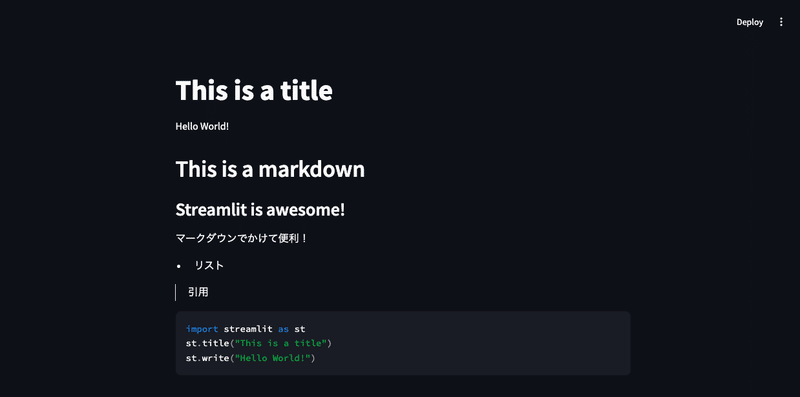
3. 別のページスクリプトの作成:
pagesディレクトリ内に各ページのスクリプトを作成します。ファイル名の先頭に番号を付けると、ナビゲーションメニューでの表示順序を制御できます。
• myprofile.py:
import streamlit as st
st.markdown("""## My Profile
my name is **クラッシャーバンバンFuji**
my favorite programming language is <strong>Python</strong>
My Special moves are F-U and DDT.
Streamlit is awesome
Here is Streamlit's [official website](https://streamlit.io/)
""")
• about.py:
import streamlit as st
st.markdown("""## About
This WebApp is created by **クラッシャーバンバンFuji**.
This WebApp is created for the purpose of learning Streamlit.
I hope you enjoy this WebApp.
""")
4. アプリケーションの実行:
ターミナルからStreamlitアプリケーションを実行します。
streamlit run main.py
左側にサイドバーが生成される
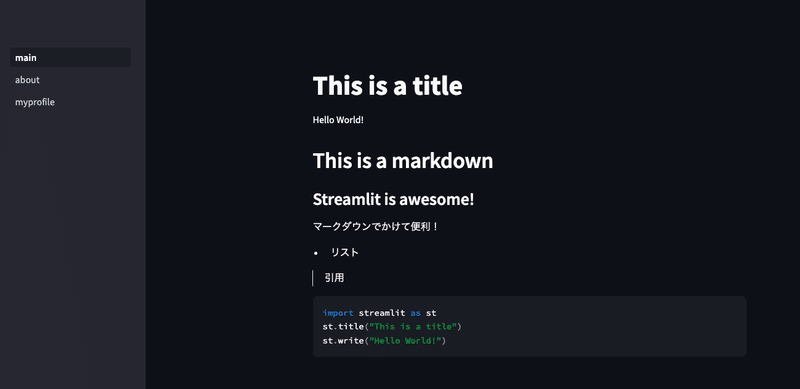
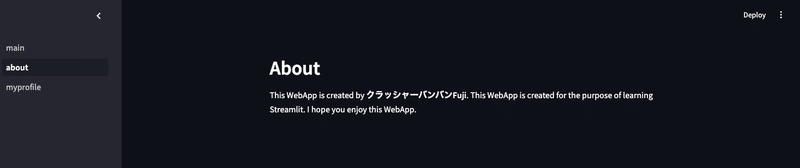
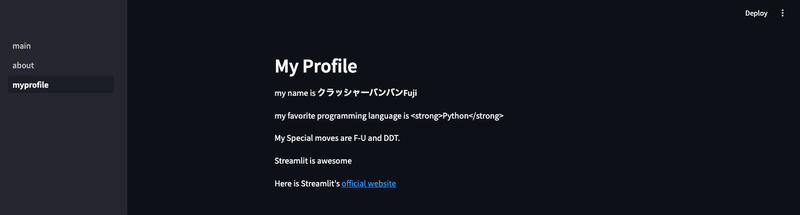
注意点
• ページファイルの命名:
ファイル名に番号を付けることで、ナビゲーションメニューでのページの順序を制御できます。
myapp
├── main.py
└── pages
├── 1_myprofile.py
└── 2_about.py
上記のように先頭に番号を振ってあげる
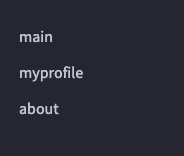
カスタマイズをしたければnavigationを使う
pagesをなくしてみる
myapp
├── contents
│ ├── about.py
│ ├── config.py
│ ├── myprofile.py
│ └── top_page.py
└── main.py
もちろん、上記のようにすればサイドバーはなくなる
例えばサイドバーに表示したいページを制限したいときはst.Pageとst.navigationを使う
config.pyは表示したくないとかあった場合は以下のようにmain.pyを書き換える
main.pyを書き換える
import streamlit as st
st.set_page_config(page_title="Streamlit App", page_icon=":shark:")
top_page = st.Page(page="contents/top_page.py", title="Top", icon=":material/home:")
my_profile = st.Page(page="contents/myprofile.py", title="自己紹介ぺージ", icon=":material/open_with:")
about = st.Page(page="contents/about.py", title="About", icon=":material/apps:")
config = st.Page(page="contents/config.py", title="Config", icon=":material/settings_applications:")
pg = st.navigation([top_page, my_profile, about])
pg.run()
# top_page.py
import streamlit as st
st.title("This is a title")
st.write("Hello World!")
st.markdown("""## This is a markdown
### Streamlit is awesome!
マークダウンでかけて便利!
- リスト
> 引用
```python
import streamlit as st
st.title("This is a title")
st.write("Hello World!")
```
""")
# myprofile.py
import streamlit as st
st.markdown("""## My Profile
my name is **クラッシャーバンバンFuji**
my favorite programming language is <strong>Python</strong>
My Special moves are F-U and DDT.
Streamlit is awesome
Here is Streamlit's [official website](https://streamlit.io/)
""")
# about.py
import streamlit as st
st.markdown("""## About
This WebApp is created by **クラッシャーバンバンFuji**.
This WebApp is created for the purpose of learning Streamlit.
I hope you enjoy this WebApp.
""")
# config.py このページは表示したくない
import streamlit as st
st.markdown("""## About
This page is secret page.
I don't want to show this page to everyone.
""")
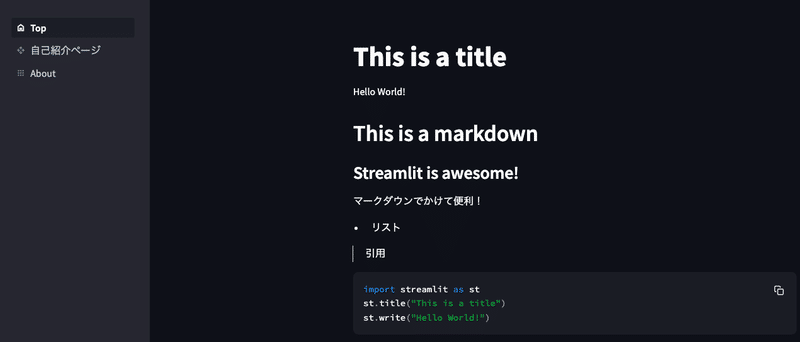
解説
ページオブジェクトの定義:
top_page = st.Page(page="contents/top_page.py", title="Top", icon=":material/home:")
my_profile = st.Page(page="contents/myprofile.py", title="自己紹介ぺージ", icon=":material/open_with:")
about = st.Page(page="contents/about.py", title="About", icon=":material/apps:")
config = st.Page(page="contents/config.py", title="Config", icon=":material/settings_applications:")
st.Pageを使って各ページを定義します。pageにはページのPythonファイルのパスを、titleにはページのタイトルを、iconにはアイコンを設定します。 ナビゲーションの設定: 表示したくないconfigはnavigationには入れない
pg = st.navigation([top_page, my_profile, about])
st.navigationを使ってナビゲーションオブジェクトを作成します。ページオブジェクトのリストを渡します。
ナビゲーションの実行:
pg.run()
pg.run()を呼び出すことで、ナビゲーションを実行し、ユーザーがページ間を移動できるようにします。 重要なポイント st.Pageとst.navigationを使うことで、アプリケーションのページ構造を動的に定義し、ユーザーが簡単にページ間を移動できるようになります
WebAppの最初のページはst.navigation()に渡すリストの最初のst.Pageオブジェクトが最初のページとなる
TopページのURL

自己紹介ページ

挙動に関して
st.navigationに渡すst.Pageオブジェクトが一つだとサイドバーは生成されない
pg = st.navigation([top_page])
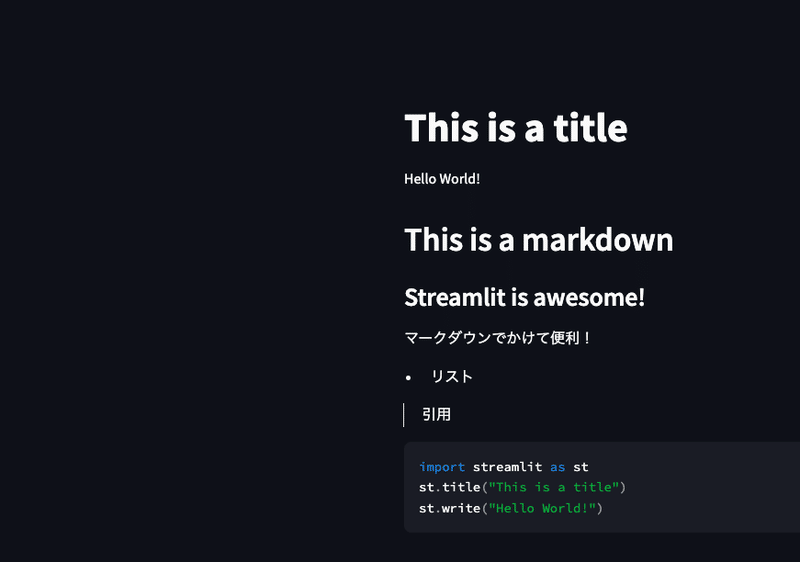
この記事が気に入ったらサポートをしてみませんか?