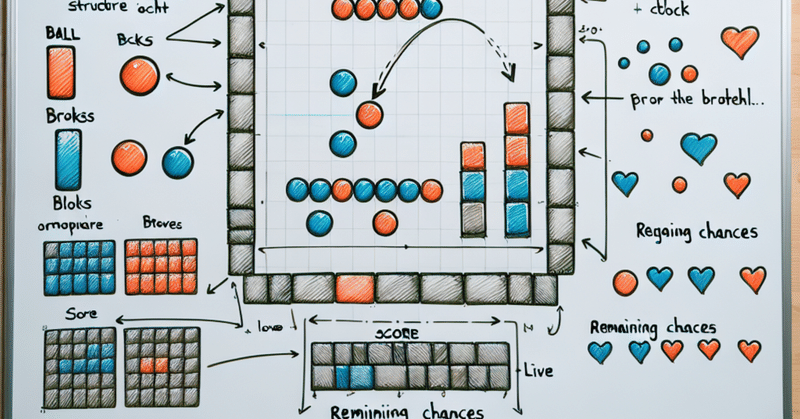
作りたいゲームを指示すると概念図を作ってから概念図に基づいてゲームの開発計画を立て、自動的にプログラミングするアプリを作ろうとした #GPTハッカソン24耐
tldrawに影響されて、なんとかこの性質を応用できないか考えたところ、言葉から概念図をまず作り、それに基づいて計画を立て、長いプログラム(128Kトークンを活用)を書かせるようなアプリを作りました。
GPT4でDALL-Eのプロンプトを書き、DALL-Eが概念図を描き、それをGPT-4Vが読み込んで開発計画を立て、GPT-4がゲーム本体を生成するという段取りです。
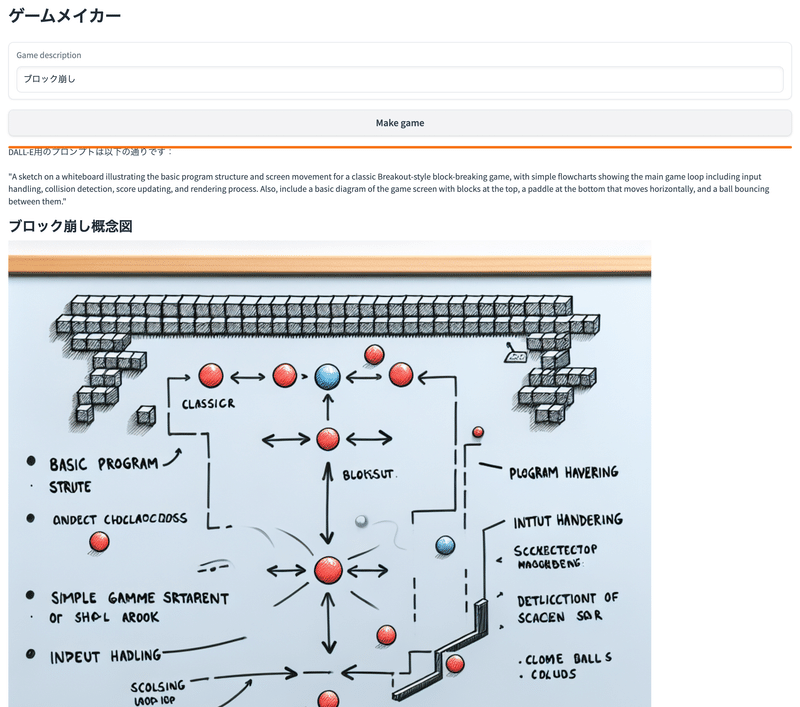
例えばブロック崩しだとまず以下のようなプロンプトが生成されます。
DALL-E用のプロンプトは以下の通りです:
"A sketch on a whiteboard illustrating the basic program structure and screen movement for a classic Breakout-style block-breaking game, with simple flowcharts showing the main game loop including input handling, collision detection, score updating, and rendering process. Also, include a basic diagram of the game screen with blocks at the top, a paddle at the bottom that moves horizontally, and a ball bouncing between them."
そこからDALL-E3で概念図が生成されました
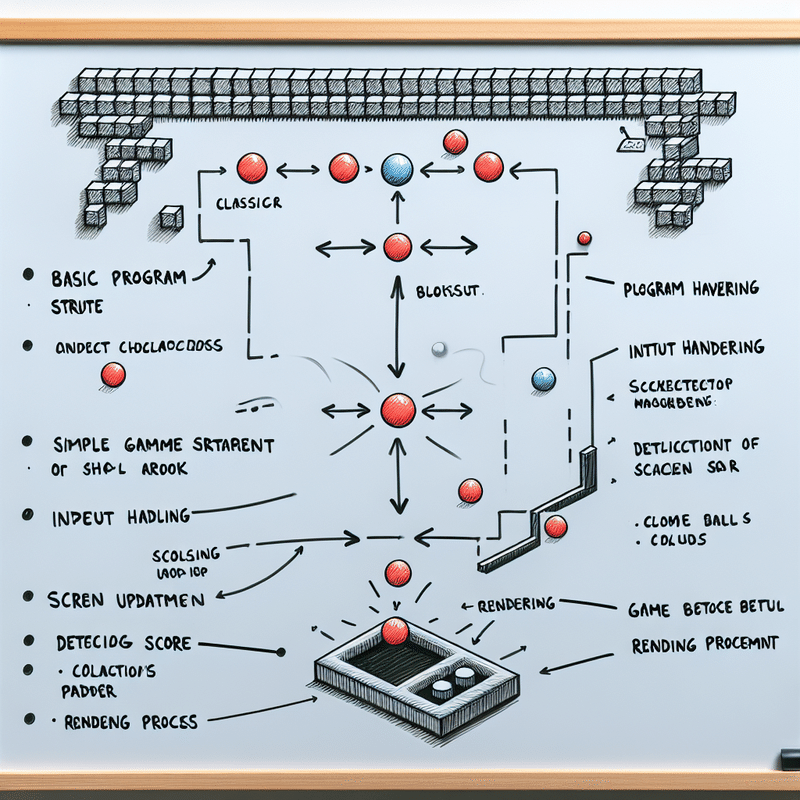
この図をGPT-4Vで解釈して開発計画を立てます。
この画像はブロック崩しゲームのコンセプトを示しており、Pythonでゲームを作成するために必要なコンポーネントとその関係性を概念的に説明しています。この概念図を元に、プログラム計画を立案してみましょう。
1. 基本プログラム構造 (Basic Program Structure)
- メインループの設定
- ゲーム状態(スタート画面、ゲーム中、ゲームオーバー)の管理
2. ゲームの要素の初期化 (Simple Game Statement or Setup)
- ブロック、ボール、パドルのオブジェクトの定義
- ゲームのパラメータ(スコア、ライフなど)の設定
3. 入力処理 (Input Handling)
- ユーザーからの入力(キーボード、マウスなど)に応じてパドルを動かす
- ゲームを制御するための入力(ポーズ、リスタートなど)
4. ゲームロジックの更新 (Screen Update)
- ボールの動きの計算
- ボールがブロックに当たったときの処理
- ゲームの状態(スコア、ゲームオーバーなど)の更新
5. スコアの検出と計算 (Detecting Score and Calculation's Part)
- ブロックを壊した際のスコアの加算
- スコアボードの更新
6. レンダリングプロセス (Rendering Process)
- 画面にゲームの状態を描画
- ブロック、ボール、パドルなどのグラフィックの表示
Pythonには様々なゲーム開発ライブラリがありますが、ここでは`pygame`というライブラリを使用することを想定しています。以下は`pygame`を用いたブロック崩しゲームのための簡単なプログラムの骨組みです:
これをGPT4でロングコンテキストなプログラムにしていきます
```python
import pygame
import sys
# 初期化
pygame.init()
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
clock = pygame.time.Clock()
# ゲームのオブジェクト定義
# パドル、ボール、ブロックなど...
# ゲームループ
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 入力処理
# キー入力によるパドルの移動など...
# ゲームロジックの更新
# ボールの動き、衝突判定など...
# スコア計算
# 画面の描画
screen.fill((0, 0, 0)) # 画面を黒でクリア
# パドル、ボール、ブロックの描画など...
pygame.display.flip() # 描画内容を画面に表示
clock.tick(60) # FPSを60に
# 終了処理
pygame.quit()
sys.exit()
```
上記は非常に基礎的な骨組みであり、ゲームの具体的な詳細や機能は、このコードを基にしてさらに追加・拡張する必要があります。さて、`pygame`を使用して基本的なブロック崩しゲームを作成するプランを詳しく見ていきましょう。以下にゲームの基本的な構造を示すコードを示します:
```python
import pygame
import sys
# 定数
WIDTH, HEIGHT = 800, 600
FPS = 60
PADDLE_WIDTH, PADDLE_HEIGHT = 100, 20
BALL_SIZE = 20
BLOCK_WIDTH, BLOCK_HEIGHT = 60, 20
# カラー
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
BLUE = (0, 0, 255)
RED = (255, 0, 0)
# パドルクラス
class Paddle:
def __init__(self):
self.width = PADDLE_WIDTH
self.height = PADDLE_HEIGHT
self.x = (WIDTH - self.width) / 2
self.y = HEIGHT - self.height - 10
self.color = WHITE
self.speed = 10
def draw(self, screen):
pygame.draw.rect(screen, self.color, (self.x, self.y, self.width, self.height))
def move(self, movement):
self.x += movement * self.speed
if self.x < 0:
self.x = 0
elif self.x + self.width > WIDTH:
self.x = WIDTH - self.width
# ボールクラス
class Ball:
def __init__(self):
self.size = BALL_SIZE
self.x = WIDTH / 2 - self.size / 2
self.y = HEIGHT / 2 - self.size / 2
self.color = WHITE
self.x_speed = 5
self.y_speed = -5
def draw(self, screen):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.size)
def move(self):
self.x += self.x_speed
self.y += self.y_speed
# 壁の衝突判定
if self.x <= 0 or self.x >= WIDTH - self.size:
self.x_speed *= -1
if self.y <= 0:
self.y_speed *= -1
# TODO: パドルやブロックとの衝突判定
# ブロッククラス
class Block:
def __init__(self, x, y):
self.width = BLOCK_WIDTH
self.height = BLOCK_HEIGHT
self.x = x
self.y = y
self.color = BLUE
def draw(self, screen):
pygame.draw.rect(screen, self.color, (self.x, self.y, self.width, self.height))
# ゲーム初期化
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('ブロック崩し')
clock = pygame.time.Clock()
# ゲームオブジェクトの初期化
paddle = Paddle()
ball = Ball()
blocks = [Block(x * BLOCK_WIDTH, y * BLOCK_HEIGHT) for y in range(5) for x in range(WIDTH // BLOCK_WIDTH)]
# メインループ
running = True
while running:
screen.fill(BLACK)
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
paddle.move(-1)
if keys[pygame.K_RIGHT]:
paddle.move(1)
# ボールの動きと衝突判定
ball.move()
# TODO: パドルやブロックとの衝突判定
# スコアとゲームオーバーの検出
# TODO: スコア計算とゲームオーバー処理
# 描画
paddle.draw(screen)
ball.draw(screen)
for block in blocks:
block.draw(screen)
pygame.display.flip() # 画面更新
clock.tick(FPS) # FPSを60に
# 終了処理
pygame.quit()
sys.exit()
```
このコードはまだ完全ではありませんが、ゲームオブジェクトの初期化、メインループでの画面描画とオブジェクトの更新、ユーザーの入力処理が含まれています。衝突の検出と処理、スコアの計算は、コメントで示された`TODO`部分に実装する必要があります。
上記の骨組みをベースにしてブロック崩しのゲームを完成させることができます。各クラスを拡張し、ゲームの論理を組み込んでいき、さらにユーザーインターフェースを改善していくことで、フル機能のブロック崩しゲームに発展させることができます。
Pythonを生成させようとするとインデントが消えることが多いので自動生成にはC++やJavaScriptのような言語が向いているのかもしれないと思いました。
tldrawが上手くいくのは、多分Webサイトというかなり限定された部品だからなのかもしれません。
試しにtldraw make realのプロンプトを参考にしてみます。
DALL-E3へのプロンプト
"A prompt for DALL-E to create a simple whiteboard-style diagram illustrating the program structure and screen movement for a Breakout game. The diagram should depict the paddle, ball, and bricks layout along with arrows showing the ball's movement, and should include labeled blocks representing the main components of the game logic such as 'Input Handling', 'Physics', 'Collision Detection', 'Score Management', and 'Game State Control'."
これに従って作られた概念図
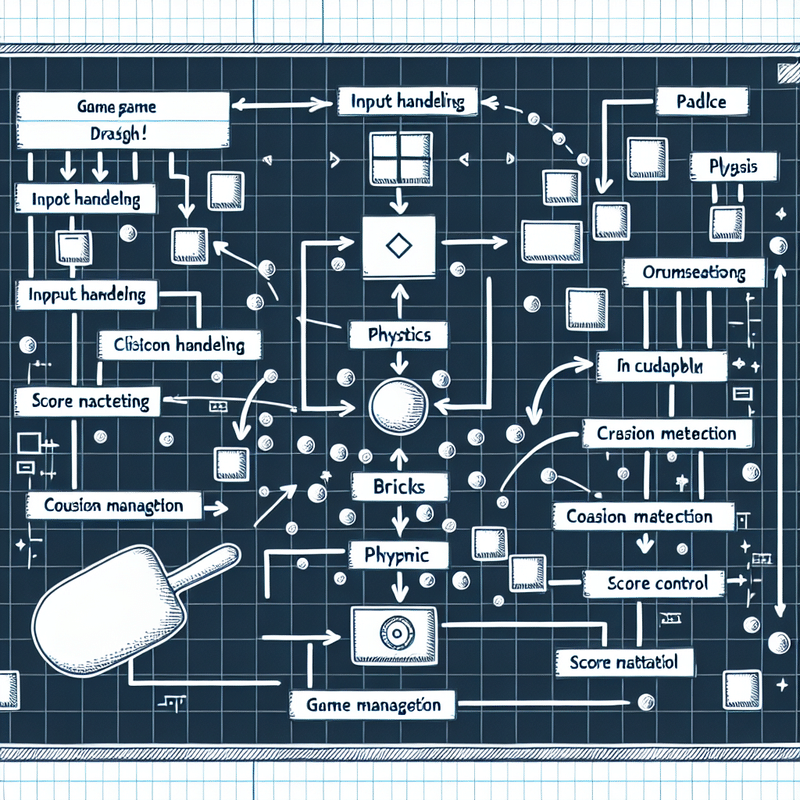
これを受けて立てられた計画
The image you've shared appears to depict a concept design for a game, drawn on a whiteboard with various annotations. The design suggests a game that includes elements such as a paddle, ball, and an array of blocks that likely need to be broken or passed by the ball when it is hit by the paddle.
There are different terms on the whiteboard, some of which seem to be playful variations or misspellings of words commonly associated with brick-breaking or breakout-style games. For example, "PADDLE" is written as "PLADLE," "BALL" is "BACLLE," and "BLOCKS" appears as "BLOOCKS."
If you're planning to implement this in JavaScript, you'll likely be creating a game where the paddle is moved horizontally along the bottom of the playing area to keep the ball moving upwards to hit and remove blocks. Implementing collision detection will be important so the game knows when the ball hits the paddle, blocks, or walls. Additionally, considerations for ball movement, physics, and possibly power-ups or special gameplay mechanics might be derived from your concept design.
JavaScript's capabilities in handling animation, user input, and game logic make it a good choice for creating such a game. Using HTML5's canvas element can facilitate the drawing of the game elements and animation frames. Good luck with your project!
この後色々やったが上手くいかなかった。
明日の朝大学の授業をやらないといけないので今日はこの辺で一旦寝る