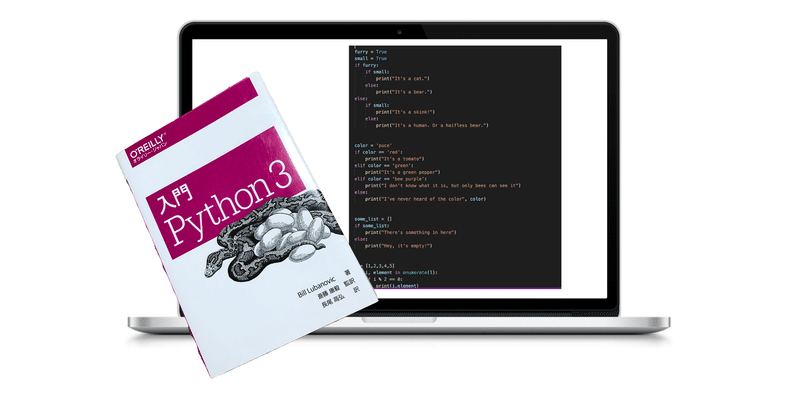
Python3 自習・復習vol.012
今日の投稿はだいぶ遅くなってしまいましたが・・・
関数
・処理をひとまとめにしたもの
・名前をつけて、再実行を可能にする
・関数にパラメータとして引数を渡すこと(入力)ができて、結果を返すこと(出力)が出来る。
関数名
・関数に付与する名前で、実行の際にはその名前で呼び出す。
・使用可能な名前の文字列は、変数名と同じで、先頭に数字や記号は使えないが、数字は先頭以外・記号は( _ )のみ使用可能。*日本語も使えるが基本的には使われていない。
関数の処理
・関数内で処理(加算・四則演算・変数の代入・条件分岐・繰返しなど)を実行させる。
・1行でも複数の処理でも実行することが可能
・関数内で関数を実行することも可能
引数
・関数内で使う値を、入力値として渡すもので、何個でも構わない。
・文字列や数値など使用可能だが、なくても構わない。
・変数に代入可能なもの(Pythonのオブジェクトであれば何でも)は使用可能。
戻り値
・処理の結果などを返すもので、文字列や数値などを結果として返すことができる。
・変数にい入れられるものなら何でも返せ、数は無制限で、複数返すことが可能だが、戻り値を明示しない場合は、何もないことを示す 'None' が返る。
#関数を定義する方法
def add_tax(price): #add_taxが関数名でpriceが引数・パラメータ
tax_rate = 0.1 #関数を表すブロックはインデントで示す
tax = price * tax_rate
total = price + tax
return total #戻り値は return戻り値
#関数を使う方法
price = 100
total = add_tax(price)
print(total)
110.0
#引数がない関数や戻り値がない関数も作れる
def say_hello_ten():
for i in range(10):
print('hello')
say_hello_ten()
hello
hello
hello
hello
hello
hello
hello
hello
hello
hello
#複数の引数を与えることもできる
def say_something_many(thing, times): #仮引数(parameter)
for i in range(times):
print(thing)
say_something_many('Ouch!', 5) #実引数(argument)
Ouch!
Ouch!
Ouch!
Ouch!
Ouch!
#天気予報をランダムに表示する関数を作る import random
weathers = ['晴れ', '曇り', '雨']
def todays_weather():
weather = random.randint(0,2)
print('今日の天気は', weathers[weather], 'です')
todays_weather()
今日の天気は 雨 です
今日の天気は 雨 です
今日の天気は 晴れ です
今日の天気は 曇り です
今日の天気は 雨 です
#現在時刻を表示する関数を作る import datetime
def what_time_is_it_now():
time = datetime.datetime.now().strftime('%Y.%m.%d-%H:%M')
print(time)
what_time_is_it_now()
2021.08.21-11:37
#特定の日付から現在までの経過日数を返す関数を作る import datetime
def elapsed_days_to_day():
the_day = datetime.datetime(2000,1,1)
today = datetime.datetime.now()#.strftime('%Y.%m.%d')
print('特定の日(', the_day, ')から現在までの経過日数は', (the_day - today).days, '日です')
elapsed_days_to_day()
特定の日( 2000-01-01 00:00:00 )から現在までの経過日数は 7904 日です
#特定の日から現在までの年数を返す関数 import datetime
def elapsed_years():
the_day = datetime.datetime(1980,5,1)
today = datetime.datetime.now()
print(the_day, 'から現在までの年数は', ((today - the_day) / 365).days, '年です')
elapsed_years()
2000-01-01 00:00:00 から現在までの年数は 21 年です
最後までお読みいただき、本当にありがとうございます。 明日も継続して学習のアウトプットを続けていきたいと思いますので、また覗きにきていただけると嬉しいです! 一緒に学べる仲間が増えると、もっと喜びます!笑 これからも宜しくお願い致しますm(__)m