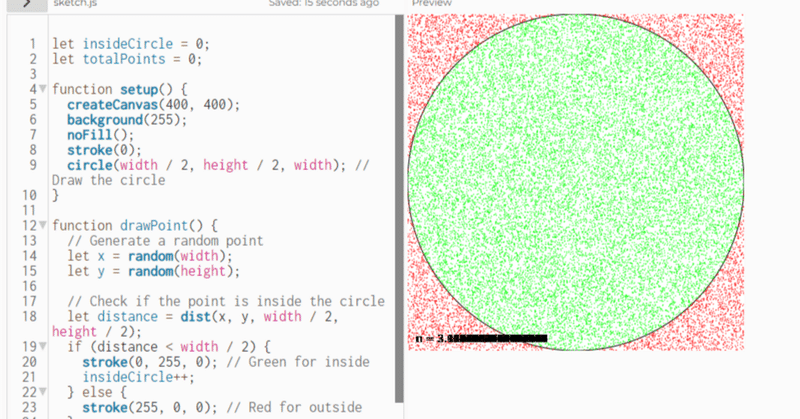
🎲モンテカルロ法もp5で再現できますか?
モンテカルロ法を使って円周率(π)を推定するプログラムも、p5.jsを使用して実装できます。この方法では、単位円内とその外側にランダムに点を打ち、円内に入った点の比率からπの値を推定します。
以下のステップでモンテカルロ法を用いたπの推定を行います:
描画領域を準備する:正方形のキャンバスを作成し、その一部に単位円を描画します。
ランダムな点を打つ:正方形の領域内にランダムに点を打ちます。
点が円内にあるか判定する:各点が円の中にあるか外にあるかを判定します。点の座標を(x, y)とすると、x^2 + y^2 <= 1ならばその点は円内にあると言えます。
πの値を推定する:円内に入った点の割合は、正方形の面積に対する単位円の面積の割合に相当します。正方形の面積は4倍の単位円の面積なので、円内に入った点の割合を4倍することでπの近似値を得られます。
これを実装するための基本的なp5.jsのコードスケッチを作成しましょう。
以下のコードはp5.jsを使用してモンテカルロ法による円周率(π)の推定を行うものです。このプログラムでは、正方形のキャンバス内に単位円を描画し、ランダムに点を打ち、それらが円内に入る割合からπの近似値を計算します。
let insideCircle = 0;
let totalPoints = 0;
function setup() {
createCanvas(400, 400);
background(255);
noFill();
stroke(0);
circle(width / 2, height / 2, width); // Draw the circle
}
function drawPoint() {
// Generate a random point
let x = random(width);
let y = random(height);
// Check if the point is inside the circle
let distance = dist(x, y, width / 2, height / 2);
if (distance < width / 2) {
stroke(0, 255, 0); // Green for inside
insideCircle++;
} else {
stroke(255, 0, 0); // Red for outside
}
point(x, y);
// Update total points
totalPoints++;
}
function draw() {
for (let i = 0; i < 100; i++) { // Increase for more precision
drawPoint();
}
// Estimate Pi
let piEstimate = (insideCircle / totalPoints) * 4;
fill(0);
noStroke();
text(`π ≈ ${piEstimate}`, 10, height - 10);
}
function mouseClicked() {
insideCircle = 0; // Reset inside circle count
totalPoints = 0; // Reset total points
clear();
background(255);
circle(width / 2, height / 2, width); // Redraw the circle
}
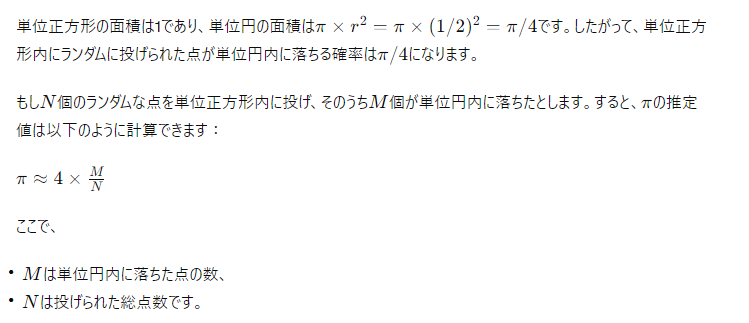
この式は、モンテカルロ法を用いてπを推定する際の基本的な数学的表現です。この方法の精度は、点の数Nが増えるにつれて向上します。モンテカルロ法の美しさは、そのシンプルさと、複雑な形状や高次元の問題にも容易に適用できる汎用性にあります。
お願い致します