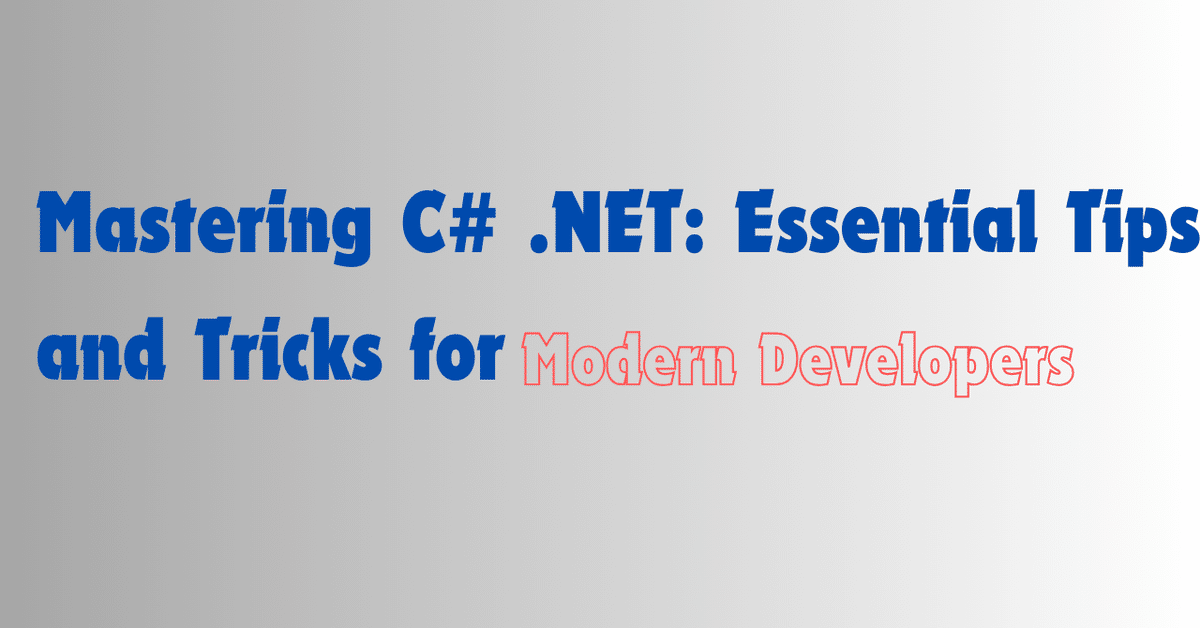
Mastering C# .NET: Essential Tips and Tricks for Modern Developers
C# .NET remains one of the most powerful and versatile frameworks for modern application development. As a robust language backed by Microsoft, C# .NET is widely used in various domains, from web and desktop applications to mobile and cloud-based solutions. Whether you're a beginner or a seasoned developer, there's always something new to learn. Here are some essential tips and tricks to help you master C# .NET and enhance your development skills.
1. Leverage LINQ for Efficient Data Queries
Language Integrated Query (LINQ) is one of the most powerful features in C#. It allows you to query collections in a declarative manner, similar to SQL. LINQ can significantly reduce the complexity and increase the readability of your code.
Example:
var filteredList = myList.Where(item => item.Age > 18).OrderBy(item => item.Name).ToList();
By using LINQ, you can perform complex queries on collections with minimal code.
2. Use Asynchronous Programming
Asynchronous programming is crucial for creating responsive applications. The async and await keywords in C# make it easier to write asynchronous code, improving the performance of your applications, especially those that involve I/O operations.
Example:
public async Task<string> GetDataAsync(string url)
{
using (var client = new HttpClient())
{
return await client.GetStringAsync(url);
}
}
Asynchronous methods help in keeping the UI responsive by not blocking the main thread.
3. Master Exception Handling
Proper exception handling is vital for creating robust applications. Use try-catch blocks to handle exceptions gracefully and ensure that your application can recover or fail gracefully.
Example:
try
{
// Code that may throw an exception
}
catch (Exception ex)
{
// Handle the exception
Console.WriteLine($"An error occurred: {ex.Message}");
}
finally
{
// Cleanup code
}
Using specific exception types in your catch blocks can provide more precise control over error handling.
4. Utilize the .NET Core CLI
The .NET Core Command-Line Interface (CLI) is a cross-platform toolchain for developing, building, running, and publishing .NET applications. Familiarize yourself with common commands like dotnet new, dotnet build, dotnet run, and dotnet publish to streamline your workflow.
Example:
dotnet new console -n MyConsoleApp
cd MyConsoleApp
dotnet run
The CLI tools can significantly speed up your development process by automating common tasks.
5. Optimize Performance with Span<T> and Memory<T>
For performance-critical applications, Span<T> and Memory<T> provide a way to work with contiguous memory regions efficiently. They help reduce allocations and improve performance, especially when working with large datasets or in scenarios requiring high performance.
Example:
Span<int> numbers = stackalloc int[100];
for (int i = 0; i < numbers.Length; i++)
{
numbers[i] = i;
}
Using Span<T> can help you write high-performance code by avoiding unnecessary heap allocations.
6. Take Advantage of Dependency Injection
Dependency Injection (DI) is a design pattern that helps create loosely coupled and testable code. .NET Core has built-in support for DI, making it easier to manage dependencies in your applications.
Example:
public class MyService
{
private readonly IMyDependency _dependency;
public MyService(IMyDependency dependency)
{
_dependency = dependency;
}
public void DoWork()
{
_dependency.PerformTask();
}
}
Using DI can improve the testability and maintainability of your applications by decoupling components.
7. Keep Up with the Latest Features
C# and .NET are constantly evolving. Stay updated with the latest features and improvements in the language and framework. Regularly check the official Microsoft documentation and participate in community forums to keep your skills current.
Recent Features to Explore:
Records
Pattern matching enhancements
Nullable reference types
Default interface methods
Staying current with the latest features ensures that you can leverage the full power of C# and .NET in your projects.
8. Write Unit Tests
Unit testing is essential for ensuring the quality and reliability of your code. Use frameworks like xUnit, NUnit, or MSTest to write and run unit tests for your applications.
Example with xUnit:
public class CalculatorTests
{
[Fact]
public void Add_ShouldReturnCorrectSum()
{
var calculator = new Calculator();
var result = calculator.Add(2, 3);
Assert.Equal(5, result);
}
}
Writing unit tests can help you catch bugs early and ensure that your code behaves as expected.
Mastering C# .NET requires a combination of understanding the language's core features, staying updated with new enhancements, and applying best practices in your development workflow. By leveraging these tips and tricks, you can improve your efficiency, write cleaner code, and build more robust applications. Happy coding!
この記事が気に入ったらサポートをしてみませんか?