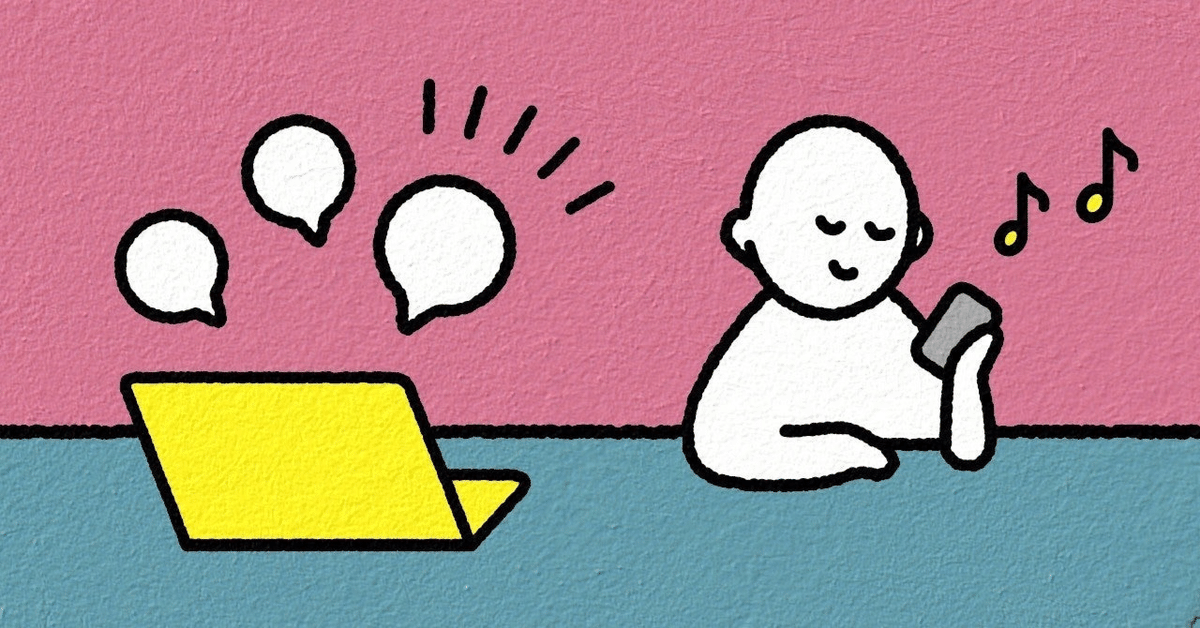
Photo by
nanahoshi_d
【ツール】ブラウザだけで画面録画をするツール その②
前に紹介したブラウザだけで特定のソフトを使わずに画面録画をするツールに指定時間で自動的に録画を停止する機能をつけてみました。
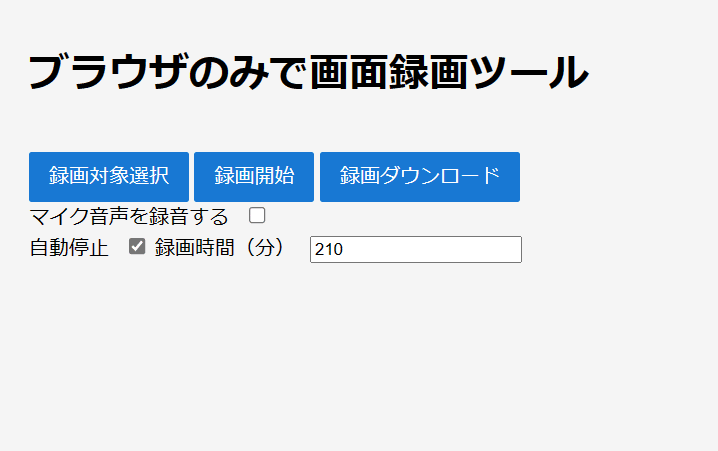
これでウェビナーを受講するときに録画ボタンを押した後、外に出てもOK(^^♪
指定時間後に停止するので延々録画することがなくなります。
使い方は以下のコードをメモ帳などで「ブラウザ録画.htm」など拡張子.htmなどで保存しEdgeやChromeで開くと使えます。
<!DOCTYPE html>
<html>
<head>
<title>ブラウザのみで画面録画ツール</title>
<style>
body {
font-family: Segoe UI, Arial, sans-serif;
padding: 1em;
background-color: #f5f5f5;
}
button {
margin-top: 1em;
padding: 0.5em 1em;
border: none;
background-color: #0078d7;
color: white;
border-radius: 2px;
font-size: 1em;
cursor: pointer;
}
button:disabled {
background-color: #a6a6a6;
}
label {
margin-right: 0.5em;
}
</style>
</head>
<body>
<h1>ブラウザのみで画面録画ツール</h1>
<button id="start">録画対象選択</button>
<button id="record" disabled>録画開始</button>
<button id="download" disabled>録画ダウンロード</button>
<br>
<label for="audio">マイク音声を録音する</label>
<input type="checkbox" id="audio">
<br>
<label for="autoStop">自動停止</label>
<input type="checkbox" id="autoStop">
<label for="duration">録画時間(分)</label>
<input type="number" id="duration" value="1" disabled>
<script>
let mediaRecorder;
let recordedBlobs;
let recordingTimeoutId;
const startButton = document.querySelector('button#start');
const recordButton = document.querySelector('button#record');
const downloadButton = document.querySelector('button#download');
const audioCheckbox = document.querySelector('input#audio');
const durationInput = document.querySelector('input#duration');
const autoStopCheckbox = document.querySelector('input#autoStop');
startButton.onclick = startScreenSharing;
recordButton.onclick = toggleRecording;
downloadButton.onclick = downloadRecording;
autoStopCheckbox.onchange = toggleDurationInput;
async function startScreenSharing() {
const screenStream = await navigator.mediaDevices.getDisplayMedia({video: true, audio: true});
let audioStream;
if (audioCheckbox.checked) {
audioStream = await navigator.mediaDevices.getUserMedia({audio: true});
} else {
audioStream = new MediaStream();
}
const tracks = [...screenStream.getTracks(), ...audioStream.getAudioTracks()]
const combinedStream = new MediaStream(tracks);
mediaRecorder = new MediaRecorder(combinedStream);
mediaRecorder.ondataavailable = (event) => {
if (event.data && event.data.size > 0) {
recordedBlobs.push(event.data);
}
};
recordButton.disabled = false; // 画面共有が開始されたら録画ボタンを有効にする
}
function toggleRecording() {
if (recordButton.textContent === '録画開始') {
startRecording();
recordButton.textContent = '録画停止';
} else {
stopRecording();
recordButton.textContent = '録画開始';
downloadButton.disabled = false;
}
}
function startRecording() {
recordedBlobs = [];
mediaRecorder.start();
downloadButton.disabled = true;
if (autoStopCheckbox.checked) {
const duration = Number(durationInput.value) * 60 * 1000; // Convert duration from minutes to milliseconds
recordingTimeoutId = setTimeout(stopRecording, duration);
}
}
function stopRecording() {
clearTimeout(recordingTimeoutId); // Ensure the timeout is cleared if recording is manually stopped
mediaRecorder.stop();
// Add these lines to update the button states when recording stops
recordButton.textContent = '録画開始';
downloadButton.disabled = false;
}
function downloadRecording() {
const blob = new Blob(recordedBlobs, {type: 'video/webm'});
const url = window.URL.createObjectURL(blob);
const a = document.createElement('a');
a.style.display = 'none';
a.href = url;
a.download = 'screen_recording.webm';
document.body.appendChild(a);
a.click();
setTimeout(() => {
document.body.removeChild(a);
window.URL.revokeObjectURL(url);
}, 100);
}
function toggleDurationInput() {
durationInput.disabled = !autoStopCheckbox.checked;
}
</script>
</body>
</html>
前回の記事はこちら
この記事が気に入ったらサポートをしてみませんか?