Python
What is Python?
Python is a popular programming language. It was created by Guido van Rossum, and released in 1991.
It is used for:
web development (server-side),
software development,
mathematics,
system scripting.
What can Python do?
Python can be used on a server to create web applications.
Python can be used alongside software to create workflows.
Python can connect to database systems. It can also read and modify files.
Python can be used to handle big data and perform complex mathematics.
Python can be used for rapid prototyping, or for production-ready software development.
Why Python?
Python works on different platforms (Windows, Mac, Linux, Raspberry Pi, etc).
Python has a simple syntax similar to the English language.
Python has syntax that allows developers to write programs with fewer lines than some other programming languages.
Python runs on an interpreter system, meaning that code can be executed as soon as it is written. This means that prototyping can be very quick.
Python can be treated in a procedural way, an object-oriented way or a functional way.
Good to know
The most recent major version of Python is Python 3, which we shall be using in this tutorial. However, Python 2, although not being updated with anything other than security updates, is still quite popular.
In this tutorial Python will be written in a text editor. It is possible to write Python in an Integrated Development Environment, such as , Pycharm, Netbeans or Eclipse which are particularly useful when managing larger collections of Python files.
Python Syntax compared to other programming languages
Python was designed for readability, and has some similarities to the English language with influence from mathematics.
Python uses new lines to complete a command, as opposed to other programming languages which often use semicolons or parentheses.
Python relies on indentation, using whitespace, to define scope; such as the scope of loops, functions and classes. Other programming languages often use curly-brackets for this purpose.
Python Install
To check if you have python installed on a Linux or Mac, then on linux open the command line or on Mac open the Terminal and type:
python --version
Python Quickstart
Python is an interpreted programming language, this means that as a developer you write Python (.py) files in a text editor and then put those files into the python interpreter to be executed.
Python Indentation
Indentation refers to the spaces at the beginning of a code line.
Where in other programming languages the indentation in code is for readability only, the indentation in Python is very important.
Python uses indentation to indicate a block of code.
Python Comments
❮Comments can be used to explain Python code.
Comments can be used to make the code more readable.
Comments can be used to prevent execution when testing code.
#print("Hello, World!")
print("Cheers, Mate!")
Creating a Comment
Comments starts with a #, and Python will ignore them:
#This is a comment
print("Hello, World!")
print("Hello, World!") #This is a comment
Multiline Comments
Python does not really have a syntax for multiline comments.
To add a multiline comment you could insert a # for each line:
Or, not quite as intended, you can use a multiline string.
Since Python will ignore string literals that are not assigned to a variable, you can add a multiline string (triple quotes) in your code, and place your comment inside it:
#This is a comment
#written in
#more than just one line
print("Hello, World!")
"""
This is a comment
written in
more than just one line
"""
print("Hello, World!")
Python Variables
Variables
Variables are containers for storing data values.
Creating Variables
Python has no command for declaring a variable.
A variable is created the moment you first assign a value to it.
Variables do not need to be declared with any particular type, and can even change type after they have been set.
x = 4 # x is of type int
x = "Sally" # x is now of type str
print(x)
Casting
If you want to specify the data type of a variable, this can be done with casting.
x = str(3) # x will be '3'
y = int(3) # y will be 3
z = float(3) # z will be 3.0
Get the Type
You can get the data type of a variable with the type() function.
x = 5
y = "John"
print(type(x))
print(type(y))
Single or Double Quotes?
String variables can be declared either by using single or double quotes:
x = "John"
# is the same as
x = 'John'
Case-Sensitive
Variable names are case-sensitive.
a = 4
A = "Sally"
#A will not overwrite a
Python - Variable Names
Variable Names
A variable can have a short name (like x and y) or a more descriptive name (age, carname, total_volume). Rules for Python variables:
A variable name must start with a letter or the underscore character
A variable name cannot start with a number
A variable name can only contain alpha-numeric characters and underscores (A-z, 0-9, and _ )
Variable names are case-sensitive (age, Age and AGE are three different variables)
A variable name cannot be any of the Python keywords.
Multi Words Variable Names
Variable names with more than one word can be difficult to read.
There are several techniques you can use to make them more readable:
Camel Case
Each word, except the first, starts with a capital letter:
myVariableName = "John"
Pascal Case
Each word starts with a capital letter:
MyVariableName = "John"
Snake Case
Each word is separated by an underscore character:
my_variable_name = "John"
Python Variables - Assign Multiple Values
Python allows you to assign values to multiple variables in one line:
x, y, z = "Orange", "Banana", "Cherry"
Note: Make sure the number of variables matches the number of values, or else you will get an error.
One Value to Multiple Variables
And you can assign the same value to multiple variables in one line:
x = y = z = "Orange"
Unpack a Collection
If you have a collection of values in a list, tuple etc. Python allows you to extract the values into variables. This is called unpacking.
Unpack a list:
fruits = ["apple", "banana", "cherry"]
x, y, z = fruits
Unpacking a tuple:
fruits = ("apple", "banana", "cherry")
(green, yellow, red) = fruits
Note: The number of variables must match the number of values in the tuple, if not, you must use an asterisk(*) to collect the remaining values as a list.
Assign the rest of the values as a list called "red":
fruits = ("apple", "banana", "cherry", "strawberry", "raspberry")
(green, yellow, *red) = fruits
If the asterisk is added to another variable name than the last, Python will assign values to the variable until the number of values left matches the number of variables left.
fruits = ("apple", "mango", "papaya", "pineapple", "cherry")
(green, *tropic, red) = fruits
Python - Output Variables
The Python print() function is often used to output variables. In the print() function, you output multiple variables, separated by a comma(,):
x = "Python"
y = "is"
z = "awesome"
print(x, y, z)
#output:
Python is awesome
You can also use the + operator to output multiple variables:
x = "Python"
y = "is"
z = "awesome"
print(x + y + z)
#output:
Pythonisawesome
() is necessary otherwise error occurs
For numbers, the + character works as a mathematical operator.
In the print() function, when you try to combine a string and a number with the + operator, Python will give you an error:
x = 5
y = "John"
print(x + y)
#error
The best way to output multiple variables in the print() function is to separate them with commas, which even support different data types:
x = 5
y = "John"
print(x, y)
Python - Global Variables
Global Variables
Variables that are created outside of a function are known as global variables.
Global variables can be used by everyone, both inside of functions and outside.
If you create a variable with the same name inside a function, this variable will be local, and can only be used inside the function. The global variable with the same name will remain as it was, global and with the original value.
x = "awesome"
def myfunc():
x = "fantastic"
print("Python is " + x)
myfunc()
print("Python is " + x)
#output:
Python is fantastic
Python is awesome
The global Keyword
To create a global variable inside a function, you can use the global keyword, the variable belongs to the global scope:
def myfunc():
global x
x = "fantastic"
myfunc()
print("Python is " + x)
#output:
Python is fantastic
To change the value of a global variable inside a function, refer to the variable by using the global keyword:
x = "awesome"
def myfunc():
global x
x = "fantastic"
myfunc()
print("Python is " + x)
#output:
Python is fantastic
Python Data Types
Built-in Data Types
In programming, data type is an important concept.
Variables can store data of different types, and different types can do different things.
Python has the following data types built-in by default(a preselected option), in these categories:
Text Type: str
Numeric Types: int, float, complex(x = 1j
Sequence Types: list, tuple, range(x = range(6)
Mapping Type: dict
Set Types: set(x = {"apple", "banana", "cherry"}), frozenset(x = frozenset({"apple", "banana", "cherry"}))
Boolean Type: bool
Binary Types: bytes(x = b"Hello"), bytearray(x = bytearray(5)), memoryview(x = memoryview(bytes(5)))
None Type: NoneType
Getting the Data Type
You can get the data type of any object by using the type() function.
Setting the Specific Data Type
If you want to specify the data type, you can use the following constructor functions:
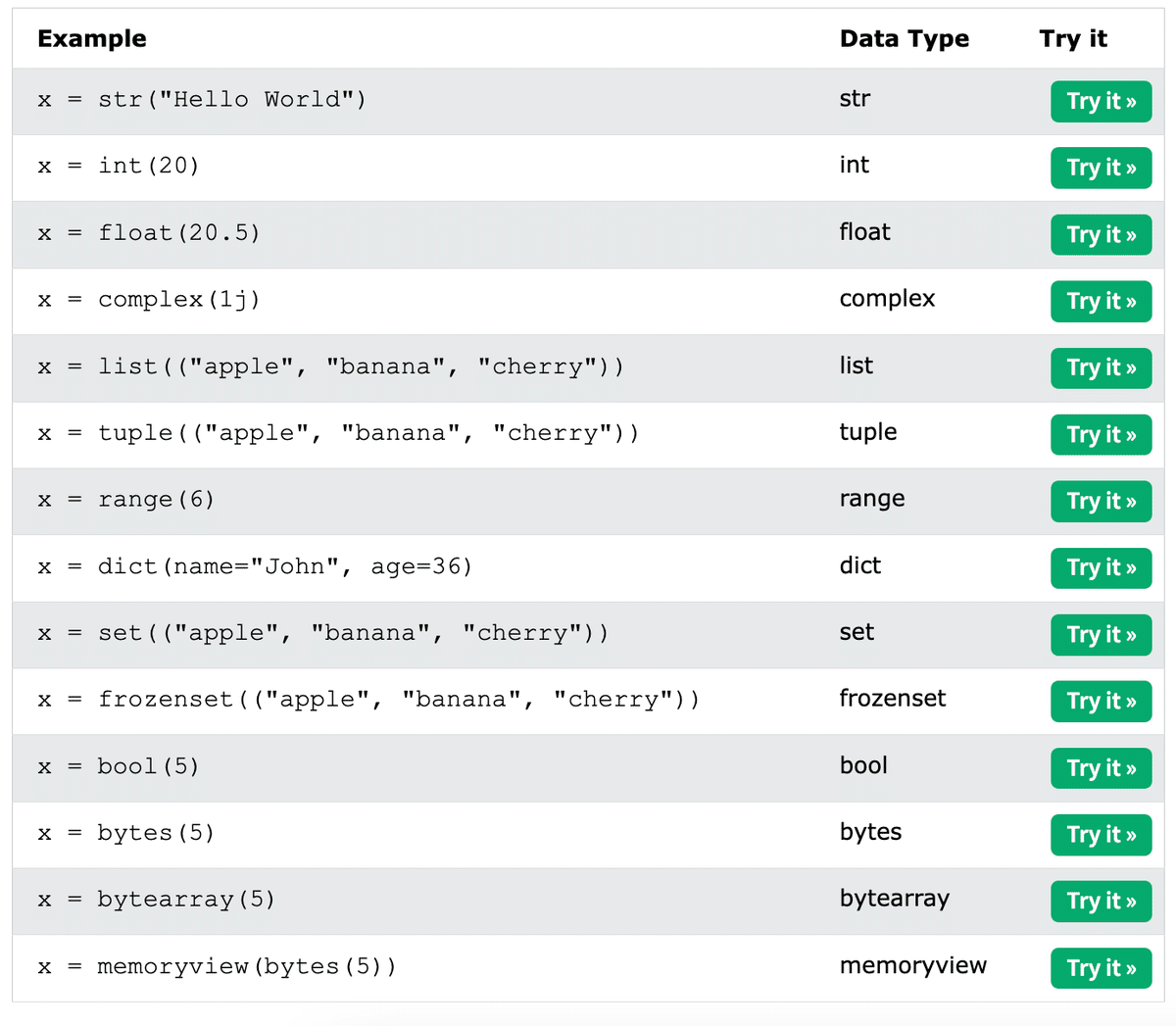
Python Numbers
Int
Int, or integer, is a whole number, positive or negative, without decimals, of unlimited length.
Float
Float, or "floating point number" is a number, positive or negative, containing one or more decimals.
Float can also be scientific numbers with an "e" to indicate the power of 10.
x = 35e3
y = 12E4
z = -87.7e100
Complex
Complex numbers are written with a "j" as the imaginary(虚数部) part:
x = 3+5j
y = 5j
z = -5j
Type Conversion
You can convert from one type to another with the int(), float(), and complex() methods.
Note: You cannot convert complex numbers into another number type.
Random Number
Python does not have a random() function to make a random number, but Python has a built-in module called random that can be used to make random numbers:
# display a random number between 1 and 9
import random
print(random.randrange(1, 10))
In our Random Module Reference you will learn more about the Random module.
Python Casting
Specify a Variable Type
There may be times when you want to specify a type on to a variable. This can be done with casting. Python is an object-orientated language, and as such it uses classes to define data types, including its primitive types.
Casting in python is therefore done using constructor functions:
int() - constructs an integer number from an integer literal, a float literal (by removing all decimals), or a string literal (providing the string represents a whole number)
float() - constructs a float number from an integer literal, a float literal or a string literal (providing the string represents a float or an integer)
str() - constructs a string from a wide variety of data types, including strings, integer literals and float literals
Python Strings
Strings
Strings in python are surrounded by either single quotation marks, or double quotation marks.
'hello' is the same as "hello".
Assign String to a Variable
Assigning a string to a variable is done with the variable name followed by an equal sign and the string.
Multiline Strings
You can assign a multiline string to a variable by using three quotes or three single quotes:
a = """Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt
ut labore et dolore magna aliqua."""
a = '''Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt
ut labore et dolore magna aliqua.'''
#output:
Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt
ut labore et dolore magna aliqua.
Note: in the result, the line breaks are inserted at the same position as in the code.
Strings are Arrays
Like many other popular programming languages, strings in Python are arrays of bytes representing unicode characters.
However, Python does not have a character data type, a single character is simply a string with a length of 1.
Square brackets[] can be used to access elements of the string.
a = "Hello, World!"
print(a[1])
Looping Through a String
Since strings are arrays, we can loop through the characters in a string, with a for loop.
String Length
To get the length of a string, use the len() function.
Check String
To check if a certain phrase or character is present in a string, we can use the keyword in. Use it in an if statement:
if "x" in "text":
Check if NOT
To check if a certain phrase or character is NOT present in a string, we can use the keyword not in.
Python - Slicing Strings
Slicing
You can return a range of characters by using the slice syntax.
Specify the start index and the end index, separated by a colon(:), to return a part of the string.
Slice From the Start
By leaving out the start index, the range will start at the first character.
Slice To the End
By leaving out the end index, the range will go to the end.
Negative Indexing
Use negative indexes to start the slice from the end of the string:
b = "Hello, World!"
print(b[2:5])
print(b[:5])
print(b[2:])
print(b[-5:-2]) #Get the characters: From: "o" in "World!" (position -5) To, but not included: "d" in "World!" (position -2):
Python - Modify Strings
Upper Case
The upper() method returns the string in upper case:
a = "Hello, World!"
print(a.upper())
#output:
HELLO, WORLD!
Lower Case
The lower() method returns the string in lower case:
a = "Hello, World!"
print(a.lower())
#output:
hello, world
Remove Whitespace
Whitespace is the space before and/or after the actual text, and very often you want to remove this space. The strip() method removes any whitespace from the beginning or the end.
Replace String
The replace() method replaces a string with another string:
a = "Hello, World!"
print(a.replace("H", "J"))
Split String
The split() method returns a list where the text between the specified separator becomes the list items.
a = "Hello, World!"
print(a.split(",")) # returns ['Hello', ' World!']
Learn more about String Methods with our String Methods Reference
Python - String Concatenation
String Concatenation
To concatenate, or combine, two strings you can use the + operator.
a = "Hello"
b = "World"
c = a + b
c = a + " " + b
Python - Format - Strings
String Format
But we can combine strings and numbers by using the format() method!
The format() method takes the passed arguments, formats them, and places them in the string where the placeholders {} are:
The format() method takes unlimited number of arguments, and are placed into the respective placeholders:
You can use index numbers {0} to be sure the arguments are placed in the correct placeholders:
quantity = 3
itemno = 567
price = 49.95
myorder = "I want {} pieces of item {} for {} dollars."
print(myorder.format(quantity, itemno, price))
myorder = "I want to pay {2} dollars for {0} pieces of item {1}."
print(myorder.format(quantity, itemno, price))
Python - Escape Characters
Escape Character
To insert characters that are illegal in a string, use an escape character.
An escape character is a backslash \ followed by the character you want to insert. The escape character allows you to use double quotes when you normally would not be allowed:
txt = "We are the so-called \"Vikings\" from the north."
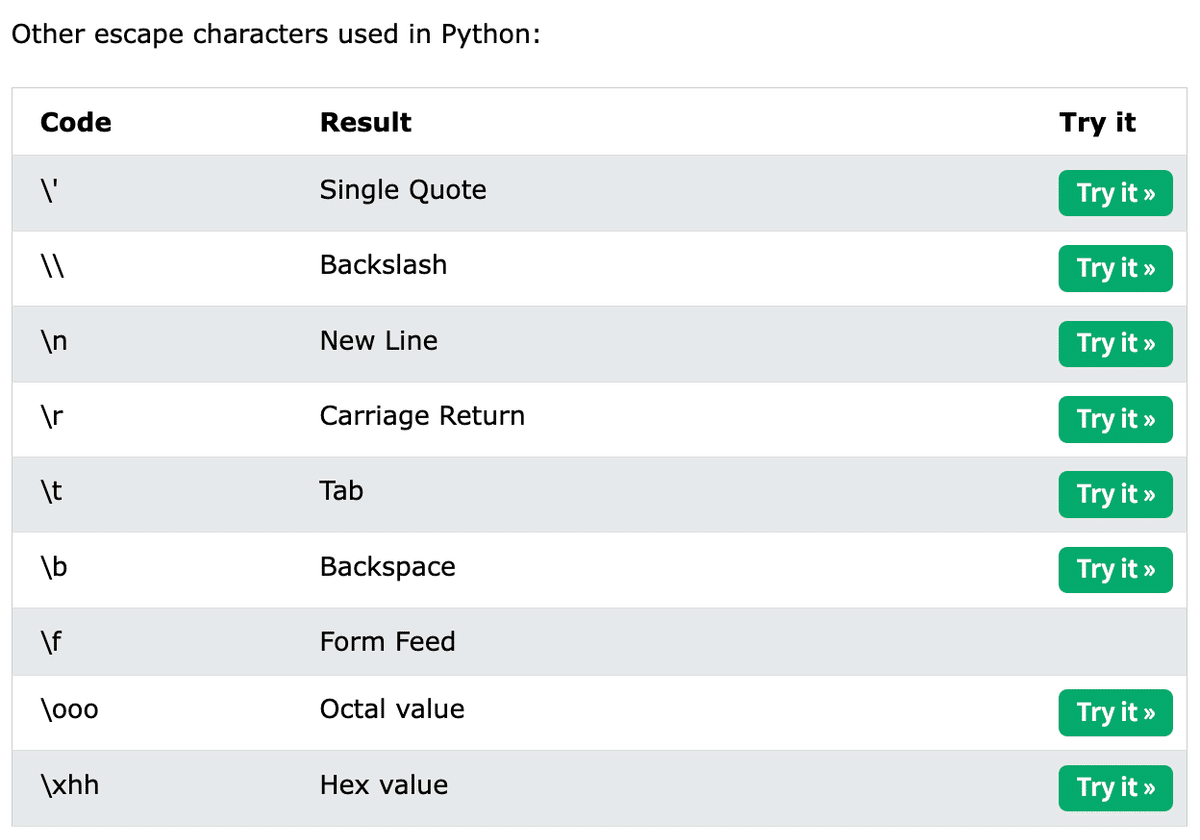
Python Booleans
Booleans represent one of two values: True or False.
Boolean Values
In programming you often need to know if an expression is True or False.
You can evaluate any expression in Python, and get one of two answers, True or False.
When you compare two values, the expression is evaluated and Python returns the Boolean answer.
Evaluate Values and Variables
The bool() function allows you to evaluate any value, and give you True or False in return,
Most Values are True
Almost any value is evaluated to True if it has some sort of content.
Any string is True, except empty strings.
Any number is True, except 0.
Any list, tuple, set, and dictionary are True, except empty ones.
Some Values are False
In fact, there are not many values that evaluate to False, except empty values, such as (), [], {}, "", the number 0, and the value None. And of course the value False evaluates to False.
bool(False)
bool(None)
bool(0)
bool("")
bool(())
bool([])
bool({})
One more value, or object in this case, evaluates to False, and that is if you have an object that is made from a class with a __len__ function that returns 0 or False.
Functions can Return a Boolean
You can create functions that returns a Boolean Value.
Python also has many built-in functions that return a boolean value, like the isinstance() function, which can be used to determine if an object is of a certain data type:
x = 200
print(isinstance(x, int))
Python Operators
Operators are used to perform operations on variables and values.
Python divides the operators in the following groups:
Arithmetic operators: Arithmetic operators are used with numeric values to perform common mathematical operations.
x = 2
y = 5
print(x ** y) #same as 2*2*2*2*2
# + - * / %(Modulus) // **
Assignment operators: Assignment operators are used to assign values to variables.
=
x += 3# x = x + 3
x -= 3# x = x - 3
...
Comparison operators: ==/!=…
Logical operators: and/or/not
Identity operators: is/is not
Membership operators: in/not in
Bitwise operators: Bitwise operators are used to compare (binary) numbers(&/|/^/~/<</>>)
Operator Precedence
Operator precedence describes the order in which operations are performed.
Parentheses has the highest precedence, meaning that expressions inside parentheses must be evaluated first. If two operators have the same precedence, the expression is evaluated from left to right.
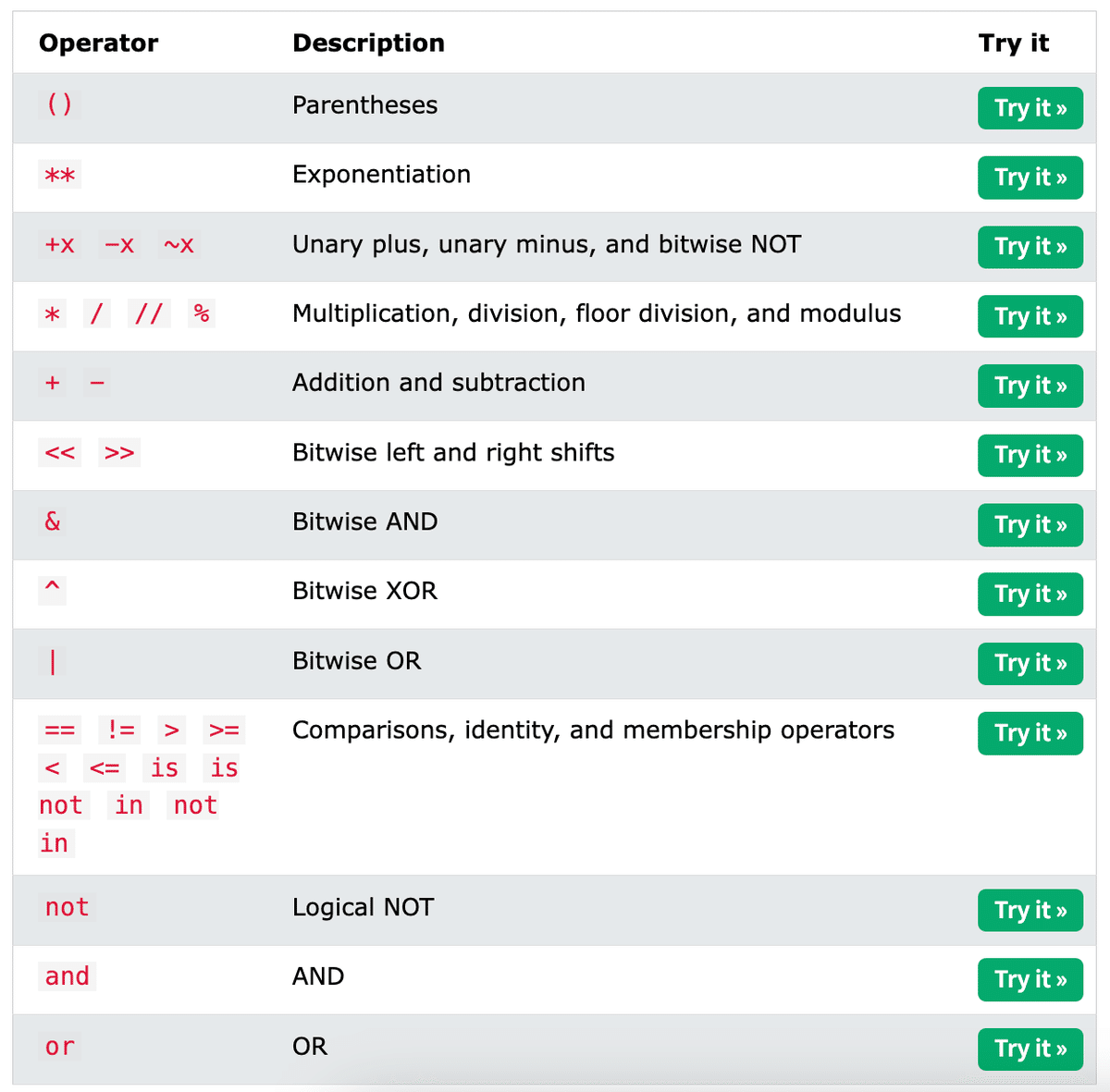
Python Lists
Lists are used to store multiple items in a single variable.
Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage.
List Items
List items are ordered, changeable, and allow duplicate values.
List items are indexed, the first item has index [0], the second item has index [1] etc.
Ordered
When we say that lists are ordered, it means that the items have a defined order, and that order will not change.
If you add new items to a list, the new items will be placed at the end of the list.
Note: There are some list methods that will change the order, but in general: the order of the items will not change.
Changeable
The list is changeable, meaning that we can change, add, and remove items in a list after it has been created.
Allow Duplicates
Since lists are indexed, lists can have items with the same value.
List Length
To determine how many items a list has, use the len() function.
List Items - Data Types
List items can be of any data type. A list can contain different data types.
The list() Constructor
It is also possible to use the list() constructor when creating a new list.
Python Collections (Arrays)
There are four collection data types in the Python programming language:
List is a collection which is ordered and changeable. Allows duplicate members.
Tuple is a collection which is ordered and unchangeable. Allows duplicate members.
Set is a collection which is unordered, unchangeable*, and unindexed. No duplicate members.
Dictionary is a collection which is ordered** and changeable. No duplicate members.
*Set items are unchangeable, but you can remove and/or add items whenever you like.
**As of Python version 3.7, dictionaries are ordered. In Python 3.6 and earlier, dictionaries are unordered.
Python - Access List Items
Access Items
List items are indexed and you can access them by referring to the index number.
Negative Indexing
Negative indexing means start from the end
-1 refers to the last item, -2 refers to the second last item etc.
Range of Indexes
You can specify a range of indexes by specifying where to start and where to end the range.
When specifying a range, the return value will be a new list with the specified items.
thislist[2:5]
thislist[:4]
thislist[:4]
thislist[-4:-1]
Check if Item Exists
if "apple" in thislist:
Python - Change List Items
Change Item Value
thislist[1] = "blackcurrant"
Change a Range of Item Values
To change the value of items within a specific range, define a list with the new values, and refer to the range of index numbers where you want to insert the new values:
thislist[1:3] = ["blackcurrant", "watermelon"]
If you insert more items than you replace, the new items will be inserted where you specified, and the remaining items will move accordingly:
If you insert less items than you replace, the new items will be inserted where you specified, and the remaining items will move accordingly:
thislist[1:2] = ["blackcurrant", "watermelon"]
thislist[1:3] = ["watermelon"]
Note: The length of the list will change when the number of items inserted does not match the number of items replaced.
Insert Items
To insert a new list item, without replacing any of the existing values, we can use the insert() method.
The insert() method inserts an item at the specified index:
thislist = ["apple", "banana", "cherry"]
thislist.insert(2, "watermelon")
#output:
['apple', 'banana', 'watermelon', 'cherry']
Python - Add List Items
Append Items
To add an item to the end of the list, use the append() method:
thislist.append("orange")
Extend List
To append elements from another list to the current list, use the extend() method.
thislist = ["apple", "banana", "cherry"]
tropical = ["mango", "pineapple", "papaya"]
thislist.extend(tropical)
Add Any Iterable
The extend() method does not have to append lists, you can add any iterable (反復可能) object (tuples, sets, dictionaries etc.).
thislist = ["apple", "banana", "cherry"]
thistuple = ("kiwi", "orange")
thislist.extend(thistuple)
Python - Remove List Items
Remove Specified Item
The remove() method removes the specified item.
thislist.remove("banana")
If there are more than one item with the specified value, the remove() method removes the first occurance:
Remove Specified Index
The pop() method removes the specified index.
If you do not specify the index, the pop() method removes the last item.
thislist.pop(1)
thislist.pop()
The del keyword also removes the specified index.
The del keyword can also delete the list completely.
del thislist[0]
del thislist
Clear the List
The clear() method empties the list.
The list still remains, but it has no content.
thislist.clear()
Python - Loop Lists
You can loop through the list items by using a for loop:
for x in thislist:
Loop Through the Index Numbers
You can also loop through the list items by referring to their index number.
Use the range() and len() functions to create a suitable iterable.
for i in range(len(thislist)):
Using a While Loop
i = 0
while i < len(thislist):
print(thislist[i])
i = i + 1
Looping Using List Comprehension
[print(x) for x in thislist]
Python - List Comprehension
List comprehension offers a shorter syntax when you want to create a new list based on the values of an existing list.
Example:
Based on a list of fruits, you want a new list, containing only the fruits with the letter "a" in the name.
fruits = ["apple", "banana", "cherry", "kiwi", "mango"]
newlist = []
for x in fruits:
if "a" in x:
newlist.append(x)
print(newlist)
newlist = [x for x in fruits if "a" in x]
The Syntax
newlist = [expression for item in iterable if condition == True]
The return value is a new list, leaving the old list unchanged.
Condition
The condition is like a filter that only accepts the items that valuate to True.
The condition if x != "apple" will return True for all elements other than "apple", making the new list contain all fruits except "apple".
The condition is optional and can be omitted:
newlist = [x for x in fruits]
Iterable
The iterable can be any iterable object, like a list, tuple, set etc.
newlist = [x for x in range(10)]
Expression
The expression is the current item in the iteration, but it is also the outcome, which you can manipulate before it ends up like a list item in the new list:
newlist = [x.upper() for x in fruits]
newlist = ['hello' for x in fruits]
The expression can also contain conditions, not like a filter, but as a way to manipulate the outcome:
#Return "orange" instead of "banana"
newlist = [x if x != "banana" else "orange" for x in fruits]
Python - Sort Lists
Sort List Alphanumerically
List objects have a sort() method that will sort the list alphanumerically, ascending, by default:
(A-Z そして a-z | number大小|mixed is error
thislist = ["orange", "Mango", "miwi", "pineapple", "banana"]
thislist.sort()
print(thislist)
#output:
['Mango', 'banana', 'miwi', 'orange', 'pineapple']
Sort Descending
To sort descending, use the keyword argument reverse = True:
thislist.sort(reverse = True)
Customize Sort Function
You can also customize your own function by using the keyword argument key = function.
The function will return a number that will be used to sort the list (the lowest number first):
Case Insensitive Sort
By default the sort() method is case sensitive, resulting in all capital letters being sorted before lower case letters.
Luckily we can use built-in functions as key functions when sorting a list.So if you want a case-insensitive sort function, use str.lower as a key function:
thislist.sort(key = str.lower)
Reverse Order
What if you want to reverse the order of a list, regardless of the alphabet?
The reverse() method reverses the current sorting order of the elements.
thislist = ["banana", "Orange", "Kiwi", "cherry"]
thislist.reverse()
#output:
['cherry', 'Kiwi', 'Orange', 'banana']
Python - Copy Lists
You cannot copy a list simply by typing list2 = list1, because: list2 will only be a reference to list1, and changes made in list1 will automatically also be made in list2.
There are ways to make a copy, one way is to use the built-in List method copy().
Another way to make a copy is to use the built-in method list().
mylist = thislist.copy()
mylist = list(thislist)
Python - Join Lists
There are several ways to join, or concatenate, two or more lists in Python.
One of the easiest ways are by using the + operator.
Another way to join two lists is by appending all the items from list2 into list1, one by one:
Or you can use the extend() method, where the purpose is to add elements from one list to another list:
list1 = ["a", "b", "c"]
list2 = [1, 2, 3]
list3 = list1 + list2
for x in list2:
list1.append(x)
list1.extend(list2)
#output:
['a', 'b', 'c', 1, 2, 3]
Python - List Methods
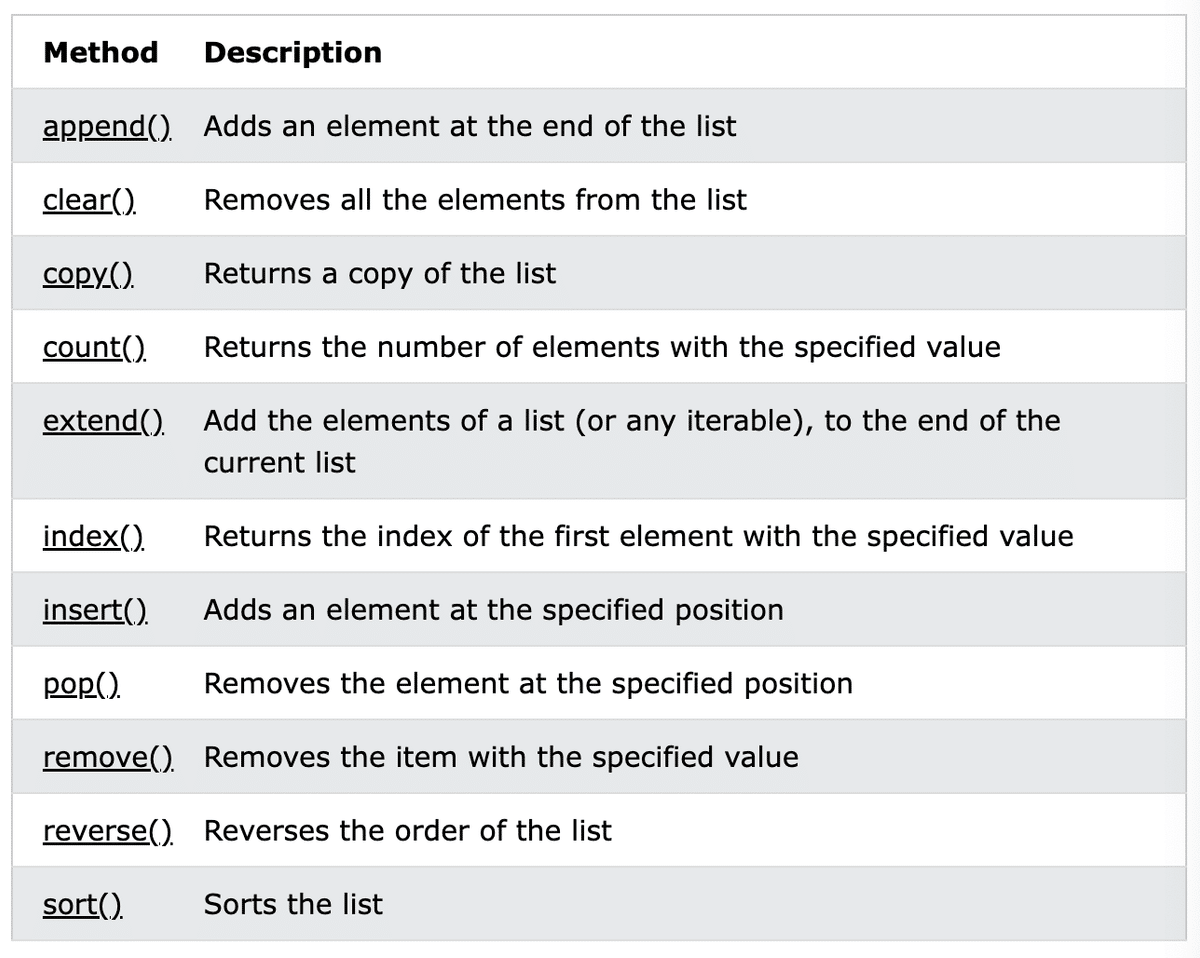
Python List index() Method
x = mylist.index("cherry")
#output:
2
Definition and Usage
The index() method returns the position at the first occurrence of the specified value.
Syntax
list.index(elmnt)
elmnt: Required. Any type (string, number, list, etc.). The element to search for
Python List count() Method
Definition and Usage
The count() method returns the number of elements with the specified value.
Syntax
list.count(value)
value: Required. Any type (string, number, list, tuple, etc.). The value to search for.
numlist = [1, 2, 4, 5, 2, 2, 7]
x = numlist.count(2)
#output:
3
Python Tuples
Tuples are used to store multiple items in a single variable.
Tuple is one of 4 built-in data types in Python used to store collections of data.
A tuple is a collection which is ordered and unchangeable.
Tuples are written with round brackets.
mytuple = ("apple", "banana", "cherry")
Tuple Items
Tuple items are ordered, unchangeable, and allow duplicate(COPY) values.
Tuple items are indexed.
Ordered
When we say that tuples are ordered, it means that the items have a defined order, and that order will not change.
Unchangeable
Tuples are unchangeable, meaning that we cannot change, add or remove items after the tuple has been created.
Allow Duplicates
Since tuples are indexed, they can have items with the same value.
len(thistuple)
Create Tuple With One Item
To create a tuple with only one item, you have to add a comma(,) after the item, otherwise Python will not recognize it as a tuple.
thistuple = ("apple",)
#NOT a tuple BUT a str
thistuple = ("apple")
Tuple Items - Data Types
Tuple items can be of any data type, and can contain different data types.
The tuple() Constructor
It is also possible to use the tuple() constructor to make a tuple.
Access Tuple Items
The same as list
Python - Update Tuples
But there are some workarounds.
x = ("apple", "banana", "cherry")
y = list(x)
y[1] = "kiwi"
x = tuple(y)
Add Items & Remove Items
Convert into a list: Just like the workaround for changing a tuple, you can convert it into a list, add your item(s), and convert it back into a tuple.
thistuple = ("apple", "banana", "cherry")
y = list(thistuple)
y.append("orange")
thistuple = tuple(y)
Add tuple to a tuple: You are allowed to add tuples to tuples, so if you want to add one item, (or many), create a new tuple with the item(s), and add it to the existing tuple:
thistuple = ("apple", "banana", "cherry")
y = ("orange",)
thistuple += y
you can delete the tuple completely:
thistuple = ("apple", "banana", "cherry")
del thistuple
print(thistuple) #this will raise an error because the tuple no longer exists
Python - Unpack Tuples
previous description
Python - Loop Tuples
the same as list
Python - Join Tuples
the same as list but:
Multiply Tuples & List
If you want to multiply the content of a tuple a given number of times, you can use the * operator:
mytuple = tuple * 2
mylist = list * 2
Tuple Methods
Python has two built-in methods that you can use on tuples.
count() and index()
Python Sets
Sets are used to store multiple items in a single variable.
Set is one of 4 built-in data types in Python used to store collections of data.
A set is a collection which is unordered, unchangeable*, and unindexed.
Sets are written with curly brackets.
myset = {"apple", "banana", "cherry"}
Note: Sets are unordered, so you cannot be sure in which order the items will appear.
Set Items
Set items are unordered, unchangeable, and do not allow duplicate values.
Unordered
Unordered means that the items in a set do not have a defined order.
Set items can appear in a different order every time you use them, and cannot be referred to by index or key.
Unchangeable
Once a set is created, you cannot change its items, but you can remove items and add new items.
Duplicates Not Allowed
Sets cannot have two items with the same value.
Note: The values True and 1 are considered the same value in sets, and are treated as duplicates.
Note: The values False and 0 are considered the same value in sets, and are treated as duplicates.
The set() Constructor
It is also possible to use the set() constructor to make a set.
Python - Add Set Items
To add one item to a set use the add() method.
thisset.add("orange")
Add Sets
To add items from another set into the current set, use the update() method.
#Add elements from tropical into thisset
thisset.update(tropical)
Add Any Iterable
The object in the update() method does not have to be a set, it can be any iterable object (tuples, lists, dictionaries etc.).
thisset = {"apple", "banana", "cherry"}
mylist = ["kiwi", "orange"]
thisset.update(mylist)
Python - Remove Set Items
To remove an item in a set, use the remove(), or the discard() method.
thisset.remove("banana")
thisset.discard("banana")
Note: If the item to remove does not exist, remove() will raise an error.
Note: If the item to remove does not exist, discard() will NOT raise an error.
You can also use the pop() method to remove an item, but this method will remove a random item, so you cannot be sure what item that gets removed.
The return value of the pop() method is the removed item.
x = thisset.pop()
The clear() method empties the set:
The del keyword will delete the set completely:
thisset.clear()
#output:
set()
del thisset
#output: this will raise an error because the set no longer exists
Python - Join Sets
You can use the union() method that returns a new set containing all items from both sets, or the update() method that inserts all the items from one set into another.
set3 = set1.union(set2)
set1.update(set2)
Note: Both union() and update() will exclude any duplicate items.
Keep ONLY the Duplicates
The intersection_update() method will keep only the items that are present in both sets.
set1.intersection_update(set2)
The intersection() method will return a new set, that only contains the items that are present in both sets.
set3 = set1.intersection(set2)
Keep All, But NOT the Duplicates
The symmetric_difference_update() method will keep only the elements that are NOT present in both sets.
The symmetric_difference() method will return a new set, that contains only the elements that are NOT present in both sets.
set1.symmetric_difference_update(set2)
set3 = set1.symmetric_difference(set2)
Set Methods
Python has a set of built-in methods that you can use on sets.
Python Dictionaries
Dictionary
Dictionaries are used to store data values in key:value pairs.
A dictionary is a collection which is ordered, changeable and do not allow duplicates.
Dictionaries are written with curly brackets, and have keys and values.
thisdict = {"brand": "Ford", "model": "Mustang", "year": 1964}
Dictionary Items
Dictionary items are ordered, changeable, and does not allow duplicates.
Dictionary items are presented in key:value pairs, and can be referred to by using the key name.
And that order will not change.
print(thisdict["brand"])
Changeable
Dictionaries are changeable, meaning that we can change, add or remove items after the dictionary has been created.
Duplicates Not Allowed
Dictionaries cannot have two items with the same key.
Dictionary Items - Data Types
The values in dictionary items can be of any data type.
The dict() Constructor
It is also possible to use the dict() constructor to make a dictionary.
thisdict = dict(name = "John", age = 36, country = "Norway")
Python - Access Dictionary Items
the same as list but:
There is also a method called get() that will give you the same result:
x = thisdict.get("model")
Get Keys
The keys() method will return a list of all the keys in the dictionary.
x = thisdict.keys()
#output:
dict_keys(['brand', 'model', 'year'])
Get Values
The values() method will return a list of all the values in the dictionary.
x = thisdict.values()
Get Items
The items() method will return each item in a dictionary, as tuples in a list.
x = thisdict.items()
#output:
dict_items([('brand', 'Ford'), ('model', 'Mustang'), ('year', 1964)])
Check if Key Exists
To determine if a specified key is present in a dictionary use the in keyword.
Python - Change Dictionary Items
Change Values
You can change the value of a specific item by referring to its key name:
thisdict["year"] = 2018
Update Dictionary
The update() method will update the dictionary with the items from the given argument.
The argument must be a dictionary, or an iterable object with key:value pairs.
thisdict.update({"year": 2020})
Python - Add Dictionary Items
Adding Items
Adding an item to the dictionary is done by using a new index key and assigning a value to it.
Update Dictionary
The update() method will update the dictionary with the items from a given argument. If the item does not exist, the item will be added.
The argument must be a dictionary, or an iterable object with key:value pairs.
thisdict["color"] = "red"
thisdict.update({"color": "red"})
Python - Remove Dictionary Items
The popitem() method removes the last inserted item (in versions before 3.7, a random item is removed instead):
thisdict.pop("model")
thisdict.popitem()
del thisdict["model"]
#The del keyword can also delete the dictionary completely
del thisdict
#The clear() method empties the dictionary.
thisdict.clear()
Python - Loop Dictionaries
values()
keys()
items()
for x in thisdict:
print(x)
for x in thisdict:
print(thisdict[x])
for x in thisdict.values():
for x in thisdict.keys():
for x, y in thisdict.items():
Python - Copy Dictionaries
mydict = thisdict.copy()
mydict = dict(thisdict)
Python - Nested Dictionaries
A dictionary can contain dictionaries, this is called nested dictionaries.
myfamily = {
"child1" : {
"name" : "Emil",
"year" : 2004
},
"child2" : {
"name" : "Tobias",
"year" : 2007
},
"child3" : {
"name" : "Linus",
"year" : 2011
}
}
child1 = {
"name" : "Emil",
"year" : 2004
}
child2 = {
"name" : "Tobias",
"year" : 2007
}
child3 = {
"name" : "Linus",
"year" : 2011
}
myfamily = {
"child1" : child1,
"child2" : child2,
"child3" : child3
}
Access Items in Nested Dictionaries
To access items from a nested dictionary, you use the name of the dictionaries, starting with the outer dictionary:
print(myfamily["child2"]["name"])
Python Dictionary Methods
Python has a set of built-in methods that you can use on dictionaries.
Python Conditions and If statements
if
elif
else
Short Hand If
If you have only one statement to execute, you can put it on the same line as the if statement.
if a > b: print("a is than")
Short Hand If ... Else
If you have only one statement to execute, one for if, and one for else, you can put it all on the same line:
print("A") if a > b else print("B")
This technique is known as Ternary Operators(Ternary operator "x ? y : z" in many programming languages, whose value is y if x evaluates to true and z otherwise), or Conditional Expressions.
#One line if else statement, with 3 conditions:
a = 330
b = 330
print("A") if a > b else print("=") if a == b else print("B")
#output:
=
and or not:
if not a > b:
Nested If
You can have if statements inside if statements, this is called nested if statements.
The pass Statement
if statements cannot be empty, but if you for some reason have an if statement with no content, put in the pass statement to avoid getting an error.
if a > b:
pass
Python While Loops
Python has two primitive loop commands:
while loops
for loops
Note: remember to increment i, or else the loop will continue forever.
The break Statement
With the break statement we can stop the loop even if the while condition is true.
The continue Statement
With the continue statement we can stop the current iteration, and continue with the next:
#output:
1
2
4
5
6
i = 0
while i < 6:
i += 1
if i == 3:
continue
print(i)
The else Statement
With the else statement we can run a block of code once when the condition no longer is true.
この記事が気に入ったらサポートをしてみませんか?