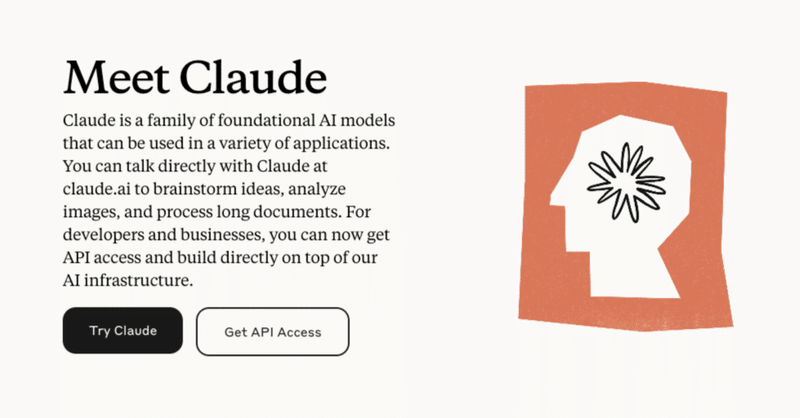
ClaudeのFunctionCallingを試す
こんにちは、ニケです。
皆さんClaude使っていますでしょうか?
時間もないので、もう前置きは置いといてサクッと行きますね。
GPT APIでお世話になったFunctionCallingがどうやらClaudeにもあるっぽいので試してみました。
やってみた
ドキュメントはこちらです。
ちょっとだけ違和感のある日本語ですが、量も少なくわかりやすいと思います。
ただ、XMLだとちょっとやりづらいなと思った方もいるのではないでしょうか?
というわけで公式が出してるリポジトリがあったのでこちらを使ってみます。
Pythonで完結するのでわかりやすいですね。
⚠ ただし、アルファ版なので継続して更新する予定はないようです。
おそらくそのうちもっとわかりやすい方法が公式から出ると思われるので、急ぎでない方はそれ待ちでも良いかと思います。
ではまずリポジトリを用意…
> git clone https://github.com/anthropics/anthropic-tools.git
> cd anthropic-tools
以降はREADMEに書いてある方法で環境を構築します。
# MacOS
> export ANTHROPIC_API_KEY={your_anthropic_api_key}
> python3 -m venv anthropictools
> source anthropictools/bin/activate
> pip install -r requirements.txt
あとはテストスクリプトを実行するだけです。
> python -m tool_use_package.calculator_example
John now has 2 apples + 2 bananas = 4 fruit total
In summary:
John has 2 apples and 2 bananas, so 4 fruit total
Maggie has 10 apples
Tim has 2 bananas
解説
ではスクリプトを読んでみましょう。
全コードはこちら。
https://github.com/anthropics/anthropic-tools/blob/main/tool_use_package/calculator_example.py
関数定義
# Define our addition tool
class AdditionTool(BaseTool):
"""Adds together two numbers, a + b."""
def use_tool(self, a, b):
return a+b
# Define our subtraction tool
class SubtractionTool(BaseTool):
"""Subtracts one number from another, a - b."""
def use_tool(self, a, b):
return a-b
Claudeにわたす説明
# Initialize an instance of each tool
addition_tool_name = "perform_addition"
addition_tool_description = """Add one number (a) to another (b), returning a+b.
Use this tool WHENEVER you need to perform any addition calculation, as it will ensure your answer is precise."""
addition_tool_parameters = [
{"name": "a", "type": "float", "description": "The first number in your addition equation."},
{"name": "b", "type": "float", "description": "The second number in your addition equation."}
]
subtraction_tool_name = "perform_subtraction"
subtraction_tool_description = """Subtract one number (b) from another (a), returning a-b.
Use this tool WHENEVER you need to perform any subtraction calculation, as it will ensure your answer is precise."""
subtraction_tool_parameters = [
{"name": "a", "type": "float", "description": "The minuend in your subtraction equation."},
{"name": "b", "type": "float", "description": "The subtrahend in your subtraction equation."}
]
Toolsとして設定
addition_tool = AdditionTool(addition_tool_name, addition_tool_description, addition_tool_parameters)
subtraction_tool = SubtractionTool(subtraction_tool_name, subtraction_tool_description, subtraction_tool_parameters)
# Pass the tool instance into the ToolUser
tool_user = ToolUser([addition_tool, subtraction_tool])
user_inputを入れて実行
if __name__ == '__main__':
messages = [{"role":"user", "content":"John has three apples. Maggie has nine apples. Tim has 4 bananas. If John gives Maggie 1 apple and Tim gives John 2 bananas, how much fruit does each person have?"}]
print(tool_user.use_tools(messages, execution_mode="automatic"))
簡単ですね。
オリジナルの関数設定もすぐできました。
いえーい
— ニケちゃん@美少女AI開発 (@tegnike) March 7, 2024
ごみ収集スケジュールファンクション動いたぜ〜〜 https://t.co/F5VG25mYw9 pic.twitter.com/SvnZs2WESG
この記事が気に入ったらサポートをしてみませんか?