ChatGPTにコーディングさせてみた③ ゲーム作成
前回までのおさらい
前々回よりはそれっぽくなってはいるのですが、これはテトリスではないですよね。テトリスとは何かを再度定義してあげる必要がありそうです。
【前回のラスト】
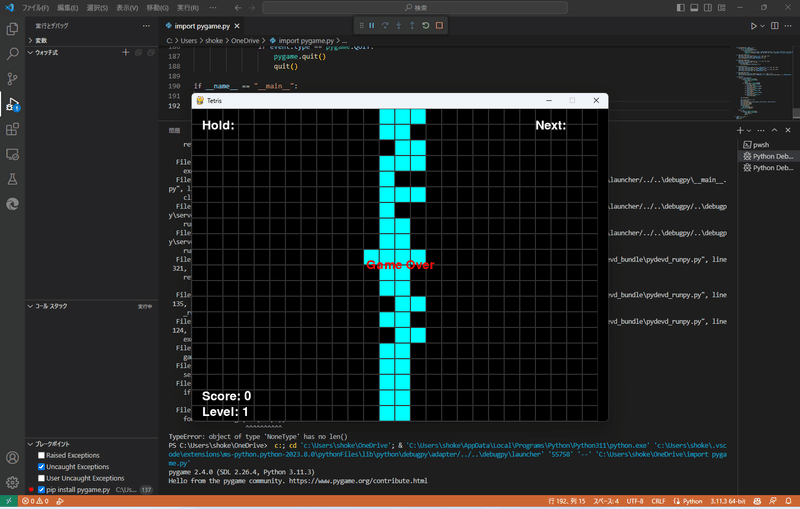
テトリス再整理
以下にテトリスの要素を再定義しました。
日本語より英語の方が適しているとだろうとの判断のもと、以下のように整理しました。(ChatGPT活用)
1.Game Mechanics:
・Tetrominoes: The game features a set of seven different tetromino shapes, composed of four squares each. The shapes include the I, J, L, O, S, T, and Z tetrominoes.
・Falling Tetrominoes: Tetrominoes appear at the top of the game area and descend vertically. Players can control the movement of the falling tetrominoes using keyboard inputs or touch controls.
・Movement: Players can move the falling tetrominoes horizontally, either to the left or right, using the corresponding controls. Additionally, they can rotate the tetrominoes clockwise or counterclockwise to fit them into the desired position.
・Falling Speed: The falling speed of the tetrominoes gradually increases as the game progresses, adding to the challenge.
・Locking Mechanism: When a tetromino reaches the bottom of the game area or collides with existing blocks, it locks into place, becoming part of the static structure.
・Line Clearing: When a horizontal line is completely filled with blocks, it clears from the game area, and the player earns points. Clearing multiple lines simultaneously (e.g., two, three, or four lines) yields additional points.
Game Over: If the stack of tetrominoes reaches the top of the game area, the game ends, and the player's score is recorded.
2.User Interface:
・Game Area: A rectangular grid serves as the main playing field. Typically, the grid dimensions are 10 cells wide and 20 cells tall, but variations exist.
Next Tetromino Preview: A smaller area shows the upcoming tetromino, allowing players to plan their moves in advance.
・Score Display: The current score and possibly other relevant information, such as the level, lines cleared, and high score, are presented to the player.
Controls: Clear instructions on how to control the movement and rotation of the falling tetrominoes should be provided. This may include arrow keys, WASD, or touchscreen controls.
3.Gameplay Flow:
・Game Start: The game begins with an empty game area and a randomly selected tetromino appearing at the top.
・Continuous Falling: The tetromino continuously descends at a steady pace until it locks into place or is manipulated by the player.
・Player Actions: The player can move the tetromino horizontally, rotate it, or make it descend faster by pressing the corresponding controls.
・Locking and Line Clearing: When a tetromino locks into place, the game checks for completed lines. If a line is filled, it clears, and the player earns points. The game area adjusts accordingly.
・Increasing Difficulty: As the game progresses, the falling speed of the tetrominoes gradually increases, making the gameplay more challenging.
Game Over: The game continues until the stack of tetrominoes reaches the top of the game area, at which point the game ends. The player's score is displayed, and they may have the option to restart or quit.
Additional Features (optional):
・Levels: The game can have multiple levels, where the falling speed increases or additional challenges are introduced as the player progresses.
Power-ups: Special abilities or power-ups can be included to provide players with advantages or unique gameplay mechanics.
・Multiplayer: Implementing multiplayer functionality allows players to compete or cooperate with others in real-time.
・Visual and Audio Effects: Engaging graphics, animations, and sound effects enhance the overall gaming experience.
ChatGPTを用いたコードの作成
上記の条件のゲームができるように再度、コーディングさせました。
そして出来上がったコードがこちらです。
【出来上がったコード】
import pygame
import random
# テトロミノの形状と色を定義
SHAPES_COLORS = [
([[1, 1, 1, 1]], (0, 255, 255)), # I - Cyan
([[1, 1], [1, 1]], (255, 255, 0)), # O - Yellow
([[0, 1, 1], [1, 1]], (0, 255, 0)), # S - Green
([[1, 1, 0], [0, 1, 1]], (255, 0, 0)), # Z - Red
([[1, 1, 1], [0, 1, 0]], (128, 0, 128)), # T - Purple
([[1, 1, 1], [1, 0, 0]], (255, 165, 0)), # L - Orange
([[1, 1, 1], [0, 0, 1]], (0, 0, 255)) # J - Blue
]
class Tetris:
block_size = 20
grid_width = 10
grid_height = 20
def __init__(self):
pygame.init()
pygame.display.set_caption('Tetris')
self.screen = pygame.display.set_mode((self.grid_width * self.block_size, self.grid_height * self.block_size))
self.grid = [[(0, 0, 0) for _ in range(self.grid_width)] for _ in range(self.grid_height)]
self.reset_tetromino()
def reset_tetromino(self):
self.tetromino, self.tetromino_color = random.choice(SHAPES_COLORS)
self.tetromino_x = self.grid_width // 2 - len(self.tetromino[0]) // 2
self.tetromino_y = 0
if self.check_collision(0, 0):
self.grid = [[(0, 0, 0) for _ in range(self.grid_width)] for _ in range(self.grid_height)]
print("Game Over")
pygame.quit()
def check_collision(self, dx, dy):
for y, row in enumerate(self.tetromino):
for x, cell in enumerate(row):
try:
if cell and (self.grid[y + self.tetromino_y + dy][x + self.tetromino_x + dx] != (0, 0, 0)):
return True
except IndexError:
return True
return False
def rotate_tetromino(self):
self.tetromino = [list(x) for x in zip(*self.tetromino[::-1])]
if self.check_collision(0, 0):
self.tetromino = [list(x) for x in zip(*self.tetromino[::-1])]
self.tetromino = [list(x) for x in zip(*self.tetromino[::-1])]
self.tetromino = [list(x) for x in zip(*self.tetromino[::-1])]
def lock_tetromino(self):
for y, row in enumerate(self.tetromino):
for x, cell in enumerate(row):
if cell:
self.grid[y + self.tetromino_y][x + self.tetromino_x] = self.tetromino_color
def clear_lines(self):
full_rows = [i for i, row in enumerate(self.grid) if all(cell != (0, 0, 0) for cell in row)]
if full_rows:
for i in sorted(full_rows, reverse=True):
del self.grid[i]
self.grid.insert(0, [(0, 0, 0) for _ in range(self.grid_width)])
def draw_grid(self):
for y, row in enumerate(self.grid):
for x, cell in enumerate(row):
pygame.draw.rect(self.screen, cell, (x * self.block_size, y * self.block_size, self.block_size, self.block_size), not cell == (0, 0, 0))
def draw_tetromino(self):
for y, row in enumerate(self.tetromino):
for x, cell in enumerate(row):
if cell:
pygame.draw.rect(self.screen, self.tetromino_color, ((self.tetromino_x + x) * self.block_size, (self.tetromino_y + y) * self.block_size, self.block_size, self.block_size))
def run(self):
clock = pygame.time.Clock()
while True:
self.screen.fill((0, 0, 0))
self.draw_grid()
self.draw_tetromino()
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
self.rotate_tetromino()
if event.key == pygame.K_DOWN and not self.check_collision(0, 1):
self.tetromino_y += 1
if event.key == pygame.K_LEFT and not self.check_collision(-1, 0):
self.tetromino_x -= 1
if event.key == pygame.K_RIGHT and not self.check_collision(1, 0):
self.tetromino_x += 1
if not self.check_collision(0, 1):
self.tetromino_y += 1
else:
self.lock_tetromino()
self.clear_lines()
self.reset_tetromino()
clock.tick(5 + (1 * self.tetromino_y // 5)) # 速度増加
if __name__ == "__main__":
Tetris().run()
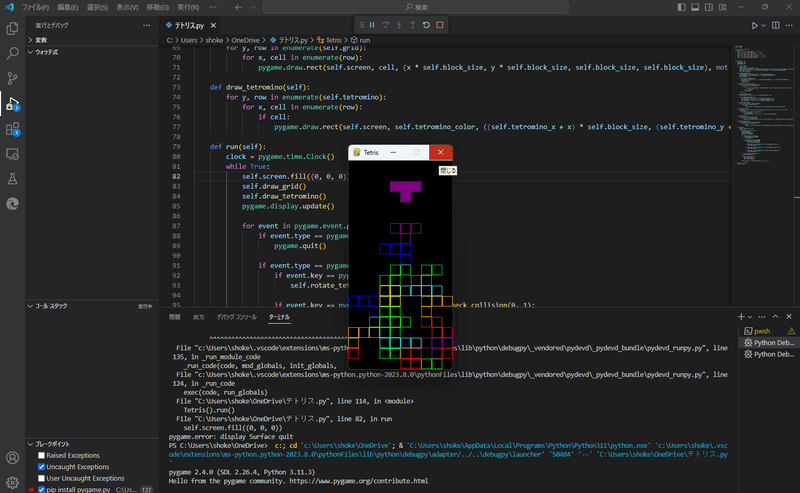
様々な色形のブロックが落ちてくる、操作ができる、一列揃ったら消える、限界まで積み重なると終了する、といったテトリスとして成り立つ最低限のことは再現できました。
コーディングの限界?
テトリス作成はこれにていったん終了としたいと思います。
と言いますのも、以下のスクショのようにChatGPTから"困難です"との返答があったためです。
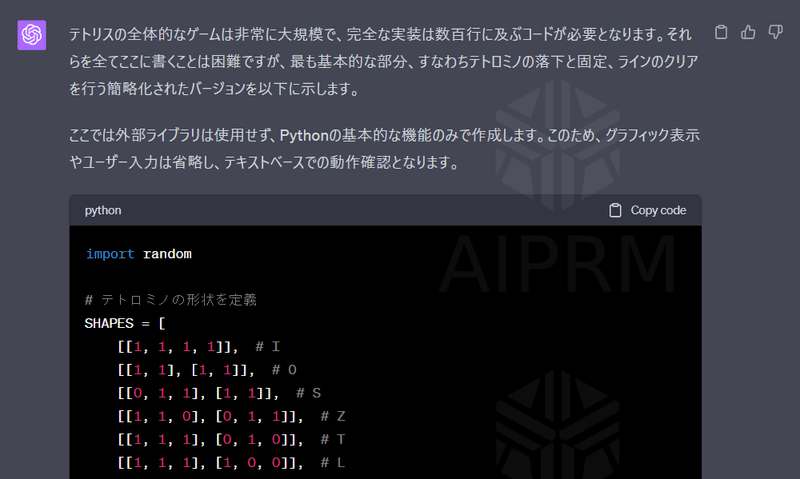
さらに、困難である理由をChatGPTに効いてみました。
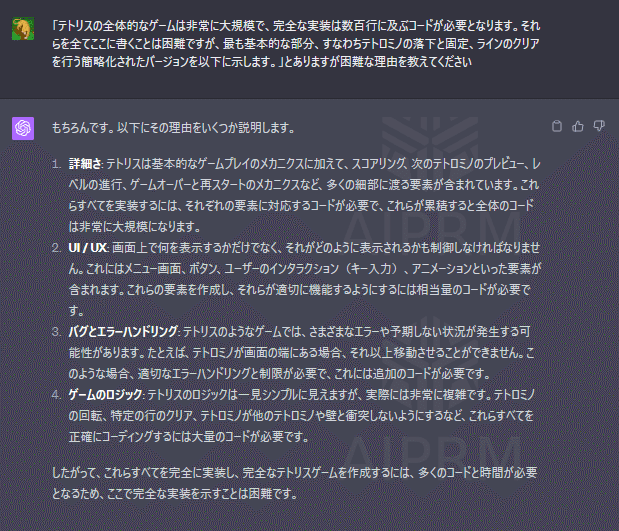
つまり、簡単に言うと膨大な量のコーディングは無理ですよとのことでした。
これは今後も立ちはだかってくる大きな壁となりそうです。
しかし、ここで折れるのではなく、ChatGPTのこれからの発展を期待しつつも、何か良い他の方法はないか模索し続けていきます!
最後に
コーディングさせてみると意外といけるもんだなと、ド素人ながら感じております。ただ、やはり現場で活躍されるエンジニアの方からしたら遊びの範疇にも及ばないものであるもの事実。
日々修行して、これまでにない新しいエンジニア?みたいなもんになれればいいなとか思いながら、毎日noteを書かせて頂いております。
では、明日も新しい取り組みを頑張って参ります!さらば!
この記事が気に入ったらサポートをしてみませんか?