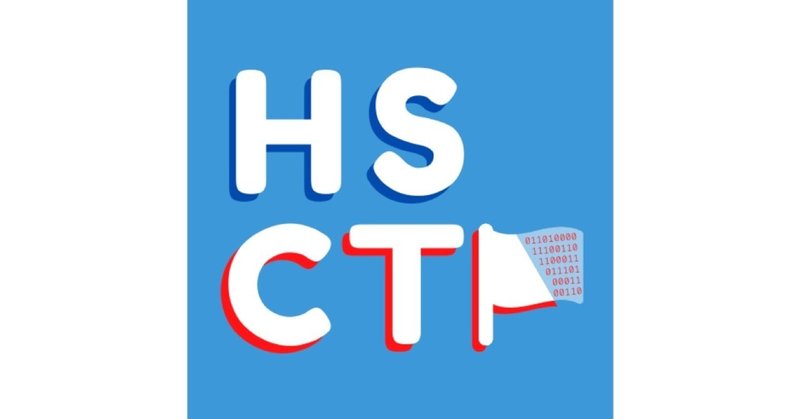
HSCTF 7 write-up
6月1日12:00〜6日00:00 (UTC)に開催されたHSCTFにソロで参加しました。1120ポイントで1466チーム中474位でした。
以下、writeupです。
---------- Reverse Engineering ----------
* AP Lab: Computer Science Principles
This activity will ask you to reverse a basic program and solve an introductory reversing challenge. You will be given an output that is to be used in order to reconstruct the input, which is the flag.
Author: AC
与えられたプログラムは以下の通りである。
import java.util.Scanner;
public class ComputerSciencePrinciples
{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String inp = sc.nextLine();
if (inp.length()!=18) {
System.out.println("Your input is incorrect.");
System.exit(0);
}
inp=shift2(shift(inp));
if (inp.equals("inagzgkpm)Wl&Tg&io")) {
System.out.println("Correct. Your input is the flag.");
}
else {
System.out.println("Your input is incorrect.");
}
System.out.println(inp);
}
public static String shift(String input) {
String ret = "";
for (int i = 0; i<input.length(); i++) {
ret+=(char)(input.charAt(i)-i);
}
return ret;
}
public static String shift2(String input) {
String ret = "";
for (int i = 0; i<input.length(); i++) {
ret+=(char)(input.charAt(i)+Integer.toString((int)input.charAt(i)).length());
}
return ret;
}
}
入力(inp)に対して、shiftとshift2の関数を適用して、結果が"inagzgkpm)Wl&Tg&io"と等しいか確認していることがわかる。shiftとshift2は文字コードをずらしているだけなので、逆を行うようにするとフラグを得られる。
import java.util.*;
public class Main {
public static void main(String[] args) throws Exception {
String inp = "inagzgkpm)Wl&Tg&io";
inp = shift_rev(shift2_rev(inp));
System.out.println(inp);
}
public static String shift_rev(String input) {
String ret = "";
for (int i = 0; i<input.length(); i++) {
ret+=(char)(input.charAt(i)+i);
}
return ret;
}
public static String shift2_rev(String input) {
String ret = "";
for (int i = 0; i<input.length(); i++) {
ret+=(char)(input.charAt(i)-Integer.toString((int)input.charAt(i)).length());
}
return ret;
}
}
Flag: flag{intr0_t0_r3v}
* AP Lab: English Language
The AP English Language activity will ask you to reverse a program about manipulating strings and arrays. Again, an output will be given where you have to reconstruct an input.
Author: AC
今回のプログラムは以下の通りである。
i
mport java.util.Scanner;
public class EnglishLanguage
{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String inp = sc.nextLine();
if (inp.length()!=23) {
System.out.println("Your input is incorrect.");
System.exit(0);
}
for (int i = 0; i<3; i++) {
inp=transpose(inp);
inp=xor(inp);
}
if (inp.equals("1dd3|y_3tttb5g`q]^dhn3j")) {
System.out.println("Correct. Your input is the flag.");
}
else {
System.out.println("Your input is incorrect.");
}
}
public static String transpose(String input) {
int[] transpose = {11,18,15,19,8,17,5,2,12,6,21,0,22,7,13,14,4,16,20,1,3,10,9};
String ret = "";
for (int i: transpose) {
ret+=input.charAt(i);
}
return ret;
}
public static String xor(String input) {
int[] xor = {4,1,3,1,2,1,3,0,1,4,3,1,2,0,1,4,1,2,3,2,1,0,3};
String ret = "";
for (int i = 0; i<input.length(); i++) {
ret+=(char)(input.charAt(i)^xor[i]) ;
}
return ret;
}
}
上と同じように入力(inp)にtransposeとxorを3回適用して、結果が"1dd3|y_3tttb5g`q]^dhn3j"と等しいか確認している。transposeは配列の順番を入れ替えているだけ、xorは再度xorをかけるともとに戻ることを利用して逆算すると、フラグを得られる。
import java.util.*;
public class Main {
public static void main(String[] args) throws Exception {
String inp = "1dd3|y_3tttb5g`q]^dhn3j";
for (int i = 0; i<3; i++) {
inp=xor(inp);
inp=transpose_rev(inp);
}
System.out.println(inp);
}
public static String transpose_rev(String input) {
//int[] transpose = {11,18,15,19,8,17,5,2,12,6,21,0,22,7,13,14,4,16,20,1,3,10,9};
int[] transpose_rev = {11,19,7,20,16,6,9,13,4,22,21,0,8,14,15,2,17,5,1,3,18,10,12};
String ret = "";
for (int i: transpose_rev) {
ret+=input.charAt(i);
}
return ret;
}
public static String xor(String input) {
int[] xor = {4,1,3,1,2,1,3,0,1,4,3,1,2,0,1,4,1,2,3,2,1,0,3};
String ret = "";
for (int i = 0; i<input.length(); i++) {
ret+=(char)(input.charAt(i)^xor[i]) ;
}
return ret;
}
}
Flag: flag{n0t_t00_b4d_r1ght}
* Recursion Reverse
Jimmy needs some help figuring out how computers process text, help him out!
Author: AD
今回のプログラムは以下です。
import java.util.Scanner;
public class AscII {
static int num = 0;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter your guess: ");
String guess = sc.nextLine();
if (guess.length()!= 12)
System.out.println("Sorry, thats wrong.");
else {
if(flagTransformed(guess).equals("I$N]=6YiVwC"))
System.out.println("Yup the flag is flag{" + guess + "}");
else
System.out.println("nope");
}
}
public static String flagTransformed(String guess) {
char[] transformed = new char[12];
for(int i = 0; i < 12; i++) {
num = 1;
transformed[i] = (char)(((int)guess.charAt(i) + pickNum(i + 1)) % 127);
}
char[] temp = new char[12];
for(int i = 11; i >= 0; i--)
temp[11-i] = transformed[i];
return new String(temp);
}
private static int pickNum(int i) {
for(int x = 0; x <= i; x++)
num+=x;
if(num % 2 == 0)
return num;
else
num = pickNum(num);
return num;
}
}
同じように逆の演算をするとフラグを得られらます。
import java.util.*;
public class Main {
static int num = 0;
public static void main(String[] args) {
String guess = "I$N]=6YiVwC";
guess = flagTransformed(guess);
System.out.println(guess);
}
public static String flagTransformed(String guess) {
char[] temp = new char[12];
for(int i = 11; i >= 0; i--)
temp[11-i] = guess.charAt(i);
char[] transformed = new char[12];
for(int i = 0; i < 12; i++) {
num = 1;
int j = 0;
int pick = pickNum(i + 1);
while(true) {
int k = j*127 + (int)temp[i] - pick;
if(k > 0){
transformed[i] = (char)k;
break;
}
j++;
}
}
return new String(transformed);
}
private static int pickNum(int i) {
for(int x = 0; x <= i; x++)
num+=x;
if(num % 2 == 0)
return num;
else
num = pickNum(num);
return num;
}
}
Flag: flag{AscII is key}
---------- Binary Exploitation ----------
* Intro to Netcat 2: Electric Boogaloo
Intro to Netcat was too difficult, seeing as 32% of teams failed to solve it.
To get the flag, install Netcat.
nc pwn.hsctf.com 5001
Author: meow
$ nc pwn.hsctf.com 5001
Hey, here's your flag! flag{https://youtu.be/-TVWst0YqCI}
Flag: flag{https://youtu.be/-TVWst0YqCI}
---------- Forensics ----------
* Comments
I found this file on my Keith's computer... what could be inside?
Author: AC
Hint: Look through the comments :eyes:
Hint: http://kb.winzip.com/help/winzip/help_comment.htm
Command.zipというファイルをダウンロードできる。解凍すると1.zipができ、1.zipを解凍すると2.zipができるのを繰り返し、8.zipを解凍するとflag.txtが手に入るが、「No flag here. :(」とテキストがあるだけでフラグは手に入らない。
ヒントにあるようにコメントが鍵のようなので、zipinfoコマンドを使って情報を表示する。
$ zipinfo -v Comments.zip
Archive: Comments.zip
There is no zipfile comment.
End-of-central-directory record:
-------------------------------
Zip archive file size: 1471 (00000000000005BFh)
Actual end-cent-dir record offset: 1449 (00000000000005A9h)
Expected end-cent-dir record offset: 1449 (00000000000005A9h)
(based on the length of the central directory and its expected offset)
This zipfile constitutes the sole disk of a single-part archive; its
central directory contains 1 entry.
The central directory is 76 (000000000000004Ch) bytes long,
and its (expected) offset in bytes from the beginning of the zipfile
is 1373 (000000000000055Dh).
Central directory entry #1:
---------------------------
1.zip
offset of local header from start of archive: 0
(0000000000000000h) bytes
file system or operating system of origin: Unix
version of encoding software: 3.0
minimum file system compatibility required: MS-DOS, OS/2 or NT FAT
minimum software version required to extract: 1.0
compression method: none (stored)
file security status: not encrypted
extended local header: no
file last modified on (DOS date/time): 2020 May 25 13:17:54
file last modified on (UT extra field modtime): 2020 May 26 02:17:53 local
file last modified on (UT extra field modtime): 2020 May 25 17:17:53 UTC
32-bit CRC value (hex): 2e050a96
compressed size: 1310 bytes
uncompressed size: 1310 bytes
length of filename: 5 characters
length of extra field: 24 bytes
length of file comment: 1 characters
disk number on which file begins: disk 1
apparent file type: binary
Unix file attributes (100777 octal): -rwxrwxrwx
MS-DOS file attributes (00 hex): none
The central-directory extra field contains:
- A subfield with ID 0x5455 (universal time) and 5 data bytes.
The local extra field has UTC/GMT modification/access times.
- A subfield with ID 0x7875 (Unix UID/GID (any size)) and 11 data bytes:
01 04 e8 03 00 00 04 e8 03 00 00.
------------------------- file comment begins ----------------------------
f
-------------------------- file comment ends -----------------------------
同様に、1.zip等の情報も確認して、繋げるとフラグとなる。
Flag: flag{4n6}
---------- Algorithms ----------
* Pythagorean Tree Fractal 1
Please see the attached file for more details (and ignore the red dots on the images).
Author: Plate_of_Sunshine
Stageが1あがるごとに正方形の一辺に2つの正方形が追加されていくフラクタル図形に関する問題である。Stage 50のときの正方形の数がフラグである。
Stage nだと正方形方形の数は2^n-1となるので、答えは2^50-1となる。
Flag: flag{1125899906842623)
* Pythagorean Tree Fractal 2
Pythagorean Tree 2
Because every good thing must have a sequel ;)
Please see the attached file for more details (and ignore the red dots on the images).
Note: Don't worry about overlapping squares!
Author: Plate_of_Sunshine
Stage 1の面積が70368744177664のとき、Stage 25では面積はいくつになるかという問題である。計算すると、Stage 2では面積は2倍、Stage 3では面積は3倍になることがわかるので、Stage 25では70368744177664 * 25となり、フラグを得られる。
Flag: flag{1759218604441600}
----------- Forensics ----------
* Meta Mountains
It seems that mountains are a great place for hiding secrets. Maybe you could find this one!
Author: wooshi
Stringsコマンドで可読部分を表示させると、3パートにわかれたフラグが見つかる。
$ strings mountains_hsctf.jpg -n 10
part 1/3: flag{h1dd3n_w1th1n_
part 2/3: th3_m0unta1ns_
2012:02:03 11:18:05
part 3/3: l13s_th3_m3tadata}
...
おそらく本来はExifのメタデータを確認するのが狙いだと思うので、EXIF確認くんで情報を見ても同じくフラグが見つかる。
Flag: flag{h1dd3n_w1th1n_th3_m0unta1ns_l13s_th3_m3tadata}
---------- Web Exploitation ----------
* Blurry Eyes
I can't see :(
https://blurry-eyes.web.hsctf.com
Author: meow
指定されたページにアクセスすると、フラグが書かれているであろう部分は隠されていて見えないようになっている。
ページのソースを確認すると、CSSでスタイルを変更していることがわかる。
CSSファイルを確認すると、フラグがわかる。
Flag: flag{glasses_are_useful}
* Inspector Gadget
https://inspector-gadget.web.hsctf.com/
Author: Madeleine
アクセスしてソースを見るとそのまま書いてある。
Flag: flag{n1ce_j0b_0p3n1nG_th3_1nsp3ct0r_g4dg3t}
* Very Safe Login
Bet you can't log in.
https://very-safe-login.web.hsctf.com/very-safe-login
Author: Madeleine
webページにアクセスすると、ユーザー名とパスワードを求められる。
ページのソースを確認すると、スクリプトにユーザー名とパスワードがの条件式があるので、これらを入力するとフラグが手に入る。
Flag: flag{cl13nt_51de_5uck5_135313531}
---------- Milestones -----------
* Does CTFd Work?
Does the Catch The Flag daemon work? Here's the flag: flag{y3S_i7_d03s_3xcl4m4710n_m4rk_890d9a0b}
Flag: flag{y3S_i7_d03s_3xcl4m4710n_m4rk_890d9a0b}
* Discord Flag
Join the HSCTF Discord: https://discord.gg/vU7tFKx
Flag: flag{good_luck_have_fun}
この記事が気に入ったらサポートをしてみませんか?