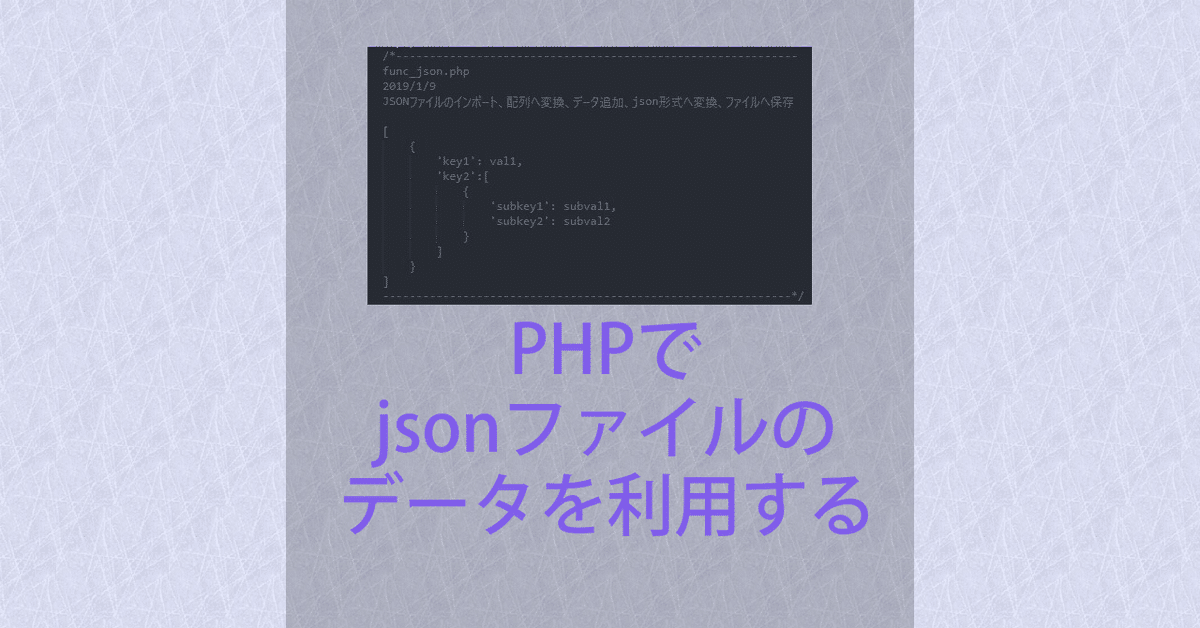
PHPで手軽にjsonデータを利用する
PHPでテキストデータを保存する
大量のデータを保存、取得する時はMySQLやPostgreなどのデータベースを利用しますが、扱うデータがそれほど多くない場合はテキストファイルを利用するのが便利です。
通常のテキストデータを扱うにはfopen関数、fwrite関数、fclose関数など手続きが割と煩雑で、またfile関数で1行ごとに取得して加工しなければならないのですが、jsonファイルを利用すると簡単に配列としてデータを取得することができます。
jsonファイルはjavascriptでデータのやり取りをするときに使うというイメージが強いですが、PHPでも問題なく利用できます。
jsonデータを配列に
jsonファイルのデータをやり取りするための関数が、json_decode関数とjson_encode関数です。
http://php.net/manual/ja/function.json-decode.php
http://php.net/manual/ja/function.json-encode.php
file_get_contents関数でjsonファイルを読み込み、テキストデータ全文を代入した変数をjson_decode関数の引数に渡してあげると配列として返してくれます。
$json = file_get_contents('text.json');
$arrdata = json_decode($json,true);
http://php.net/manual/ja/function.file-get-contents.php
ファイルとして保存する時は、配列をjson_encode関数の引数に渡してあげた戻り値をfile_put_contents関数でテキストファイルに保存します。
戻り値には成功した場合は保存したファイルのバイト数、失敗した場合はfalseが返されます。
$json = json_encode($arrdata)
$result = file_put_contents('data.json', $json);
たとえば、こんな感じのjsonファイルが
[
{
"dir": "AAA",
"title": "contents1",
"file": "index.html"
},
{
"dir": "BBB",
"title": "contents2",
"file": "index.html",
},
{
"dir": "CCC",
"title": "contents3",
"file": "index.html"
}
]
こんな感じの配列になります。
array (size=3)
0 =>
array (size=3)
'dir' => string 'AAA' (length=3)
'title' => string 'contents1' (length=9)
'file' => string 'index.html' (length=10)
1 =>
array (size=3)
'dir' => string 'BBB' (length=3)
'title' => string 'contents2' (length=9)
'file' => string 'index.html' (length=10)
2 =>
array (size=3)
'dir' => string 'CCC' (length=3)
'title' => string 'contents3' (length=9)
'file' => string 'index.html' (length=10)
データが数十件程度であればデータベースを作ってSQLで抽出などしなくても手軽に活用できると思います。
もちろんフィールド数が多くて抽出条件が複雑な場合はSQLでやった方が良いですが。
共有ファイルにfunctionとして保存
より簡単に利用できるように、jsonファイルを扱う処理をcommonファイルに抽出しました。エディタの色分けが判りやすいかとキャプチャしてみました。参考までにコードは最下部に載せてます。
jsonファイルの読み込み
読み込んだ配列にデータを追加
配列をjsonファイルに保存
一連の流れを簡易的に処理するモジュール
ーーー サンプルコード ーーー
function get_json($jsonfile, $order='ascend'){
$json = @file_get_contents($jsonfile);
$json = mb_convert_encoding($json, 'UTF8', 'ASCII,JIS,UTF-8,EUC-JP,SJIS-WIN');
$arrdata = json_decode($json,true);
//降順にするとき---
if($order == 'descend'){
rsort($arrdata);
}
return $arrydata;
}
function merge_json($arrydata, $addarry){
//追加するデータ(配列)---
$addarry = array();
$f_merge = false;
if(count($arrydata) && count($addarry)){
$f_merge = true;
}
if(!$f_merge){
$resarry = array_merge_recursive($arrdata, $addarry);
}
return $resarry;
}
function put_json($resarry, $filename){
$result = null;
$result = file_put_contents($filename, json_encode($resarry));
return $result;
}
//標準的な処理---
function modify_json($jsonfile, $order='ascend', $addarry='', $filename=''){
$arrydata = get_json($jsonfile, $order);
if(is_array($addarry)){
$arrydata = merge_json($arrydata, $addarry);
}
if($filename){
$result = put_json($resarry, $filename);
}
return array($arrydata, $result);
}
この記事が気に入ったらサポートをしてみませんか?