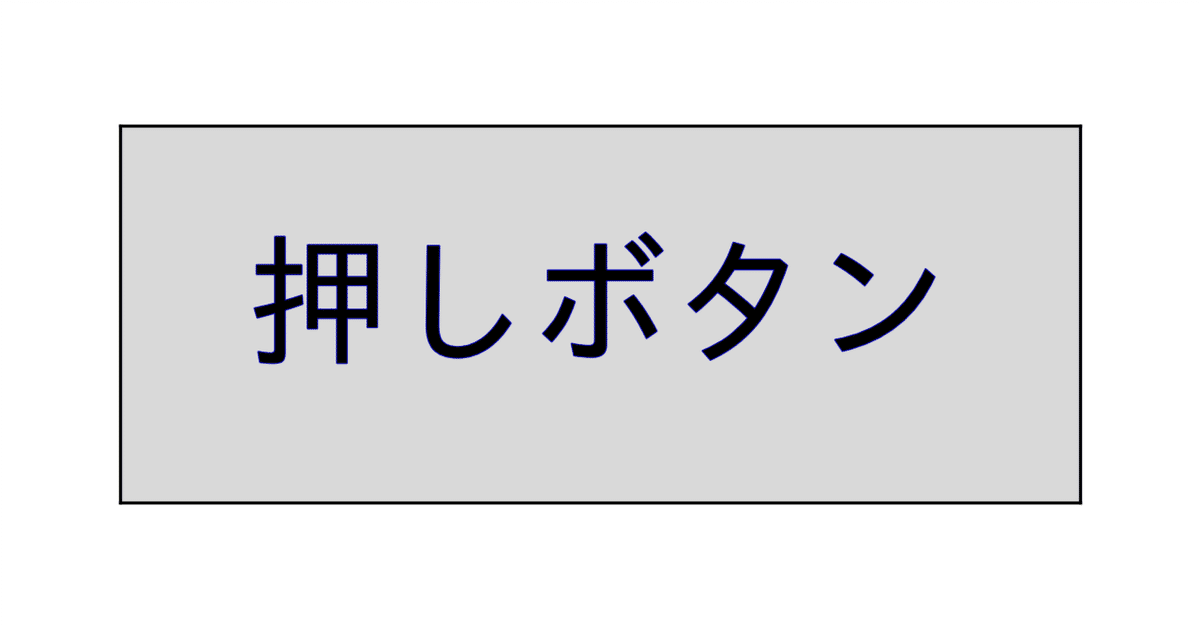
Button (matplotlib )
とりあえず動くものを作ってみよう、ということで、カウンターです。真ん中の数字が、「count up」を押すと1増え、「count down」を押すと1減ります。
一番上の例が Widget の Button を使った場合、二番目と三番目が Text をボタンとして使用した場合です。
Text のベースとなる Artist には、マウスクリックなどのイベントがその中で起きたかどうかを判定する関数(contain)や、その中でイベントが起こると指定した共通の関数を呼ぶ機能(pick)が用意されています。ニ番目では contain を、三番目では pickを使ってみました。
matplotlib では、大体の描画要素がArtist をベースとしているので、原理的にはそれらすべてをボタンとして動作させる事ができます。
pick と contain をどう使い分けるべきなのか、現時点であまり理解しておりません。pick では判定領域を広げたりできるようなので、小さいものとか細いもの(点とか線)をボタンにしたいときにいいのかも?
使いながら学習していきたいと思います。
#"counter.py"
import matplotlib.pyplot as plt
from matplotlib.widgets import Button
class Counter():
def __init__(self):
self.fig = plt.figure()
self.count = 0
fig = self.fig
self.text = fig.text(0.5,0.55,self.count,size = 50, ha="center")
ax_up = fig.add_axes([0.55, 0.2, 0.35, 0.15])
ax_down = fig.add_axes([0.1, 0.2, 0.35, 0.15])
self.b_up = Button(ax_up, 'count up')
self.b_down = Button(ax_down, 'count down')
self.b_up.label.set_fontsize(25)
self.b_down.label.set_fontsize(25)
def count_up(self, event):
self.count += 1
self.update()
def count_down(self, event):
self.count -= 1
self.update()
def update(self):
self.text.set_text(self.count)
self.fig.canvas.draw_idle()
def connect(self):
self.b_up.on_clicked(self.count_up)
self.b_down.on_clicked(self.count_down)
counter = Counter()
counter.connect() #doesn't work inside class definition
plt.show()
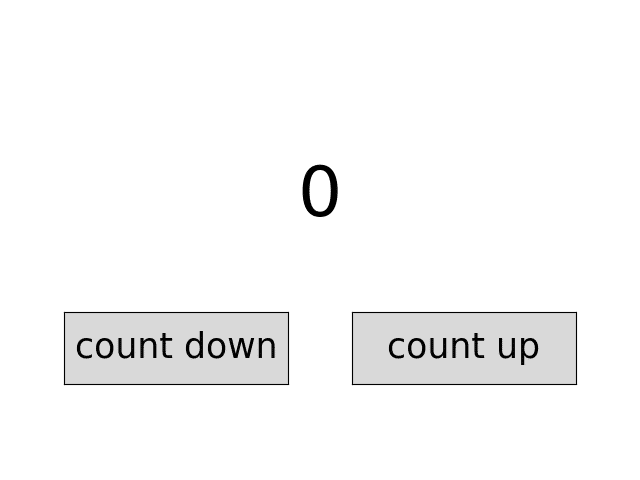
#"counter2.py"
import matplotlib.pyplot as plt
class Counter():
def __init__(self):
self.fig = plt.figure()
self.count = 0
fig = self.fig
self.text = fig.text(0.5,0.55,self.count,size = 50, ha="center")
self.up = fig.text(0.7,0.15," count up ",size=30,backgroundcolor="grey", ha="center")
self.down = fig.text(0.3,0.15,"count down",size=30,backgroundcolor="grey", ha="center")
fig.canvas.mpl_connect('button_press_event', self.on_click)
def on_click(self, event):
if self.up.contains(event)[0]:
self.count += 1
elif self.down.contains(event)[0]:
self.count -= 1
self.text.set_text(self.count)
self.fig.canvas.draw_idle()
counter = Counter()
plt.show()
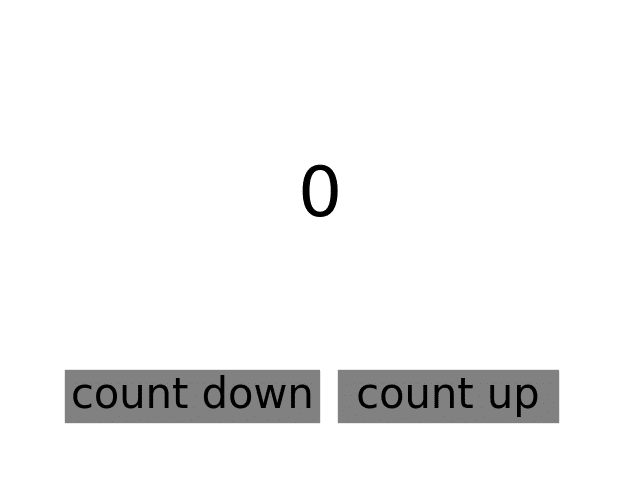
#"counter3.py"
import matplotlib.pyplot as plt
class Counter():
def __init__(self):
self.fig = plt.figure()
self.count = 0
fig = self.fig
self.text = fig.text(0.5,0.55,self.count,size = 50, ha="center")
self.up = fig.text(0.7,0.15," count up ", picker=True, size=30,backgroundcolor="grey", ha="center")
self.down = fig.text(0.3,0.15,"count down", picker=True, size=30,backgroundcolor="grey", ha="center")
fig.canvas.mpl_connect('pick_event', self.on_pick)
def on_pick(self, event):
if event.artist == self.up:
self.count += 1
elif event.artist == self.down:
self.count -= 1
self.text.set_text(self.count)
self.fig.canvas.draw_idle()
counter = Counter()
plt.show()
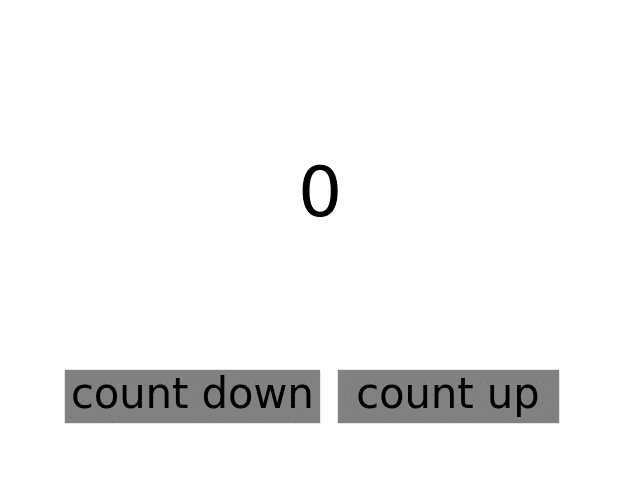
(見た目は counter2.py の結果と同じ)
参考URL:
https://matplotlib.org/stable/gallery/widgets/buttons.html
https://matplotlib.org/stable/users/explain/figure/event_handling.html
この記事が気に入ったらサポートをしてみませんか?